Difference between mockClear, mockReset, mockRestore in Jest
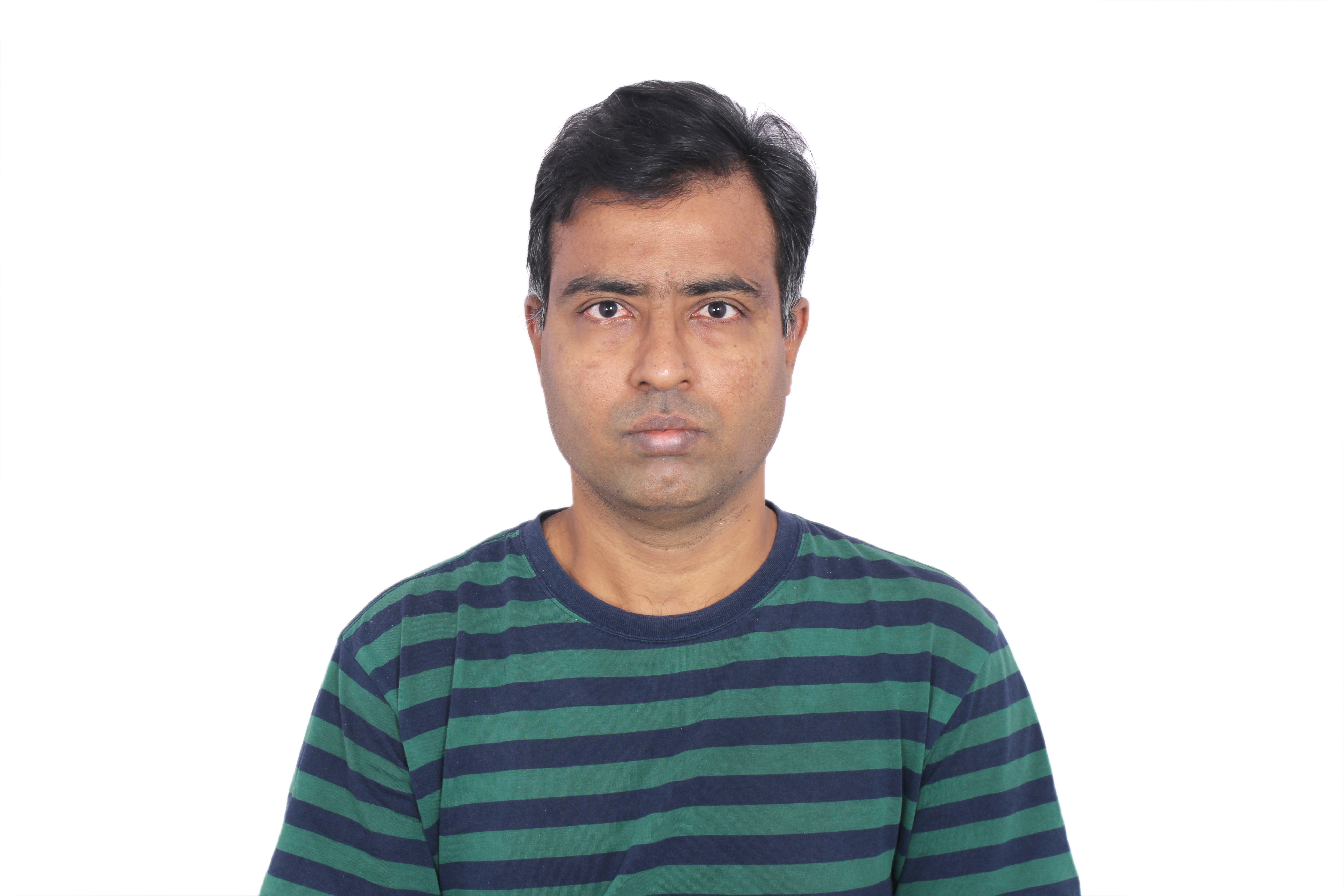

Some questions that came up while working on Jest.
Difference between mockClear, mockReset, mockRestore
mockClear clears the call history of a mock.
const mockFn = jest.fn();
mockFn(); // call 1
mockFn(); // call 2
expect(mockFn).toHaveBeenCalledTimes(2);
mockFn.mockClear();
expect(mockFn).toHaveBeenCalledTimes(0);
mockReset clears the call history and removes any mock implementation specified for the mock.
const mockFn = jest.fn(() => 'initial');
mockFn.mockImplementation(() => 'changed');
mockFn(); // returns 'changed'
mockFn.mockReset();
mockFn(); // returns initial
mockRestore removes the mock.
const originalFunction = jest.spyOn(Math, 'abs');
originalFunction.mockImplementation(() => 'mocked');
console.log(originalFunction(-5)); // 'mocked'
originalFunction.mockRestore();
console.log(Math.abs(-5)); // 5
When to use act() in RTL?
