Essential JavaScript Built-In Functions for Problem Solving
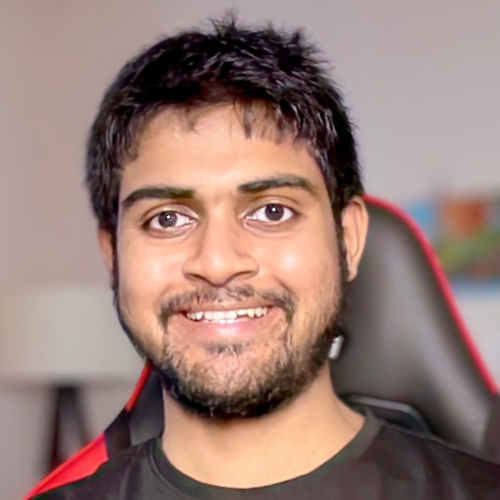
Table of contents
- 1. Array.prototype.map()
- 2. Array.prototype.filter()
- 3. Array.prototype.reduce()
- 4. Array.prototype.sort()
- 5. String.prototype.split() and Array.prototype.join()
- 6. Math.max() and Math.min()
- 7. Array.prototype.splice()
- 8. Set and Array.from()
- 9. JSON.stringify() and JSON.parse()
- 10. Array.prototype.includes()
- 11. Math.random()
- 12. Array.prototype.every() and Array.prototype.some()
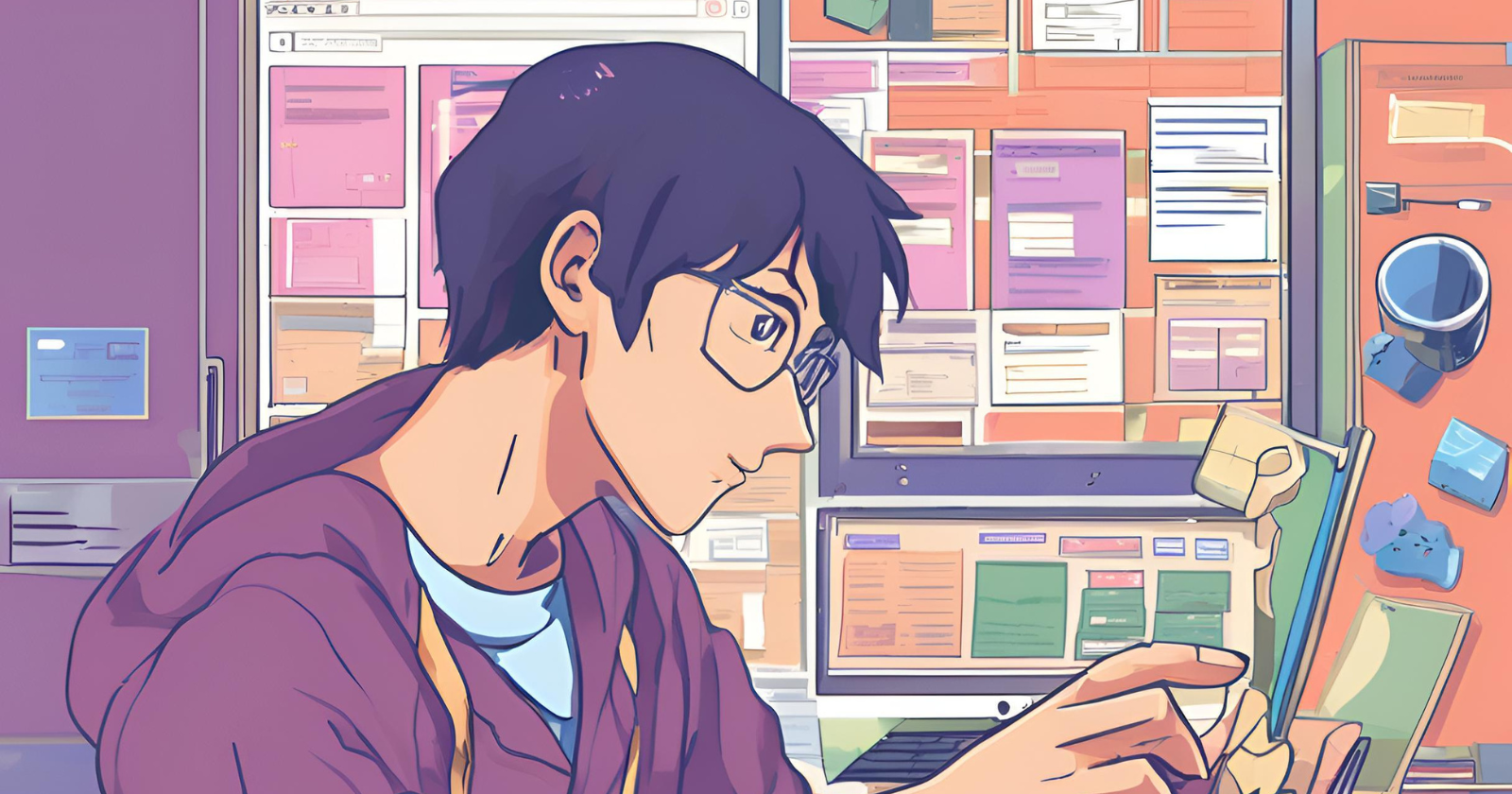
JavaScript comes with a treasure trove of built-in functions that make solving algorithmic and coding challenges a lot easier. While some of these functions might not appear frequently in day-to-day development, they can be game-changers when tackling coding problems. In this tutorial, we’ll explore some essential JavaScript built-in functions that every problem solver should know, along with their use cases and examples.
