Beginner-Friendly Guide to Stacks in JavaScript
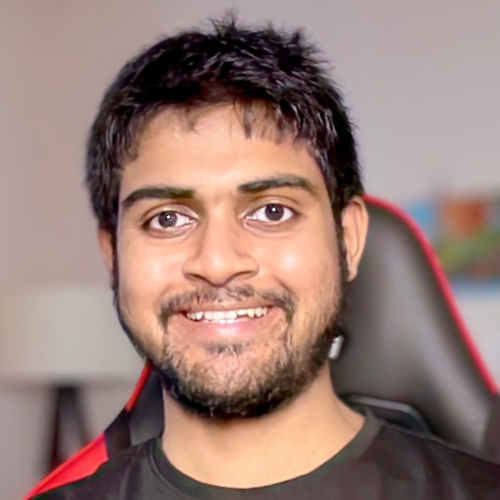
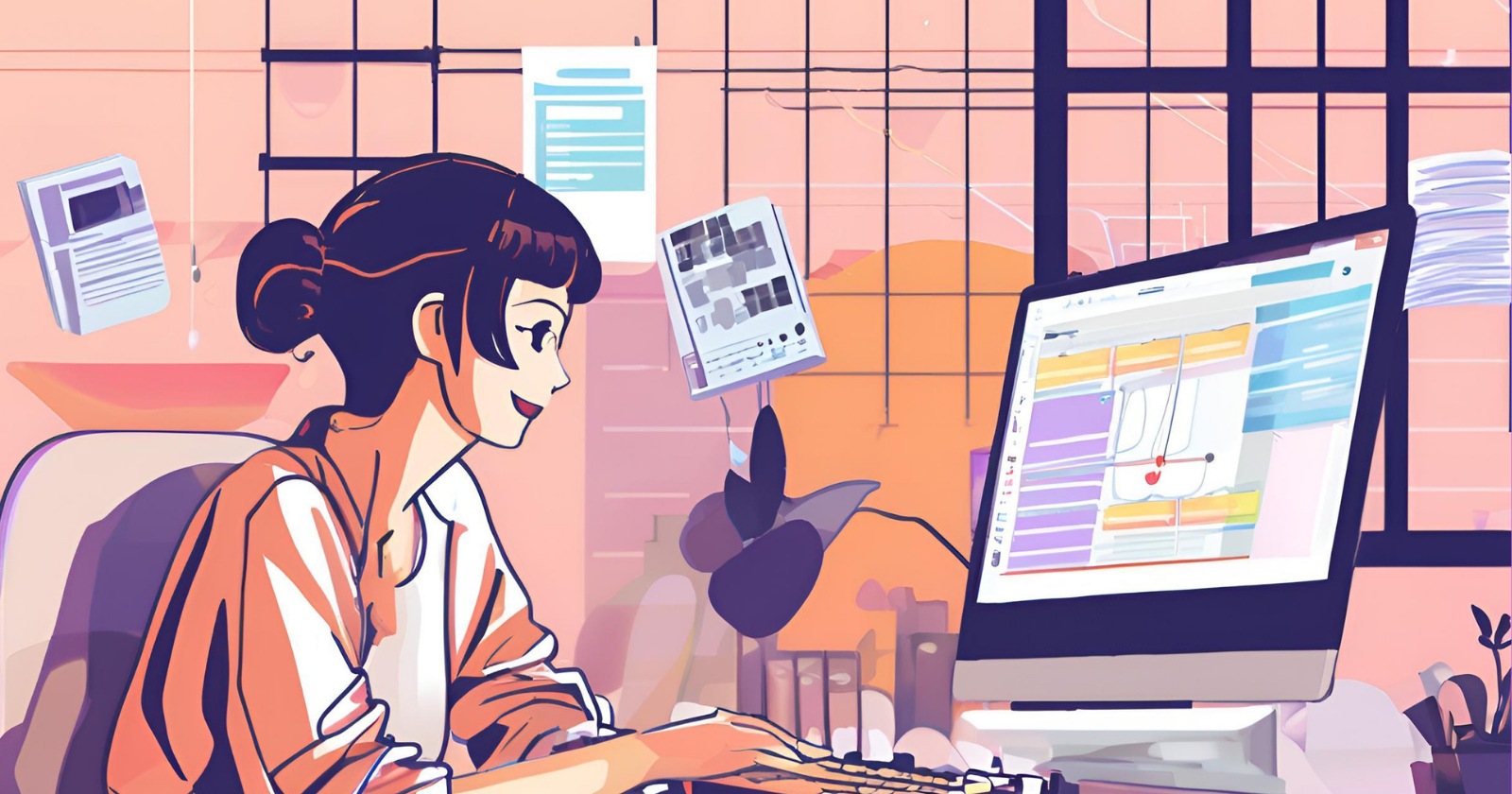
Stacks are a fundamental data structure in computer science. This tutorial will teach you how to implement a stack in JavaScript, focusing on the following operations:
Push: Add an element to the top of the stack.
