Boost Your Golang Projects with Advanced Debugging Tools and Tips
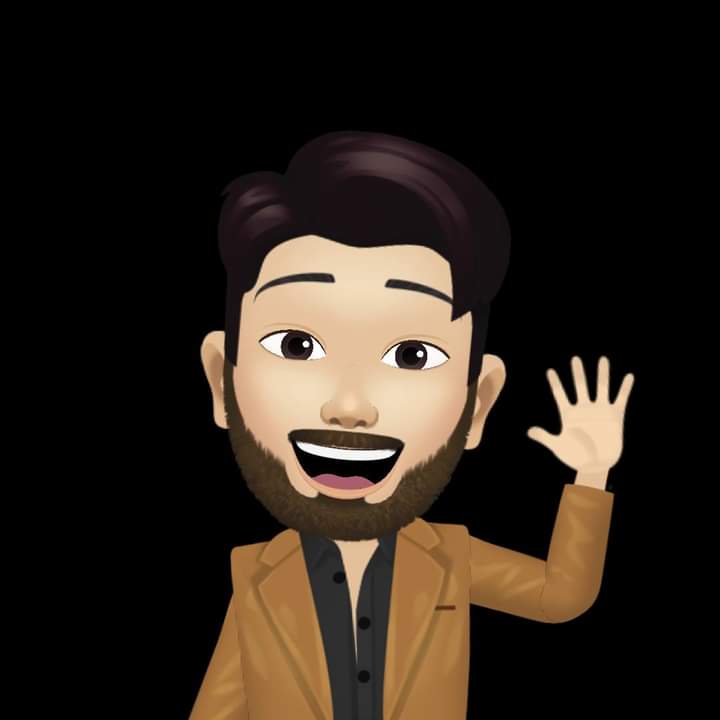

Debugging is a critical skill for any developer, and mastering advanced debugging techniques is especially important when working with complex systems. In Golang, a statically-typed and compiled language known for its efficiency and concurrency, debugging takes on unique challenges and opportunities. This post explores powerful debugging tools and techniques, focusing on advanced strategies like using the Delve debugger, diagnosing memory leaks, profiling performance bottlenecks, and troubleshooting distributed systems. We'll also delve into sophisticated tools like flamegraphs and tracing to uncover deeper insights into application behavior.
The Power of Delve: A Go-Specific Debugger
What is Delve?
Delve is the official debugger for Go and is specifically designed to help developers troubleshoot and inspect Go programs. It integrates with IDEs like Visual Studio Code, IntelliJ, and GoLand, but can also be run in a terminal for more granular control. Delve is indispensable for understanding complex bugs in Go code and can be used in both local development environments and production systems.
Key Delve Features
Breakpoints: Set breakpoints to pause execution at a certain point in the code to inspect variable values and call stacks.
Stepping: Step through code line by line (or instruction by instruction) to observe how the program behaves as it runs.
Stack Traces: Capture and view stack traces to understand where and why the program crashed or entered an unexpected state.
Variable Inspection: Evaluate variables and expressions during debugging sessions to verify their values at runtime.
Example: Debugging with Delve
# Start your program with Delve
dlv debug main.go
# Set a breakpoint at a specific line
(dlv) break main.go:42
# Start the program and hit the breakpoint
(dlv) continue
# Step through the code
(dlv) step
# Inspect variables
(dlv) print myVariable
Delve also allows remote debugging, which is essential for debugging applications running in production or on remote servers. To enable remote debugging, you can use:
dlv debug --headless --listen=:2345 --api-version=2 --accept-multiclient
Then connect your IDE or debugger to this remote instance to begin your debugging session.
Debugging Memory Leaks in Go
Memory leaks in Go can occur when your program allocates memory without releasing it, leading to increased memory usage and eventually causing the application to crash. Although Go has a garbage collector (GC), it doesn’t automatically manage all memory allocations, especially in cases where objects are unintentionally retained.
Identifying Memory Leaks
- Heap Profiling with pprof: Go’s built-in
pprof
package helps you identify memory usage patterns. By generating heap profiles, you can observe how memory is allocated and retained over time.
import (
"net/http"
"net/http/pprof"
)
func init() {
go func() {
log.Println(http.ListenAndServe("localhost:6060", nil))
}()
}
After adding the above code, you can access heap profiling data via the following URL: http://localhost:6060/debug/pprof/heap
.
