Battle of the Architectures: Dynamic UI with RTK Query vs. Next.js Simplicity
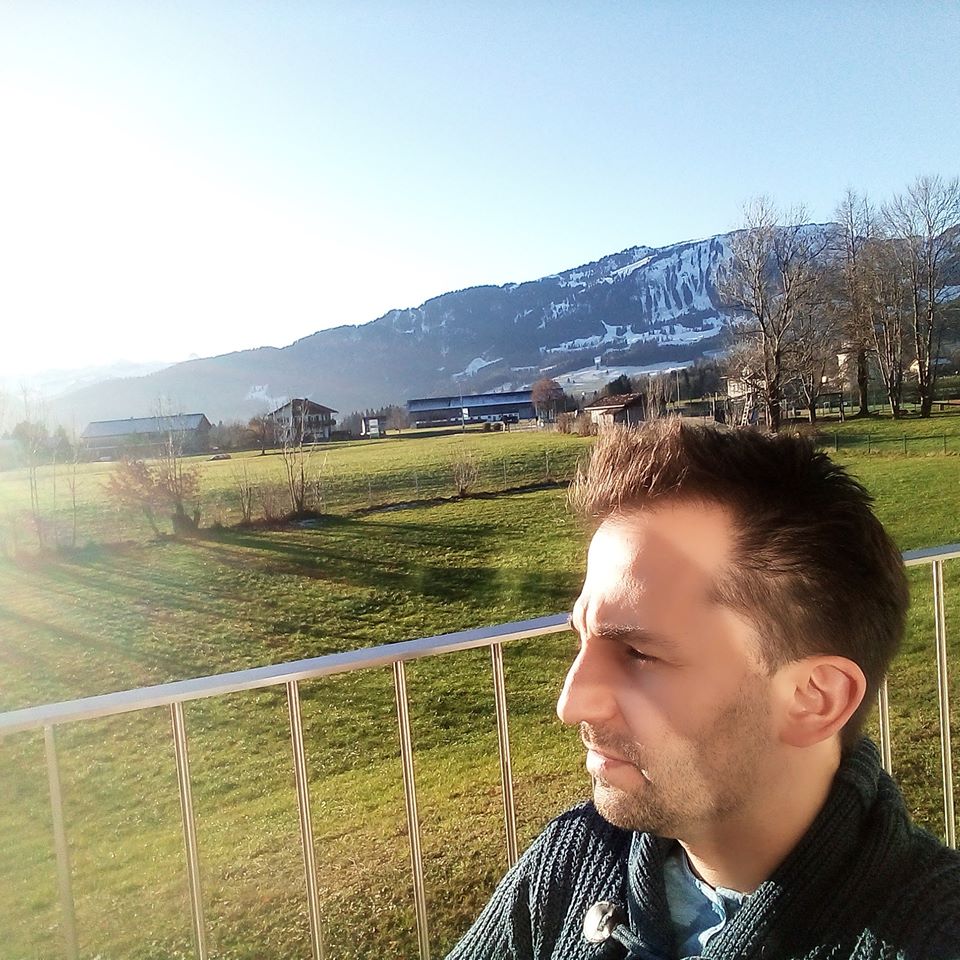

1. Introduction
When building a modern web application, developers are spoiled for choice with tools and frameworks. On one side, we have RTK Query and React Router, providing flexibility and control over data fetching and routing. On the other, Next.js, a full-stack framework, offers out-of-the-box solutions for routing, server-side rendering (SSR), and static site generation (SSG). In this article, we’ll compare these two architectures and help you decide which one suits your project best.
TldR Table Comparison:
Feature | RTK Query + React Router | Next.js |
Routing | Manual with React Router | File-based routing |
Data Fetching | RTK Query for client-side fetching | Integrated SSG, SSR, and CSR |
Rendering | CSR (Client-Side Rendering) | CSR, SSR, SSG, ISR |
SEO Optimization | Requires tools like React Helmet or SSR setup | Built-in SEO-friendly rendering |
Setup Complexity | High (manual integration) | Low (built-in features) |
In the ever-evolving world of web development, developers have a plethora of tools and frameworks to choose from when building modern applications. Two popular approaches stand out when working with React:
RTK Query + React Router: A combination of the Redux Toolkit's RTK Query for efficient data fetching and React Router for client-side routing. This architecture provides flexibility and control, making it ideal for applications where customization is key. However, it requires manual integration for features like SEO optimization or server-side rendering.
Next.js: A React-based full-stack framework that simplifies development with features like file-based routing, server-side rendering (SSR), static site generation (SSG), and incremental static regeneration (ISR). It's a go-to solution for applications requiring high performance, built-in SEO optimizations, and ease of use.
In this article, we’ll compare these two architectures, exploring their strengths, weaknesses, and when to use each. To ground the discussion, we'll use a blog application as an example.
