Pagination Basics for New Developers: An Easy-to-Follow Guide
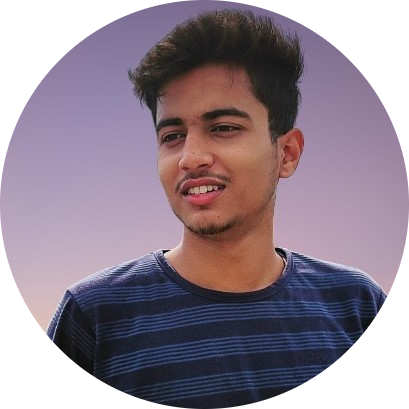

Pagination is a fundamental concept in web development that enhances user experience and performance by breaking down large datasets into smaller, manageable chunks. This guide will walk you through the concept of pagination, its importance, and how to implement it in your projects.
What is Pagination?
Pagination is a technique used to divide content across multiple pages rather than displaying all the data at once. Imagine you're browsing an online store with thousands of products—loading all of them at once would take a long time and overwhelm the user. Pagination addresses this by showing a limited number of items per page, with navigation controls to move between pages.
Why is Pagination Important?
Improved Performance: Reduces the amount of data sent to the client, speeding up page load times.
Enhanced User Experience: Simplifies navigation by presenting data in smaller, digestible chunks.
Optimized Resource Usage: Limits server and database load by fetching only the required data.
How Does Pagination Work?
Pagination typically involves two key components:
Backend Logic: Determines which subset of data to send based on the page number and items per page.
Frontend Logic: Displays the data and provides navigation options for users to switch between pages.
Example Implementation
Let’s create a basic pagination system using Node.js (backend) and React (frontend).
Backend: Node.js
In this example, we’ll assume you’re fetching data from a MongoDB database.
const express = require('express');
const mongoose = require('mongoose');
const app = express();
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const Item = mongoose.model('Item', new mongoose.Schema({ name: String }));
// Pagination endpoint
app.get('/items', async (req, res) => {
const page = parseInt(req.query.page) || 1; // Default to page 1
const limit = parseInt(req.query.limit) || 10; // Default to 10 items per page
const skip = (page - 1) * limit; // Calculate items to skip
const total = await Item.countDocuments(); // Total items in the database
const items = await Item.find().skip(skip).limit(limit); // Fetch paginated items
res.json({
total,
page,
totalPages: Math.ceil(total / limit),
items,
});
});
app.listen(3000, () => console.log('Server running on port 3000'));
Frontend: React
Here’s how you can display the paginated data and provide navigation.
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const PaginatedList = () => {
const [items, setItems] = useState([]);
const [page, setPage] = useState(1);
const [totalPages, setTotalPages] = useState(0);
useEffect(() => {
const fetchItems = async () => {
const response = await axios.get(`/items?page=${page}&limit=10`);
setItems(response.data.items);
setTotalPages(response.data.totalPages);
};
fetchItems();
}, [page]);
const handlePrevious = () => {
if (page > 1) setPage(page - 1);
};
const handleNext = () => {
if (page < totalPages) setPage(page + 1);
};
return (
<div>
<ul>
{items.map(item => (
<li key={item._id}>{item.name}</li>
))}
</ul>
<div>
<button onClick={handlePrevious} disabled={page === 1}>
Previous
</button>
<span>Page {page} of {totalPages}</span>
<button onClick={handleNext} disabled={page === totalPages}>
Next
</button>
</div>
</div>
);
};
export default PaginatedList;
Tips for Effective Pagination
Default Page and Limit: Always define default values to handle missing or invalid query parameters.
Error Handling: Ensure your backend handles cases where
page
orlimit
exceeds the total number of items.Accessibility: Use clear and accessible navigation controls for better usability.
SEO: If your pagination is used for public content, implement proper metadata and canonical tags.
Conclusion
Pagination is an essential tool for managing large datasets efficiently. By combining backend logic to fetch data dynamically and frontend logic for user navigation, you can build a seamless and scalable user experience. Start practicing today by implementing pagination in your own projects!
Subscribe to my newsletter
Read articles from Saurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
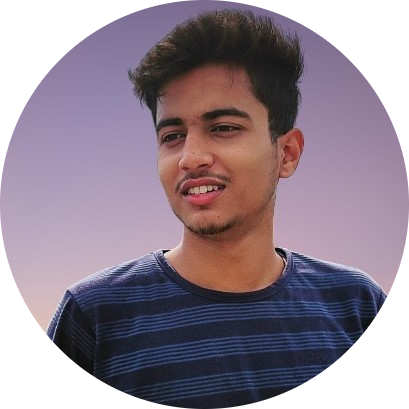
Saurav Kumar
Saurav Kumar
Build WebApps | Powered by Next.js, Node.js, Express.js, MongoDB & many more Styling, Auth, Db, Security, Deployment Tools & Techs