Is the Model making right predictions? - Part 5 of 5 on Evaluation of Machine Learning Models

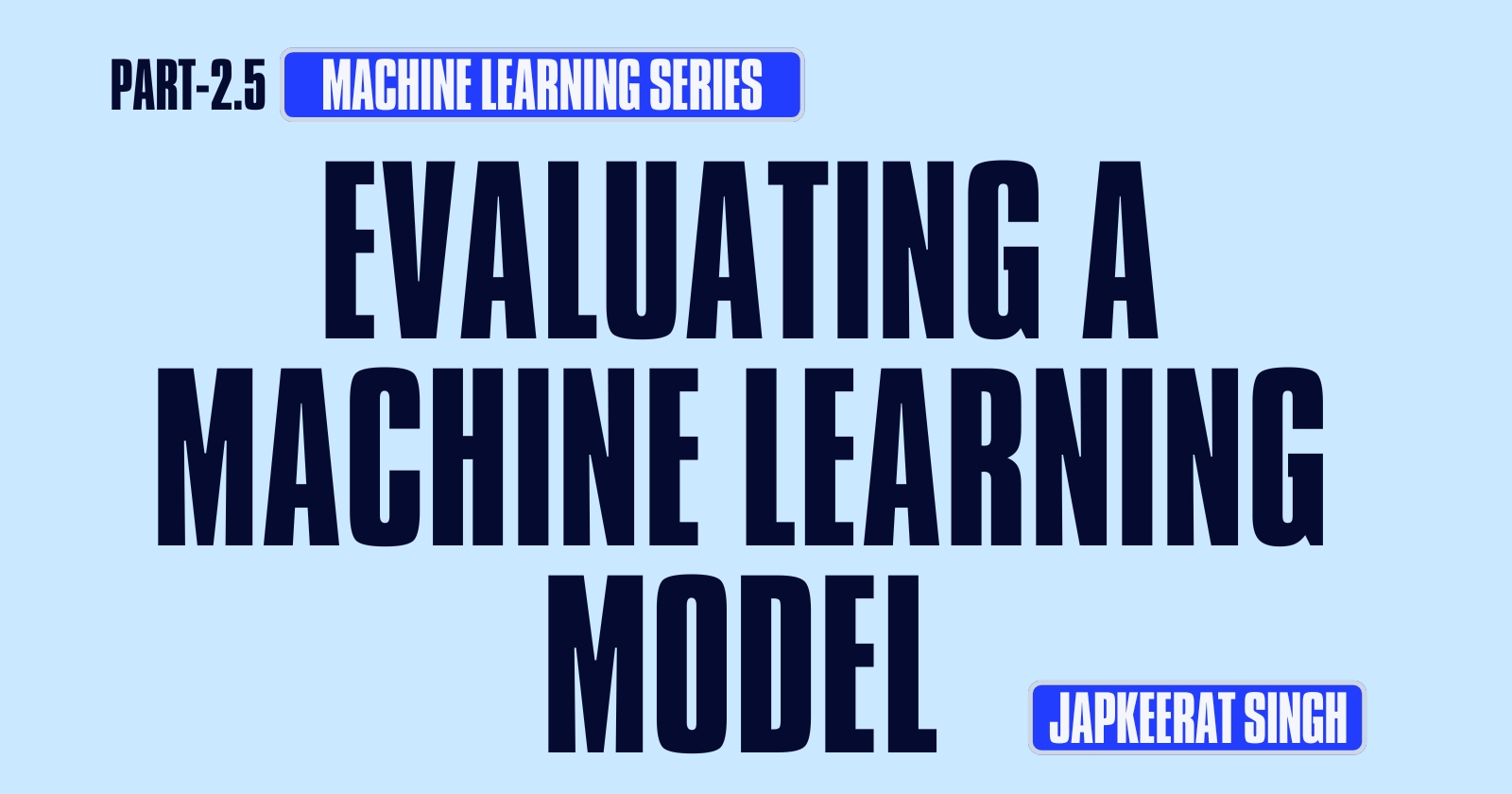
Preparing your dataset, especially the test set, is a crucial step in building reliable and high-performing machine learning models. Proper dataset preparation ensures that your model is not only accurate but also generalizable to new, unseen data. Let’s delve into the various techniques and best practices to achieve this effectively.
Train-Test Split: The Basics
The train-test split is a fundamental step in machine learning workflows. It divides your data into two parts:
Training Set: This portion is used to train the model. The model learns patterns and relationships from this data.
Test Set: This part is reserved for evaluating the model’s performance. It simulates how the model will behave on unseen data.
Python’s scikit-learn
library provides a simple way to perform this split:
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
In this example, 30% of the data is set aside for testing (test_size=0.3
). Setting a random_state
ensures reproducibility. The train-test split is critical for assessing whether your model is overfitting or generalizing well.
Time Series Data: Handle with Care
Time series data requires a different approach because the order of observations carries meaningful information. Randomly shuffling the data can break the temporal patterns, leading to unreliable evaluations. For instance, testing a model on past data after training it on future data doesn’t reflect real-world scenarios.