Use act() when there are state updates that need to happen after firing an event.
act(() => {
fireEvent.click(button);
});
Difference between toBeCalled() and toHaveBeenCalled()
toHaveBeenCalled checks if a mock is called at least once. toBeCalled also does the same thing. But it has some variations - toBeCalledWith() that checks the arguments that the mock is called with. The number of times that it is called is also checked in toBeCalledWith assertion.
Subscribe to my newsletter
Read articles from Vijay Thirugnanam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
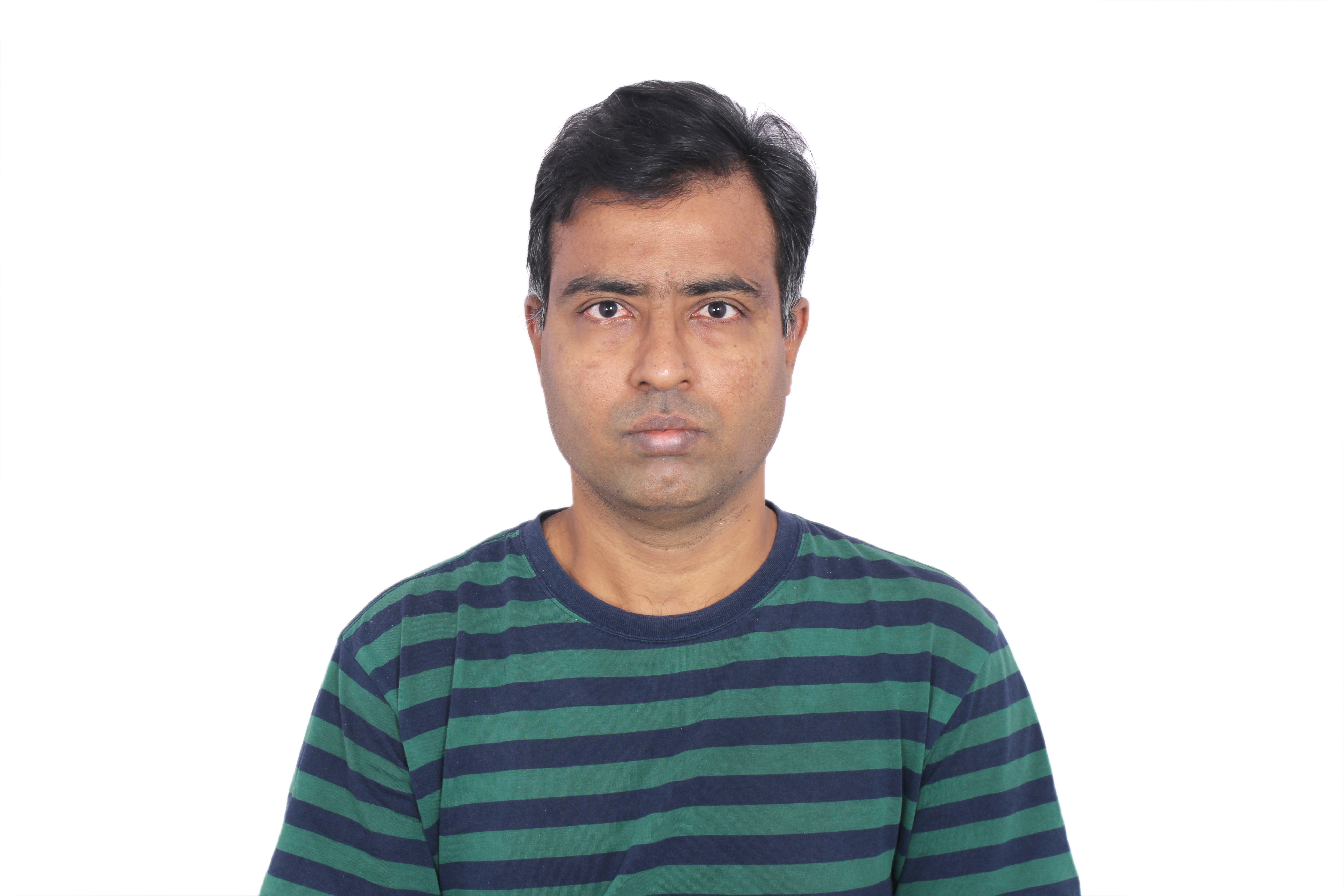
Vijay Thirugnanam
Vijay Thirugnanam
Engineer working in Cerebras. Latest work is Cerebras Inference. I love writing code in React, TypeScript, C++, Python. I spend most of my work hours tinkering with code.