Exploring the Power of ObjectDumper in C#
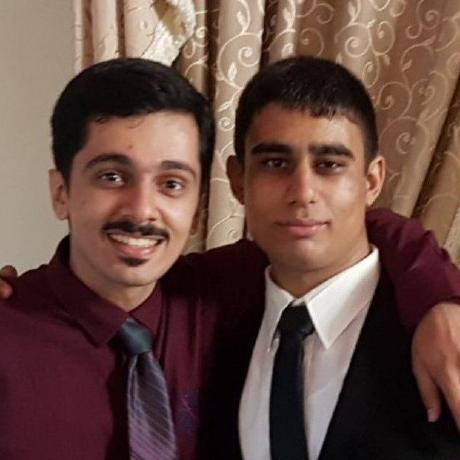

What is ObjectDumper?
ObjectDumper is a utility package available on GitHub, specifically designed for C# developers. It's a tool that serializes C# objects into strings for purposes like debugging and logging. The package can be easily added to any .NET project through NuGet, making it accessible for developers working on diverse platforms including Xamarin Android, iOS, Windows Phone, and others. ObjectDumper provides two primary serialization styles: DumpStyle.Console for human-readable logs and DumpStyle.CSharp for generating C# initializer code.
How Can ObjectDumper Help in Debugging and Testing?
Debugging and testing in C# often involve inspecting complex object states. Here's how ObjectDumper can enhance these processes:
Visibility: It allows developers to see the full structure of an object including nested properties, which can be particularly useful when dealing with complex data models or when debugging issues in data transformation pipelines.
Serialization: By converting objects to human-readable strings or C# code, ObjectDumper makes it easier to track changes in object states over time, helping in identifying where and why unexpected changes occur.
Logging: It can be used to log object states at different stages of program execution, providing a detailed log for troubleshooting or for reproducing issues in different environments.
Testing: When writing unit tests, especially for serialization or deserialization, ObjectDumper can quickly verify the contents of objects without writing extensive custom assertions for each property.
A Simple Project Using ObjectDumper
Let's create a small C# console application to demonstrate how ObjectDumper works:
Setup: First, install the ObjectDumper package via NuGet:
Install-Package ObjectDumper.NET
Create a Sample Object:
using System; using System.Collections.Generic; using ObjectDumper.NET; class Person { public string Name { get; set; } public int Age { get; set; } } class Program { static void Main(string[] args) { List<Person> persons = new List<Person> { new Person { Name = "John", Age = 20 }, new Person { Name = "Thomas", Age = 30 } }; // Dump the list of persons to console output var personsDump = ObjectDumper.Dump(persons); Console.WriteLine(personsDump); // Keep console window open Console.ReadLine(); } }
This code will output each Person object's properties in a readable format to the console.
Execution: Running this program will display:
{Person} Name: "John" Age: 20 {Person} Name: "Thomas" Age: 30
This example shows how simple it is to use ObjectDumper to visualize complex structures directly in your console application or any other logging mechanism.
In the end
ObjectDumper is an invaluable tool for any C# developer focused on debugging or needing to log complex object structures. Its ability to quickly serialize objects into readable formats or C# code snippets not only speeds up the debugging process but also helps in maintaining clean and understandable logs. Whether you're testing new features or diagnosing issues in existing code, integrating ObjectDumper into your workflow can significantly improve your productivity and clarity in understanding object states. For any developer looking to streamline their debugging and testing, ObjectDumper is certainly a package worth exploring.
If you have any questions about it you can ask them in the comments.
Subscribe to my newsletter
Read articles from behrooz bozorgchamy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
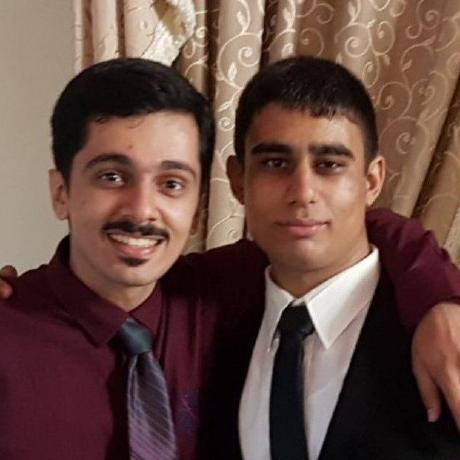