Implement HTTPS in microservices and FE
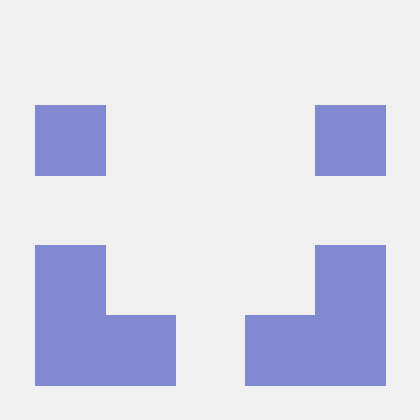
Hi. This is the twenty-third part of the diary about developing the “Programmers’ diary” blog. Previous part: https://hashnode.programmersdiary.com/catching-up-with-tests-in-security-lib. The open source code of this project is on https://github.com/TheProgrammersDiary. The first diary entry explains why this project was done: https://medium.com/@vievaldas/developing-a-website-with-microservices-part-1-the-idea-fe6e0a7a96b5.
Next entry:
—————
2024-01-20
[Note: text in these brackets means insights I got some time after writing this diary entry.]
Since our tests are caught up with written code, we can continue adding features. When the blog website will be running it will need encryption. Let’s check how to setup HTTPS.
First, we need to download openssl (if not already installed on your computer). Even if ssl is installed, it might give you an error then running specific commands, so I installed v 3.2.0: https://www.firedaemon.com/download-firedaemon-openssl.
After that we need to generate file containing a certificate (public key) and encrypted private key:
keytool -genkeypair -alias blog -keyalg RSA -keysize 2048 -storetype PKCS12 -keystore blog.p12 -validity 3650
It will prompt us for passphrase. The intruder will only be able to acquire our private key if he somehow got your passphrase, otherwise it will need to brute force the key. And with this key it will take a very long time to steal it.
After the keys are generated we go to blog microservice application-docker.properties:
server:
ssl:
key-store: classpath:blog.p12
key-store-password: ${ssl_blog_passphrase}
key-store-type: pkcs12
key-alias: blog
key-password: ${ssl_blog_passphrase}
ssl_blog_passphrase is an environmental variable, we need to pass it to docker-compose.yaml which is in GlobalTests repo: https://github.com/TheProgrammersDiary/Docker/commit/3fca8868046e27a07665b205d989f3c9d2544a08#diff-3fde9d1a396e140fefc7676e1bd237d67b6864552b6f45af1ebcc27bcd0bb6e9R18.
After adding ssl properties, we can modify the SecurityConfig:
@Bean
SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.requiresChannel(channel ->
channel.anyRequest().requiresSecure())
After that we use IDE searching tools to change http to https in all the code [I was over enthusiastic about this and also changed http to https in development code.].
Full changes are in commit https://github.com/TheProgrammersDiary/Blog/commit/d3997219f7cf1976281c9ba0dac7cb5a900a43dd.
Post microservice needs similar setup. Note that while doing integration tests, either
RestAssured.useRelaxedHTTPSValidation()
is needed, or a trustStore can be declared. I decided to add ssl config to application-it.properties and use given().trustStore(”path to .p12 file”, “password”). Since blog.p12 is inside resources folder, I only need to provide blog.p12 as path. With this setup the HTTP calls made from tests trust our post service. This lead me to an understanding: if we use the same certificate in all microservices and we should have no trust issues.
Note that docker-compose.yaml must be updated for post microservice to include the passphrase as env variable.
Adding HTTPS also forces us to update Github Actions. In the workflow the passphrase to decrypt the certificate’s private key is required:
run: mvn -B -s .github/maven-settings.xml -f post/pom.xml -Dssl_blog_passphrase=${{secrets.ssl_blog_passphrase}} package
so a new Github secret must be added.
Since multiple code repos were changed with many commits I will not post them all here. You can find them by looking for 2024-01-20 commits. Having said that let's continue.
In GlobalTests repo we copy the blog.p12 file and put it to test resources folder. Then add this:
private final String sslPassword = System.getenv("ssl_blog_passphrase");
inside PostCommentTest and add trustStore for each given() statement. The commit: https://github.com/TheProgrammersDiary/Docker/commit/3fca88. Having this setup will allow end to end tests to read the HTTPS.
We also need to update the docker-compose.yaml in blog microservice by adding a passphrase as env variable:
blog:
# blog container config
environment:
# other env variables
ssl_blog_passphrase: ${ssl_blog_passphrase}
We also do this for post and FE.
