Application Load Balancer in AWS using Terraform

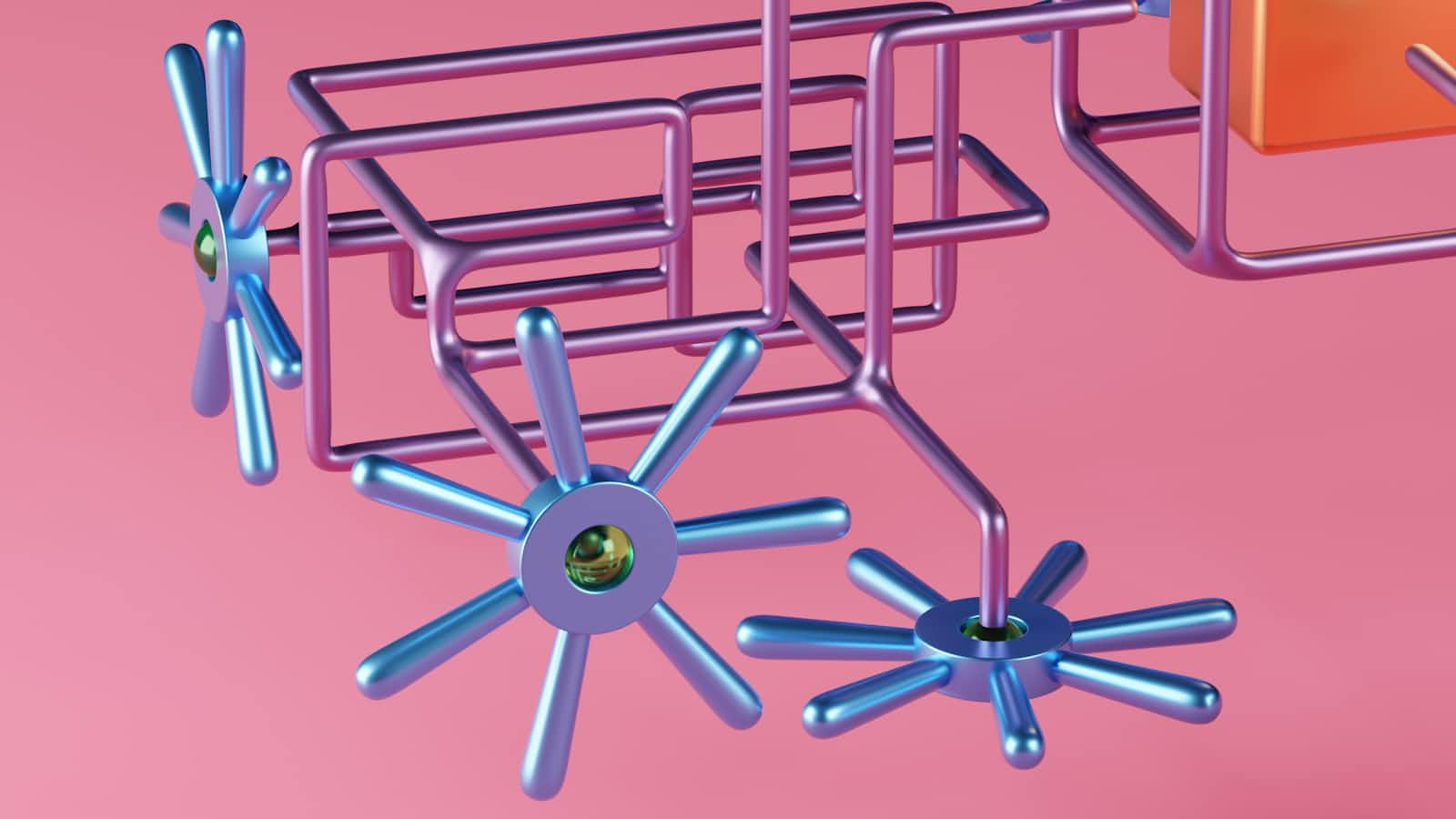
Introduction
Here is a scenario for implementing ALB in AWS using Terraform
Create ALB using terraform and select all its dependencies
Access different components of an app, for example, vote app running on private instance using ALB.
Ensure running of a complete micro-service like vote app by using docker compose file in Jenkins.
Step by step Guide
1. Create an ALB Using Terraform and Select Its Dependencies
To create an Application Load Balancer (ALB) in AWS using Terraform, you must define its dependencies like subnets, security groups, and target groups.
