Automating AWS EC2 Instance Creation with Ansible Playbooks
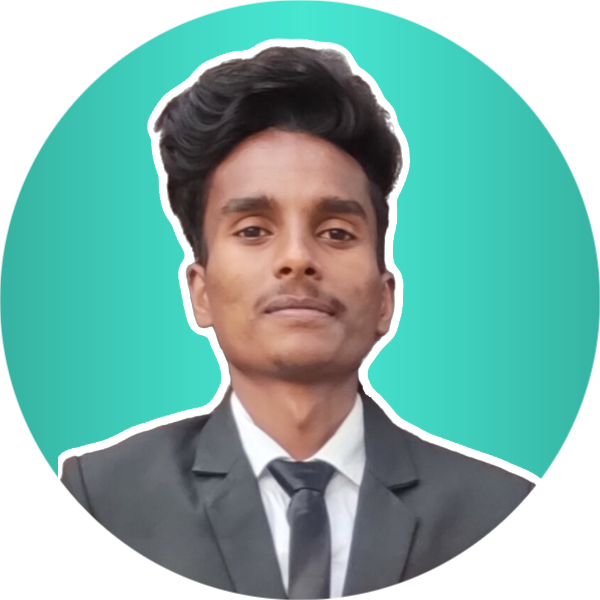

Automating AWS EC2 Instance Creation with Ansible Loops
Introduction
Automation is the backbone of modern DevOps practices. In this blog, I’ll guide you through a real-world project where I automated the creation of AWS EC2 instances using Ansible. The goal was to deploy two Ubuntu instances and one CentOS instance using a structured, secure, and repeatable method.
We’ll also dive into why certain tools, like Boto3, are used and what each command does in detail.
What is an Ansible Playbook?
An Ansible playbook is a structured YAML file used to define a set of tasks for automating IT processes. It’s like a recipe where each step describes an action, such as installing software, configuring servers, or, in this case, provisioning cloud resources like AWS EC2 instances.
Playbooks are designed to:
Be declarative: You specify the desired state, and Ansible figures out the necessary steps to achieve it.
Support idempotency: Running the same playbook multiple times doesn’t result in duplicate changes; Ansible ensures the environment matches the desired state.
Handle complex workflows: Through loops, conditional logic, and task dependencies, playbooks can manage complex deployments efficiently.
In this project, our playbook automates the creation of three EC2 instances on AWS (two Ubuntu and one CentOS), demonstrating how playbooks can simplify cloud infrastructure management.
Step 1: Set Up Your Ansible Control Node
To begin, we need an instance to act as the Ansible control node where the playbook will run. You can use any machine, but I used a Linux EC2 instance for this purpose.
Once logged into the instance, follow these steps:
Update the system packages:
sudo yum update -y
This command ensures your instance has the latest security patches and software updates.
Install Python and Pip:
sudo yum install -y python3 python3-pip
Python is required because Ansible and Boto3 (AWS SDK for Python) are Python-based. Pip is a package manager for installing Python libraries.
Install Ansible:
sudo pip3 install ansible ansible --version
The
pip3 install ansible
command fetches and installs Ansible.ansible --version
confirms the installation and displays the installed version.
Install Boto3:
sudo pip3 install boto3 python3 -c "import boto3; print(boto3.__version__)"
Boto3 is AWS's SDK for Python, enabling Ansible to interact with AWS services like EC2.
Why use Boto3?
Boto3 is the official AWS SDK for Python. It enables Ansible to interact with AWS services, like creating EC2 instances, managing S3 buckets, and more. While Ansible provides AWS modules, these modules rely on Boto3 under the hood to communicate with AWS APIs.
Step 2: Set Up an IAM User for Ansible
To allow Ansible to manage AWS resources securely, create a dedicated IAM user.
Why Create an IAM User?
Using a dedicated IAM user:
Improves security by granting limited permissions.
Helps track and manage AWS actions performed by Ansible.
Steps to Create the IAM User:
Log into the AWS Management Console and navigate to IAM > Users.
Click Add User and enter a username, such as
ansible-user
.Select Programmatic Access (no need for console access).
Attach the AmazonEC2FullAccess policy.
- This allows the user to create, modify, and delete EC2 resources.
Open the
ansible-user
in IAM, go to the Security credentials tab, and create a new access key.- Select the use case Application running outside AWS to generate the access key.
Download the Access Key ID and Secret Access Key.
Step 3: Secure AWS Credentials with Ansible Vault
Hardcoding sensitive data like access keys into a playbook is risky. To address this, we’ll use Ansible Vault for encryption.
Create a Vault Password File
openssl rand -base64 2048 > vault.pass
This command generates a random password and saves it to vault.pass
. The password will be used to encrypt and decrypt your data.