RTK Query + React Router: A Dynamic Approach
When using RTK Query and React Router, you manage routing and data fetching independently. Here's how you might implement a blog post page:
// BlogPost.js
import { useParams } from 'react-router-dom';
import { useGetBlogPostQuery } from './services/blogApi';
function BlogPost() {
const { id } = useParams();
const { data: post, isLoading, error } = useGetBlogPostQuery(id);
if (isLoading) return <p>Loading...</p>;
if (error) return <p>Error loading blog post.</p>;
return (
<article>
<h1>{post.title}</h1>
<p>{post.content}</p>
</article>
);
}
export default BlogPost;
To make this work:
- React Router handles routing:
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import BlogPost from './BlogPost';
function App() {
return (
<Router>
<Routes>
<Route path="/blog/:id" element={<BlogPost />} />
</Routes>
</Router>
);
}
export default App;
RTK Query fetches data dynamically:
import { createApi, fetchBaseQuery } from '@reduxjs/toolkit/query/react';
export const blogApi = createApi({
reducerPath: 'blogApi',
baseQuery: fetchBaseQuery({ baseUrl: '/api' }),
endpoints: (builder) => ({
getBlogPost: builder.query({
query: (id) => `/posts/${id}`,
}),
}),
});
export const { useGetBlogPostQuery } = blogApi;
This approach allows for high flexibility but requires additional configuration to optimize SEO, performance, and server-side rendering.
Next.js: A Simplified, Full-Stack Framework
Next.js streamlines the creation of dynamic pages with its file-based routing and built-in data-fetching methods. Here’s how the same blog post page might look:
// pages/blog/[id].js
export async function getStaticPaths() {
const res = await fetch('https://api.example.com/posts');
const posts = await res.json();
const paths = posts.map((post) => ({
params: { id: post.id.toString() },
}));
return { paths, fallback: false };
}
export async function getStaticProps({ params }) {
const res = await fetch(`https://api.example.com/posts/${params.id}`);
const post = await res.json();
return {
props: { post },
};
}
export default function BlogPost({ post }) {
return (
<article>
<h1>{post.title}</h1>
<p>{post.content}</p>
</article>
);
}
In this case:
Routing is handled automatically based on the file structure (
pages/blog/[id].js
).Data fetching is managed through
getStaticProps
andgetStaticPaths
, enabling static generation and prefetching for better SEO and performance.
Extended Comparison Table
Feature | RTK Query + React Router | Next.js |
Routing | Manual setup with React Router. Example: <Route path="/blog/:id" element={<BlogPost />} /> . | File-based routing. Example: pages/blog/[id].js . |
Data Fetching | Client-side fetching with RTK Query, triggered when the page is loaded. | Built-in options for SSG (getStaticProps ), SSR (getServerSideProps ), or CSR. |
Rendering | Client-Side Rendering (CSR) by default. Requires additional setup for SSR with tools like Express. | Supports Static Site Generation (SSG), Server-Side Rendering (SSR), and hybrid rendering. |
SEO | Needs tools like React Helmet or SSR for better SEO. | Native SEO optimizations with server-side rendering and static generation. |
Performance | Requires manual lazy loading and optimization for images and assets. | Built-in optimizations like automatic image optimization and page prefetching. |
API Integration | Requires a separate backend for API endpoints (e.g., Express, Koa). | API routes built into the framework under pages/api/ . |
Ease of Use | More configuration and boilerplate code for routing, data fetching, and SSR setup. | Simplified setup with ready-to-use features. |
Flexibility | Highly flexible. You can use only the libraries and features you need. | Opinionated and less flexible, but includes everything needed for a full-stack application. |
Comparison in Context
With RTK Query + React Router, you have full control over the architecture but must manually address concerns like server-side rendering and SEO.
With Next.js, these features are built-in, making it easier to create a high-performance, SEO-friendly blog application with less manual effort.
Real-World Scenarios
When to Use RTK Query + React Router
Highly Customized Applications:
- If your application requires unique routing logic or a custom data-fetching strategy, this architecture gives you full control.
Single Page Applications (SPAs):
- Ideal for internal tools, dashboards, or applications where SEO is not a priority.
Advanced Data Handling:
- Perfect for apps where complex client-side state management is needed, leveraging RTK Query for efficient data fetching and caching.
Example Scenario: A real-time analytics dashboard for an e-commerce platform where SEO is irrelevant but dynamic state management and routing customization are crucial.
<Route path="/analytics" element={<AnalyticsDashboard />} />
const { data: salesData } = useGetSalesDataQuery();
When to Use Next.js
Public-Facing Websites:
- If your application needs to be SEO-friendly and fast-loading for users worldwide.
Content-Driven Applications:
- Ideal for blogs, e-commerce sites, and marketing pages where SEO and performance are key.
Full-Stack Projects:
- Suitable when you want to combine front-end and back-end in a single project, using API routes for backend logic.
Example Scenario: A multi-author blog platform that needs to pre-render posts for SEO and load quickly with static pages.
// pages/blog/[id].js
export async function getStaticProps({ params }) {
const post = await fetch(`https://api.example.com/posts/${params.id}`).then((res) => res.json());
return { props: { post } };
}
Conclusion: Choosing the right architecture depends on your project needs. For maximum flexibility and fine-grained control, RTK Query + React Router is a great choice. If you’re looking for an all-in-one solution with modern performance and SEO optimization, Next.js stands out.