Terraform Code Example:
hclCopy code# Provider setup
provider "aws" {
region = "us-east-1"
}
# VPC and Subnet
resource "aws_vpc" "main" {
cidr_block = "10.0.0.0/16"
}
resource "aws_subnet" "public" {
vpc_id = aws_vpc.main.id
cidr_block = "10.0.1.0/24"
map_public_ip_on_launch = true
}
# Security Group
resource "aws_security_group" "alb_sg" {
vpc_id = aws_vpc.main.id
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
# Target Group
resource "aws_lb_target_group" "tg" {
name = "vote-app-tg"
port = 80
protocol = "HTTP"
vpc_id = aws_vpc.main.id
}
# Application Load Balancer
resource "aws_lb" "alb" {
name = "vote-app-alb"
internal = false
security_groups = [aws_security_group.alb_sg.id]
subnets = [aws_subnet.public.id]
load_balancer_type = "application"
}
# Listener
resource "aws_lb_listener" "http" {
load_balancer_arn = aws_lb.alb.arn
port = 80
protocol = "HTTP"
default_action {
type = "forward"
target_group_arn = aws_lb_target_group.tg.arn
}
}
Dependencies:
VPC and Subnets: Ensure you have a VPC and at least two public subnets in different availability zones.
Security Groups: Configure ALB to allow HTTP traffic.
Target Group: Define the backend instances or services ALB will route traffic to.
2. Access Different Components of an Application Using ALB
If the vote app is running on private instances, configure the ALB and Target Group properly:
Register Instances with Target Group:
- Ensure the private instances (where the vote app is running) are part of the target group created above. This can be done in Terraform:
hclCopy coderesource "aws_lb_target_group_attachment" "tg_attach" {
target_group_arn = aws_lb_target_group.tg.arn
target_id = "i-xxxxxxxxxxxxxxxxx" # Instance ID
port = 80
}
Route Different Paths to Specific Components:
- If the app has different components accessible via specific paths (e.g.,
/vote
,/results
), configure path-based routing in the ALB listener rules:
- If the app has different components accessible via specific paths (e.g.,
hclCopy coderesource "aws_lb_listener_rule" "vote_rule" {
listener_arn = aws_lb_listener.http.arn
priority = 1
conditions {
field = "path-pattern"
values = ["/vote*"]
}
actions {
type = "forward"
target_group_arn = aws_lb_target_group.tg.arn
}
}
Access the App:
- Once set up, accessing
http://<ALB-DNS-Name>/vote
routes the traffic to the backend service hosting the voting component.
- Once set up, accessing
3. Run a Complete Microservice (e.g., Vote App) Using Docker Compose in Jenkins
To orchestrate running a multi-container app using docker-compose
via Jenkins, follow these steps:
Steps to Implement:
Prepare Docker Compose File:
Example
docker-compose.yml
for the Vote App:yamlCopy codeversion: '3' services: vote: image: voting-app:latest ports: - "5000:5000" networks: - app-network redis: image: redis:alpine networks: - app-network worker: image: worker-app:latest networks: - app-network db: image: postgres:latest environment: POSTGRES_USER: user POSTGRES_PASSWORD: password networks: - app-network results: image: results-app:latest ports: - "5001:5001" networks: - app-network networks: app-network: driver: bridge
Jenkins Pipeline to Deploy the Application:
Create a Jenkins pipeline script (
Jenkinsfile
) to deploy the app:groovyCopy codepipeline { agent any stages { stage('Checkout') { steps { checkout scm } } stage('Build Images') { steps { sh 'docker-compose build' } } stage('Deploy Containers') { steps { sh 'docker-compose up -d' } } stage('Health Check') { steps { sh 'curl -f http://localhost:5000 || exit 1' } } } post { always { sh 'docker-compose down' } } }
Integrate Jenkins with Docker:
Ensure Jenkins can communicate with Docker:
Install the Docker Pipeline plugin in Jenkins.
Provide Jenkins user access to Docker CLI (
sudo usermod -aG docker jenkins
).Test the Docker setup in Jenkins by running a simple
docker run
command.
Run the Pipeline:
- Trigger the Jenkins pipeline to build, deploy, and test the Vote App.
Summary
Terraform creates ALB with subnets, security groups, and target groups.
ALB routes traffic to app components using path-based rules.
Jenkins automates deploying a Docker Compose-based microservice.
Subscribe to my newsletter
Read articles from Siddhesh Prabhugaonkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Siddhesh Prabhugaonkar
Siddhesh Prabhugaonkar
I'm Siddhesh, a Microsoft Certified Trainer and cloud architect. As an instructor at Pluralsight, I'm dedicated to providing top-notch educational content. I share my expertise in Microsoft .NET, Azure, cloud technologies, certifications, and the latest in the tech world through various channels, including YouTube, online courses, blogs, and newsletters. Stay informed and up-to-date by subscribing to my newsletter at Cloud Authority.