Learning C: Phase 2 (20 days)

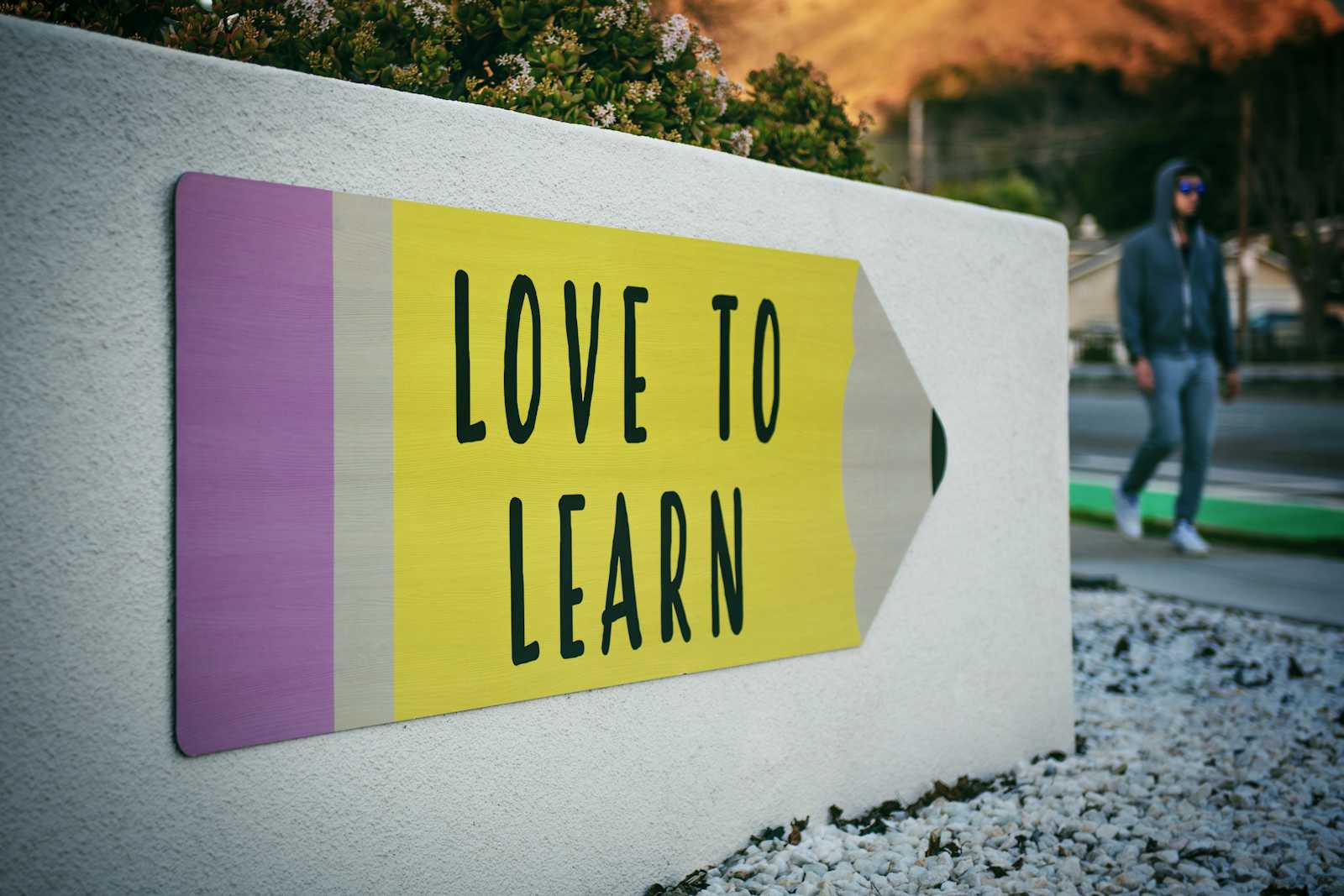
Introduction
In my previous article, I shared my journey of learning the basics of C programming over 10 days. I covered the fundamentals such as data types, operators, variables, control structures like if-else statements, and the ternary operator. I also practiced solving various problems related to these topics.
