How I made a basic email contact form with SendLayer API and Cloudflare Pages

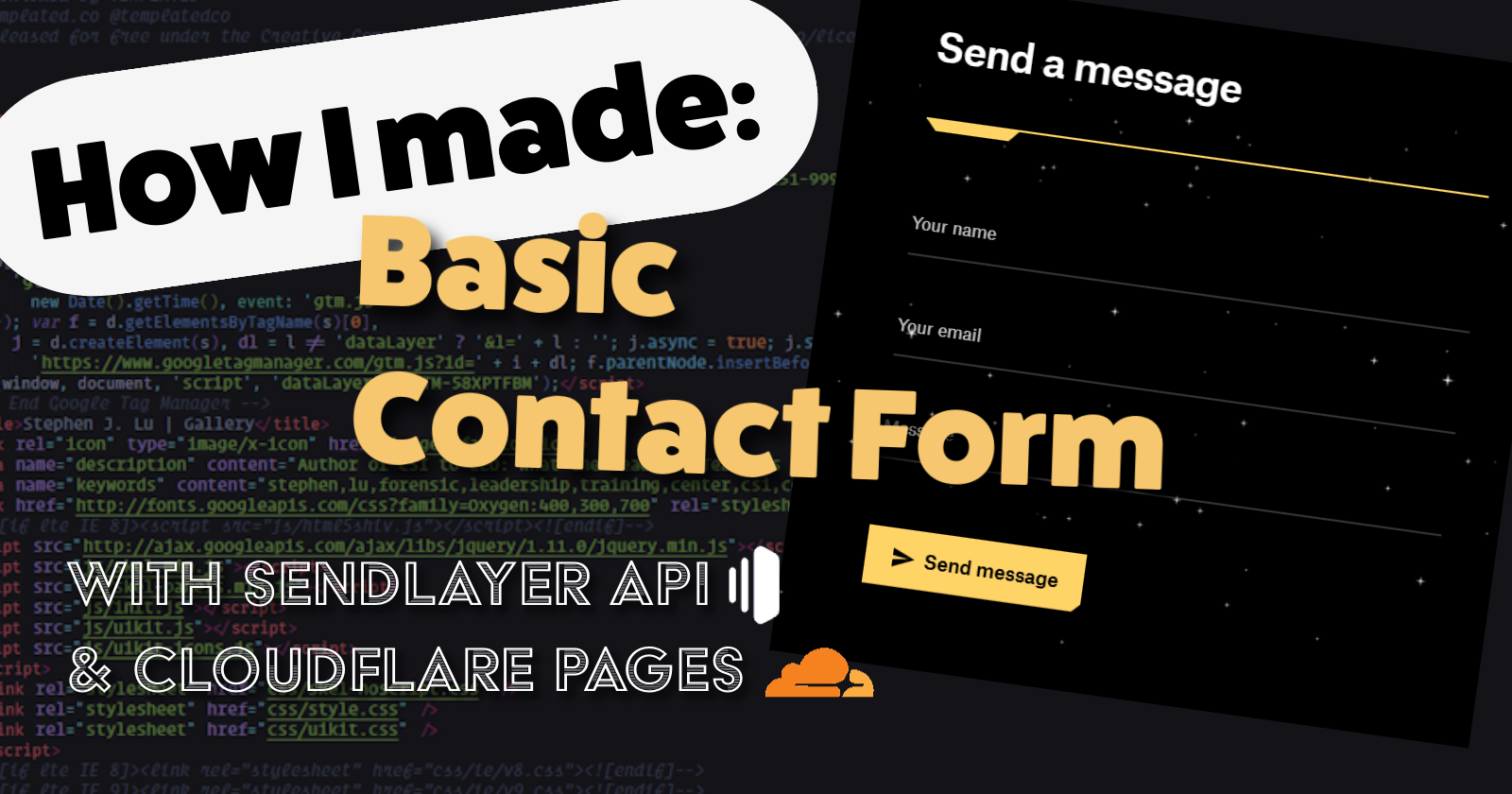
This article outlines the process of integrating a basic contact form on a website using the SendLayer API and Cloudflare Pages. It explains how to create a SendLayer API key, configure an environment variable in Cloudflare, and connect the app to the variable. The guide provides code snippets for implementing the form and emphasizes the importance of keeping sensitive information secure.
