Domain-Driven Design Error Handling Using Result Pattern
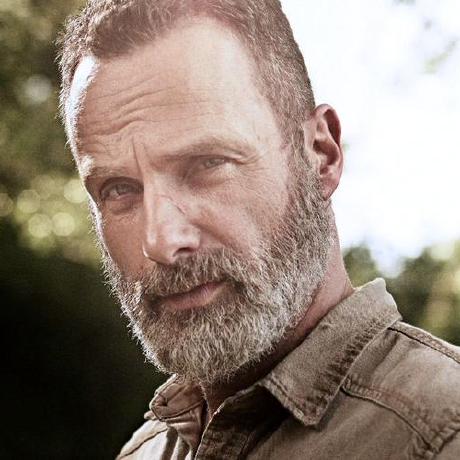
Introduction
When implementing Domain-Driven Design (DDD), one of the most challenging aspects is handling domain errors effectively. While traditionally, the approach has been to throw exceptions from the domain layer, this article presents a more elegant and efficient solution using the Result Pattern, which provides better separation of concerns and simplifies internationalization.
The Traditional Approach and Its Problems
Traditionally, domain errors in DDD were handled by throwing exceptions:
public class Order
{
public void AddItem(Product product, int quantity)
{
if (quantity <= 0)
throw new DomainException("Invalid quantity for order item");
if (product == null)
throw new DomainException("Product cannot be null");
// Process order item
}
}
This approach has several drawbacks:
Exceptions are expensive in terms of performance
Domain layer becomes tightly coupled to presentation concerns (error messages)
Internationalization becomes complex
Multiple validation errors cannot be returned simultaneously
A Better Solution: Result Pattern with Error Codes
Let's implement a more elegant solution using Result Pattern combined with error codes:
1. First, define your error codes:
public static class OrderErrors
{
public const string InvalidQuantity = "ORDER.INVALID_QUANTITY";
public const string ProductRequired = "ORDER.PRODUCT_REQUIRED";
public const string InsufficientStock = "ORDER.INSUFFICIENT_STOCK";
}
2. Create a Result class (or use Ardalis.Result):
public class Result<T>
{
public bool IsSuccess { get; }
public T Value { get; }
public List<ErrorDetail> Errors { get; }
private Result(bool isSuccess, T value, List<ErrorDetail> errors)
{
IsSuccess = isSuccess;
Value = value;
Errors = errors;
}
public static Result<T> Success(T value) =>
new Result<T>(true, value, new List<ErrorDetail>());
public static Result<T> Failure(List<ErrorDetail> errors) =>
new Result<T>(false, default, errors);
}
public class ErrorDetail
{
public string Code { get; }
public string Field { get; }
public ErrorDetail(string code, string field = null)
{
Code = code;
Field = field;
}
}
3. Implement domain logic using Result Pattern:
public class Order
{
public Result<OrderItem> AddItem(Product product, int quantity)
{
var errors = new List<ErrorDetail>();
if (quantity <= 0)
errors.Add(new ErrorDetail(OrderErrors.InvalidQuantity, nameof(quantity)));
if (product == null)
errors.Add(new ErrorDetail(OrderErrors.ProductRequired, nameof(product)));
if (errors.Any())
return Result<OrderItem>.Failure(errors);
var orderItem = new OrderItem(product, quantity);
return Result<OrderItem>.Success(orderItem);
}
}
4. Handle errors in the presentation layer:
[ApiController]
public class OrderController : ControllerBase
{
private readonly IOrderService _orderService;
private readonly IErrorMessageService _errorMessageService;
[HttpPost("items")]
public async Task<IActionResult> AddOrderItem(AddOrderItemRequest request)
{
var result = await _orderService.AddOrderItem(request.ProductId, request.Quantity);
if (!result.IsSuccess)
{
var errorResponse = result.Errors.Select(error => new
{
Field = error.Field,
Message = _errorMessageService.GetMessage(error.Code,
HttpContext.Request.Headers["Accept-Language"])
});
return BadRequest(errorResponse);
}
return Ok(result.Value);
}
}
5. Implement error message service for localization:
public interface IErrorMessageService
{
string GetMessage(string errorCode, string language);
}
public class ErrorMessageService : IErrorMessageService
{
private readonly IStringLocalizer _localizer;
public string GetMessage(string errorCode, string language)
{
// Use string localizer or resource files to get localized message
return _localizer[errorCode, language];
}
}
Benefits of This Approach
Separation of Concerns
Domain layer focuses on business rules and returns only error codes
Presentation layer handles message formatting and localization
Clear separation between validation logic and error presentation
Improved Performance
Avoids the overhead of exception handling
Allows for bulk validation and returning multiple errors
Better Localization Support
Error messages are managed in one place
Easy to add new languages without modifying domain code
Consistent error message formatting across the application
Enhanced Maintainability
Error codes are centralized and documented
Easy to modify error messages without touching domain logic
Clear audit trail of possible error conditions
Conclusion
This approach to error handling in DDD offers significant advantages over traditional exception-based approaches. By using the Result Pattern with error codes, we achieve:
Better separation of concerns
Improved performance
Simplified internationalization
More maintainable codebase
Better developer experience
The key insight is recognizing that while the domain layer should identify what went wrong, it shouldn't be responsible for how that error is presented to the user. This separation allows for more flexible error handling and easier maintenance of the application.
Next Steps
Consider implementing this pattern with some additional enhancements:
Use Ardalis.Result for a more robust implementation
Add error severity levels (Error, Warning, Info)
Implement error logging and monitoring
Add support for error parameters in localization
Create middleware for consistent error handling
Subscribe to my newsletter
Read articles from Rick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
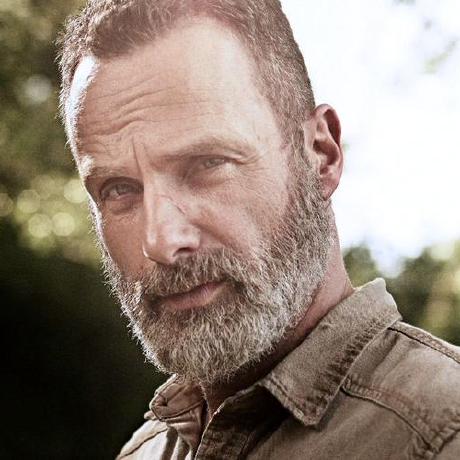
Rick
Rick
15+ years of experience having fun building apps with .NET I began my professional career in 2006, using Microsoft technologies where C# and Windows Forms and WPF were the first technologies I started working with during that time. I had the opportunity to actively participate in the Windows ecosystem as an MVP and Windows 8/Windows Phone application developer from 2013-2018. Throughout my career, I have used Azure as my default cloud platform and have primarily worked with technologies like ASP.NET Core for multiple companies globally across the US, UK, Korea, Japan, and Latin America. I have extensive experience with frameworks such as: ASP.NET Core Microsoft Orleans WPF UWP React with TypeScript Reactive Extensions Blazor I am an entrepreneur, speaker, and love traveling the world. I created this blog to share my experience with new generations and to publish all the technical resources that I had been writing privately, now made public as a contribution to enrich the ecosystem in which I have developed my career.