Guide to Identity Resolution Algorithms for CDP
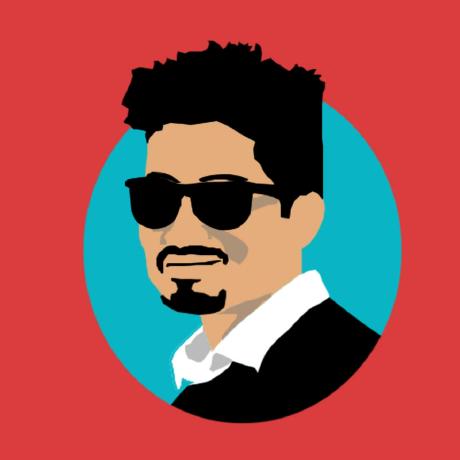

Identity resolution is the cornerstone of any Customer Data Platform (CDP). It involves consolidating disparate data points into unified customer profiles by resolving identities across multiple sources. This guide explores the algorithms, techniques, and challenges involved in building an identity resolution system.
1. Introduction to Identity Resolution
1.1 What is Identity Resolution?
Identity resolution is the process of matching and merging fragmented data about a single individual from various sources (web, mobile, CRM, email marketing tools) into a unified profile.
1.2 Why is it Important?
Enables personalized marketing by providing a holistic view of customer behavior.
Reduces data duplication and fragmentation.
Ensures compliance with GDPR and CCPA by consolidating data for deletion or access requests.
2. Key Steps in Identity Resolution
2.1 Data Collection
Sources: CRM, transactional databases, web analytics, mobile apps.
Types:
Personally Identifiable Information (PII): Name, email, phone, address.
Behavioral Data: Clickstreams, purchase history.
Device Data: Cookies, device IDs, IP addresses.
2.2 Data Preprocessing
Standardization:
- Convert all data to a consistent format (e.g., uppercase email addresses, remove special characters).
Normalization:
- Normalize phone numbers, addresses, and names using libraries like libphonenumber or usaddress.
3. High-Level Steps
Data Ingestion: Collect data from diverse sources.
Preprocessing: Standardize, clean, and normalize data.
Blocking: Group potential matches to reduce computational overhead.
Scoring: Compute match scores for candidate pairs.
Classification: Classify pairs as matches or non-matches using deterministic or ML-based models.
Clustering: Merge matched pairs into unified profiles.
Profile Generation: Store resolved profiles in a database for downstream applications.
4. The Algorithms
4.1 Data Ingestion
Input
Data streams or batch imports from multiple sources (CRM, web, mobile, e-commerce).
Example input schema:
{ "source": "CRM", "customer_id": "12345", "name": "John Doe", "email": "john.doe@example.com", "phone": "+1-202-555-0123", "address": "123 Elm St, Springfield", "device_id": "abc123", "ip_address": "192.168.1.1" }
Implementation
Use Apache Kafka or AWS Kinesis for real-time ingestion.
Use ETL pipelines (e.g., Apache Airflow) for batch ingestion.
4.2 Preprocessing
Standardization
Normalize text fields:
Convert to lowercase.
Remove special characters (e.g.,
+
,-
, spaces).Example (Python):
def normalize_text(text): return re.sub(r'[^a-zA-Z0-9]', '', text.lower())
Standardize numeric fields:
Normalize phone numbers using libraries like
libphonenumber
.Geocode addresses using APIs like Google Maps or OpenCage.
Error Handling
Handle missing or incomplete data:
def handle_missing(data): return {k: v if v else "UNKNOWN" for k, v in data.items()}
Deduplication
- Remove exact duplicates using a hash-based deduplication mechanism.
4.3 Blocking
Purpose
Reduce the comparison space by grouping similar records.
Techniques
Hash-Based Blocking:
Create a hash of key fields (e.g., first 3 letters of last name + ZIP code).
