Become expert in React Query
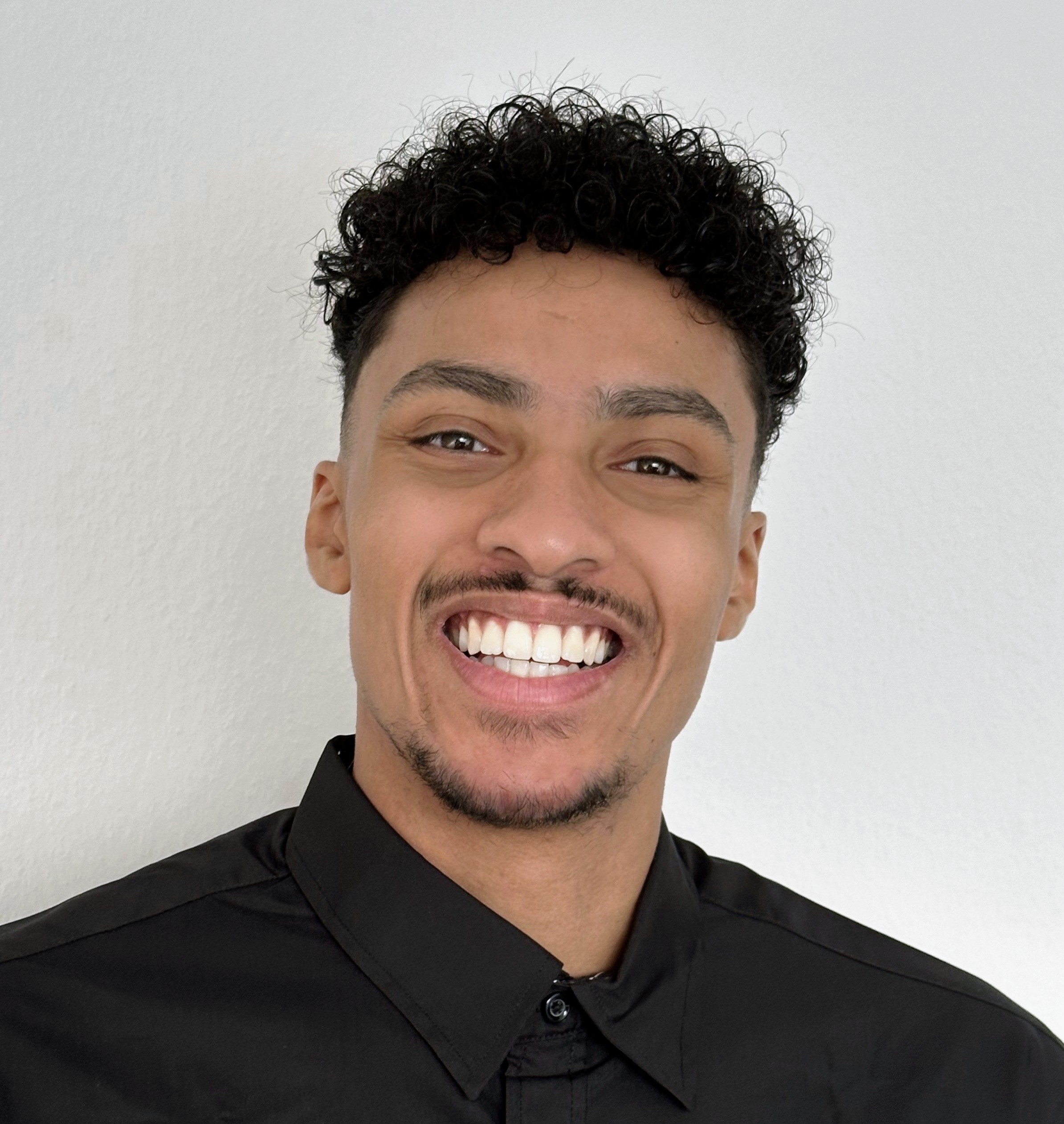
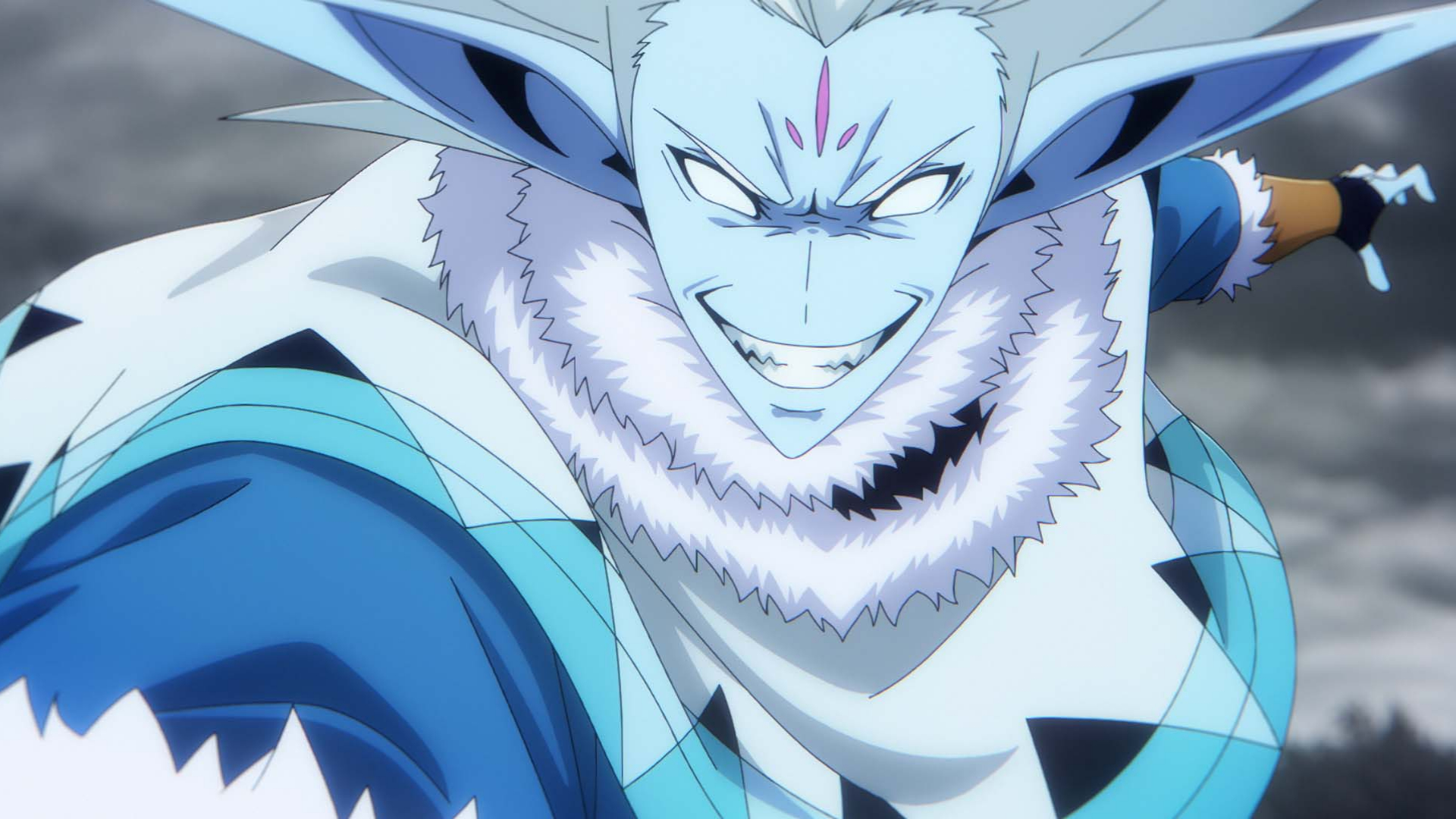
Introduction
I've used React Query for a while and built cool things with it. I’m writing this post to my younger self.
This post is for people who have already touched React Query, but not leveraged it's full power. If you’ve not worked with yet, go try it out first.
I'll preface this by saying read the documentation along the way. They're fantastic and have practical examples for everything I'll cover.
Big shout out to my friend TkDodo for proof reading this. ❤️
Glossary
Let me introduce you to some React Query terms that confused me at first. If you still don't understand any of them, that's totally fine. They'll make more sense in the end.
Stale: When data might be outdated. In React Query, stale data is still shown to users while fresh data loads.