When using Github actions we need to inject ssl passphrase to docker-compose.yaml when running tests:
run: mvn -B -f GlobalTests/pom.xml -Dssl_blog_passphrase=${{secrets.ssl_blog_passphrase}} test
Remember to add ssl_blog_passphrase as Github secret.
For FE, we need to provide .pem instead of .p12. I have split certificate and private key with commands:
openssl pkcs12 -in blog.p12 -clcerts -nokeys -out ssl_certificate.pem
openssl pkcs12 -in blog.p12 -nocerts -out ssl_private_key.pem
I also create a new file server.js:
const { createServer } = require('https');
const { parse } = require('url');
const next = require('next');
const fs = require('fs');
const port = 3000;
const passphrase = process.env.ssl_blog_passphrase;
const dev = process.env.NODE_ENV !== 'production';
const app = next({ dev });
const handle = app.getRequestHandler();
const httpsOptions = {
key: fs.readFileSync('./ssl_private_key.pem'),
passphrase: passphrase,
cert: fs.readFileSync('./ssl_certificate.pem')
};
app.prepare().then(() => {
try {
createServer(httpsOptions, async (req, res) => {
const parsedUrl = parse(req.url, true);
await handle(req, res, parsedUrl);
}).listen(port, (err) => {
if (err) throw err;
console.log('ready - started server on url: <https://localhost>:' + port);
});
} catch (error) {
console.error('Error starting the server:', error);
}
});
This script reads the certificate and also a private key (since the scripts gets the passphrase injected) and starts a https server on port 3000. After that, We need to change package.json dev script to execute command, node server.js:
“dev”: “node server.js”.
Also, we need to update Github Actions workflow of FE:
run: ssl_blog_passphrase=${{secrets.SSL_BLOG_PASSPHRASE}} npx playwright test
Again, new Github actions secret needs to be added.
After that in FE we simply search the code for http and update it to https.
Last thing is to add self-signed certificate to the browser’s trust store. Although our services will trust between each other since they use the same certificate, the browser will not trust the self-signed certificate by default. So the browser needs the certificate imported.
After that, the system works with https.
Note that the last commit(s) contain(s) cors changes in post and blog microservices. Instead of using @CrossOrigin annotation in every controller, we can use this in SecurityConfig:
@Bean
public CorsFilter corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowedOrigins(Collections.singletonList(frontendUrl));
config.setAllowedMethods(List.of("GET", "POST"));
config.setAllowedHeaders(List.of("Origin", "Content-Type", "Accept", "Authorization"));
config.setAllowCredentials(true);
source.registerCorsConfiguration("/**", config);
return new CorsFilter(source);
}
Also, instead of using https://localhost:3000 in multiple places we can place it into a property:
post:
frontend-url: "https://localhost:3000"
and inject it:
@Value("${post.frontend-url}")
private String frontendUrl;
In FE, https://localhost:port is also replaced with a variable. For that a file next.config.js is created:
module.exports = {
blogUrl: process.env.blogUrl || "<https://localhost:8080>",
postUrl: process.env.postUrl || "<https://localhost:8081>",
};
Then the variables can be imported like this:
import {blogUrl} from "../../../next.config.js";
We managed to implements HTTPS in our services.
[Obviously, the HTTP could be implemented simpler and later, only to be used with the prod env. However, this is what happens when you want to explore how difficult it is to implement things on microservices and you don’t have any deadlines.
Most importantly, this setup works. We have learned how to use a certificate in non-unit tests so if we need to test the prod env by making direct calls to programmersdiary website, we already know how to do it.]
—————
Thanks for reading.
The project logged in this diary is open source, so if you would like to code or suggest changes, please visit https://github.com/TheProgrammersDiary.
Next part: https://hashnode.programmersdiary.com/implementing-double-submit-csrf-in-microservices.
Subscribe to my newsletter
Read articles from Evaldas Visockas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
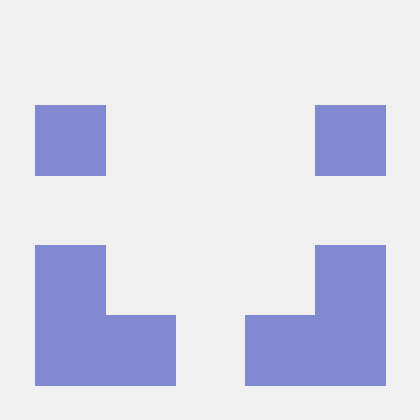