What's in a component

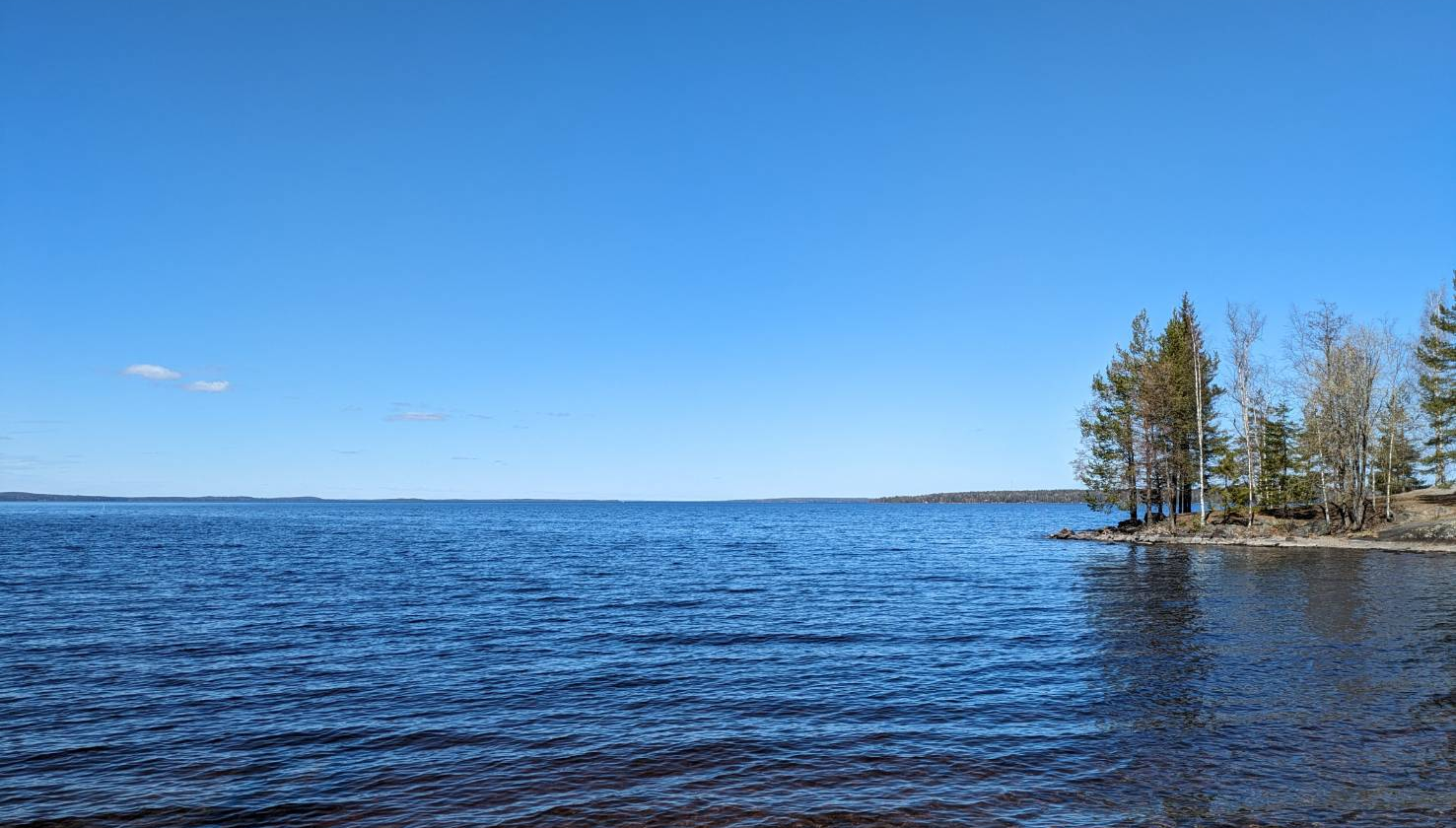
What’s in a component? That which implemented in any other way would fell as reusable
The complete source code displayed in this article can be found in Vrac’s repository.