1. Array.prototype.map()
Description
The map()
function creates a new array by applying a provided function to each element of the original array. It is frequently used for transformations.
Use Case in Problem Solving
Transforming an array of numbers (e.g., squaring elements, converting units).
Mapping data to a desired structure.
Example
const numbers = [1, 2, 3, 4, 5];
// Square each number
const squares = numbers.map(num => num * num);
console.log(squares); // [1, 4, 9, 16, 25]
2. Array.prototype.filter()
Description
The filter()
function creates a new array containing only the elements that satisfy the condition specified by the callback function.
Use Case in Problem Solving
- Filtering data based on specific criteria (e.g., primes, even numbers).
Example
const numbers = [1, 2, 3, 4, 5];
// Get even numbers
const evens = numbers.filter(num => num % 2 === 0);
console.log(evens); // [2, 4]
3. Array.prototype.reduce()
Description
The reduce()
function applies a reducer function to each element of the array, resulting in a single output value.
Use Case in Problem Solving
- Calculating sums, products, or finding a single value based on an array (e.g., finding the maximum or minimum).
Example
const numbers = [1, 2, 3, 4, 5];
// Calculate sum
const sum = numbers.reduce((acc, num) => acc + num, 0);
console.log(sum); // 15
4. Array.prototype.sort()
Description
The sort()
function sorts the elements of an array in place and returns the sorted array.
Use Case in Problem Solving
- Sorting arrays for problems involving comparisons (e.g., finding the kth smallest/largest element).
Example
const numbers = [5, 1, 4, 2, 3];
// Sort in ascending order
numbers.sort((a, b) => a - b);
console.log(numbers); // [1, 2, 3, 4, 5]
5. String.prototype.split() and Array.prototype.join()
Description
split()
divides a string into an array based on a specified delimiter.join()
combines elements of an array into a single string with a specified separator.
Use Case in Problem Solving
- Reversing strings, manipulating text.
Example
const str = "hello world";
// Reverse the string
const reversed = str.split('').reverse().join('');
console.log(reversed); // "dlrow olleh"
6. Math.max() and Math.min()
Description
Math.max()
returns the largest number from a list of numbers.Math.min()
returns the smallest number from a list of numbers.
Use Case in Problem Solving
- Quickly finding the largest or smallest number in an array.
Example
const numbers = [1, 2, 3, 4, 5];
const max = Math.max(...numbers);
const min = Math.min(...numbers);
console.log(max); // 5
console.log(min); // 1
7. Array.prototype.splice()
Description
The splice()
function can add, remove, or replace elements in an array. It modifies the original array.
Use Case in Problem Solving
- Removing or inserting elements at specific positions.
Example
let arr = [1, 2, 3, 4];
// Remove 2 elements starting from index 1
arr.splice(1, 2);
console.log(arr); // [1, 4]
8. Set and Array.from()
Description
A
Set
is a collection of unique values.Array.from()
converts aSet
(or other iterable) back into an array.
Use Case in Problem Solving
- Removing duplicates from an array.
Example
const numbers = [1, 2, 2, 3, 4, 4, 5];
// Remove duplicates
const uniqueNumbers = Array.from(new Set(numbers));
console.log(uniqueNumbers); // [1, 2, 3, 4, 5]
9. JSON.stringify() and JSON.parse()
Description
JSON.stringify()
converts an object into a JSON string.JSON.parse()
converts a JSON string back into an object.
Use Case in Problem Solving
- Deep cloning objects or comparing nested structures.
Example
const obj = { a: 1, b: 2 };
// Deep clone
const clone = JSON.parse(JSON.stringify(obj));
console.log(clone); // { a: 1, b: 2 }
10. Array.prototype.includes()
Description
The includes()
function checks if an array contains a specific element.
Use Case in Problem Solving
- Checking for membership in an array.
Example
const numbers = [1, 2, 3, 4, 5];
console.log(numbers.includes(3)); // true
console.log(numbers.includes(6)); // false
11. Math.random()
Description
The Math.random()
function generates a random number between 0 (inclusive) and 1 (exclusive).
Use Case in Problem Solving
- Generating random numbers for simulations or random sampling.
Example
const randomNum = Math.floor(Math.random() * 100) + 1;
console.log(randomNum); // Random number between 1 and 100
12. Array.prototype.every() and Array.prototype.some()
Description
every()
checks if all elements in an array satisfy a condition.some()
checks if at least one element satisfies a condition.
Use Case in Problem Solving
- Validating conditions on arrays.
Example
const numbers = [2, 4, 6, 8];
console.log(numbers.every(num => num % 2 === 0)); // true
console.log(numbers.some(num => num > 5)); // true
Conclusion
These JavaScript built-in functions are powerful tools for problem solving. While some might seem niche in everyday coding, they shine during algorithm challenges. Mastering these functions will improve your efficiency and confidence in tackling complex problems.
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
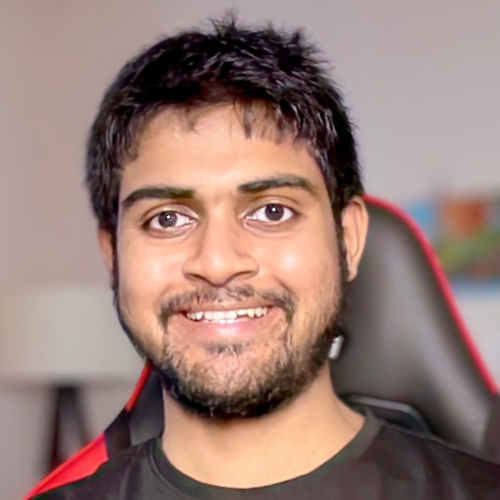
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.