Elegant Trick to Swap Variables Without Extra Memory

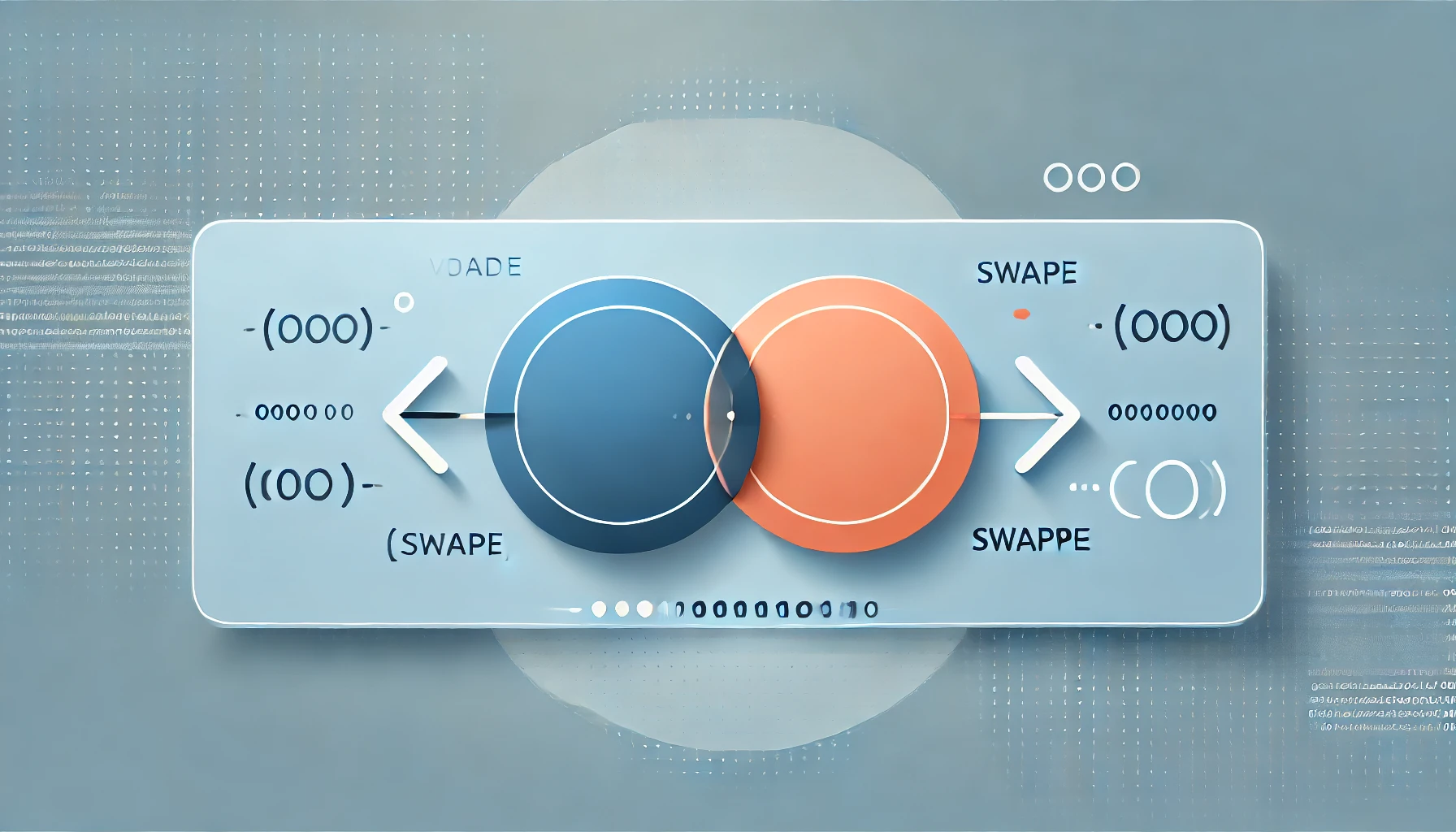
Imagine you are in C++ programming language exam session. You relaxed and answering the questions one after another. After a while you solved all the quests and now it’s time to solve the last one.
Question Title:
We have two variables like below:
int a = 10; int b = 12;
Swap variable’s content and print the result.
At first, you think, wow! what a simple question. Let’s get free scores. But suddenly you notice something at the bottom of the question:
(Note: you can not use a any third variable!)
Wait! What? Without any third variable? How it’s even possible?
Here is how:
You may know that in compiled language like C++ everything will be converted to binary (Zero and One).
So you can convert the variable’s content to binaries also. By doing that and replacing the content of the variables with the binary one and printing them to output, You’ll see something like this:
...
std::cout<<"A contains: "<<a<<endl;
str::cout<<"B contains: "<<b;
...
Output:
A contains: 1010
B contains: 1100
And looks normal.
Step 2:
Now it’s time to play the magic trick. If we apply the XOR operation three times in each digits column of the binaries content, we could see that the variables just swapped automatically!
Don’t you believe it? Let’s give it a try!
“XOR” an abbreviation for “Exclusively-OR.” The simplest XOR gate is a two-input digital circuit that outputs a logical “1” if the two input values differ, i.e., its output is a logical “1” if either of its inputs are 1, but not at the same time (exclusively).
The first column of digits are two number ones. If we apply XOR on them, it will be 0. right?
Now we’ll put the operation result in place of the first A’s digit and A looks like 0010
. Great. Then we need do the same process two more times and each time put the operation result in the opposite variable name (we put the result of XOR between A & B in A, So in the same way B & A in B. The order is important).
A: 1010 // Zero step.
B: 1100
A: 0010 // First step for the first colume of digits.
B: 1100 // Second step for the first colume of digits.
A: 1010 // Third step for the first colume of digits.
Step 3 (Final):
Great! now it’s enough to just do the same process for the 3 remaining columns of digits. (As done below)
Note that in the below codes, the process were applied at a same time for all columns to prevent boring calculations:
A: 1010 // Zero step. definition.
B: 1100
A: 0110 // First step
B: 1010 // Second step
A: 1100 // Last step. just look at the result!
Did you see that? At the end of the third step, the content of our two variables are completely swapped!
The strange thing is that the whole process will work for any binary values and there are no any exceptions!
You can try it by your self with different values and see that it works fine with any binary value.
One more note:
At the end, if you want to test on any other datatype apart from int datatype, It will work fine. BUT the important note is that you should convert your data into binary before you start the process, and after swapping the values convert them back to your considered datatype.
Conclusion:
In this article, we demonstrated a clever way to swap two variables without using a third variable in C++ by applying the XOR bitwise operation. This technique works by manipulating the binary representation of the variables through a series of XOR steps, resulting in the values being swapped.
Here, we used C++ language to explain our subject. But it works fine with all programming languages those you’re able to convert datatypes into binaries by them; Because as you know, binary isn’t depend on programming languages.
Subscribe to my newsletter
Read articles from Mostafa Motahari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mostafa Motahari
Mostafa Motahari
Back-End developer and Python-Django lover.