RTK Query + React Router:
Use this architecture when you need full customization and are building dynamic, client-centric applications.
Be prepared for additional setup to handle SEO and performance optimizations.
Next.js:
Ideal for developers seeking a streamlined solution with built-in features for SEO, performance, and server-side capabilities.
A great choice for projects where time-to-market and simplicity are priorities.
Conclusion
Choosing the right architecture ultimately depends on the specific needs and goals of your project. Both RTK Query + React Router and Next.js offer distinct advantages, but they cater to different use cases.
RTK Query + React Router is ideal for projects where flexibility and control are paramount. This architecture allows developers to fine-tune every aspect of the application, from routing logic to data fetching and state management. It shines in scenarios where customization is a priority, such as building highly interactive dashboards, SPAs, or applications that require complex client-side state handling. However, it does require more effort to integrate features like server-side rendering or SEO optimizations, making it better suited for internal tools or apps where these features are not critical.
On the other hand, Next.js is a robust, all-in-one solution designed for modern web development. It simplifies the development process with built-in features like file-based routing, server-side rendering (SSR), static site generation (SSG), and image optimization. These features make it a perfect choice for public-facing applications where performance, SEO, and time-to-market are critical. From blogs and e-commerce sites to marketing pages and multi-author platforms, Next.js provides an excellent foundation for building scalable and performant web applications with minimal configuration.
When deciding between the two, it’s essential to consider your project requirements:
Do you need maximum control over the architecture, or is a ready-to-use framework more appealing?
Are SEO and performance optimizations a priority, or are you focused on creating a dynamic and interactive client-side experience?
By aligning the choice of architecture with your project’s priorities and technical needs, you can ensure a smoother development process and a better end result.
Subscribe to my newsletter
Read articles from Carmine Tambascia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
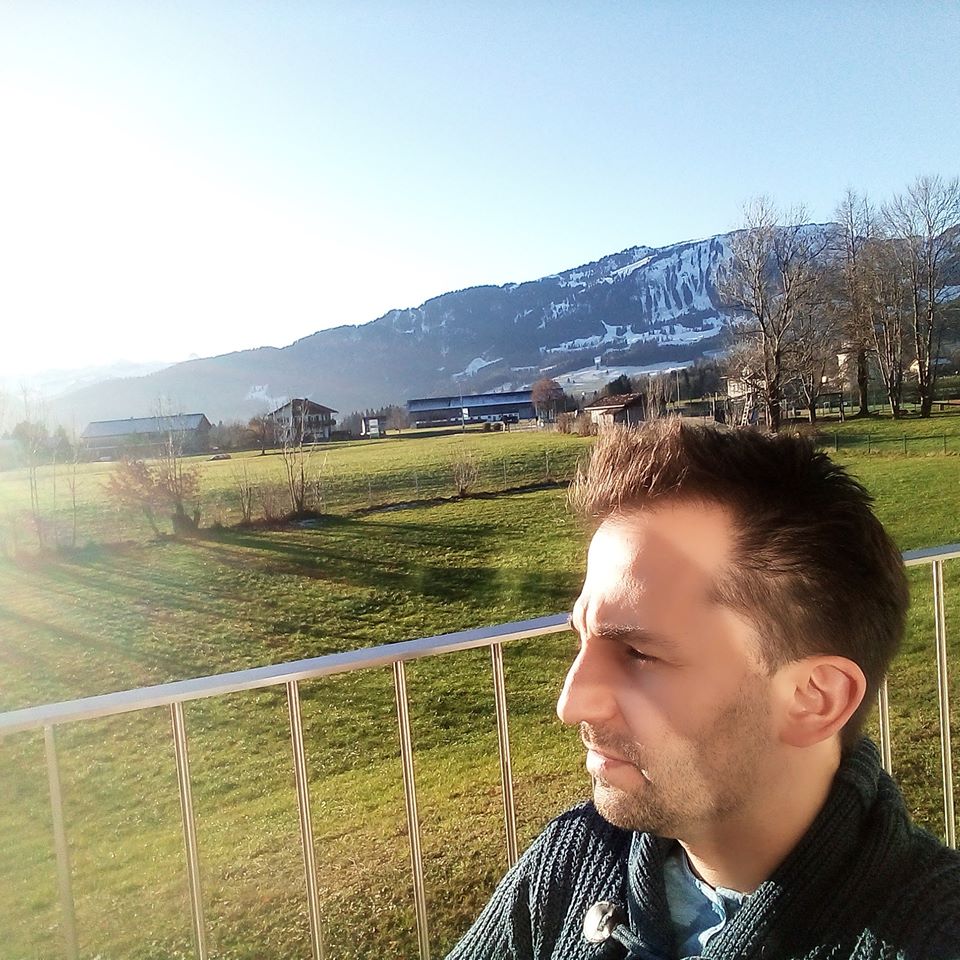