Understanding xUnit: How Class Fixtures Work
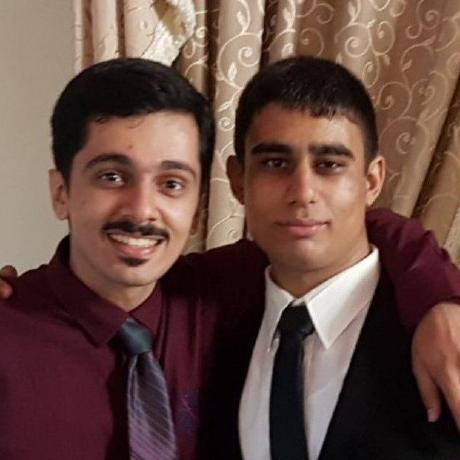

xUnit is a popular unit testing framework for .NET applications, known for its simplicity and flexibility, especially in how it manages test fixtures. In this blog, we'll dive into the concept of Class Fixtures and how they interact with test classes, using a practical example to illustrate the concepts.
What is a Class Fixture?
A Class Fixture in xUnit is designed to share context among tests in a test class. It's particularly useful when you need to setup and teardown resources that are needed across multiple tests but not within the scope of a single test method. Here’s how it works:
Initialization: The class fixture is instantiated once for the entire test class, rather than for each test method. This means you can initialize resources that are heavy or time-consuming to set up just once.
Cleanup: Similarly, cleanup operations are performed once after all test methods in the class have run, through the Dispose method if the fixture implements IDisposable.
The Test Class
A test class in xUnit can look like this:
public class UnitTest1 : IClassFixture<TestD>, IDisposable
{
private readonly ITestOutputHelper _testOutputHelper;
public UnitTest1(ITestOutputHelper testOutputHelper)
{
// Constructor of the test class, runs before any test method
}
[Fact]
public void Test1()
{
// A test method
}
[Fact]
public void Test2()
{
// Another test method
}
public void Dispose()
{
// Cleanup for the test class, runs after all tests
}
}
Key Points:
IClassFixture<T>: By implementing IClassFixture<TestD>, the test class tells xUnit to create one instance of TestD for all tests within this class.
ITestOutputHelper: This is injected into the constructor for logging purposes, which is useful for debugging.
The Class Fixture
Here's the fixture class:
public class TestD : IDisposable
{
public TestD()
{
// Runs before all tests in the class start
}
public void Dispose()
{
// Runs after all tests in the class are completed
}
}
Breakdown:
Constructor: Anything placed here will be executed once before the first test of the class runs. This is where you would initialize databases, setup services, etc.
Dispose: This method ensures that resources are cleaned up after all tests have finished running.
How They Relate
Initialization Order:
The fixture's constructor (TestD) runs before any test class constructor or test method in UnitTest1.
The test class constructor runs for each test method, allowing you to set up test-specific context.
Execution Order:
- Test methods (Test1, Test2) run in the order they are defined or as specified by attributes like [Theory] or [Fact].
Cleanup Order:
The Dispose method of the test class (UnitTest1) runs after each test method to clean up any test-specific resources.
Finally, the Dispose method of the fixture (TestD) runs once after all tests in the class have finished.
Practical Benefits
Resource Sharing: Avoids repetitive setup for shared resources.
Performance: Reduces the overhead by initializing heavy resources only once.
Consistency: Ensures a consistent state across tests where needed.
In the end
Understanding class fixtures in xUnit can significantly enhance your testing strategy by managing test setup and teardown more efficiently. This approach not only saves time but also keeps your tests clean and focused. Remember, the key to effective testing is not just writing tests but also structuring them to work harmoniously with your application's architecture and test environment. If you have any questions about it you can ask them in the comments.
Subscribe to my newsletter
Read articles from behrooz bozorgchamy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
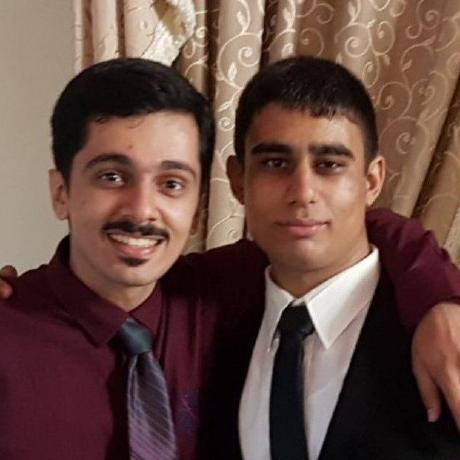