API Stress Test with Grafana K6 on Google Cloud
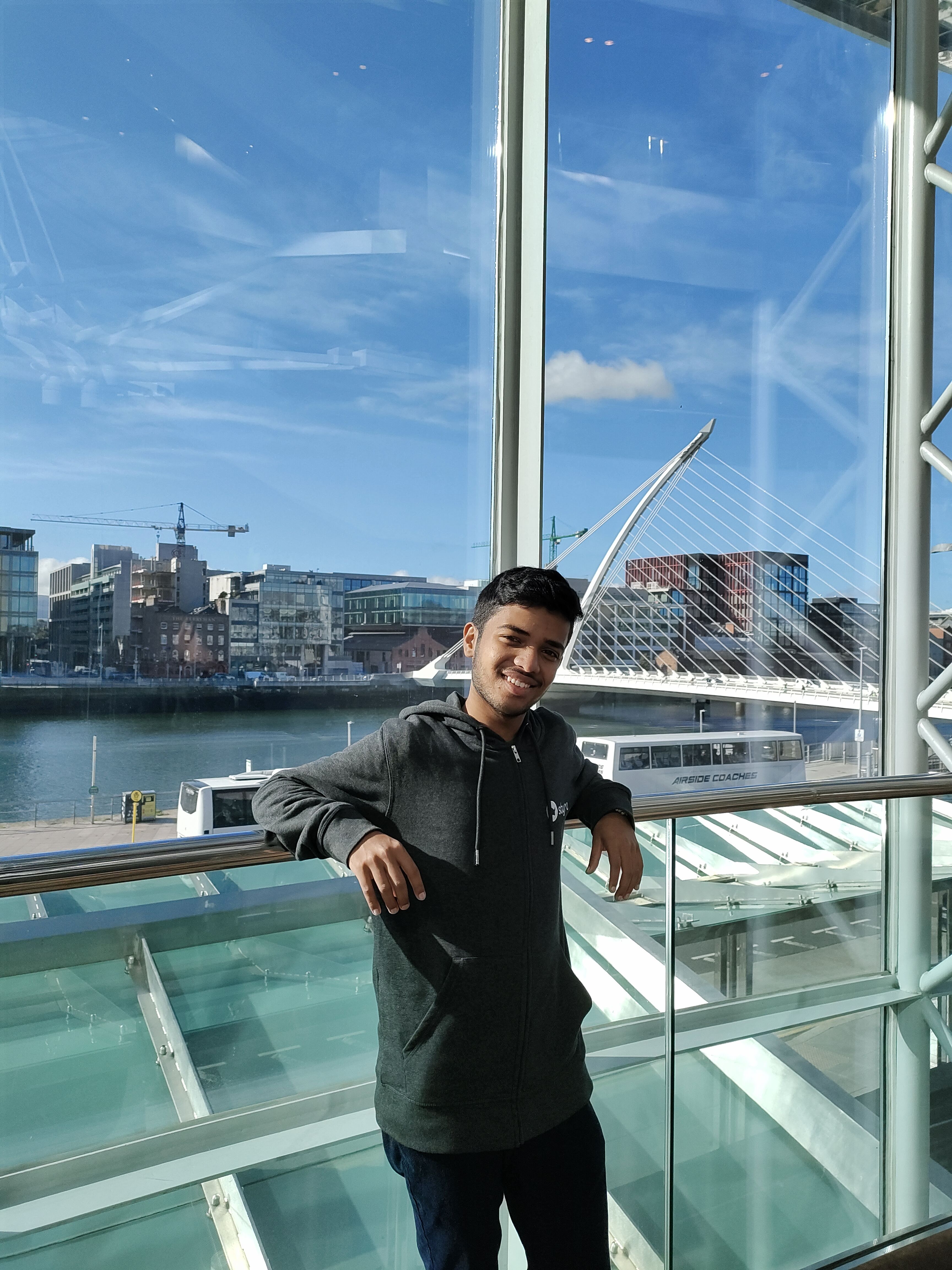
Created this Tutorial for my talk at Cloud @ DevFest Kolkata 2025
1. Objective
Set up a minimal API on Google Cloud and test its performance under load using Grafana K6.
