Mastering Telegram Bot Hosting: Webhook vs. Long Polling Explained

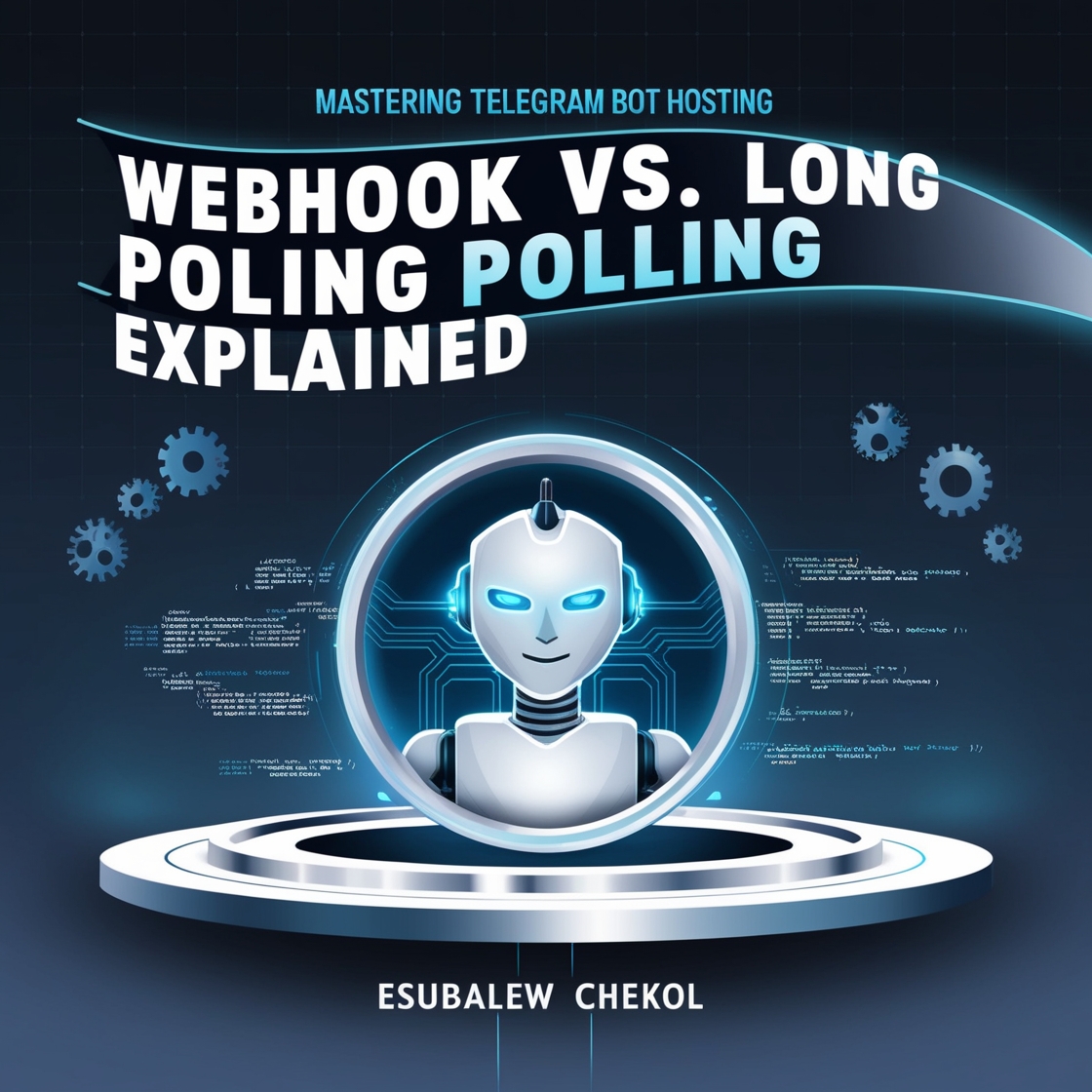
Hosting a Telegram bot can be accomplished in two primary ways: Webhook and Long Polling. Each approach has its own advantages, use cases, and considerations. Let’s dive deeper into these methods and provide practical examples using python-telegram-bot
(PTB) version 20+.