The Idea
I wanted to add a basic contact form to my website using SendLayer API. I already use Cloudflare Pages to serve my websites, so the integration using environment variables was a nice benefit, and made the integration a lot easier. Here’s how I did it in three simple steps.
Note: If you’re looking to create your own form, you can use the same method with any service that provides email delivery via API. Just make sure that you meet the payload requirements when your app POSTs or otherwise.
The Execution
Step One: Create a SendLayer API Key
Head over to your SendLayer settings to create a new API key for your application.
Step Two: Set Up an Environment Variable in Cloudflare
Head over to Cloudflare Pages, select your project, then go to Settings. Under Variables and Secrets, click on +Add
to add a new secret. Make sure that it matches the API from SendLayer, with no extra spaces or characters.
After you set up an environment variable, you’ll need to create a deployment in order to make it effective. I recommend checking the deployment details for your variable before implementing your app.
Step Three: Link Your App with the Environment Variable
In your code, you’ll need to insert a reference to Cloudflare’s environment variables to access the secret. In my case, Cloudflare Pages is on a Remix framework, so I had @remix-run/cloudflare
available to use.
Here is the primary action function:
/* example-contact-form.tsx
...
Imports as necessary
...
*/
import { json } from '@remix-run/cloudflare';
/* Primary action function */
export async function action ({ request, context }:
{ request: Request, context: any }) {
/* SendLayer's API Endpoint. Adjust based on documentation */
const sendLayerEndpoint = 'https://console.sendlayer.com/api/v1/email';
/*...
...
...*/
try {
const response = await fetch(sendLayerEndpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
/* Retrieving the environment variable here */
'Authorization': `Bearer ${context.cloudflare.env.SL_API_KEY}`,
},
Then, the JSON payload:
/* Make sure your payload meets the requirements.
This particular example meets SendLayer's
required payload */
body: JSON.stringify({
"from": {
"name": "Example Contact Form Submission",
"email": "no-reply@example.com"
},
"to": [
{
"name": "John Doe",
"email": "john@example.com"
}
],
"subject": "New Contact Form Submission",
"ContentType": "HTML",
"HTMLContent": `<html><body>
<p>You have a new contact form submission:</p>
<p><strong>Name:</strong> ${name}</p>
<p><strong>Email:</strong> ${email}</p>
<p><strong>Message:</strong><br/>${message}</p>
</body></html>`,
"PlainContent": `You have a new contact form submission:
Name: ${name}
Email: ${email}
Message:
${message}`,
"Tags": [
"testing",
"example contact form"
],
"Headers": {
"X-Mailer": "example.com",
"X-Test": "test headers"
}
}),
});
Then error handling and console checks for development. At the end is your contact form rendering, as you will.
/* Error handling and console checks */
if (!response.ok) {
const errorData = await response.json();
console.error('SendLayer Error:', errorData);
return json({ error: 'Failed to send email.
Please try again later.' }, { status: 500 });
}
console.log('Received POST request');
console.log('Name:', name);
console.log('Email:', email);
console.log('Message:', message);
return json({ success: true }, { status: 200 });
} catch (error) {
console.error('Error sending email:', error);
return json({ error: 'An unexpected error occurred.' },
{ status: 500 });
}
};
/* And now, your contact form itself! */
export const Contact = () => {
//...
}
Fin…
That’s it! Three easy steps. If you want to test your app before going live, you can create a .dev.vars
file in the root folder of your project.
Remember .gitignore .dev.vars! You don’t want to expose secrets.
//dev.vars//
SL_API_KEY=ENTER_YOUR_SECRET
If you want to see it in action, head over to my website, https://www.StephenJLu.com/contact, and send me a quick hello to tell me it worked ;)
Subscribe to my newsletter
Read articles from Stephen J. Lu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Stephen J. Lu
Stephen J. Lu
Stephen has studied everything from mosquitoes and disease biology to bloodstain patterns, bullet trajectories, and digging up clandestine graves.