Building Your First GraphQL API with gqlgen

Table of contents
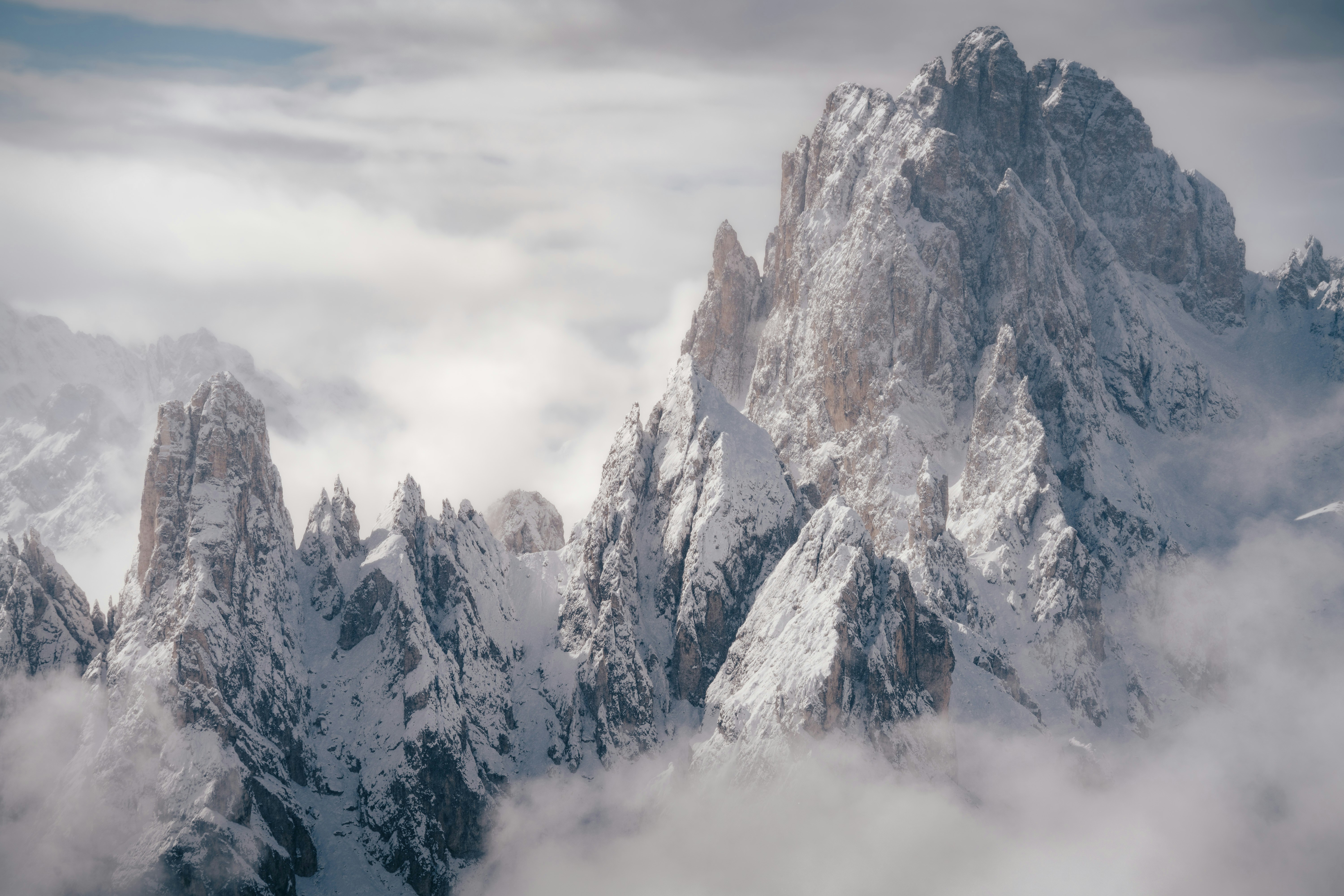
GraphQL is a powerful query language for APIs, and gqlgen
is one of the most efficient libraries for implementing GraphQL in Go. This step-by-step guide will help you build your first GraphQL API using gqlgen
. We'll define a simple schema, implement resolvers, run the server, and test the API using GraphQL Playground.
Why Use gqlgen?
Strong Type Safety: gqlgen generates code based on your GraphQL schema, ensuring that your API and resolvers match the schema.
Ease of Use: With gqlgen, you can get a fully functional GraphQL server up and running in minutes.
Customizability: You have complete control over the generated code and can easily customize it as needed.
Step 1: Install gqlgen
To get started, install gqlgen and its dependencies:
Install gqlgen:
go install github.com/99designs/gqlgen@latest
Verify Installation:
gqlgen version
- This command should display the installed version of gqlgen.
Step 2: Set Up Your Project
Step 2.1: Initialize a Go Module
Create a Project Directory:
mkdir gqlgen-hello-world cd gqlgen-hello-world
Initialize a Go Module:
go mod init gqlgen-hello-world
Step 2.2: Initialize gqlgen
Generate the Boilerplate Code:
gqlgen init
This command creates several files, including:
schema.graphqls
: A file to define your GraphQL schema.server.go
: The entry point for your server.resolver.go
: Contains resolver functions for your GraphQL queries and mutations.
Step 3: Define a Simple Schema
The schema defines the structure of your API, including its queries, mutations, and types. Let’s create a basic schema with a single query, hello
.
Edit the
schema.graphqls
File:type Query { hello: String! }
- This schema defines a
Query
type with a single field,hello
, which returns a non-nullable string (String!
).
- This schema defines a
Step 4: Implement Resolvers
Resolvers are functions that handle fetching data for the fields defined in your schema.
Edit the
resolver.go
File:Locate the
Hello
resolver function underqueryResolver
.Implement the function:
package gqlgen_hello_world // Resolver function for the "hello" query func (r *queryResolver) Hello() (string, error) { return "Hello, gqlgen!", nil }
Code Breakdown
queryResolver
: This struct contains all resolvers for query fields.Hello
Function: Returns the string "Hello, gqlgen!". Errors can also be returned if needed.
Step 5: Run the Server
To serve your API, you’ll use the auto-generated server.go
file.
Start the Server:
go run server.go
Access GraphQL Playground:
- Open your browser and navigate to
http://localhost:8080
. You’ll see the GraphQL Playground interface.
- Open your browser and navigate to
Step 6: Test Your API
GraphQL Playground allows you to interact with your API and send queries.
Send a Query:
Query:
query { hello }
View the Response:
Response:
{ "data": { "hello": "Hello, gqlgen!" } }
Additional Notes
Advantages of Using gqlgen
Code Generation: gqlgen auto-generates much of the boilerplate code, saving development time.
High Performance: gqlgen is optimized for performance, making it suitable for production use.
Extensibility: You can easily extend your API by adding new fields, types, and resolvers.
Next Steps
Expand the schema to include additional queries and mutations.
Integrate a database to make your API dynamic.
Implement features like authentication and authorization.
Congratulations! You’ve successfully built and tested your first GraphQL API using gqlgen. This foundation allows you to create more complex and powerful APIs to suit your application needs.
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