In the last post “Making HTML reactive using Signaali“, we hooked up our code to HTML elements already existing in an HTML page. That’s not how modern web development work, nowadays we create them within our program and refer to them from there.
So, let’s represent HTML in our program. But wait .. which format should we choose? Hiccup is using Clojure vectors for both HTML and data, sometimes causing ambiguity for people reading the source code and security issues at runtime. UIx is using a syntax which is not ambiguous, so let’s reuse it!
We go from:
<main>
<h1>Developing Vrac from scratch - components</h1>
<p>This demonstrates how Vrac is implemented.</p>
<h2>Reactive counter</h2>
<div>
Counter value: <span id="counter-element">n/a</span>
<div>
<button id="inc-counter-button">Increment</button>
<button id="reset-counter-button">Reset</button>
</div>
</div>
<h2>Lazy effects</h2>
<div>
The effects are lazy, the user decides when to re-run them.
Click the button to update the DOM.
<div>
<button id="run-effects-button">Run effects</button>
</div>
</div>
</main>
and convert it to:
(defn my-html []
($ :main
($ :h1 "Developing Vrac from scratch - components")
($ :p "This demonstrates how Vrac is implemented.")
($ :h2 "Reactive counter")
($ :div "Counter value:" ($ :span#counter-element "n/a")
($ :div
($ :button#inc-counter-button "Increment")
($ :button#reset-counter-button "Reset")))
($ :h2 "Lazy effects")
($ :div "The effects are lazy, the user decides when to re-run them. Click the button to update the DOM."
($ :div
($ :button#run-effects-button "Run effects")))))
There are a few changes to do:
We want to stop referring to HTML elements by id and instead refer to them contextually or via Clojure symbols.
The
:span
element looks a bit useless now that we don’t use theid
, let’s remove it and directly refer to a text node instead.We want to have a Signaali state for the counter.
We want to avoid verbosity for the effect which updates a counter text node, so we want it to be implicit.
We want to add event listeners on the buttons.
With a bit of magic, it could look like:
(defn my-html []
($ :main
($ :h1 "Developing Vrac from scratch - components")
($ :p "This demonstrates how Vrac is implemented.")
($ :h2 "Reactive counter")
(let [counter-state (sr/create-state 0)]
($ :div "Counter value:" counter-state
($ :div
($ :button {:on-click #(swap! counter-state inc)} "Increment")
($ :button {:on-click #(reset! counter-state 0)} "Reset"))))
($ :h2 "Lazy effects")
($ :div "The effects are lazy, the user decides when to re-run them. Click the button to update the DOM."
($ :div
($ :button {:on-click #(sr/re-run-stale-effectful-nodes)} "Run effects")))))
The function my-html
starts to look like a real React component now. But what is a component alone? We should have more! How do we compose them? Our components are functions, so we compose them by calling those functions — naturally.
You can count on me like 1-2-3, I’ll be there …
Let’s say that we want more counters in our small experiment — 3 of them.
(defn my-counter [counter-state]
($ :div "Counter value:" counter-state
($ :div
($ :button {:on-click #(swap! counter-state inc)} "Increment")
($ :button {:on-click #(reset! counter-state 0)} "Reset"))))
(defn my-html [nb-counters] ;; nb-counters is 3
($ :main
($ :h1 "Giving shape to components")
($ :p "This demonstrates how Vrac is implemented.")
($ :h2 "Reactive counters")
(for [i (range nb-counters)]
(let [counter-state (sr/create-state (* i 100))]
(my-counter counter-state)))
($ :h2 "Lazy effects")
($ :div "The effects are lazy, the user decides when to re-run them. Click the button to update the DOM."
($ :div
($ :button {:on-click #(sr/re-run-stale-effectful-nodes)} "Run effects")))))
The $
function
The obvious first question is: what kind value should it return?
Well, we chose this function mainly to remove any ambiguity. But we want it to work similarly to Hiccup to represent an HTML structure which can be transformed later.
