Chapter 14: Functions and Objects
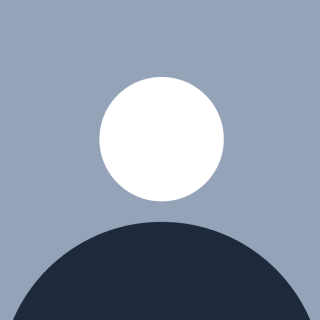
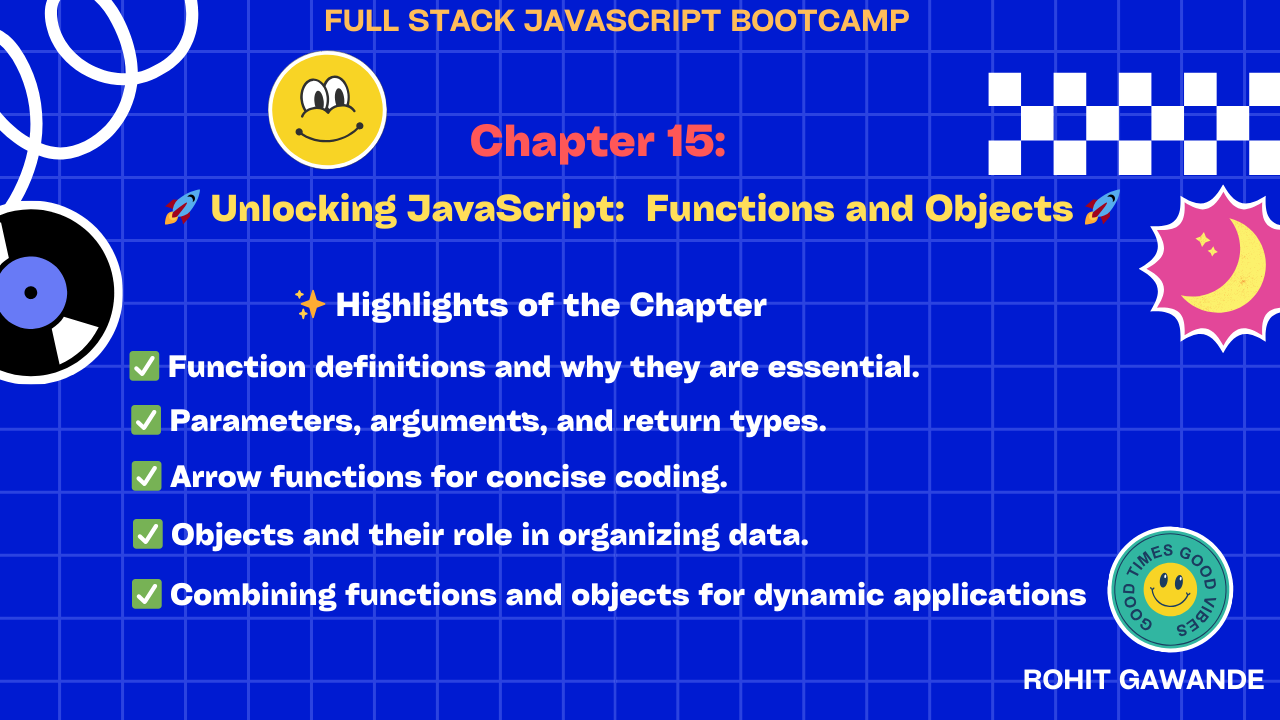
Introduction
Functions and objects are fundamental concepts in JavaScript, enabling developers to create reusable, modular, and efficient code. This chapter explores these concepts in depth, covering function definitions, parameters, return types, and objects. We'll also examine how to combine functions and objects to build dynamic and robust applications.
Part 1: Functions
1. Function Definition
A function is a block of code designed to perform a specific task. You define a function once and reuse it multiple times, making your code modular and easier to maintain.
Example:
function sum() {
console.log("Sum of two numbers:", 20 + 10);
}
sum();
2. Why Do We Need Functions?
Functions:
Reduce code repetition.
Increase readability.
Make debugging easier by isolating logic.
Enable modular programming by separating concerns.
