Exploring Flutter's DevTools for Debugging and Performance Tuning

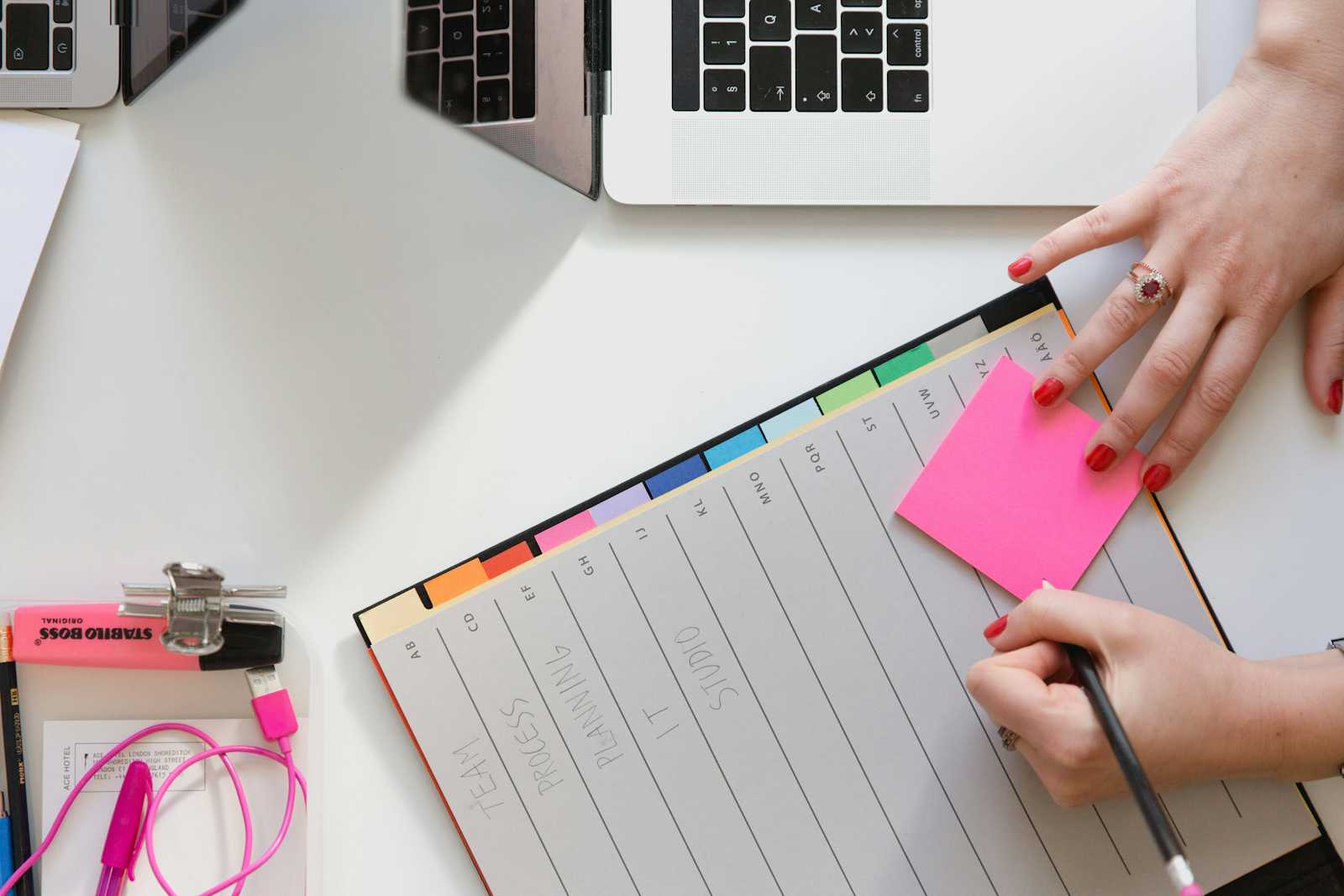
Developing a high-performing mobile application is a priority for every developer. Flutter, Google's UI toolkit, empowers developers to create natively compiled applications for mobile, web, and desktop from a single codebase. But even the most well-written applications require debugging and optimization to ensure optimal performance. This is where Flutter’s DevTools come into play.
In this blog, we’ll explore Flutter’s DevTools—a suite of performance and debugging tools—and how you can leverage them for efficient debugging and performance tuning.
What Are Flutter DevTools?
Flutter DevTools is an open-source suite of debugging and performance tools specifically designed for Flutter developers. These tools allow you to inspect your application’s widget hierarchy, monitor rendering performance, diagnose layout issues, track network requests, and more.
