Kotlin : Sequences for Efficient Data Processing
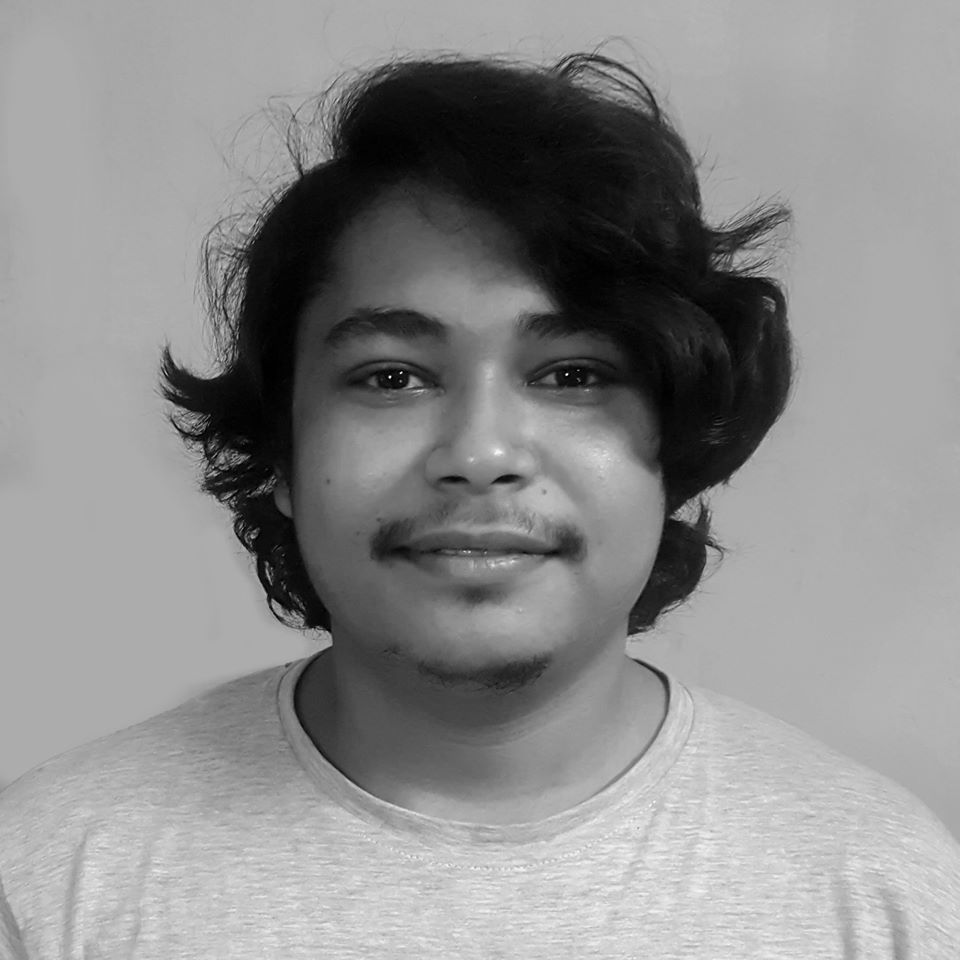
Table of contents
- What Are Sequences in Kotlin?
- This lazy approach makes sequences great for handling large datasets or when you need to perform several transformations, as they use less memory and run faster.
- Example Use Case: Complex Filtering with Sequences
- Step-by-Step Explanation
- Why Use Sequences?
- When Not to Use Sequences
- Conclusion
- Kotlin sequences are a useful tool for processing collections efficiently, especially with large datasets or complex transformation pipelines. By using lazy evaluation, sequences help lower memory use and boost performance.

In Kotlin, efficiently handling collections is important when working with large datasets or performing multiple transformations. One tool Kotlin offers for these situations is sequences, which allow lazy evaluation to reduce memory usage and improve performance.
In this article, we'll look at how to use sequences with multiple filtering criteria, focusing on situations where we want to process a list efficiently.
What Are Sequences in Kotlin?
A sequence in Kotlin is a type of collection that uses lazy evaluation. Unlike regular collections like List
or Set
, sequences don't create intermediate results. Instead, transformations like map
or filter
are only applied when a final operation like toList
or toSet
is called.
This lazy approach makes sequences great for handling large datasets or when you need to perform several transformations, as they use less memory and run faster.
Example Use Case: Complex Filtering with Sequences
Let's consider a scenario where we have a list of skills, and we need to:
Extract the
id
of each skill.Apply multiple filtering criteria.
Convert the filtered IDs into a
Set
for quick lookup.
