Next.js use cache directive
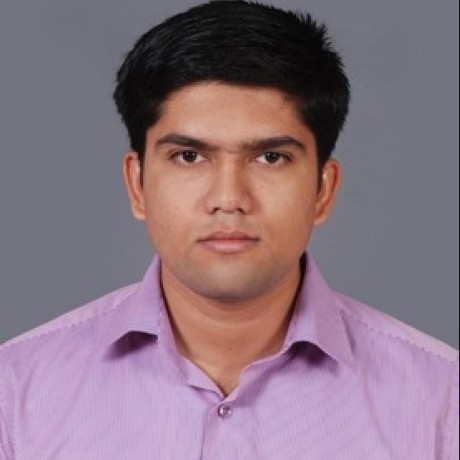

useCache directive from Next.js designates a component and/or a function to be cached. It can be used at the top of a file to indicate that all exports in the file are cacheable, or inline at the top of a function or component to inform Next.js the return value should be cached and reused for subsequent requests.
useCache is an experimental Next.js feature and not a native React feature like useClient
or use Server
.
1.What is use cache
useCache is a directive —a string literal you add in your code—which signals to the Next.js compiler to enter a different “boundary”. For example, going from the server to the client.
Directives are compiler instructions that define where code should run, allowing the framework to optimize and orchestrate individual pieces for you.
2.How to use use cache
To use the use cache directive you need to make the dynamicIO to true in next.config.ts like below
import type { NextConfig } from 'next'
const nextConfig: NextConfig = {
experimental: {
dynamicIO: true,
},
}
export default nextConfig
And we can use the use cache directive at the file, component or function level
// File level
'use cache'
export default async function Page() {
// ...
}
// Component level
export async function ProductComponent() {
'use cache'
return <></>
}
// Function level
export async function getData() {
'use cache'
const data = await fetch('/api/data')
return data
}
3.How it works?
Let’s go with a simple example
async function getProduct(id) {
'use cache';
let res = await fetch(`https://api.acme.app/product/${id}`);
return res.json();
}
Behind the scenes, Next.js transforms this code into a server function due to the 'use cache' directive. During compilation, the “dependencies” of this cache entry are found and used as part of the cache key.
For example, id becomes part of the cache key. If we call getProduct(5) multiple times, we return the memoized output from the cached server function. Changing this value will create a new entry in the cache.
4.Caching entire routes with use cache directive
To prerender an entire route, add use cache to the top both the layout and page files. Each of these segments are treated as separate entry points in your application, and will be cached independently.
'use cache'
import { unstable_cacheLife as cacheLife } from 'next/cache'
export default function Layout({ children }: { children: ReactNode }) {
return <div>{children}</div>
}
Any components imported and nested in page
file will inherit the cache behavior of page
.
'use cache'
import { unstable_cacheLife as cacheLife } from 'next/cache'
async function Products() {
const users = await fetch('/api/acme/products')
// loop through users
}
export default function Page() {
return (
<main>
<Products />
</main>
)
}
5.Caching component output using use cache
You can implement caching at the component level to store the results of fetch operations or computations within the component. When the component is reused across your application, it can share the same cache entry, provided the structure of the props remains consistent.
The cache key is generated based on the serialized props. As long as the serialized props yield the same value in each instance, the corresponding cache entry will be reused.
export async function Bookings({ type = 'vegan' }: BookingsProps) {
'use cache'
async function getBookingsData() {
const data = await fetch(`/api/bookings?type=${encodeURIComponent(type)}`)
return data
}
return //...
}
interface BookingsProps {
type: string
}
6.Caching function output with use cache
use cache
can be added to any asynchronous function, its use isn’t restricted to caching components or routes. You can cache various operations, such as network requests, database queries, or slow computations. By applying use cache to a function performing such tasks, the function becomes cacheable, and repeated calls will share the same cache entry, improving efficiency.
export async function getData() {
'use cache'
const data = await fetch('/api/data')
return data
}
7.Revalidating
By default, Next.js applies a revalidation period of 15 minutes when you use the use cache
directive. Additionally, it sets a near-infinite expiration duration, making it ideal for content that rarely requires updates.
While this default setting is suitable for static or infrequently changing content, you can fine-tune the caching behavior using the following APIs:
cacheLife
: Configure time-based revalidation intervals.cacheTag
: Enable on-demand revalidation for greater control.
These options allow you to adapt caching strategies to match your content's update frequency and requirements.
Subscribe to my newsletter
Read articles from nidhinkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
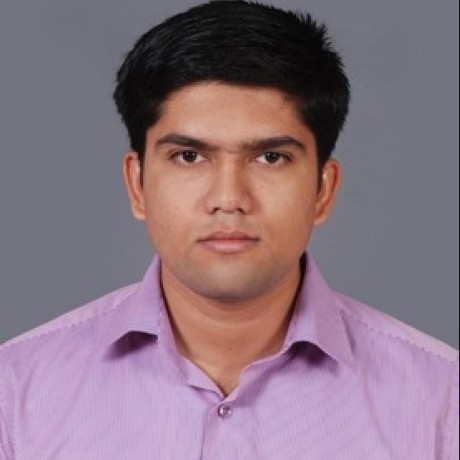