Clean and Scalable React Native Expo Project Structure for Modern Apps
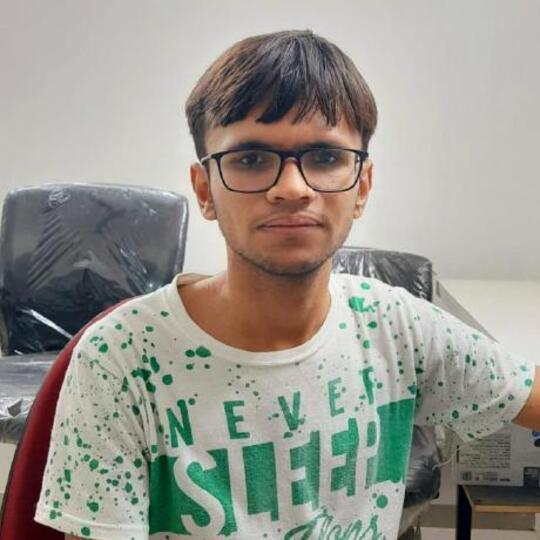
Table of contents

Introduction ๐
When building a React Native app with Expo, having a clean and scalable folder structure can make a huge difference. A well-organized project helps developers work efficiently, reduces bugs, and makes it easier to scale features in the future.
In this blog, Iโll guide you through:
A production-ready folder structure for React Native Expo.
How to organize your files and folders.
Best practices for building modern and scalable mobile apps.
Why a Clean Project Structure Matters ๐ ๏ธ
A messy project can lead to:
Difficulty finding files.
Duplicate code and components.
Confusion for team members or future developers.
With a clean folder structure, you get:
โ
Scalability: Add new features easily.
โ
Maintainability: Manage code efficiently.
โ
Reusability: Share components, constants, and logic.
A good folder structure saves time and makes your app development smoother!
Overview of the Folder Structure ๐๏ธ
root/
โโโ assets/ # Static assets like fonts, images, and icons.
โ โโโ fonts/ # Custom fonts (e.g., Roboto, OpenSans).
โ โโโ images/ # App images (e.g., product placeholders, logos).
โ โโโ icons/ # App icons (e.g., cart, profile icons).
โโโ src/ # Main source folder for all app logic.
โ โโโ app/ # Expo Router-based file system routing.
โ โ โโโ _layout.tsx # Root layout wrapping navigation logic.
โ โ โโโ index.tsx # Home screen showing featured products.
โ โ โโโ login/ # Screens for user login.
โ โ โโโ register/ # Screens for user registration.
โ โ โโโ products/ # Routes for product listings and details.
โ โ โ โโโ _layout.tsx # Layout wrapper for products.
โ โ โ โโโ index.tsx # Products listing screen.
โ โ โ โโโ [productId].tsx # Dynamic product detail page.
โ โ โโโ cart/ # Screens for shopping cart.
โ โ โโโ orders/ # User order history and details.
โ โ โโโ profile/ # User profile and settings screens.
โ โโโ components/ # Reusable UI components for consistency.
โ โ โโโ common/ # Common components (e.g., Button, Input, Loader).
โ โ โโโ ProductCard.tsx # Component to display product cards in list views.
โ โ โโโ CartItem.tsx # Component for individual cart items.
โ โโโ constants/ # App-wide constants for reusability.
โ โ โโโ colors.ts # Centralized color palette.
โ โ โโโ routes.ts # Route names for navigation.
โ โ โโโ messages.ts # Static messages or text content.
โ โโโ hooks/ # Custom hooks for logic abstraction.
โ โ โโโ useAuth.ts # Hook for handling authentication.
โ โ โโโ useCart.ts # Hook for cart-related logic.
โ โโโ redux/ # State management using Redux Toolkit.
โ โ โโโ store.ts # Redux store configuration.
โ โ โโโ slices/ # Individual Redux slices for state.
โ โ โ โโโ authSlice.ts # Auth state logic.
โ โ โ โโโ productSlice.ts # Product state logic.
โ โ โ โโโ cartSlice.ts # Cart state logic.
โ โโโ services/ # API services for network calls.
โ โ โโโ apiClient.ts # Axios instance with base URL.
โ โ โโโ productService.ts # APIs for product listings and details.
โ โ โโโ authService.ts # APIs for login and registration.
โ โโโ styles/ # Global and theme-based styles.
โ โ โโโ globalStyles.ts # Global reusable styles.
โ โ โโโ theme.ts # Theme configuration (colors, fonts, spacing).
โ โโโ types/ # TypeScript interfaces and types.
โ โ โโโ Product.ts # Product type definition.
โ โ โโโ Cart.ts # Cart item type definition.
โ โโโ utils/ # Utility functions for app logic.
โ โโโ helpers.ts # General helper functions.
โ โโโ formatCurrency.ts # Function to format currency values.
โโโ App.tsx # Entry point for the React Native app.
โโโ package.json # Project dependencies and scripts.
โโโ tsconfig.json # TypeScript configuration file.