In this article, I'll be sharing my progress over the next 20 days, where I dove deeper into the world of C programming. I explored loops, pattern printing, and functions and applied my knowledge to solve the problems. In this article, I'll be sharing my experiences, key takeaways, and future plans from my journey so far.
What Iโve learned so far
Over the next 20 days, I dived deeper into the world of C programming, learning about loops, pattern printing, and functions. I gained hands-on experience with for, while, and do-while loops, and practiced printing patterns of various numbers and characters such as pyramid patterns, diamond patterns, Floyd's triangle, and more. I also learned about declaring and defining functions, function arguments and return types which helped me to write more efficient and modular code. By solving problems related to these topics, I improved my coding skills and gained a deeper understanding of C programming concepts.
Challenges I faced
Gradually as I progressed through my learning journey, I faced several challenges that tested my understanding and coding skills. One of the biggest challenges I faced was understanding some concepts of functions and implementing them correctly in my code. I also struggled while solving some problems. However, with persistence and practice, I was able to overcome these challenges and develop a better understanding of C programming concepts. I learned to approach problems more systematically and logically.
Key takeaways
After completing the next 20 days of my C programming journey, I have taken away the main key insight that will help me in my future coding journey.
Firstly, I learned the importance of practice and persistence in overcoming challenges and developing a deeper understanding of programming concepts.
Secondly, Sometimes I would get angry when the code ran incorrectly. But then I would patiently observe everything again and realize my own mistakes.
Finally, I realized the value of breaking down complex problems into smaller, more manageable parts and approaching them systematically and logically with patience. These takeaways have not only improved my coding skills but have also helped me to develop a more analytical approach to problem-solving.
A Code example
So here is a program. This C program prints Pascal's Triangle up to the nth row, where n is the input provided by the user.
#include <stdio.h>
int factorial(int x){
int fact=1;
for (int i=2;i<=x;i++){
fact = fact*i;
}
return fact;
}
int combination(int n, int r){
int ncr = factorial(n)/(factorial(r)*factorial(n-r));
return ncr;
}
int main() {
int n;
printf("Enter your number : ");
scanf("%d",&n);
for (int i=0;i<=n;i++){
for(int k = 0; k<= n-i; k++){
printf(" ");
}
for(int j=0;j<=i;j++){
int icj = combination(i,j);
printf("%d ",icj);
}
printf("\n");
}
return 0;
}
The program uses a combination function to calculate the binomial coefficients, which are then printed in a triangular shape. Its called the Pascalโs Triangle. This program is the perfect example of using concept of loop and functions. :)
This is the output :-
My resources
I'm learning C programming by watching tutorials by Raghab Sir from the College Walah channel on YouTube. Along with his tutorials, I also follow CodeWithHarry channel to gain more knowledge and insight.
Future Plans
In the next 10 days, I will dive deeper into the recursion. I aim to solve a variety of problems related to recursion to solidify my understanding of this topic. Additionally, I will begin exploring the concept of arrays in C programming, which will lay the foundation for more advanced data structures and algorithms. By focusing on these topics, I hope to develop my problem-solving skills and programming expertise.
Conclusion
the past month has been a valuable learning experience, and I'm excited to continue building on the foundations I've established. Through my studies and practice, I've gained a deeper understanding of C programming concepts, including loops, functions. Now I'm looking forward to apply these skills to more complex problems and projects in the future. I'm confident that I can continue to grow and improve as a programmer.
Thanks for reading till now!! Feel free to share your feedback in the comment section.
๐๐๐ ๐ฎ๐ค๐ช ๐๐ฃ ๐ฉ๐๐ ๐ฃ๐๐ญ๐ฉ ๐ฅ๐ค๐จ๐ฉ !!
Subscribe to my newsletter
Read articles from Arghya Roy Chowdhury directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
