The Future of DevSecOps
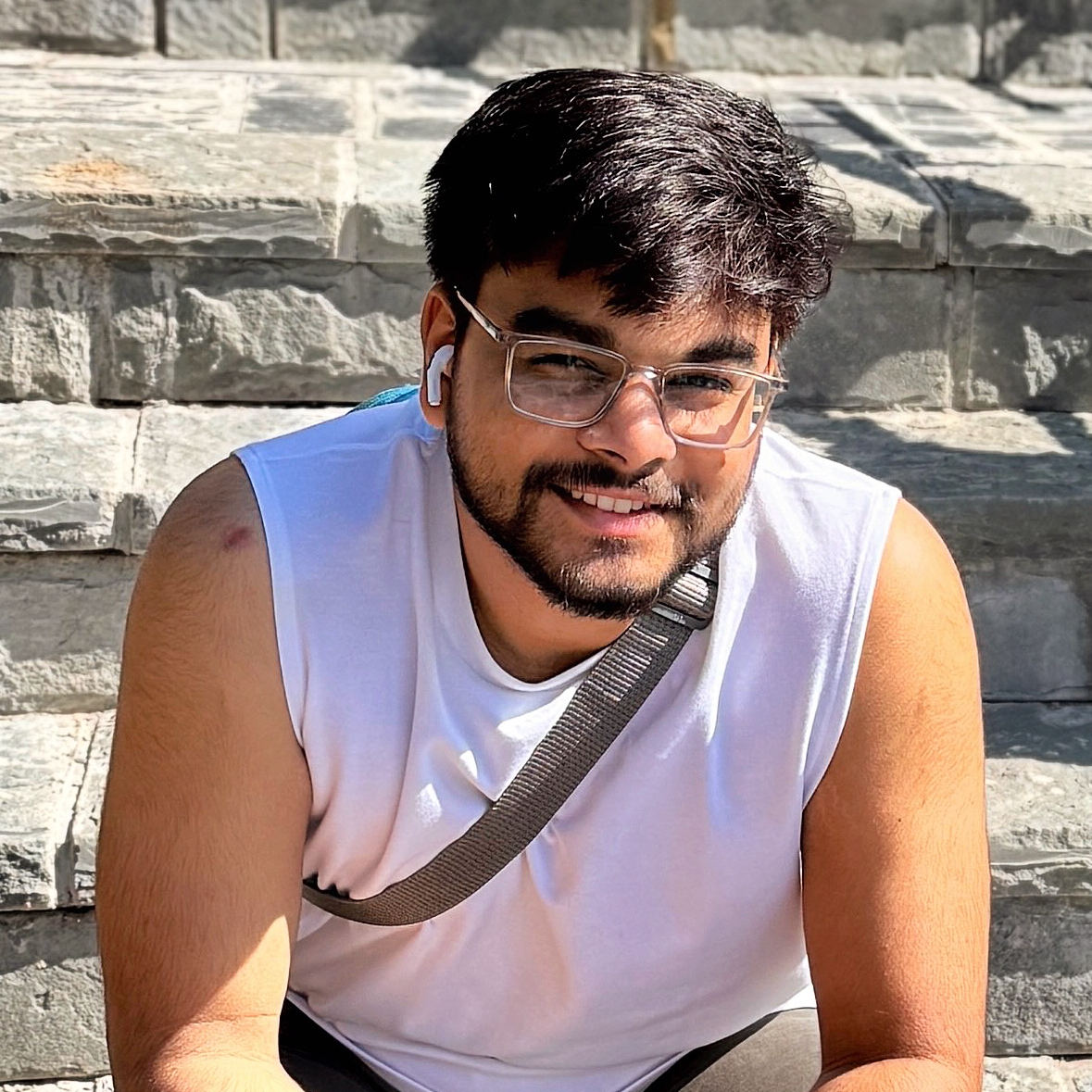

In today’s hyper-connected world, building software often involves diverse, distributed teams collaborating across multiple organizations. Traditional DevSecOps pipelines rely on centralized tools and services, which can introduce single points of failure, opaque audit trails, or the possibility of tampering. Enter Decentralized DevSecOps Pipelines where blockchain (or other decentralized infrastructure) brings verifiability, immutability, and multi-party trust to the entire build, test, and deploy cycle.