Folder/File | Description | Example Content |
assets/ | Static resources like fonts, images, and icons. | logo.png , productPlaceholder.png . |
app/ | Expo Router-based folder for navigation and screens. | products/index.tsx , cart/index.tsx . |
components/ | Reusable UI components for buttons, inputs, cards, and lists. | Button.tsx , ProductCard.tsx . |
constants/ | Centralized app constants like colors, routes, and messages. | COLORS.primary , ROUTES.PRODUCTS . |
hooks/ | Custom hooks to abstract logic like authentication or cart management. | useAuth.ts , useCart.ts |
redux/ | State management setup using Redux Toolkit. | authSlice.ts , cartSlice.ts . |
services/ | API logic using Axios for interacting with backend services. | productService.ts , authService.ts |
styles/ | Global styles and themes for consistent UI design. | globalStyles.ts , theme.ts . |
types/ | TypeScript type definitions for API responses, components, or state. | Product.ts , Cart.ts . |
utils/ | Utility functions for formatting, validation, or common operations. | formatCurrency.ts , helpers.ts |
Detailed Explanation of Each Folder ๐
assets/ ๐จ
Purpose: File-based routing using Expo Router. Each folder represents a route.
โโโ app/
โ โโโ _layout.tsx # Root layout for navigation
โ โโโ index.tsx # Home screen
โ โโโ products/ # Product screens
โ โ โโโ index.tsx # Product list
โ โ โโโ [productId].tsx # Dynamic product details
app/ ๐ฑ
Purpose: File-based routing using Expo Router. Each folder represents a route.
โโโ app/
โ โโโ _layout.tsx # Root layout for navigation
โ โโโ index.tsx # Home screen
โ โโโ products/ # Product screens
โ โ โโโ index.tsx # Product list
โ โ โโโ [productId].tsx # Dynamic product details
components/ ๐งฉ
Purpose: Reusable UI components.
Example:
Button.tsx
(Custom Button component).ProductCard.tsx
(Reusable product card component).
constants/ ๐
Purpose: Centralized app constants (colors, routes, messages).
export const COLORS = {
primary: '#3498db',
secondary: '#2ecc71',
};
hooks/ ๐ฃ
Purpose: Custom React hooks to abstract logic.
Example:
useAuth.ts
(Handle user login/logout).useCart.ts
(Manage cart items).
redux/ ๐๏ธ
Purpose: State management using Redux Toolkit.
redux/
โโโ store.ts # Redux store
โโโ slices/
โ โโโ authSlice.ts # Authentication state
โ โโโ productSlice.ts # Product state
โ โโโ cartSlice.ts # Cart state
services/ ๐
Purpose: API services and network logic.
Example:
globalStyles.ts
(Reusable global styles).theme.ts
(Colors, font sizes, spacing).
types/ ๐
Purpose: TypeScript types for props, state, and API responses.
export interface Product {
id: string;
name: string;
price: number;
image: string;
}
utils/ ๐ ๏ธ
Purpose: Utility functions for common tasks.
Example:
formatCurrency.ts
(Format prices like $99.99
).
Key Best Practices ๐ก
Keep It Modular: Break code into reusable components.
Centralize Constants: Use constants for routes, colors, and messages.
State Management: Use Redux or Context API for managing app state.
TypeScript: Define types for props, states, and API responses.
Clean Code: Use tools like ESLint and Prettier for consistency.
Conclusion ๐
By following this clean and scalable project structure, you can:
โ
Build organized, maintainable apps.
โ
Scale your app effortlessly as it grows.
โ
Improve team collaboration and code quality.
Start implementing this in your React Native Expo projects today, and build apps that are production-ready and easy to maintain! ๐
Subscribe to my newsletter
Read articles from Nayan Radadiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
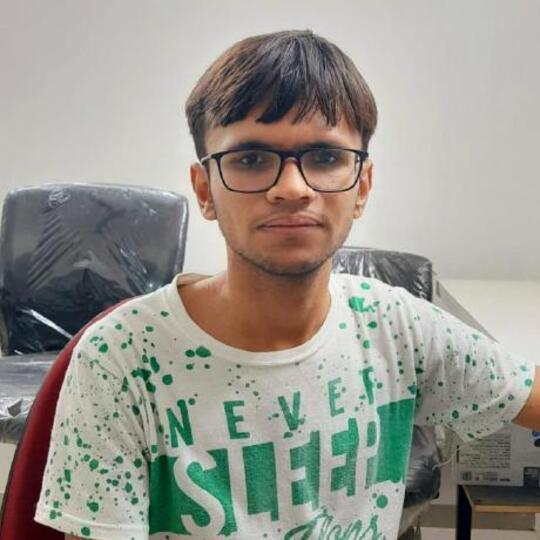
Nayan Radadiya
Nayan Radadiya
Experienced Frontend Developer with 2.5 years of expertise in Next.js, React.js, TypeScript, Javascript (ES6+), Redux, and React Native. Self-taught in blockchain development with a focus on pursuing a career as a Web3 Fronted developer. Proficient in reading smart contracts and integrating them with the frontend side.