Understanding the Limitations of Vector Embeddings in RAG Systems
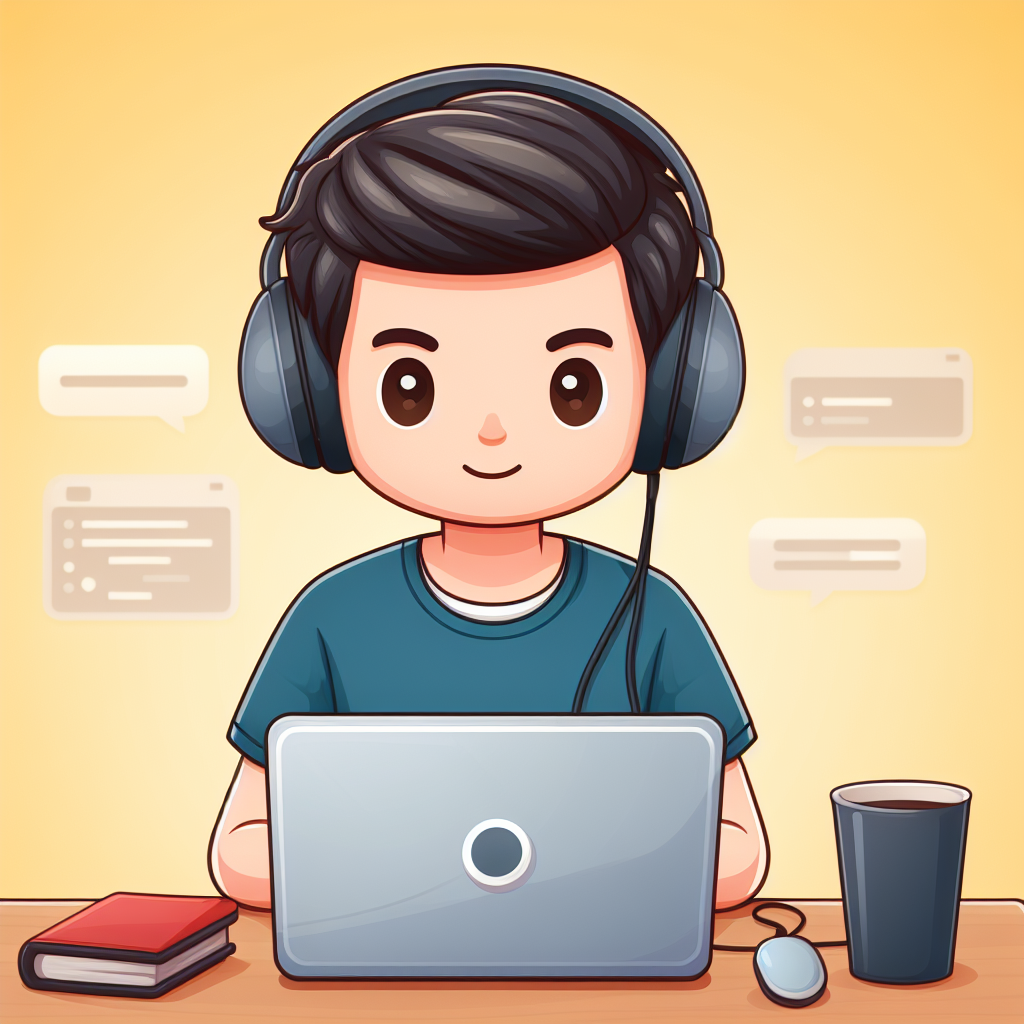
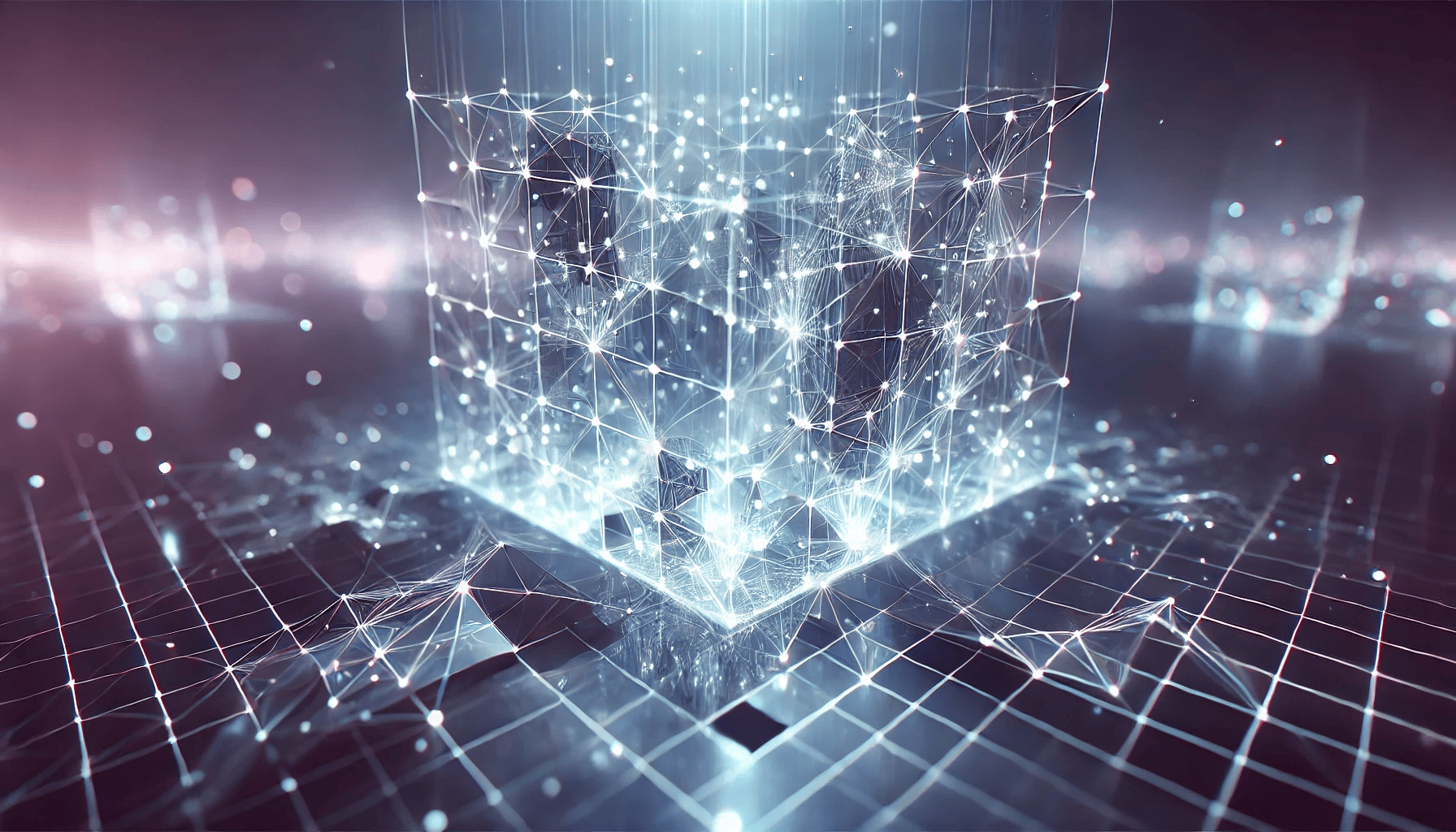
As AI solutions become more prevalent, Retrieval-Augmented Generation (RAG) has emerged as a popular approach for enhancing Large Language Models (LLMs) with external knowledge. However, there's a fundamental issue that often goes unaddressed: the reliability of vector embeddings for semantic similarity matching.
The Core Problem: Semantic Similarity vs. True Relevance
Let's examine why vector embeddings might not be the ideal tool for determining contextual relevance. Consider this practical example:
from openai import OpenAI
client = OpenAI()
# Example comparisons using embeddings
terms = {
"base": "smartphone",
"similar_but_irrelevant": "telephone",
"different_but_relevant": "mobile device"
}
# Getting similarity scores
embeddings = {term: client.embeddings.create(
input=word,
model="text-embedding-ada-002"
).data[0].embedding for term, word in terms.items()}
# Comparing similarity scores
smartphone_telephone = 0.91 # High similarity, low relevance
smartphone_mobile_device = 0.85 # Lower similarity, high relevance
In this case, "telephone" shows higher semantic similarity to "smartphone" than "mobile device" does, despite "mobile device" being more contextually relevant for most modern queries.
The Impact on Real-World Applications
This limitation affects various types of queries:
Entity Queries: When searching for information about "JavaScript", chunks about "Java" might be prioritized over those about "ECMAScript", despite the latter being more relevant.
Temporal Queries: A search for "2020s technology trends" might prioritize chunks about "2010s technology" over "contemporary tech developments".
Technical Documentation: Questions about "React hooks" might return information about "Vue composables" before "React custom hooks" due to semantic similarity patterns.
