Using Pulumi’s Apply Method

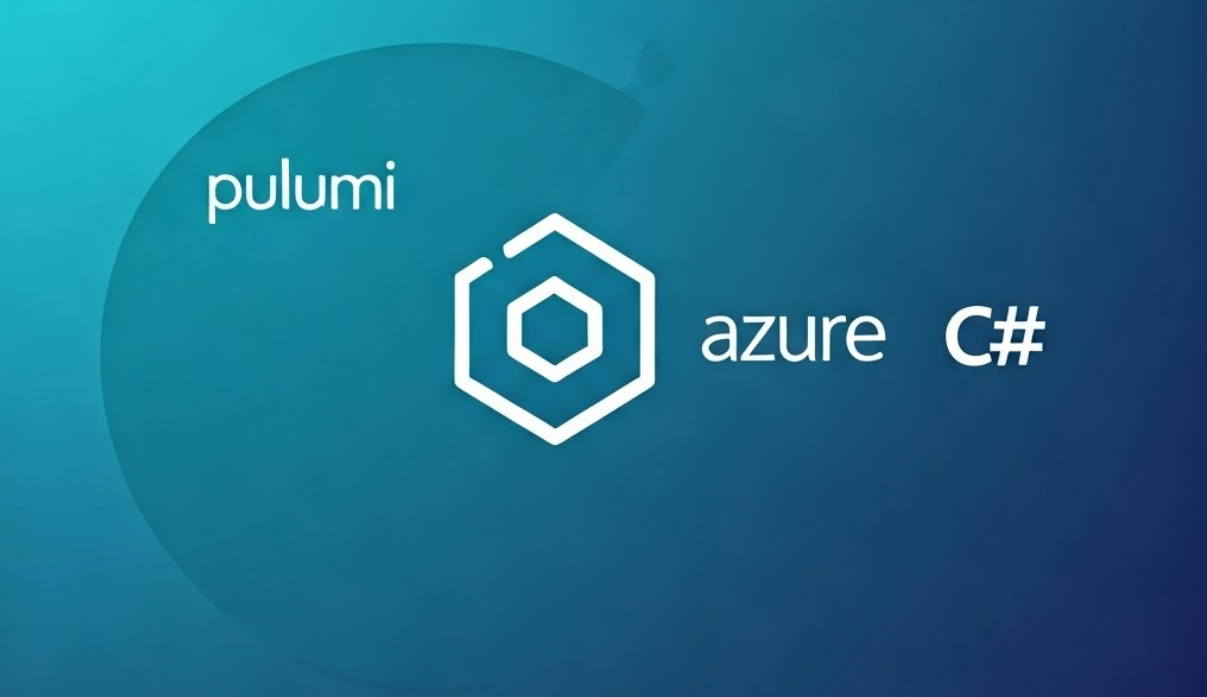
This blog post provides a concise guide on leveraging Pulumi's Apply
method within the context of Azure infrastructure and C#. Pulumi's Apply
method is a powerful tool for performing actions after a resource has been provisioned, allowing for dynamic configuration and post-deployment tasks. We'll illustrate this with clear examples.
The Apply
method allows you to interact with resources after Pulumi has created them. This enables you to execute custom logic based on the resource's properties. A common use case is to retrieve resource IDs or connection strings, which are usually available only after deployment completes. To make use of Apply
, ensure you have the necessary Azure and Pulumi packages installed in your project. You might need to set up an Azure service principal to authenticate.
Let's consider a scenario where we deploy a storage account and then retrieve its connection string using Apply
. Here's a snippet of C# code that demonstrates this:
using Pulumi;
using Pulumi.AzureNative.Resources;
using Pulumi.AzureNative.Storage;
using Pulumi.AzureNative.Storage.Inputs;
using System.Threading.Tasks;
public class MyStack : Stack
{
[Output]
public Output<string> ConnectionString { get; private set; }
public MyStack()
{
var resourceGroup = CreateResourceGroup();
var storageAccount = CreateStorageAccount(resourceGroup);
this.ConnectionString = GetStorageAccountConnectionString(resourceGroup.Name, storageAccount.Name);
}
private ResourceGroup CreateResourceGroup()
{
return new ResourceGroup("resourceGroup");
}
private StorageAccount CreateStorageAccount(ResourceGroup resourceGroup)
{
return new StorageAccount("mystorage", new StorageAccountArgs
{
ResourceGroupName = resourceGroup.Name,
AccountName = "myaccount",
Sku = new SkuArgs { Name = SkuName.Premium_LRS }
});
}
private Output<string> GetStorageAccountConnectionString(Output<string> resourceGroupName, Output<string> accountName)
{
return Output.Tuple(resourceGroupName, accountName).Apply(names =>
{
var (rgName, accName) = names;
return GetStorageAccountPrimaryKey(rgName, accName).Apply(key =>
$"DefaultEndpointsProtocol=https;AccountName={accName};AccountKey={key};EndpointSuffix=core.windows.net");
});
}
private static async Task<string> GetStorageAccountPrimaryKey(string resourceGroupName, string accountName)
{
var accountKeys = await ListStorageAccountKeys.InvokeAsync(new ListStorageAccountKeysArgs
{
ResourceGroupName = resourceGroupName,
AccountName = accountName
});
return accountKeys.Keys[0].Value;
}
}
In this example, I started by creating a Resource Group and a Storage Account using Pulumi's Azure Native provider.
To securely retrieve the primary key, I used the ListStorageAccountKeys
function, which is wrapped in an asynchronous method.
The Apply
method was then used to combine the Resource Group name and Storage Account name, passing them to the key retrieval function. Once the key was obtained, I constructed a connection string in the format required by Azure.
This approach not only ensures that sensitive information like the primary key is handled securely but also demonstrates how Pulumi's Apply
method can elegantly manage dependencies and transform outputs in a clean, readable way. By breaking the code into smaller, single-responsibility methods, the solution becomes more maintainable and easier to extend for future use cases.
Practical applications extend beyond connection strings. Imagine a scenario where you need to register a custom resource provider or configure service endpoints post-deployment. The Apply
method provides the flexibility to handle such scenarios, facilitating a more tailored and dynamic infrastructure deployment. By combining the power of Apply
with Pulumi's infrastructure-as-code approach, you can effectively manage and automate your Azure environments efficiently.
To further enhance your understanding, explore Pulumi's official documentation, which offers in-depth examples and advanced techniques. Also I found this blog post very useful. Exploring these resources will solidify your grasp on how to make the most of this powerful tool.
Subscribe to my newsletter
Read articles from Hossein Margani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