Group records by hash.
def hash_block(record):
return hash(record['name'][:3] + record['zip'])
Sorted Neighborhood:
- Sort records by key attributes (e.g., name) and compare within a sliding window.
def sorted_neighborhood(records, window=5):
records.sort(key=lambda x: x['name'])
for i in range(len(records) - window):
yield records[i:i + window]
Canopy Clustering:
- Use a loose similarity metric (e.g., Jaro-Winkler) to group records.
Output
- Candidate record pairs for matching.
4.4 Scoring
Attribute-Level Matching
String Similarity:
- Use metrics like Jaro-Winkler or Levenshtein distance for names and addresses.
from jellyfish import jaro_winkler
similarity = jaro_winkler("John Doe", "Jon Doe")
Numeric Similarity:
- Compare phone numbers or ZIP codes using exact matching or range checks.
Categorical Matching:
- Use exact or fuzzy matching for categories like city or state.
Weighted Scoring
Assign weights to attributes based on importance:
weights = { 'email': 0.5, 'phone': 0.3, 'name': 0.2 } def calculate_score(record1, record2, weights): score = 0 if record1['email'] == record2['email']: score += weights['email'] if jaro_winkler(record1['name'], record2['name']) > 0.8: score += weights['name'] return score
4.5 Classification
Rule-Based Classification
Define thresholds for deterministic matching:
THRESHOLD = 0.7 if calculate_score(record1, record2, weights) >= THRESHOLD: match = True
Machine Learning-Based Classification
Feature Engineering:
Features: String similarity scores, categorical matches, numeric differences.
Example:
features = [ jaro_winkler(record1['name'], record2['name']), record1['email'] == record2['email'], abs(record1['age'] - record2['age']) ]
Model Training:
- Train a supervised model (e.g., Random Forest, XGBoost) on labeled data.
from sklearn.ensemble import RandomForestClassifier
model = RandomForestClassifier()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
4.6 Clustering
Graph-Based Clustering
Represent records as a graph:
Nodes: Records.
Edges: Matches above a threshold.
Identify connected components:
- Use BFS or DFS to find clusters.
import networkx as nx
G = nx.Graph()
G.add_edges_from(matched_pairs)
clusters = list(nx.connected_components(G))
DBSCAN Clustering
Use DBSCAN for density-based clustering of similarity scores.
from sklearn.cluster import DBSCAN clustering = DBSCAN(eps=0.5, min_samples=2).fit(similarity_matrix)
4.7 Profile Generation
Merge matched records:
Combine attributes from all records in a cluster.
Resolve conflicts (e.g., take the most recent or most frequent value).
def merge_profiles(cluster):
unified_profile = {}
for record in cluster:
for key, value in record.items():
unified_profile[key] = resolve_conflict(unified_profile.get(key), value)
return unified_profile
Store profiles in a NoSQL database (e.g., MongoDB):
db.profiles.insert_one(unified_profile)
5. Optimization Techniques
5.1 Parallelization
- Use distributed frameworks like Apache Spark for parallel processing of record comparisons.
5.2 Incremental Matching
- Only compare new records with existing profiles to avoid re-processing.
5.3 Real-Time Systems
- Implement streaming pipelines with Kafka Streams or Apache Flink.
6. Metrics for Evaluation
Precision: Percentage of correctly matched pairs.
Recall: Percentage of true matches identified.
F1 Score: Harmonic mean of precision and recall.
Throughput: Records processed per second.
If you want to talk about ad-tech or mar-tech product, then feel free to visit me at AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
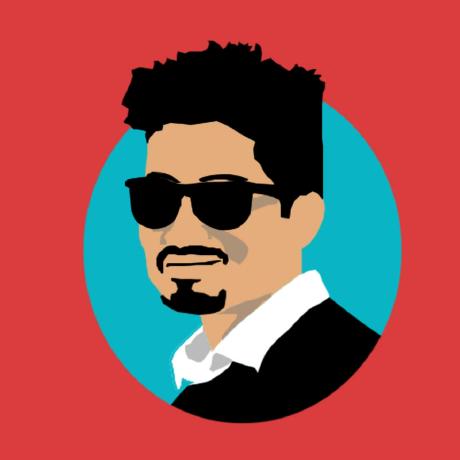