When working with time series data, it’s essential to maintain the chronological order. Train the model on historical data and evaluate it on future data. This ensures that the model’s predictions are based on past trends.
In scikit-learn
, the TimeSeriesSplit
class facilitates this type of split:
from sklearn.model_selection import TimeSeriesSplit
tscv = TimeSeriesSplit(n_splits=5)
for train_index, test_index in tscv.split(X):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
This method allows for multiple train-test splits while preserving the temporal order, providing a robust way to evaluate time series models.
K-Fold Cross-Validation: A Comprehensive Evaluation
K-Fold Cross-Validation is an effective technique for evaluating model performance. It works by dividing the dataset into ‘k’ subsets (folds). The model is trained on ‘k-1’ folds and tested on the remaining fold. This process repeats ‘k’ times, with each fold serving as the test set once. The results are then averaged to provide an overall performance metric.
Here’s how to implement K-Fold Cross-Validation in Python:
from sklearn.model_selection import KFold
kf = KFold(n_splits=5, shuffle=True, random_state=42)
for train_index, test_index in kf.split(X):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
K-Fold Cross-Validation reduces the risk of overfitting and ensures that the model is evaluated across multiple data partitions. This method is particularly useful for smaller datasets where reserving a large test set isn’t feasible.
Stratified K-Fold: Fair Evaluation for Imbalanced Data
When dealing with imbalanced datasets—where some classes are underrepresented—it’s important to ensure that the train and test sets have the same class distribution as the entire dataset. Stratified K-Fold Cross-Validation addresses this issue by maintaining class proportions in each fold.
Here’s how to implement it:
from sklearn.model_selection import StratifiedKFold
skf = StratifiedKFold(n_splits=5, shuffle=True, random_state=42)
for train_index, test_index in skf.split(X, y):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
Stratified K-Fold ensures fair representation of all classes in both training and testing phases. This leads to more reliable and unbiased performance metrics, especially in classification tasks.
Domain-Specific Splits: Tailored Testing
For certain types of data, domain-specific considerations are crucial when preparing the test set. For instance:
Spatial Data: For geospatial datasets, it’s often better to split data based on regions. For example, you might train a model on data from one geographic area and test it on another to evaluate how well the model generalizes across locations.
Demographic Data: For human-centered applications, splitting data by demographic groups can highlight biases or ensure fairness. For example, testing a healthcare model separately on age groups can reveal disparities in predictions.
Event-Specific Data: In event-driven datasets (e.g., sports or financial markets), splitting by events can ensure that the model is evaluated on entirely different scenarios.
These approaches ensure the test set reflects real-world variability and allows for a more robust evaluation.
Data Augmentation for Robust Evaluation
Data augmentation is a technique commonly used in training, but it’s also valuable for testing. By applying transformations to the test data, you can assess the robustness of your model under different scenarios. For instance:
Computer Vision: Apply augmentations such as rotations, translations, or noise to test images and evaluate if the model’s predictions remain consistent.
Natural Language Processing: Add variations like synonym replacement, misspellings, or paraphrasing to test the resilience of language models.
Audio Processing: Add background noise or change the pitch in audio test data to see how well the model adapts to real-world distortions.
Data augmentation during testing is particularly useful when you want to simulate challenging conditions or validate robustness beyond ideal scenarios.
Why Proper Dataset Preparation Matters
Proper dataset preparation directly impacts the reliability and generalizability of your machine learning models. Here are a few reasons why it’s critical:
Avoid Overfitting: Using separate datasets for training and testing prevents the model from memorizing the data rather than learning patterns.
Realistic Evaluation: Testing on unseen data mimics real-world scenarios, providing a realistic measure of the model’s performance.
Fair Metrics: Techniques like Stratified K-Fold ensure that performance metrics are not biased due to class imbalances.
Respect Temporal Patterns: For time series data, maintaining the order of observations leads to more meaningful evaluations.
Domain Relevance: Tailored splits and augmented testing ensure the model’s performance is validated in realistic and varied conditions.
Conclusion
Dataset preparation is not just a preliminary step—it’s a cornerstone of machine learning. By applying techniques like train-test splits, time series-specific methods, K-Fold Cross-Validation, Stratified K-Fold, domain-specific splits, and data augmentation, you can build models that are both accurate and generalizable. Investing time in preparing your data ensures that your models are reliable and ready to tackle real-world challenges with confidence.
Subscribe to my newsletter
Read articles from Japkeerat Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Japkeerat Singh
Japkeerat Singh
Hi, I am Japkeerat. I am working as a Machine Learning Engineer since January 2020, straight out of college. During this period, I've worked on extremely challenging projects - Security Vulnerability Detection using Graph Neural Networks, User Segmentation for better click through rate of notifications, and MLOps Infrastructure development for startups, to name a few. I keep my articles precise, maximum of 4 minutes of reading time. I'm currently actively writing 2 series - one for beginners in Machine Learning and another related to more advance concepts. The newsletter, if you subscribe to, will send 1 article every Thursday on the advance concepts.