Pop: Remove an element from the top of the stack.
IsEmpty: Check if the stack is empty.
IsFull: Check if the stack is full (for a stack with a fixed size).
Top/Peek: Retrieve the top element without removing it.
What Is a Stack?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means the last element added to the stack is the first one to be removed.
Implementation of a Stack in JavaScript
Here’s how you can create and use a stack:
class Stack {
constructor(maxSize) {
this.stack = [];
this.maxSize = maxSize; // Define the maximum size of the stack (optional)
}
// Push operation: Add an element to the top of the stack
push(element) {
if (this.isFull()) {
console.log("Stack is full. Cannot push element.");
return;
}
this.stack.push(element);
console.log(`${element} pushed to stack.`);
}
// Pop operation: Remove an element from the top of the stack
pop() {
if (this.isEmpty()) {
console.log("Stack is empty. Cannot pop element.");
return null;
}
const removedElement = this.stack.pop();
console.log(`${removedElement} popped from stack.`);
return removedElement;
}
// IsEmpty operation: Check if the stack is empty
isEmpty() {
return this.stack.length === 0;
}
// IsFull operation: Check if the stack is full (optional)
isFull() {
return this.stack.length >= this.maxSize;
}
// Top/Peek operation: Retrieve the top element without removing it
peek() {
if (this.isEmpty()) {
console.log("Stack is empty. No top element.");
return null;
}
return this.stack[this.stack.length - 1];
}
// Utility: Print the current stack
printStack() {
console.log("Current stack:", this.stack);
}
}
// Example usage
const myStack = new Stack(5); // Create a stack with a maximum size of 5
myStack.push(10); // Push elements to the stack
myStack.push(20);
myStack.push(30);
myStack.printStack();
console.log("Top element:", myStack.peek()); // Peek the top element
myStack.pop(); // Pop an element
myStack.printStack();
console.log("Is stack empty?:", myStack.isEmpty()); // Check if stack is empty
myStack.push(40);
myStack.push(50);
myStack.push(60);
myStack.push(70); // Attempt to push when stack is full
myStack.printStack();
console.log("Is stack full?:", myStack.isFull());
Explanation of Operations
Push:
Adds a new element to the end of the array (
this.stack.push(element)
).Checks if the stack is full before pushing.
Pop:
Removes and returns the last element from the array (
this.stack.pop()
).Ensures the stack is not empty before performing the operation.
IsEmpty:
- Returns
true
if the array's length is0
, otherwisefalse
.
- Returns
IsFull:
- Returns
true
if the stack's current size equals or exceeds the maximum size.
- Returns
Top/Peek:
- Accesses the last element in the array (
this.stack[this.stack.length - 1]
) without removing it.
- Accesses the last element in the array (
Output Example
10 pushed to stack.
20 pushed to stack.
30 pushed to stack.
Current stack: [ 10, 20, 30 ]
Top element: 30
30 popped from stack.
Current stack: [ 10, 20 ]
Is stack empty?: false
40 pushed to stack.
50 pushed to stack.
60 pushed to stack.
Stack is full. Cannot push element.
Current stack: [ 10, 20, 40, 50, 60 ]
Is stack full?: true
Conclusion
Stacks are an essential data structure used in various applications like expression evaluation, undo mechanisms, and more. This tutorial covered the basic operations of a stack using JavaScript. You can expand on this implementation to suit specific use cases or explore additional concepts like dynamic resizing of stacks.
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
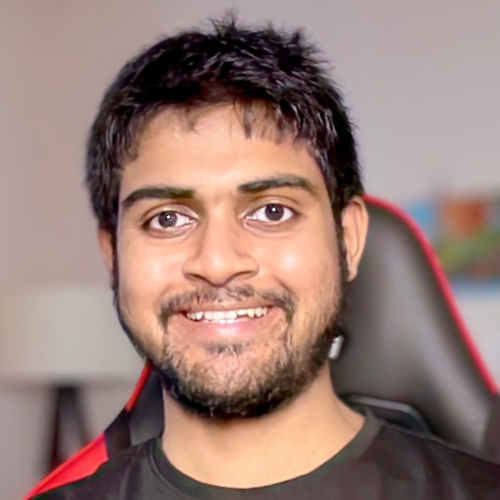
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.