The DevTools suite is accessible either as a standalone web app or integrated within popular IDEs like Android Studio and Visual Studio Code.
Key features include:
Widget inspector
Performance timeline
Memory profiler
Network tracking
Logging and diagnostics
Setting Up Flutter DevTools
Prerequisites
Before diving into DevTools, ensure that:
You have Flutter SDK installed.
You’re using a compatible IDE (Android Studio, VS Code, or IntelliJ IDEA).
Your Flutter project is set up and running either on an emulator or a physical device.
Launching DevTools
You can access Flutter DevTools in three primary ways:
Command Line: Run the following command:
flutter pub global activate devtools flutter pub global run devtools
Access the DevTools URL provided in your terminal.
IDE Integration:
In VS Code: Open the Run and Debug tab and click on the DevTools link.
In Android Studio: Click on Flutter Inspector in the right-hand panel.
Web Interface: Open the DevTools directly from the URL if it’s hosted on your local server.
Debugging with Flutter DevTools
1. Widget Inspector
The Widget Inspector is one of the most used tools in DevTools. It allows you to:
Inspect and visualize the widget tree.
Check widget properties and layout constraints.
Example Use Case
Let’s say you have a misaligned button in your UI. Open the Widget Inspector, select the button widget, and review its properties and constraints. Adjust the code accordingly.
Container(
alignment: Alignment.center,
child: ElevatedButton(
onPressed: () {},
child: Text('Click Me'),
),
)
After tweaking the alignment
property, you can instantly view the changes in the app.
2. Performance Timeline
The Performance Timeline helps you identify rendering and layout issues. It records frame timings, enabling you to debug jank (dropped frames) in your app.
Steps to Use:
Open the Performance tab in DevTools.
Run the app and interact with it.
Check the recorded frame timings for slow frames.
Pro Tip: Keep your app’s frame rate at 60 FPS for a smooth user experience.
3. Memory Profiler
Efficient memory usage is critical for Flutter apps. The Memory Profiler provides insights into:
Memory allocation trends.
Garbage collection.
Active memory usage.
Example Use Case
If your app consumes excessive memory, the profiler will highlight spikes in allocation. This could point to issues like:
Unoptimized image loading.
Leaked objects.
Fix: Ensure you’re disposing of controllers properly:
@override
void dispose() {
myController.dispose();
super.dispose();
}
4. Network Tracking
The Network tab in DevTools logs all HTTP requests and responses. This feature is especially useful for debugging APIs and optimizing network calls.
Example Use Case
Suppose your app’s API call takes longer than expected. Use the Network tab to inspect the request timeline and identify delays in server response.
Optimization Tip: Use caching and efficient data structures to reduce API response times.
5. Logging and Diagnostics
The Logging feature in DevTools captures log output, allowing you to debug issues such as exceptions and errors in your app.
Example Log
[ERROR:flutter/lib/ui/ui_dart_state.cc(177)] Unhandled Exception: FormatException: Invalid input
Use this log to pinpoint the problematic code and fix it promptly.
Performance Tuning Tips Using DevTools
Optimize Widget Builds
Rebuilding widgets unnecessarily can affect performance. Use DevTools to:
Highlight widgets that are rebuilding.
Optimize state management solutions like Provider, Riverpod, or Bloc.
Reduce Overdraw
Overdraw happens when pixels are rendered multiple times in a single frame. Use the Layer Inspector in DevTools to identify overdraw and minimize it by simplifying the widget tree.
Monitor App Startup Time
Startup time is crucial for user retention. Use the Timeline tab to analyze startup performance and reduce delays caused by heavy initialization tasks.
Real-World Example: Debugging a Flutter App
Imagine a Flutter app that has performance issues:
The home page takes too long to load.
Scrolling is janky.
Steps to Debug:
Check Network Tab: Inspect API calls for delays.
Inspect Widget Tree: Identify widgets causing excessive rebuilds.
Use Performance Timeline: Spot frames dropping below 16ms.
Optimize Code:
ListView.builder( itemCount: items.length, itemBuilder: (context, index) { return ListTile( title: Text(items[index]), ); }, )
Replace inefficient widget loading methods with lazy loading approaches.
Why Flutter DevTools Are Indispensable
Flutter DevTools is not just a debugging tool—it’s a comprehensive suite for performance optimization. Whether you're building a simple app or a complex application, DevTools helps to identify issues and deliver a seamless user experience. By integrating these tools into your development workflow, you can enhance your app's quality and efficiency. Mastering DevTools ensures that your apps not only meet but exceed user expectations.
Subscribe to my newsletter
Read articles from Arka Infotech directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