Store AWS Credentials in an Encrypted File
ansible-vault create group_vars/all/pass.yml --vault-password-file vault.pass
This creates an encrypted file named
pass.yml
.In the editor that opens, add the following credentials:
Edit the Vault File (if needed):
ansible-vault edit group_vars/all/pass.yml --vault-password-file vault.pass
By using Ansible Vault, your sensitive credentials remain encrypted and secure.
Step 4: Write the Ansible Playbook
The playbook defines the tasks needed to create EC2 instances. Let’s create one:
Create a Playbook File
codevi ec2_create.yaml
Add the following content:
yamlCopy code---
- hosts: localhost
connection: local
tasks:
- name: Create EC2 instances
amazon.aws.ec2_instance:
name: "{{ item.name }}"
key_name: "target-ans" # Replace with your key pair name
instance_type: t2.micro
security_group: default # Replace with your security group
region: us-east-2 # Replace with your region
aws_access_key: "{{ ec2_access_key }}" # From Vault
aws_secret_key: "{{ ec2_secret_key }}" # From Vault
network:
assign_public_ip: true
image_id: "{{ item.image }}"
loop:
- { image: "ami-0b4624933067d393a", name: "ubuntu-node-1" }
- { image: "ami-0b4624933067d393a", name: "ubuntu-node-2" }
- { image: "ami-036841078a4b68e14", name: "centos-node-1" }
Explanation of Key Playbook Elements:
amazon.aws.ec
2_instance
: Ansible module to create and manage EC2 instances.name
: Assigns a unique name to each instance.loop
: Iterates over multiple items (instances) to avoid repetitive tasks.image_id
: Specifies the Amazon Machine Image (AMI) for the operating system.aws_access_key
andaws_secret_key
: Securely fetched from the Ansible Vault.
Step 5: Execute the Playbook
Run the playbook using the following command:
bashCopy codeansible-playbook ec2_create.yaml --vault-password-file vault.pass
This command:
Reads the tasks from
ec2_create.yaml
.Decrypts the Vault file to fetch AWS credentials.
Provisions the EC2 instances as defined in the playbook.
Testing and Verifying
After execution, navigate to the AWS EC2 Console to verify that the instances were successfully created. Look for three instances:
Two with Ubuntu.
One with CentOS.
Conclusion
This project highlights how Ansible can simplify AWS resource provisioning:
IAM ensures secure and isolated access.
Ansible Vault protects sensitive credentials.
Playbook loops enable efficient and scalable automation.
By combining Ansible and Boto3, you can automate complex cloud deployments with ease.
Key Takeaways
Use Ansible Vault for security: Encrypt sensitive data to safeguard your infrastructure.
IAM users improve security: Assign permissions specific to the tasks you want to automate.
Ansible loops save time: Simplify repetitive tasks with concise configurations.
Subscribe to my newsletter
Read articles from Balaji Samleti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
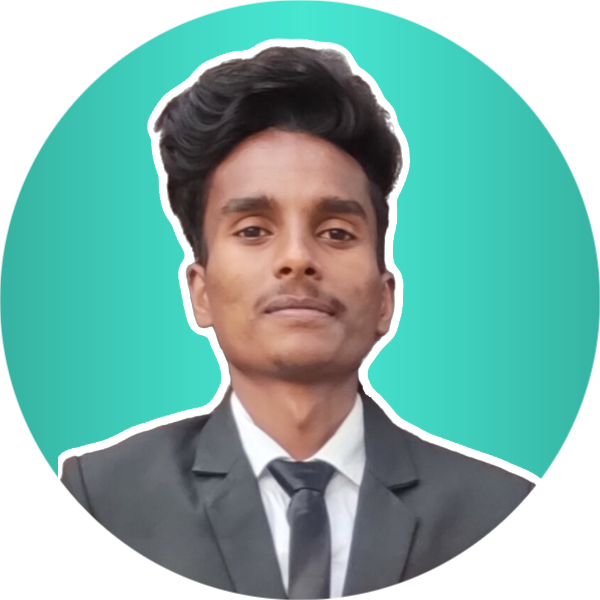
Balaji Samleti
Balaji Samleti
🌟 Certified AWS Solutions Architect Associate & Cloud Engineer @fibe.india Passionate about optimizing deployments in production and QA environments, I bring a solid foundation in Cloud & DevOps methodologies and agile practices. My expertise lies in implementing robust CI/CD pipelines and orchestrating efficient infrastructure management. With a background in AWS Cloud Engineer, Linux, and project management, I've honed my skills in cloud technologies, container orchestration, and automation tools.