Django Signals: The Silent Heroes of Your Web App π¦ΈββοΈ
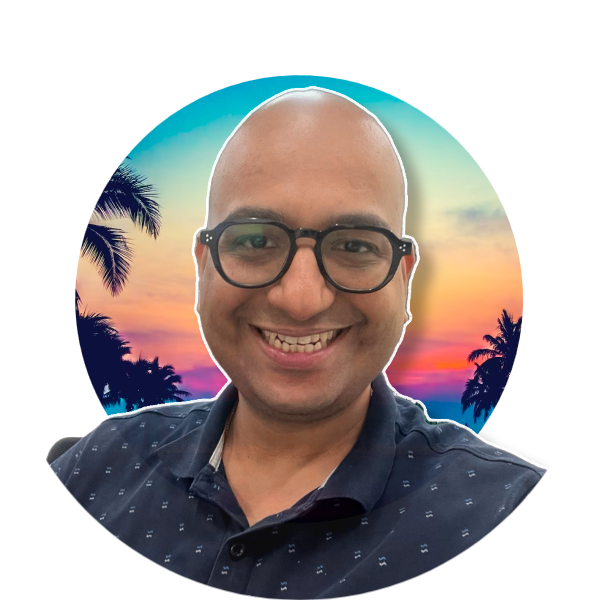

Have you ever wished your Django models could chat with each other like teenagers on social media? Well, they can! Django signals are like that friend who always knows the latest gossip and makes sure everyone stays in the loop. Let me share my journey of discovering these magical messengers.
I still remember the day I discovered Django signals. I was building a social media app where users needed profile pictures automatically created upon registration. My initial solution? A tangled mess of function calls scattered throughout the codebase like Christmas lights in January. Then, signals entered my life.
The "Aha!" Moment
from django.db.models.signals import post_save
from django.dispatch import receiver
from django.contrib.auth.models import User
from .models import Profile
@receiver(post_save, sender=User)
def create_user_profile(sender, instance, created, **kwargs):
if created:
Profile.objects.create(user=instance)
This little piece of code changed everything. It was like hiring a personal assistant who whispers "Hey, a new user just signed up, let me handle that profile creation for you." No more manual intervention needed!
The Signal Family
Think of Django signals like different types of notifications on your phone:
pre_save: The friend who tells you "Hey, you're about to post that embarrassing photo!"
@receiver(pre_save, sender=Article) def clean_up_title(sender, instance, **kwargs): instance.title = instance.title.strip().title()
post_save: The friend who announces "It's official!" after you update your relationship status
@receiver(post_save, sender=Post) def notify_followers(sender, instance, created, **kwargs): if created: instance.author.followers.notify(f"New post: {instance.title}")
pre_delete: The cautious friend asking "Are you sure you want to delete those vacation photos?"
post_delete: The cleanup crew after the party
The Plot Twist: When Signals Get Too Chatty
But here's the thing - like that friend who updates their status every five minutes, signals can sometimes be too eager to help. I learned this the hard way when my app's performance started resembling a snail in a marathon.
# Don't do this! π
@receiver(post_save, sender=User)
def do_everything(sender, instance, **kwargs):
instance.send_welcome_email()
instance.create_default_lists()
instance.analyze_profile()
instance.recommend_friends()
# ... and the kitchen sink
The Art of Signal Wisdom
After some face-palm moments, I developed my "Signal Sanity Checklist":
Is this signal really necessary? Like deciding whether to text your ex, think twice.
# Instead of a signal, sometimes a simple model method is better class User(models.Model): def save(self, *args, **kwargs): if self._state.adding: self.set_default_preferences() super().save(*args, **kwargs)
Keep it light - Signals should be more like Twitter and less like a dissertation.
Register responsibly - Put your signals in a dedicated
signals.py
file:# apps.py class MyAppConfig(AppConfig): name = 'myapp' def ready(self): import myapp.signals # Stay organized!
The Happy Ending
Once you understand signals, they're like having a well-trained orchestra in your Django app. Each instrument (model) knows exactly when to play its part, creating beautiful music (functionality) without the conductor (you) having to micromanage every note.
Pro Tips From the Trenches
Debug with style:
@receiver(post_save, sender=User) def log_user_changes(sender, instance, **kwargs): logger.info(f"π User {instance.username} updated") # Because logs should spark joy
Keep it async-friendly - Your signals should play nice with Django's async capabilities.
Test your signals - They're part of your app's functionality, not just decorative tinsel.
Wrapping Up
Django signals are like the background characters in a movie - you might not notice them when they're doing their job well, but the story wouldn't flow without them. They've saved me countless hours of writing boilerplate code and helped keep my applications maintainable and modular.
Remember, with great power comes great responsibility. Use signals wisely, and they'll be your app's silent heroes. Abuse them, and they'll become your app's hidden villains.
What's your experience with Django signals? Have they saved your day or caused unexpected troubles? Share your stories in the comments below!
About the Author: A Django enthusiast who learned about signals the hard way so you don't have to. Currently building awesome web apps and occasionally writing about it.
Image Attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
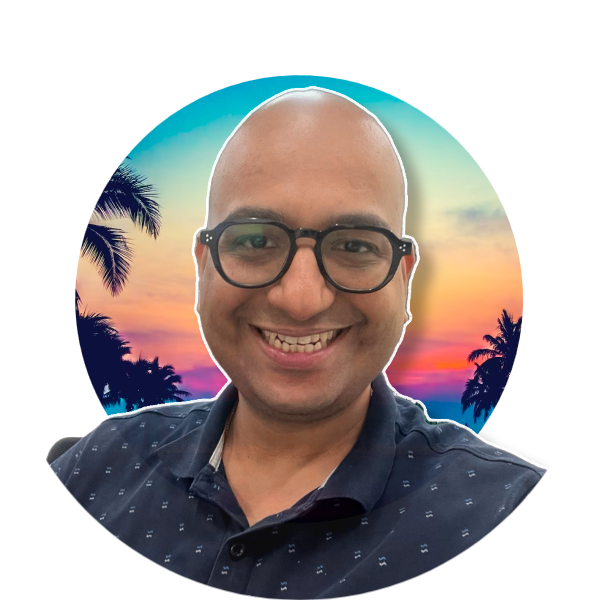
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.