Production Challenges: The Reality Check
Recent research from leading tech companies demonstrates these limitations. In a comprehensive study:
Base RAG accuracy: 47%
With reranking using fine-tuned Model: 54%
With context expansion (48K characters): 62%
# Typical production RAG implementation
class EnhancedRAG:
def retrieve(self, query, k=3):
base_results = self.vector_search(query)
reranked = self.rerank_results(base_results)
return self.llm_generate(query, reranked) # Long Context LLM
A More Balanced Approach
Instead of relying solely on vector embeddings, consider a hybrid approach:
Lexical Search: Use traditional search methods for exact matches
Semantic Filtering: Apply vector embeddings to refine results
Context Validation: Implement domain-specific validation rules
class HybridSearchSystem:
def search(self, query):
# Initial lexical search
exact_matches = self.lexical_search(query)
# Semantic refinement
if len(exact_matches) > threshold:
return self.apply_semantic_filter(exact_matches, query)
# Fall back to vector search
return self.vector_search(query)
Looking Forward
While vector embeddings remain valuable tools in our AI toolkit, they shouldn't be treated as a complete solution for RAG systems. The future lies in combining traditional NLP techniques with modern embedding approaches, creating more reliable and contextually aware systems. Remember: The goal isn't to abandon vector embeddings but to understand their limitations and use them appropriately within a broader solution architecture.
Subscribe to my newsletter
Read articles from Pratik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
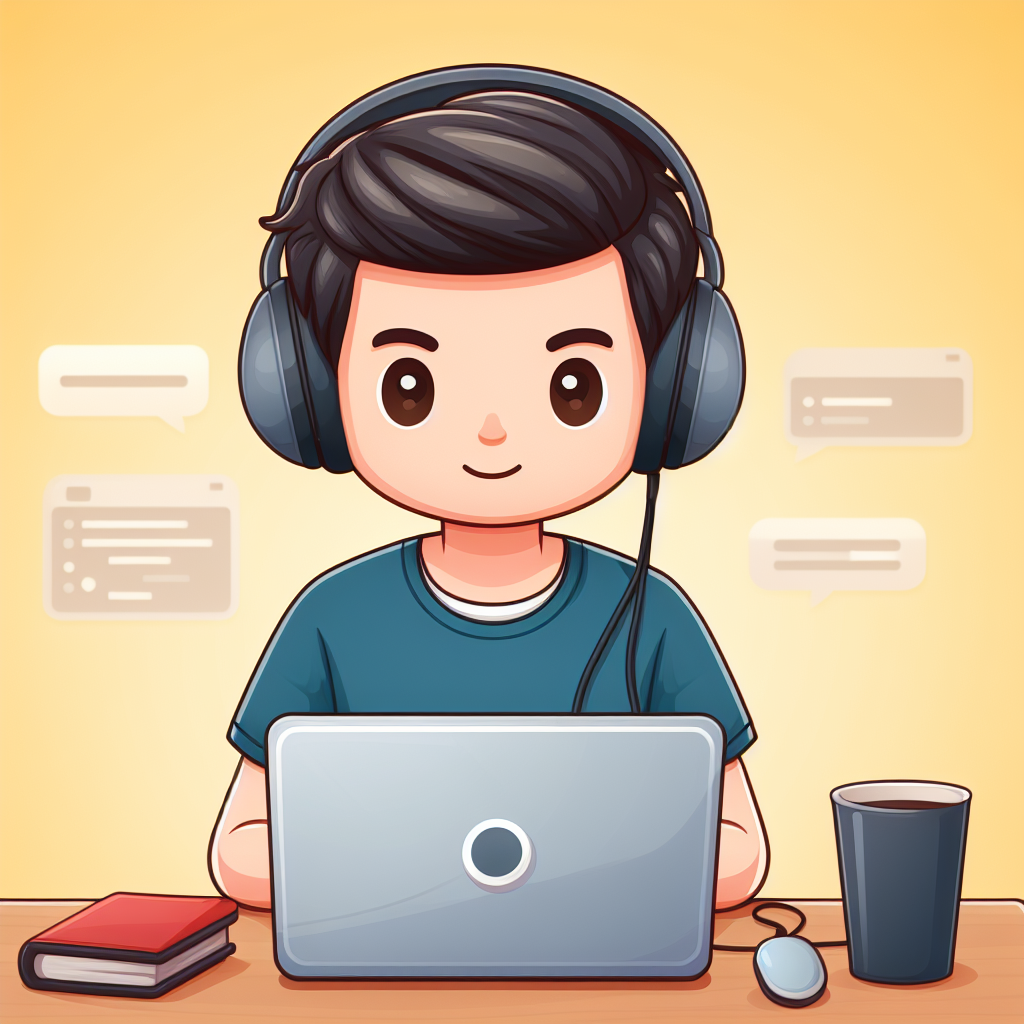
Pratik
Pratik
Hey there ๐๐ป! As a Software Developer and Entrepreneur, I'm passionate about leveraging technology to make a real impact on people's lives. I run a one-person dev agency, I'm your go-to person for turning your tech dreams into reality. Whether you have a project in mind or just want to chat tech, feel free to reach out to me via email or twitter. Let's build something amazing together!