// Data becomes stale after 5 minutes
useQuery({ staleTime: 1000 * 60 * 5 });
Cache: Where React Query stores your data. Data stays here until it's both:
Inactive (no components are using it)
Hasn't been used for the duration of gcTime
useQuery({
queryKey: ['todos'],
// If this query becomes inactive (no components using it),
// wait 10 minutes before removing it from cache
gcTime: 1000 * 60 * 10
})
To be explicitly clear what I mean by “no components using it”:
// Component A
function TodoList() {
// This query is "active" because the component is using it
const { data } = useQuery({
queryKey: ['todos'],
gcTime: 1000 * 60 * 5 // 5 minutes
})
return <div>{data.map(...)}</div>
}
// When TodoList unmounts (user navigates away), the query becomes "inactive"
// If user doesn't come back to TodoList within 5 minutes (gcTime),
// the data is removed from cache
// If they return within 5 minutes, the cached data is still there!
Invalidation: Telling React Query "hey, this data might be outdated, time to fetch fresh data!"
// After adding a todo, tell React Query todos list needs refresh
queryClient.invalidateQueries({ queryKey: ["todos"] });
Understanding React Query
Here's the thing about React Query that took me a while to truly get: it's not just a data fetching library. It's a server state manager. And to get whatever data you need, it only cares about you giving it a function that returns a Promise (doesn’t need to be fetch).
Think about it this way, your server data is like a snapshot that can go stale any second. That user profile you just fetched? Someone might have just updated it. That list of todos? Another device might have added to it.
React Query gets this. When you pass it a function that returns a Promise (through queryFn), it doesn't just fetch and forget. It:
Handles refetching in the background
Manages loading and error states
Caches results intelligently
The architecture might look complex (see the image above, taken from TkDodo's post), but you don't need to worry about most of it. Your job is simple: give React Query a way to get your data (function returning a Promise), and it handles all the complex state management behind the scenes.
A common mistake people do is try to combine useEffect and useQuery. useQuery already handles the state for you. If you're using a useEffect to somehow manage what you get from useQuery, you're doing it wrong.
// ❌ Wrong: Unnecessary useEffect with useQuery
function TodoList() {
const [todos, setTodos] = useState([])
const { data } = useQuery({
queryKey: ['todos'],
queryFn: () => fetch('/api/todos').then(r => r.json())
})
// Don't do this! useQuery already manages this for you
useEffect(() => {
if (data) {
setTodos(data)
}
}, [data])
return <div>{todos.map(todo => <Todo key={todo.id} {...todo} />)}</div>
}
// ✅ Correct: Let useQuery handle the state
function TodoList() {
const { data: todos } = useQuery({
queryKey: ['todos'],
queryFn: () => fetch('/api/todos').then(r => r.json())
})
return <div>{todos?.map(todo => <Todo key={todo.id} {...todo} />)}</div>
}
The defaults
React Query's defaults are genius, especially its stale-while-revalidate strategy. Here's how it works:
By default, your data is considered stale immediately. Wait, what...? How does it serve data instantly then...?
This is actually brilliant. When you request the same data again, React Query:
Shows you the stale data instantly (great UX!)
Fetches fresh data in the background
Updates the UI if something changed
This means your users see something immediately while still getting fresh data (could be seconds stale). Best of both worlds.
You can control this behavior through two key settings:
staleTime
: How long before data is considered stale, when should revalidation happen? (default: 0)gcTime
: How long before inactive data is garbage collected, when should the cache be cleared? (default: 5 minutes)
Quick example:
// Global configuration
const queryClient = new QueryClient({
defaultOptions: {
queries: {
staleTime: 1000 * 60, // 1 minute
gcTime: 1000 * 60 * 10, // 10 minutes
},
},
});
// Or per query
useQuery({
queryKey: ["todos"],
queryFn: fetchTodos,
staleTime: 1000 * 30, // 30 seconds
});
Query Keys
Fundamentals
We need to talk about query keys. They're in my opinion the magic sauce of React Query. If you grasp the whole architecture around observing data, you know why query keys are so important.
Here's a common mistake I see people “try” to do:
function TodoList({ filter }) {
const { data, refetch } = useQuery({
queryKey: ["todos"],
queryFn: () => fetchTodos(),
});
// ❌ Wrong! This won't work as expected
return (
<button onClick={() => refetch({ filter })}>Load {filter} todos</button>
);
}
The problem? refetch
doesn't accept arguments. That's not how React Query is designed to work. Refetch is meant for fetching the same data again. "revalidate" would've been a better name to be fair.