2. Prerequisites
Google Cloud Account: Access to a GCP project.
K6 Installed: Download from Grafana K6.
3. Steps
Step 1: Create a Simple API
Create a basic REST API using Go.
Steps:
Install Go: Follow the official guide : https://go.dev/dl
Create a project folder:
mkdir simple-api && cd simple-api
vi main.go
Create an main.go
file:
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprint(w, "Hello, World!")
})
http.HandleFunc("/ping", func(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "text/html")
fmt.Fprint(w, `
<!DOCTYPE html>
<html>
<head>
<title>Welcome</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f3f4f6;
font-family: Arial, sans-serif;
}
h1 {
font-size: 2.5rem;
font-weight: bold;
text-align: center;
color: #333;
}
</style>
</head>
<body>
<h1>Welcome to Ronit Da's Session, Ft. GDG Cloud DevFest Kolkata 2024</h1>
</body>
</html>
`)
})
fmt.Println("Server is running on port 8080")
http.ListenAndServe(":8080", nil)
}
Run the API locally to test:
go run main.go
Access the API at http://localhost:8080
.
Step 2: Deploy the API to Google Cloud
Install Google Cloud CLI: Follow the official guide.
Authenticate: Run
gcloud auth login
.Enable Cloud Run API: Enable it with:
gcloud services enable run.googleapis.com
Deploy the API:
- Create a Dockerfile:
# Use the official Go image as a base image
FROM golang:1.20
# Set the working directory inside the container
WORKDIR /app
# Copy the Go module files and download dependencies
COPY go.mod go.sum ./
RUN go mod download
# Copy the source code into the container
COPY . .
# Build the Go application
RUN go build -o main .
# Expose the port that the application listens on
EXPOSE 8080
# Command to run the application
CMD ["./main"]
Build and deploy:
gcloud builds submit --tag gcr.io/YOUR_PROJECT_ID/simple-api gcloud run deploy simple-api --image gcr.io/YOUR_PROJECT_ID/simple-api --platform managed
Replace YOUR_PROJECT_ID
with your GCP project ID. Copy the deployed API URL.
Step 3: Write a K6 Test Script
Create a test.js
file:
import http from 'k6/http';
import { check, sleep } from 'k6';
//export let options = {
// vus: 10, // Virtual Users
// duration: '30s', // Test duration
//};
export let options = {
stages: [
{ duration: '20s', target: 20 }, // Ramp-up to 20 VUs over 20 seconds
{ duration: '20s', target: 50 }, // Ramp-up to 50 VUs over 20 seconds
{ duration: '20s', target: 50 }, // Stay at 50 VUs for 20 seconds
{ duration: '20s', target: 10 }, // Ramp-down to 20 VUs over 20 seconds
],
};
export default function () {
let res = http.get('https://YOUR_API_URL');
check(res, {
'status is 200': (r) => r.status === 200,
});
sleep(1);
}
Replace https://YOUR_API_URL
with your API URL from Cloud Run.
Step 4: Run the Test
Execute the test script:
k6 run test.js
Step 5: Visualize Results with Grafana
Install Grafana: Follow Grafana installation guide.
Integrate with K6:
Run K6 with InfluxDB or another data source for Grafana:
k6 run --out influxdb=http://localhost:8086/testdb test.js
Configure Grafana to use InfluxDB as a data source and create dashboards for visualizing performance metrics.
Step 6: Analyze and Interpret Results
Use the metrics to identify bottlenecks.
Look for trends like latency and failure rates under different loads.
Best Practices
Start Small: Begin with low VU counts and shorter durations.
Use Staging: Test in a staging environment first.
Automate: Add K6 tests to your CI/CD pipeline.