1. Webhook
Overview
The webhook method enables Telegram to send updates to your bot directly by making HTTP requests to a specified URL. This approach is efficient and ideal for production bots hosted on servers.
Requirements
- A public HTTPS URL (e.g., hosted on a cloud platform or a self-hosted server with an SSL certificate).
- A web server or framework to handle incoming requests.
Advantages
- Low Latency: Updates are pushed to your bot immediately, reducing response time.
- Efficient Resource Usage: No need for continuous polling; only active when updates arrive.
- Ideal for High-Traffic Bots: Scales well for bots with high user interaction.
Considerations
- Requires a public and secure HTTPS endpoint.
- Hosting costs may apply depending on the platform used.
Example: Setting Up a Webhook with FastAPI
Here’s how you can use FastAPI to handle webhook updates:
from fastapi import FastAPI, Request
from telegram import Update, Bot
from telegram.ext import Application, CommandHandler
app = FastAPI()
BOT_TOKEN = "YOUR_BOT_TOKEN"
bot = Bot(token=BOT_TOKEN)
application = Application.builder().token(BOT_TOKEN).build()
async def start(update: Update, _):
await update.message.reply_text("Hello! This bot is using webhook updates.")
application.add_handler(CommandHandler("start", start))
@app.post("/{token}")
async def webhook(token: str, request: Request):
if token != BOT_TOKEN:
return {"error": "Invalid token"}
data = await request.json()
update = Update.de_json(data, bot)
await application.process_update(update)
return {"status": "ok"}
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8443)
This setup ensures that your bot processes updates received via HTTP POST requests.
2. Long Polling
Overview
Long polling involves the bot repeatedly requesting updates from Telegram’s servers using the getUpdates
method. This is simpler to set up and works without a public URL.
Requirements
- A machine with internet access (e.g., local development environment or server).
- No need for a public HTTPS endpoint.
Advantages
- Simple Setup: Easy to configure without additional infrastructure.
- Local Development: Ideal for testing and development purposes.
Considerations
- Higher Latency: Updates are fetched periodically, introducing slight delays.
- Resource Intensive: Requires continuous polling, which may lead to higher server usage.
- Not Ideal for High-Traffic Bots: May not scale well under heavy load.
Example: Setting Up Long Polling with PTB
Here’s a simple example using python-telegram-bot
:
from telegram import Update
from telegram.ext import Application, CommandHandler, ContextTypes
async def start(update: Update, context: ContextTypes.DEFAULT_TYPE):
await update.message.reply_text("Hello! This bot is using long polling.")
app = Application.builder().token("YOUR_BOT_TOKEN").build()
app.add_handler(CommandHandler("start", start))
if __name__ == "__main__":
app.run_polling()
This approach continuously polls Telegram servers for new updates and processes them as they arrive.
Comparison: Webhook vs. Long Polling
Feature | Webhook | Long Polling |
Latency | Low | Moderate |
Setup Complexity | High (requires HTTPS) | Low |
Resource Usage | Efficient | Resource-intensive |
Ideal For | Production environments | Development and testing |
Conclusion
Choosing between webhook and long polling depends on your project’s requirements:
- Use webhook if you need low latency and plan to host your bot in a production environment with a public HTTPS endpoint.
- Opt for long polling for simplicity, especially during development and testing.
Both methods are fully supported by python-telegram-bot
and can be seamlessly integrated into your bot projects. With PTB’s rich feature set, implementing either approach is straightforward and efficient. Whichever method you choose, building Telegram bots with PTB is a rewarding experience.
Subscribe to my newsletter
Read articles from Esubalew Chekol directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Esubalew Chekol
Esubalew Chekol
Experienced Developer in Python, Javascript, Django, Node.js React, React Natvie Jetpack Compose and Telegram bot development.