Because the ($ :div ,,,)
expressions look different from the user’s perspective and because it will contain a few new types of values, let’s give this structure a new name: “Vrac-Hiccup”, shortened as “Vcup”.
“Vcup” is made of either:
a VcupNode which is a node of children Vcup elements, or
a Vcup leaf value — text, reactive node, etc …
.. and because we use types and not Clojure data structures, there is always enough space for more types of node or leaf values.
(defrecord VcupNode [tag children])
(defn $ [tag & children]
(VcupNode. tag children))
Rendering the root component within the HTML page
With the page’s HTML being handled by the app, index.html
now looks like a standard SPA’s index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1" />
<title>Making HTML reactive using own HTML library</title>
</head>
<body>
<script src="/js/main.js" defer></script>
<div id="app"></div>
</body>
</html>
The entry point and reloading code of the app becomes:
(defn ^:dev/after-load setup! []
(render (js/document.getElementById "app")
(my-html 3)))
(defn ^:dev/before-load shutdown! []
(dispose-render-effects))
(defn start-app []
(setup!))
Starting from here in this article, all the functions belong to the Vrac library and not the example. Let’s dive in.
The render function:
processes the vcup returned by the root component, uses
process-vcup
to turn it into a DOM elements + some reactive effects,sets the DOM elements as children of the element defined in
index.html
,then runs the effects for the first time, enabling them to find their dependencies and react to them in the future.
(defn render [^js/Element parent-element vcup]
(let [{:keys [effects elements]} (process-vcup vcup)]
;; Set all the elements as children of parent-element
(.apply (.-replaceChildren parent-element) parent-element (to-array elements))
;; Run all the effects
(run! sr/run-if-needed effects)))
(defn dispose-render-effects []
;; TODO: dispose all the effects used for the rendering and the DOM updates.
;; In this article, we will skip this step.
,)
Implementing the process-vcup
function
We want to walk the vcup structure to transform it into a DOM structure, and while doing that we also want to keep track of the reactive effects which are used for updating the text nodes.
(defn- set-element-attribute [^js/Element element attribute-name attribute-value]
(cond
;; Event listener
(str/starts-with? attribute-name "on-")
(.addEventListener element
(-> attribute-name (subs (count "on-")))
attribute-value)
:else
:to-be-defined-in-next-articles))
(defn process-vcup [vcup]
(let [all-effects (atom [])
to-dom-elements (fn to-dom-elements [vcup]
(cond
(string? vcup)
[(js/document.createTextNode vcup)]
(instance? VcupNode vcup)
(let [^js/Element element (js/document.createElement (name (:element-tag vcup)))
[attributes children] (let [children (:children vcup)
x (first children)]
(if (and (map? x)
(not (record? x))) ; because map? returns true on records
[x (next children)]
[nil children]))
;; Convert the children into elements.
child-elements (into []
(comp (remove nil?)
;; Inline elements when child is a seq.
(mapcat (fn [child]
(if (seq? child)
child
[child])))
(mapcat to-dom-elements))
children)]
;; Set the attributes on the created element.
(doseq [[attribute-name attribute-value] attributes]
(set-element-attribute element (name attribute-name) attribute-value))
;; Set the element's children
(doseq [child-element child-elements]
(-> element (.appendChild child-element)))
[element])
(instance? sr/ReactiveNode vcup)
(let [element (js/document.createTextNode "")
effect (sr/create-effect (fn []
(set! (.-textContent element) @vcup)))]
(swap! all-effects conj effect)
[element])
:else
:to-be-defined-in-next-articles))
elements (to-dom-elements vcup)]
{:effects @all-effects
:elements elements}))
And that’s it! We now have the basics of a reactive web framework + a working example with only 111 lines of code in total, all thanks to Signaali’s support.
In the next article I will make the range of the for
loop dynamic — a change with a lot of technical implications. Stay tuned!
Subscribe to my newsletter
Read articles from Vincent Cantin (green-coder) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