Subscribe to my newsletter
Read articles from Ronit Banerjee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
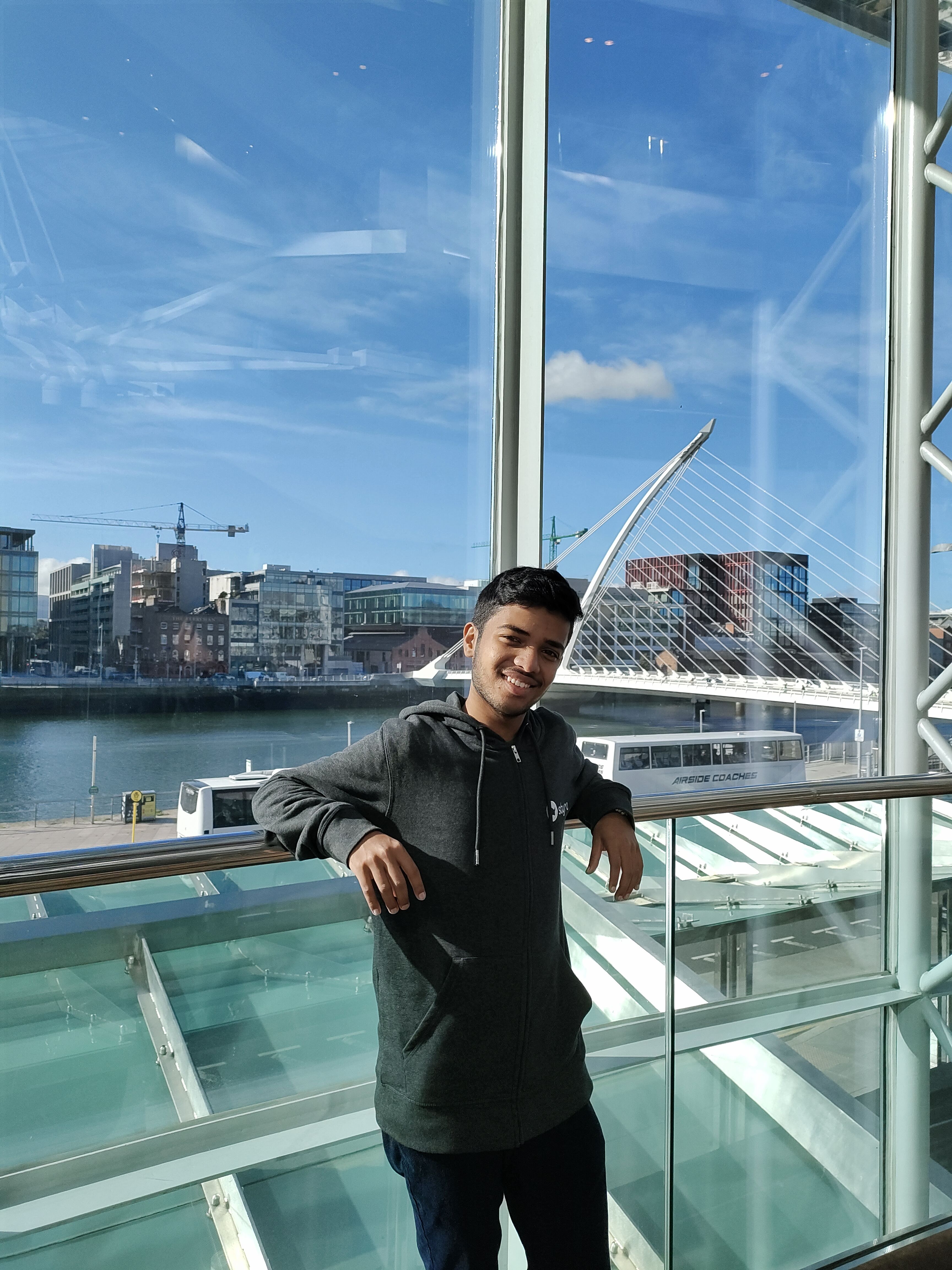
Ronit Banerjee
Ronit Banerjee
Building ProjectX.Cloud | GSoC'23 @ DBpedia | DevOps & Cloud Computing