1. Why Decentralized DevSecOps?
Orchestrating DevSecOps workflows on a blockchain ensures that every step, from code commit to deployment, is both traceable and tamper-evident. Each pipeline stage writes transaction data (e.g., test reports, security scans) onto a shared, decentralized ledger. This ledger, governed by smart contracts, enforces security policies and automates collaboration among multiple parties without a central authority.
Why It’s Unique:
Immutable Audit Trails: Every security check like static code analysis or vulnerability scanning is verified and recorded on-chain.
Multi-Party Governance: Multiple stakeholders (e.g., engineering organizations, suppliers in a supply chain, or financial consortia) can collectively govern DevSecOps policies without relying on a single, centralized authority.
End-to-End Security: Blockchain-based triggers ensure that unauthorized code cannot progress to the next stage of the pipeline. Tampering or skipping steps becomes virtually impossible.
Potential Use Cases:
Highly Distributed Engineering Organizations: Teams spread across regions can collaborate with full trust and traceability.
Consortia (Supply Chain, Financial, etc.): Multiple companies jointly develop or maintain a codebase and want verifiable proof of compliance, testing, and security reviews.
2. Real-World Example: Supply Chain Consortium
Imagine a consortium of manufacturers building a shared software platform for tracking product inventory and shipping details. Each manufacturer has its own DevSecOps environment, but they want a single pipeline that enforces the same security standards for all. They set up a private blockchain network (e.g., using Hyperledger Fabric) where each manufacturer runs a node. The DevSecOps pipeline pushes each code commit’s status, security scan results, and final deployment attestations to the blockchain.
Distributed Code Ownership: Multiple organizations contribute code modules.
Tamper-Evident Security Tests: Security scans (e.g., SAST, DAST) run automatically, with results and checksums published to the ledger.
Shared Governance: Each organization’s node can independently verify that no step is skipped or faked.
Instant Compliance: Auditors or regulators can query the blockchain for a clear history of security validations, drastically reducing compliance overhead.
3. High-Level Architecture
flowchart LR
A[Developers] -->|Push Code| B[Git Repository]
B -->|Trigger Build| C[CI/CD Orchestrator]
C -->|Run Tests & Security Scans| D[Testing & Security Services]
D -->|Write Results Hash| E[Blockchain/Smart Contracts]
E -->|Verify & Update Pipeline Status| C
C -->|Trigger Deployment| F[Deployment Environment]
F -->|Deployment Status| E
Explanation of Key Components:
Git Repository: Developers commit code here.
CI/CD Orchestrator: Automatically detects new commits, orchestrates pipeline tasks.
Testing & Security Services: Executes unit tests, integration tests, security scans (static/dynamic analysis).
Blockchain/Smart Contracts: Stores and verifies pipeline metadata (test results hashes, security policies).
Deployment Environment: Could be a Kubernetes cluster, on-premises servers, or cloud infrastructure.
4. Implementation Strategy
4.1 Set Up a Blockchain Network
Choose a Framework: Ethereum, Polygon, or Hyperledger Fabric are popular for private/permissioned setups.
Configure Nodes: Each stakeholder runs their own node. This ensures decentralized governance and eliminates the single point of failure.
Deploy Smart Contracts/Chaincode: The contract enforces pipeline policies (e.g., “Build must pass all security checks before deployment”).
Hyperledger Fabric Example
Install Fabric binaries:
curl -sSL https://bit.ly/2ysbOFE | bash -s
Generate crypto materials and bring up the network:
cd fabric-samples/test-network ./network.sh up createChannel -c mychannel ./network.sh deployCC -ccn devsecops -ccp ../chaincode/ -ccl go
This starts a sample Fabric network with a channel named
mychannel
and deploys the chaincode nameddevsecops
.
4.2 Smart Contract for DevSecOps
Below is a simplified Go chaincode example for Hyperledger Fabric that logs and enforces pipeline steps.
