Understanding Core Concepts in GraphQL

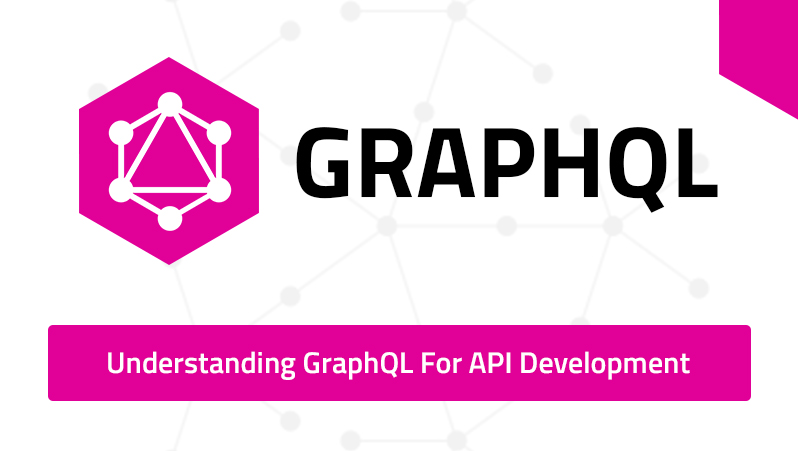
GraphQL is a versatile and intuitive query language for APIs, and understanding its core concepts is essential for anyone starting with it. In this article, we’ll cover the fundamental building blocks of GraphQL: Queries, Mutations, Subscriptions, Schemas, and Resolvers.
Queries
A Query is how you fetch data from a GraphQL server. It’s equivalent to a GET request in REST APIs. The beauty of GraphQL queries is their flexibility; you can request exactly the data you need and nothing more.
Example:
Here’s a query to fetch a user’s name and email:
query {
user(id: "1") {
name
email
}
}
Step-by-Step Explanation:
query
: This keyword initiates a query operation.user(id: "1")
: Theuser
field specifies the type of data to fetch, withid: "1"
as the argument to identify the user.name
andemail
: These are the fields requested from theuser
type.
Result:
{
"data": {
"user": {
"name": "John Doe",
"email": "john.doe@example.com"
}
}
}
The server responds with only the requested fields, making data retrieval efficient and customizable.
Mutations
A Mutation is used to modify data on the server, such as creating, updating, or deleting resources. It’s similar to POST, PUT, or DELETE requests in REST APIs.
Example:
Here’s a mutation to create a new user:
mutation {
createUser(input: { name: "Jane Doe", email: "jane.doe@example.com" }) {
id
name
email
}
}
Step-by-Step Explanation:
mutation
: This keyword starts a mutation operation.createUser
: This is the mutation field that defines the action to perform (creating a user).input: { name: "Jane Doe", email: "jane.doe@example.com" }
: Theinput
object provides the necessary data for the mutation.id
,name
, andemail
: These are the fields to return from the newly created user object.
Result:
{
"data": {
"createUser": {
"id": "2",
"name": "Jane Doe",
"email": "jane.doe@example.com"
}
}
}
Mutations not only modify data but can also return data, such as the newly created or updated resource, in the same request.
Subscriptions
Subscriptions enable real-time communication between the client and server. They allow you to listen for specific events, such as changes to data, and receive updates in real-time. Subscriptions are particularly useful for applications like chat systems, live dashboards, or notifications.
Example:
Here’s a subscription to listen for new messages:
subscription {
messageAdded {
id
content
author
}
}
Step-by-Step Explanation:
subscription
: This keyword initiates a subscription operation.messageAdded
: The subscription field listens for events when a new message is added.id
,content
, andauthor
: These fields specify the data to receive when the event triggers.
Result (Real-Time):
When a new message is added, the server pushes the following update to the client:
{
"data": {
"messageAdded": {
"id": "101",
"content": "Hello, world!",
"author": "Alice"
}
}
}
Schema
The Schema is the backbone of a GraphQL API. It defines the structure of the data available in the API, including the types, queries, mutations, and subscriptions.
Example:
Here’s a basic schema for a user:
type User {
id: ID!
name: String!
email: String!
}
type Query {
user(id: ID!): User
}
type Mutation {
createUser(input: CreateUserInput): User
}
type Subscription {
messageAdded: Message
}
Step-by-Step Explanation:
type User
: Defines theUser
object with fieldsid
,name
, andemail
. The!
indicates that these fields are non-nullable.type Query
: Specifies theuser
query that returns aUser
object by taking anid
argument.type Mutation
: Defines thecreateUser
mutation, which accepts aninput
object and returns aUser
object.type Subscription
: Specifies themessageAdded
subscription for listening to new messages.
Resolvers
Resolvers are functions that handle the logic for each field in the schema. They fetch or manipulate data from the database or another source and return it to the client. Each field in the schema must have a corresponding resolver.
Example:
Here’s a resolver for the user
query:
func (r *queryResolver) User(ctx context.Context, id string) (*User, error) {
return database.GetUserByID(id)
}
Step-by-Step Explanation:
func (r *queryResolver) User
: This defines the resolver function for theuser
query.ctx context.Context
: The context object is used for handling deadlines, cancellation signals, and other request metadata.id string
: This parameter matches theid
argument in the schema.return database.GetUserByID(id)
: Fetches the user data from the database using the providedid
.
Resolvers are the link between your schema and your data sources, enabling the API to function dynamically.
Putting It All Together
To summarize, GraphQL’s core concepts provide a powerful framework for building APIs:
Queries: Fetch data flexibly.
Mutations: Modify and return data.
Subscriptions: Enable real-time updates.
Schema: Define the API’s structure and rules.
Resolvers: Implement the logic to serve data.
By mastering these concepts, you’ll be well on your way to building efficient and scalable GraphQL APIs. Start experimenting with these building blocks, and you’ll quickly see the power and simplicity of GraphQL!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