Here's the right way (include the query key):
function TodoList({ filter }) {
const { data } = useQuery({
queryKey: ["todos", filter], // 🎯 Key changes when filter changes!
queryFn: () => fetchTodos(filter),
});
}
Query keys are reactive. When a key changes, React Query knows it needs fresh data.
This is the mental model you need:
Query keys are like dependencies in useEffect
They define the unique identity of your data
When they change, you get a refetch
Some real-world examples:
// Search with URL state
const { search } = useSearchParams();
useQuery({
queryKey: ["search", search],
queryFn: () => searchItems(search),
});
// Complex filters
useQuery({
queryKey: ["todos", { status, priority, assignee }],
queryFn: () => fetchTodos({ status, priority, assignee }),
});
The beauty of this approach is that it's declarative. You don't manually trigger refetches, you just change the key, and React Query handles the rest. Your UI becomes a reflection of your query keys.
Pro tips for query keys
Structure your query keys like a path. Start broad, then get specific.
Use the query key factory pattern for type safety. This works fantastic on scale.
Here is an example from my own side project (stock market explorer):
export const stockDetailKeys = {
all: ['stock-detail'] as const,
bySymbol: (symbol: string) => [...stockDetailKeys.all, symbol] as const,
company: (symbol: string) =>
[...stockDetailKeys.bySymbol(symbol), 'company'] as const,
price: (symbol: string, timeframe: Timeframe) =>
[...stockDetailKeys.bySymbol(symbol), 'price', timeframe] as const,
chart: (symbol: string, timeframe: Timeframe) =>
[...stockDetailKeys.bySymbol(symbol), 'chart', timeframe] as const,
technicals: {
rsi: (symbol: string, timeframe: Timeframe) =>
[
...stockDetailKeys.bySymbol(symbol),
'technicals',
'rsi',
timeframe,
] as const,
macd: (symbol: string, timeframe: Timeframe) =>
[
...stockDetailKeys.bySymbol(symbol),
'technicals',
'macd',
timeframe,
] as const,
sma: (symbol: string, timeframe: Timeframe) =>
[
...stockDetailKeys.bySymbol(symbol),
'technicals',
'sma',
timeframe,
] as const,
},
}
One thing you'll notice is timeframe
. We can get data for different timeframes. A bad mental model would be to refetch the query with different timeframe, which we also saw doesn't work. Instead, include it in the query key.
Quick pseudo code to demonstrate:
const { timeframe } = useSearchParams();
useQuery({
queryKey: stockDetailKeys.price(symbol, timeframe),
queryFn: () => fetchStockDetail(symbol, timeframe),
});
Query Functions
There are two things I want to talk about here.
It's not about fetch
The first one is that queryFn
isn't about passing a fetch
. You can pass it any function as long as it returns a Promise. This is an important distinction. So get that out of your head that it's about passing the fetch
function. It's about functions returning Promises.
// ❌ This doesn't work
// You need to pass a function
useQuery({
queryKey: ["todos"],
queryFn: Promise.resolve({ todos: [] }),
});
// ✅ This works
useQuery({
queryKey: ["todos"],
queryFn: () => Promise.resolve({ todos: [] }),
});
// ✅ Common example
useQuery({
queryKey: ["todos"],
queryFn: () => fetch("/api/todos").then((r) => r.json()),
});
You decide the job queryFn should do
With that you of the way, I wanna talk about a tricky thing I encountered. This was because I always thought queryFn
was about resolving only getting one piece of data. In my stock market explorer (code link), I needed to get multiple pieces of data to represent a single UI state.
Initially, I tried using two useQuery hooks to get the data. The latter one depended on the first one because I needed to do the first fetch
in order to do the second fetch
. This was horrible and resulted in a deep rabbit hole.
After 3 hours of stuggling, I realized that the UI represented on the page was a single state. Why not just do it in a single queryFn
?
Oh boy. It turned out so much better.
It made me again realize that queryFn
only cares about you passing it a function that returns a promise. You are the one who decides how and what you need to do to get your data.
Use the dev tools
This may be obvious, but use the DevTools. They're your best friend. They're there for a reason. Don't neglect them. They help a ton with debugging.
Keeping track of queries
One thing I find myself doing is needing to know when a specific query is running to show something in loading tate. As we learned before, React Query keeps track of queries via their query keys.