package main
import (
"encoding/json"
"fmt"
"github.com/hyperledger/fabric-contract-api-go/contractapi"
)
type DevSecOpsChaincode struct {
contractapi.Contract
}
// PipelineRecord represents a record of a pipeline execution
type PipelineRecord struct {
CommitID string `json:"commitID"`
Stage string `json:"stage"`
Status string `json:"status"`
SecurityCheck bool `json:"securityCheck"`
}
func (d *DevSecOpsChaincode) RecordStage(ctx contractapi.TransactionContextInterface, commitID string, stage string, status string, securityCheck bool) error {
record := PipelineRecord{
CommitID: commitID,
Stage: stage,
Status: status,
SecurityCheck: securityCheck,
}
recordAsBytes, _ := json.Marshal(record)
key := fmt.Sprintf("%s-%s", commitID, stage)
return ctx.GetStub().PutState(key, recordAsBytes)
}
func (d *DevSecOpsChaincode) GetStage(ctx contractapi.TransactionContextInterface, commitID, stage string) (*PipelineRecord, error) {
key := fmt.Sprintf("%s-%s", commitID, stage)
data, err := ctx.GetStub().GetState(key)
if err != nil {
return nil, err
}
if data == nil {
return nil, fmt.Errorf("Record not found")
}
var record PipelineRecord
_ = json.Unmarshal(data, &record)
return &record, nil
}
func main() {
chaincode, err := contractapi.NewChaincode(new(DevSecOpsChaincode))
if err != nil {
panic("Could not create chaincode from DevSecOpsChaincode")
}
if err := chaincode.Start(); err != nil {
panic("Failed to start chaincode")
}
}
Explanation:
RecordStage
writes a pipeline’s stage result to the ledger (e.g., “test passed,” “security check passed”).GetStage
queries the ledger to retrieve the status.You can expand this to enforce business logic (e.g., reject deployments if
securityCheck
isfalse
).
4.3 Integrate with CI/CD (e.g., GitHub Actions)
Pipeline Setup: In your
.github/workflows/ci.yml
, configure steps that call the chaincode using the Fabric SDK.On Build Completion: After your build passes, run a script to record the status:
- name: Record build status in Fabric run: | ./fabric-cli recordStage --commitID ${{ github.sha }} --stage "build" --status "SUCCESS" --securityCheck=false
Security Scan: Integrate tools like Trivy, SonarQube, or OWASP ZAP:
- name: Security Scan run: | trivy fs . # After scanning, record result in blockchain - name: Record security status run: | ./fabric-cli recordStage --commitID ${{ github.sha }} --stage "security-scan" --status "SUCCESS" --securityCheck=true
Deployment Step: The pipeline can query the chaincode to verify all prior steps have passed:
- name: Verify pipeline status run: | # Pseudocode - calls chaincode to ensure all stages have 'SUCCESS' and securityCheck == true if ! ./fabric-cli verifyPipeline --commitID ${{ github.sha }}; then echo "Pipeline did not pass all checks!" exit 1 fi - name: Deploy to Production run: | # e.g., helm upgrade, kubectl apply, etc. kubectl apply -f deployment.yaml
4.4 Testing & Validation
Local Unit Tests: Ensure your chaincode logic is correct.
Integration Tests: Spin up a local Fabric network (e.g., using Docker Compose) and run end-to-end tests that push a commit, trigger the pipeline, store records in the ledger, and confirm retrieval.
Security Hardening: Use authorized identities (MSP in Fabric) for chaincode operations. Confirm that only valid pipeline accounts can update or read pipeline status.
5. Roadmap
Provision the Blockchain: Set up a permissioned network (e.g., Hyperledger Fabric).
Deploy Smart Contract: Implement chaincode that logs pipeline data.
CI/CD Integration: Configure your pipeline to:
Run build/tests.
Publish results (stage status) to the blockchain.
Enforce security checks.
Deployment Gate: Before deployment, verify on-chain that all previous checks are green.
Audit & Monitor: Continuously log pipeline events to the ledger for easy compliance and historical reporting.
6. Conclusion & Future Directions
By merging DevSecOps best practices with decentralized infrastructure, organizations can:
Eliminate Single Points of Failure: No single entity can tamper with or hide a failed security scan.
Improve Multi-Party Trust: Each stakeholder can independently verify the pipeline integrity.
Enhance Compliance: Blockchain-based logs create an immutable audit trail for regulators and auditors.
Next Steps might include integrating advanced features like on-chain vulnerability scoring, automated patching triggers, or using distributed oracles for real-time threat intelligence. The future of secure, multi-organization software delivery is bright and decentralized.
Further Reading
Hyperledger Fabric Documentation: hyperledger-fabric.readthedocs.io
GitHub Actions: docs.github.com/actions
OWASP ZAP: https://www.hackerone.com/knowledge-center/owasp-zap
Give this decentralized pipeline a try and experience how seamless and secure next-generation DevSecOps can be!
