Laravel 11 Request-Response System: A Simplified Guide
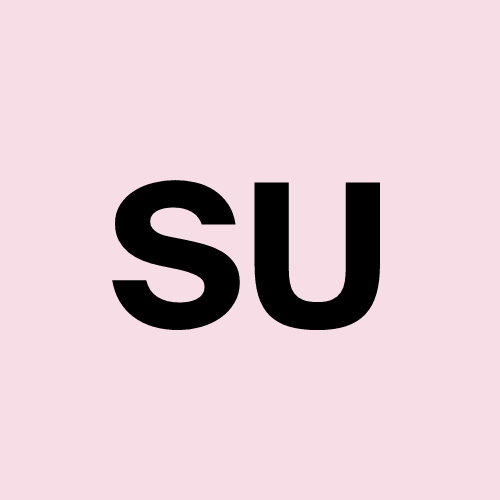

Laravel simplifies web development with its request-response system. Here’s a simple guide to how it works in Laravel 11.
How Laravel 11 Handles Requests
1. Entry Point
User requests go to public/index.php
, the starting point for all processing.
2. Middleware
Middleware filters requests:
Examples: Authentication, maintenance checks.
Purpose: Validate or modify requests, or return early responses.
3. Routes
Laravel matches requests to routes defined in routes/web.php
or routes/api.php
:
Route::get('/welcome', fn() => 'Welcome to Laravel!');
If no route matches, a 404 error is returned.
4. Controllers
Controllers handle logic:
class WelcomeController extends Controller
{
public function index()
{
return view('welcome');
}
}
5. Responses
Responses can include:
Views: Rendered with Blade templates.
JSON: Ideal for APIs.
Redirects: Navigate users to other routes.
Example:
return response('Hello!', 200)->header('Content-Type', 'text/plain');
Key Features
Request Access
Get user input easily:
$request->input('name');
$request->query('page');
Flexible Responses
Create custom responses:
response('Hello!')->header('X-Custom', 'Value');
return response()->download('file.pdf');
Custom Middleware
Add specific request filters, like logging or access control.
Simple Example
Route
Route::post('/submit', [FormController::class, 'submit']);
Controller
class FormController extends Controller
{
public function submit(Request $request)
{
$data = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|email',
]);
return response()->json(['message' => 'Form submitted!']);
}
}
Why It Matters
Efficiency: Handle requests smoothly.
Scalability: Grow your app with ease.
User-Friendly: Deliver better experiences.
Conclusion
Laravel 11’s request-response system makes development simple and efficient. Master it to build powerful, scalable applications effortlessly.
Subscribe to my newsletter
Read articles from Sharif uddin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
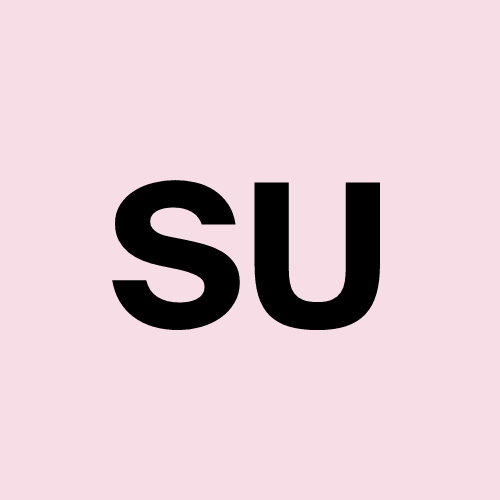