Here is a practical example from the same stock market explorer app where I need to know when search is running:
const isFetchingSearchStocks =
useIsFetching({ queryKey: tickerKeys.filtered(stockFilters) }) > 0;
useIsFetching
returns the number of queries that are currently running for the given query key. In my case, I just needed to know if search was running at all.
As you can see, the query key factory pattern comes in handy when you need to keep track of multiple queries at scale.
React Query as a state manager
In my stock market explorer, I needed to keep track of the timeframe as a global state. The user can change the timeframe on a price chart by clicking the tabs.
Initially, I was thinking of using Context or pulling in Zustand. But React Query already is a state manager.
When dealing with a global state like this, some things are different:
I need an initial value, otherwise no tabs will be selected the first time.
We don't pass a
queryFn
touseQuery
since there is nothing to fetch.Because we don't fetch anything, data can't be stale, so we set
staleTime
toInfinity
(meaning it's never stale).
Here is the hook I created to getting the timeframe:
export function useTimeframe() {
const { data: timeframe } = useQuery({
queryKey: TIMEFRAME_KEY,
staleTime: Infinity,
initialData: '1D' as Timeframe,
})
return timeframe
}
How we get it:
const timeframe = useTimeframe();
How we update it using setQueryData
:
const queryClient = useQueryClient();
queryClient.setQueryData(TIMEFRAME_KEY, "1M");
Prefetching to 10x the experience
I see so many products every day that could 10x their user experience by prefetching data. This isn't a new concept nor anything tied to React Query.
Prefetching is all about fetching the data before the user needs it.
When do you fetch the data? When the user is likely to need it e.g. if they hover over a blog post, you can prefetch the data for the blog post. So when they navigate to the blog post, the data is already there. There is nothing to load. The experience is snappy.
Although, this depends on how your cache is configured. With React Query, anything within staleTime
will be served instantly when the data is requested. Of course, if your gcTime
is zero (which it shouldn’t be!), then prefetching will never work. Why? Because you’re storing data in the cache for a query that’s not actively being observed.
Here is an example:
function prefetchBlogPost(id) {
queryClient.prefetchQuery({
queryKey: blogPostKeys.byId(id),
queryFn: () => fetchBlogPost(id),
});
}
To keep your code clean, I always recommend extracting the queryFn
into a separate function. This way, you can reuse it in other parts of your app.
Questions my younger self had
"I need optimistic updates"
The documentation have an example of how to do this.
There is no magic here. Just update the data with setQueryData
. Example from docs:
const queryClient = useQueryClient();
useMutation({
mutationFn: updateTodo,
// When mutate is called:
onMutate: async (newTodo) => {
// Cancel any outgoing refetches
// (so they don't overwrite our optimistic update)
await queryClient.cancelQueries({ queryKey: ["todos"] });
// Snapshot the previous value
const previousTodos = queryClient.getQueryData(["todos"]);
// Optimistically update to the new value
queryClient.setQueryData(["todos"], (old) => [...old, newTodo]);
// Return a context object with the snapshotted value
return { previousTodos };
},
// If the mutation fails,
// use the context returned from onMutate to roll back
onError: (err, newTodo, context) => {
queryClient.setQueryData(["todos"], context.previousTodos);
},
// Always refetch after error or success:
onSettled: () => {
queryClient.invalidateQueries({ queryKey: ["todos"] });
},
});
"I need infinite scrolling"
This is also covered in the docs.
Use the useInfiniteQuery
hook. It works phenomenally.
One important thing to note here is that isLoading
is only true for the initial query. For subsequent queries, isFetching
will be true. React Query distinguishes between isLoading
for initial queries and isFetching
for subsequent ones. It's a common pattern you find among its hooks.
"I don't want my query to run when..."
The enabled
option is your friend here. It's a boolean that tells React Query whether it should run the query or not.
Here's an example, imagine you're building a feature where users can search for other users, but you don't want to hit the API until they've typed at least 2 characters:
function UserSearch() {
const [search, setSearch] = useState("");
const { data, isLoading } = useQuery({
queryKey: ["users", search],
queryFn: () => searchUsers(search),
// Only run when search is 2 or more characters
enabled: search.length >= 2,
});
return (
<div>
<input
value={search}
onChange={(e) => setSearch(e.target.value)}
placeholder="Search users..."