Here's how we can accomplish this using sequences:
// Sample data class and input list
data class Skill(val id: Int, val name: String, val isActive: Boolean, val proficiency: Int, val category: String)
val skillsList = listOf(
Skill(1, "Kotlin", true, 5, "Programming"),
Skill(2, "Java", false, 4, "Programming"),
Skill(3, "Python", true, 3, "Programming"),
Skill(4, "JavaScript", true, 4, "Web Development"),
Skill(5, "HTML", true, 2, "Web Development"),
Skill(6, "CSS", true, 3, "Web Development"),
Skill(7, "SQL", false, 4, "Database"),
Skill(8, "MongoDB", true, 3, "Database")
)
// Processing the list with sequences
val filteredIds = skillsList
.asSequence() // Convert to a sequence for lazy processing
.filter { it.isActive } // Filter active skills
.filter { it.proficiency >= 4 } // Filter by proficiency level (e.g., 4 or higher)
.filter { it.category == "Programming" } // Additional filter for the "Programming" category
.map { it.id } // Extract the IDs
.toSet() // Terminal operation to produce a Set
println(filteredIds) // Output: [1]
Step-by-Step Explanation
asSequence()
: Turns theskillsList
into a sequence, allowing lazy evaluation for the next steps. This prevents creating extra collections during transformations.filter { it.isActive }
: Keeps only the active skills (isActive == true
) in the sequence.filter { it.proficiency >= 4 }
: Adds another filter to keep only skills with a proficiency level of 4 or higher.filter { it.category == "Programming" }
: Further limits the results to skills in the "Programming" category.map { it.id }
: Changes the filtered sequence by pulling out theid
of each skill.toSet()
: Gathers the resultingid
s into aSet
, ensuring they are unique and easy to look up.
Why Use Sequences?
Using sequences in this scenario is beneficial for several reasons:
Memory Efficiency: Without
asSequence()
, each transformation (likefilter
ormap
) creates an intermediate collection, using more memory. Sequences avoid this by applying transformations lazily.Performance Optimization: For large datasets, sequences reduce the number of iterations by combining transformations and applying them only when needed.
Flexibility with Complex Pipelines: Sequences are perfect for pipelines with multiple transformations, as they make it easy to chain operations without worrying about intermediate collections.
When Not to Use Sequences
While sequences are powerful, they are not always the best choice. Avoid using sequences when:
The dataset is small, as the overhead of creating a sequence might outweigh the benefits of lazy evaluation.
You need to access elements multiple times, as sequences process elements only once.
Conclusion
Kotlin sequences are a useful tool for processing collections efficiently, especially with large datasets or complex transformation pipelines. By using lazy evaluation, sequences help lower memory use and boost performance.
In our example, we showed how to filter and process a list of skills using sequences and multiple criteria, leading to a compact and efficient solution. Use sequences in your Kotlin projects to manage complex data operations easily!
That’s it for today. Happy Coding…
Subscribe to my newsletter
Read articles from Romman Sabbir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
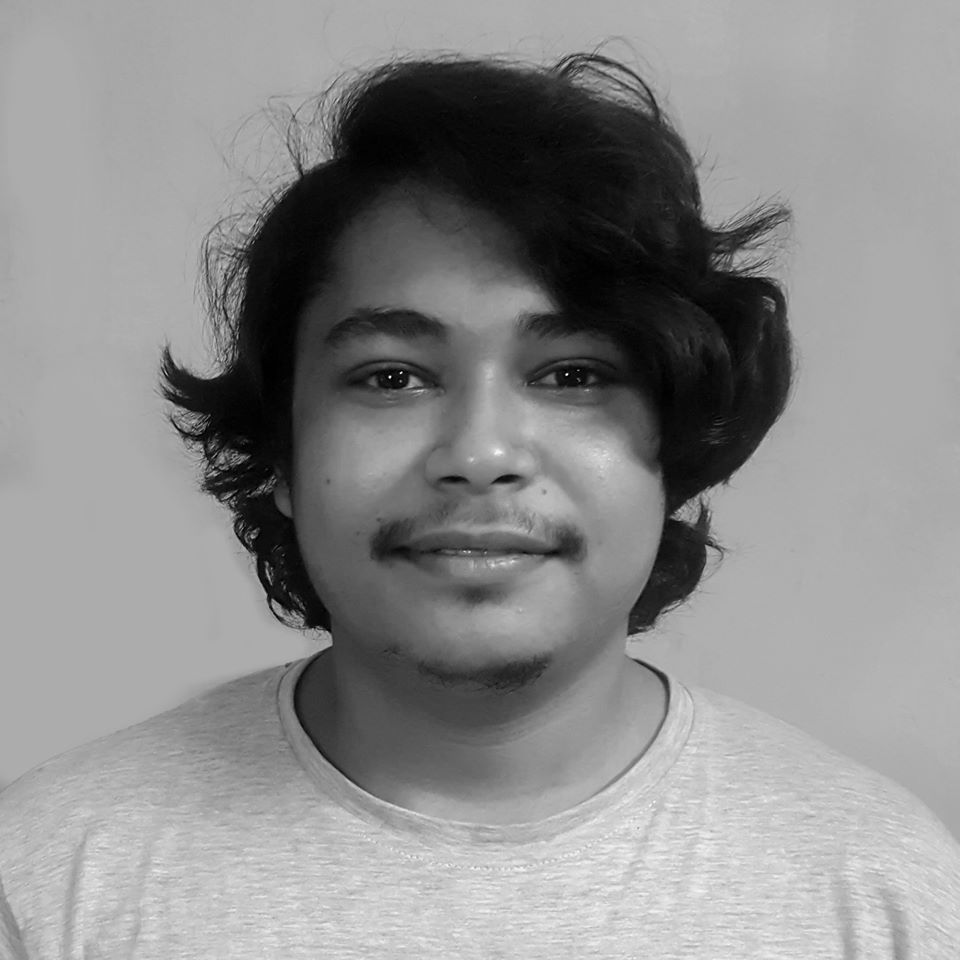
Romman Sabbir
Romman Sabbir
Senior Android Engineer from Bangladesh. Love to contribute in Open-Source. Indie Music Producer.