3. Function Parameters and Arguments
Parameters allow you to pass data into a function, making it more versatile.
Example:
function sub(value1, value2) {
console.log("Difference of two numbers:", value1 - value2);
}
sub(12, 10); // Outputs: Difference of two numbers: 2
4. Return Type
Functions can return values to the caller, enabling further operations.
Example:
function mul(value1, value2) {
return value1 * value2;
}
let result = mul(11, 11);
console.log("Product of two numbers:", result); // Outputs: Product of two numbers: 121
5. Homework: Basic Operations
Hereโs a simple task for practicing functions:
Problem: Implement functions for addition, subtraction, multiplication, and division.
Solution:
let num1 = 20;
let num2 = 10;
function sum() {
console.log("Sum of two numbers:", num1 + num2);
}
function sub() {
console.log("Difference of two numbers:", num1 - num2);
}
function mul() {
console.log("Product of two numbers:", num1 * num2);
}
function div() {
console.log("Quotient of two numbers:", num1 / num2);
}
sum();
sub();
mul();
div();
Part 2: Objects
1. Introduction to Objects
Objects are collections of properties and methods. They represent real-world entities and provide a way to group related data and functions.
Example:
let userName = {
firstName: 'Rohit',
lastName: 'Gawande',
age: 20,
role: 'Admin'
};
console.log(userName.firstName); // Outputs: Rohit
userName.age = 21;
console.log(userName.age); // Outputs: 21
2. Iterating Through Objects
Using a for...in
loop, you can iterate through an object's properties.
Example:
for (let key in userName) {
console.log(`${key}: ${userName[key]}`);
}
Part 3: Advanced Function Usage
1. Arrow Functions
Arrow functions provide a concise syntax for defining functions.
Example:
let print = (var1) => {
console.log('I am Rohit, My age:', var1);
};
print(20); // Outputs: I am Rohit, My age: 20
2. Functions with Arrays
You can pass arrays as arguments and manipulate them within functions.
Example:
function sum(arr) {
let total = 0;
for (let i = 0; i < arr.length; i++) {
total += arr[i];
}
return total;
}
let numbers = [1, 2, 3, 4, 5];
console.log("Sum of array elements:", sum(numbers)); // Outputs: 15
Part 4: Combining Functions and Objects
1. Creating Dynamic URLs
Combine strings using functions to generate URLs dynamically.
Example:
function URL(base, domain) {
return `https://${base}${domain}`;
}
let site = URL('RohitG', '.com');
console.log(site); // Outputs: https://RohitG.com
Part 5: Practice Problems
Problem 1: Implement Object Methods
Create an object calculator
with methods for addition, subtraction, multiplication, and division.
Problem 2: Sum of Arbitrary Arguments
Write a function that calculates the sum of an arbitrary number of arguments using arguments
.
Solution:
function sum() {
let total = 0;
for (let i = 0; i < arguments.length; i++) {
total += arguments[i];
}
return total;
}
console.log(sum(1, 2, 3, 4, 5)); // Outputs: 15
Conclusion
Functions and objects are essential tools for efficient JavaScript programming. By mastering these concepts, you can write code that is reusable, modular, and easier to debug.
This chapter covered:
Function definitions, parameters, and return types.
Objects and their properties.
Combining functions and objects for dynamic applications.
Other Series:
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repository and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
๐ Tech Enthusiast | Full Stack Developer | System Design Explorer ๐ป Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iโm Rohit Gawande! ๐I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iโm Currently Doing ๐น Writing an in-depth System Design Series to help developers master complex design concepts.๐น Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.๐น Exploring advanced Java concepts and modern web technologies. What You Can Expect Here โจ Detailed technical blogs with examples, diagrams, and real-world use cases.โจ Practical guides on Java, System Design, and Full Stack Development.โจ Community-driven discussions to learn and grow together. Letโs Connect! ๐ GitHub โ Explore my projects and contributions.๐ผ LinkedIn โ Connect for opportunities and collaborations.๐ LeetCode โ Check out my problem-solving journey. ๐ก "Learning is a journey, not a destination. Letโs grow together!" Feel free to customize or add more based on your preferences! ๐