Subscribe to my newsletter
Read articles from Subhanshu Mohan Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
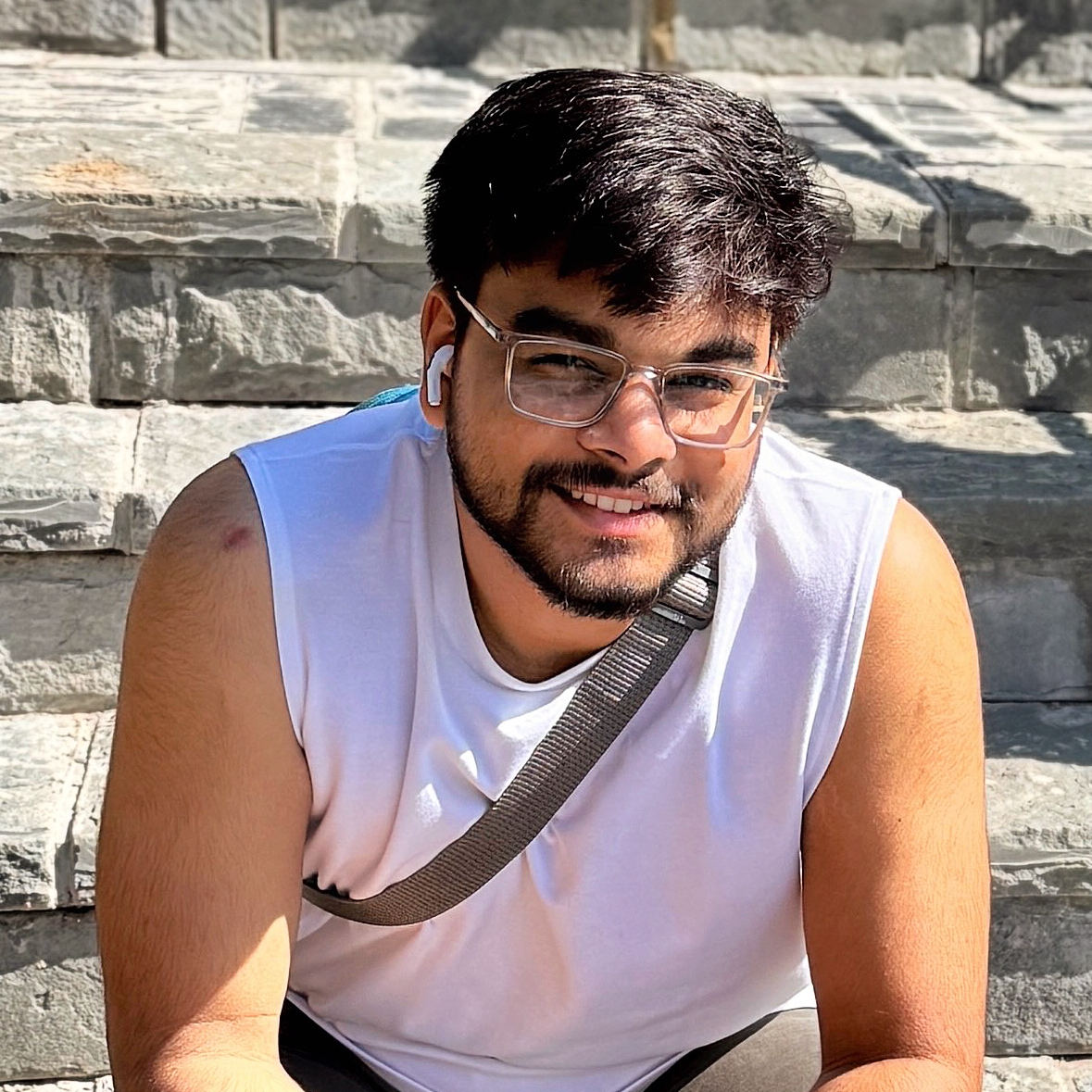
Subhanshu Mohan Gupta
Subhanshu Mohan Gupta
A passionate AI DevOps Engineer specialized in creating secure, scalable, and efficient systems that bridge development and operations. My expertise lies in automating complex processes, integrating AI-driven solutions, and ensuring seamless, secure delivery pipelines. With a deep understanding of cloud infrastructure, CI/CD, and cybersecurity, I thrive on solving challenges at the intersection of innovation and security, driving continuous improvement in both technology and team dynamics.