How to Build a Cost-Effective ChatGPT Alternative with OpenAI API
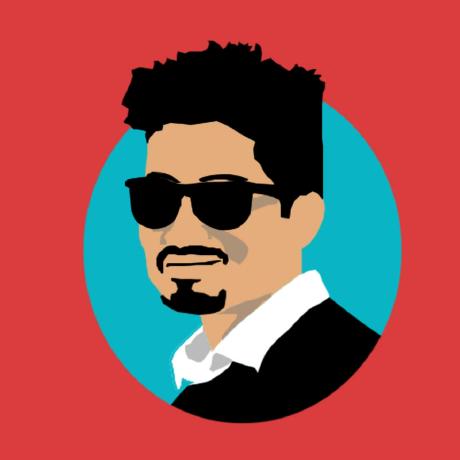

Why Choose the OpenAI API Over ChatGPT Plus?
Before diving into the implementation, let's clarify why the OpenAI API is often the better choice for tinkerers:
Cost Control:
OpenAI API charges per token, making it cheaper for sporadic or bursty usage.
You only pay for what you use, unlike the fixed $20/month of ChatGPT Plus.
Flexibility:
Tailor the behavior of the model using parameters like
temperature
andmax_tokens
.Build domain-specific or task-specific tools (e.g., code assistants).
Integration:
Embed GPT into your workflows, applications, or services.
Combine the API with other tools and APIs for powerful automation.
Custom UI:
- Unlike ChatGPT Plus, where you’re locked into a pre-built interface, the API lets you design your own interface, workflows, and user experience.
Building a Cost-Effective, Custom ChatGPT-Like Interface
We’ll use a modular approach, combining open-source tools like LangChain, Streamlit, Gradio, and pre-built UI repositories. This method ensures you have the flexibility to tinker, scale, and customize to your heart’s content.
Step 1: Planning Your Features
Before jumping into code, decide on the features you want:
Core Features:
Input box for user prompts.
Display for model responses.
Chat history with memory.
Advanced Features:
Adjustable parameters (temperature, tokens).
Token usage tracking for cost monitoring.
Multiple GPT models (e.g., GPT-3.5 and GPT-4) with easy switching.
UI Enhancements:
Dark mode/light mode toggle.
Markdown support for rich-text responses.
File upload for prompts.
Integration Features:
Plug-ins for external data (e.g., APIs, databases).
LangChain-powered tools (e.g., search integrations, calculators).
Step 2: Tools of the Trade
Here’s what we’ll use:
LangChain: To handle conversational agents with memory.
Gradio: For building a sleek, interactive UI.
Streamlit: For rapid prototyping and app deployment.
FastAPI: For a robust back-end API layer.
Pre-built Open-Source UI Templates: To save development time and focus on customization.
Step 3: Building the Back-End
Start by setting up a robust back-end to interact with the OpenAI API. We'll use FastAPI for its simplicity and speed.
Install FastAPI and Uvicorn:
pip install fastapi uvicorn openai
Create the FastAPI Server:
from fastapi import FastAPI, Request import openai openai.api_key = "your-openai-api-key" app = FastAPI() @app.post("/chat/") async def chat(request: Request): data = await request.json() prompt = data.get("prompt", "") response = openai.ChatCompletion.create( model="gpt-4", messages=[{"role": "user", "content": prompt}] ) return {"response": response["choices"][0]["message"]["content"]}
Run the Server:
uvicorn main:app --reload
Step 4: Building the Front-End UI
Option 1: Using Gradio
Gradio simplifies the creation of interactive UIs. Here's how:
Install Gradio:
pip install gradio
Create a Basic UI:
import gradio as gr import requests def chat_with_gpt(prompt): response = requests.post("http://127.0.0.1:8000/chat/", json={"prompt": prompt}) return response.json()["response"] interface = gr.Interface( fn=chat_with_gpt, inputs="text", outputs="text", title="Custom ChatGPT UI" ) interface.launch()
Option 2: Using Streamlit
Streamlit offers more flexibility for creating dashboards and UIs.
Install Streamlit:
pip install streamlit
Create a Streamlit App:
import streamlit as st import requests st.title("Custom ChatGPT Interface") user_input = st.text_input("Enter your message:") if st.button("Send"): response = requests.post("http://127.0.0.1:8000/chat/", json={"prompt": user_input}) st.write("Response:", response.json()["response"])
Run the App:
streamlit run app.py
Step 5: Adding Advanced Features
1. Token Usage Tracker
Track tokens used per conversation to monitor costs:
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "user", "content": prompt}]
)
tokens_used = response['usage']['total_tokens']
2. Model Parameter Adjustments
Add sliders in the UI for parameters like temperature and max tokens:
Gradio: Use
gr.Slider
.Streamlit: Use
st.slider
.
3. Conversation Memory
Use LangChain to add memory to your chatbot:
from langchain.chat_models import ChatOpenAI
from langchain.chains import ConversationChain
from langchain.memory import ConversationBufferMemory
llm = ChatOpenAI(model="gpt-4", api_key="your-api-key")
memory = ConversationBufferMemory()
conversation = ConversationChain(llm=llm, memory=memory)
response = conversation.run("Hello, how are you?")
Step 6: Hosting Locally or in the Cloud
1. Local Hosting
Use Docker for portability:
FROM python:3.9 WORKDIR /app COPY . . RUN pip install -r requirements.txt CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "8000"]
Build and run:
docker build -t custom-chatgpt . docker run -p 8000:8000 custom-chatgpt
2. Cloud Hosting
Use Heroku, AWS, or DigitalOcean to host your app.
Pair with a CDN for faster global access.
The End Result
You’ve now built a highly cost-effective, customizable ChatGPT-like interface. You can:
Experiment with GPT-4 without the limitations of ChatGPT Plus.
Scale your usage dynamically with precise cost control.
Tinker endlessly with features, integrations, and workflows.
Why This Approach Stands Out
Cost Efficiency: Only pay for tokens you use.
Customizability: Design the UI and workflows exactly how you want.
Scalability: From personal experiments to production-grade applications.
Fun Factor: For tinkerers, the joy of building something uniquely yours.
Feel free to reach out to me at AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
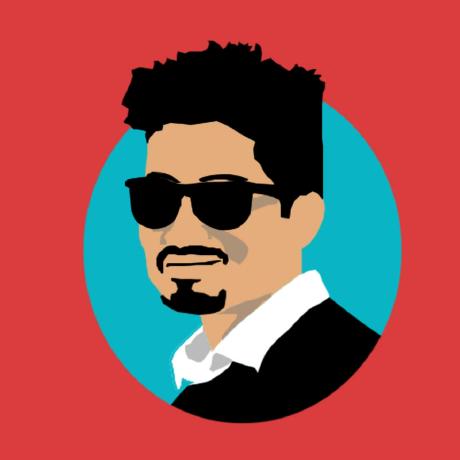