/>
{isLoading ? (
<div>Loading...</div>
) : (
data?.map((user) => <UserCard key={user.id} user={user} />)
)}
</div>
);
}
If you need to debounce the fetch here. You simply debounce the update to search
state. It’s a part of queryKey
. So when it changes, then React Query knows to fetch again.
Subscribe to my newsletter
Read articles from Tiger Abrodi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
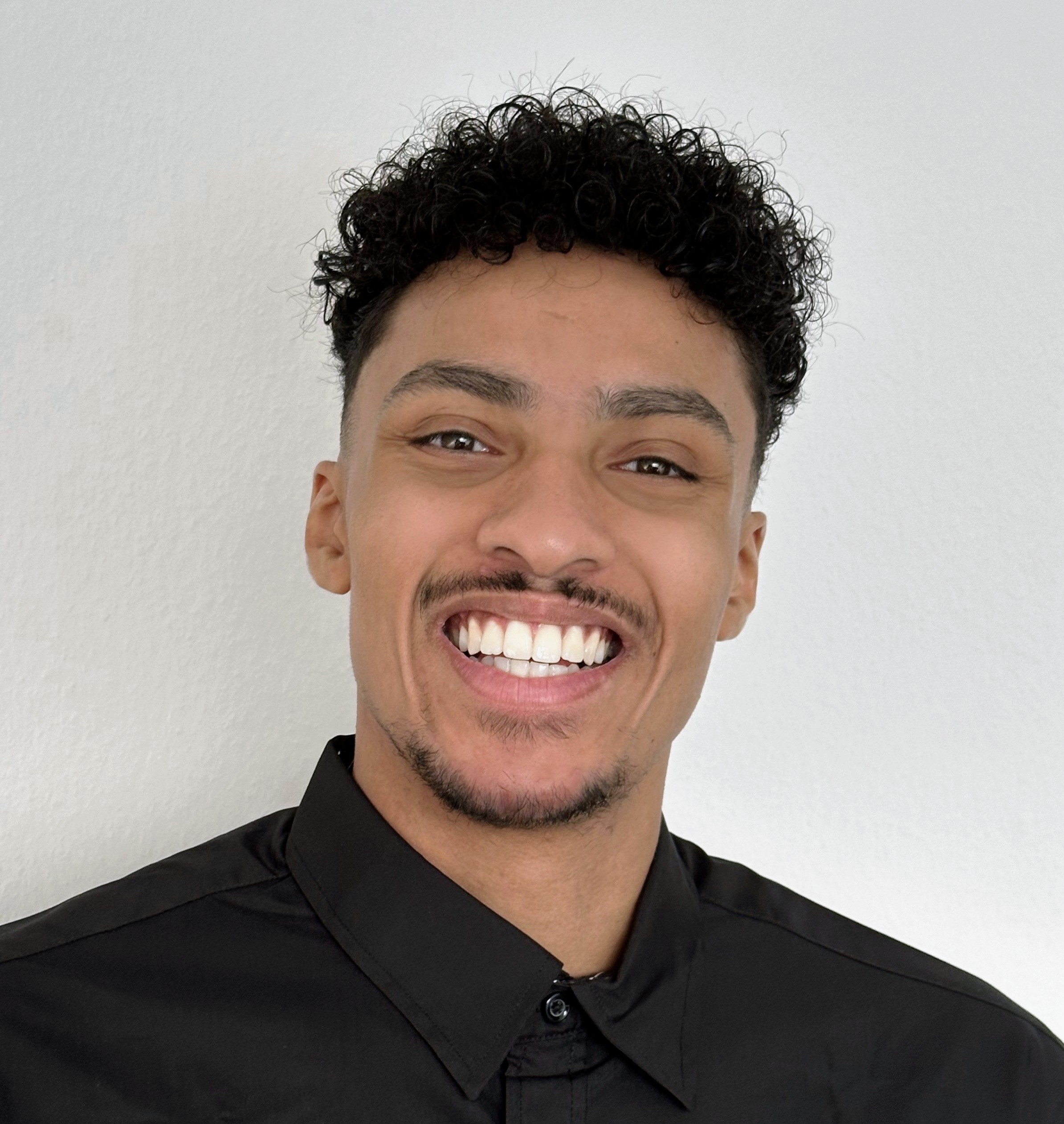
Tiger Abrodi
Tiger Abrodi
Just a guy who loves to write code and watch anime.