- Heap Dump Analysis: When you generate heap profiles, tools like Go’s
pprof
can help you analyze the memory allocations and find which objects are not being freed. You can generate a heap profile using the command:
go tool pprof http://localhost:6060/debug/pprof/heap?debug=1
Once you have the heap profile, use pprof
to visualize allocations:
go tool pprof -web heap.out
- Go Test and Benchmark Memory Usage: A good practice is to write tests and benchmarks for memory usage. By using the
testing
package and running tests with memory benchmarks, you can detect abnormal memory consumption early.
func BenchmarkMemory(b *testing.B) {
for i := 0; i < b.N; i++ {
// Code to test memory usage
}
}
Mitigating Memory Leaks
Use sync.Pool: For heavy memory usage scenarios,
sync.Pool
helps reduce memory allocations by reusing memory buffers, which can mitigate the impact of frequent GC cycles.Avoid Retaining References: Ensure that no unnecessary references are kept to objects that should be garbage collected.
Properly Close Resources: Always close network connections, file handles, or database connections after use to avoid memory leaks.
Profiling Performance Bottlenecks
Performance issues, such as high CPU usage or slow response times, can stem from many areas of a Go program. Profiling tools help identify where time is being spent and which parts of your code need optimization.
Using pprof for Performance Profiling
Go provides the pprof
package to generate and analyze CPU profiles, memory profiles, and other runtime statistics. You can integrate it into your application to start collecting performance data during runtime.
CPU Profiling
To generate a CPU profile, use runtime/pprof
to start and stop the profiler:
import (
"os"
"runtime/pprof"
)
func startCPUProfile() *os.File {
file, err := os.Create("cpu.prof")
if err != nil {
log.Fatal(err)
}
pprof.StartCPUProfile(file)
return file
}
func stopCPUProfile(file *os.File) {
pprof.StopCPUProfile()
file.Close()
}
This allows you to generate a CPU profile which you can then analyze with the go tool pprof
command.
go tool pprof cpu.prof
You can then visualize the flame graph of your CPU profile using -web
:
go tool pprof -web cpu.prof
Memory Profiling
Memory profiling is done similarly to CPU profiling, but instead of CPU time, you analyze memory allocations over time.
func startMemProfile() *os.File {
file, err := os.Create("mem.prof")
if err != nil {
log.Fatal(err)
}
pprof.WriteHeapProfile(file)
return file
}
Flamegraphs and Visualization
Flamegraphs are one of the most effective ways to visualize performance bottlenecks. They allow you to visualize the call stack and where the program spends most of its time. A well-constructed flamegraph provides clear insights into which functions or processes are causing delays.
To generate a flamegraph:
Run
go tool pprof
on your profile.Use
-web
to generate an SVG image of the flamegraph.Open the resulting SVG in a browser to analyze the CPU usage.
Tracing Distributed Systems
As your Go applications scale into distributed systems, debugging becomes more challenging. Distributed systems often involve multiple services, databases, queues, and other systems interacting asynchronously, which makes tracing requests across different parts of the system essential.
Distributed Tracing with OpenTelemetry
OpenTelemetry is a powerful framework for collecting distributed traces. It provides libraries for instrumenting Go applications with trace data. Once your application is instrumented, traces provide a visual map of how requests flow through your system.
- Setup OpenTelemetry: Install the necessary OpenTelemetry packages and set up the tracing for your Go application.
go get github.com/open-telemetry/opentelemetry-go
- Trace HTTP Requests: By using middleware, you can automatically trace incoming HTTP requests.
import (
"github.com/open-telemetry/opentelemetry-go/otel"
"github.com/open-telemetry/opentelemetry-go/otel/trace"
)
func traceMiddleware(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
ctx, span := otel.Tracer("example.com/trace").Start(r.Context(), "handleRequest")
defer span.End()
next.ServeHTTP(w, r.WithContext(ctx))
})
}
- Export Traces: OpenTelemetry allows exporting trace data to various backends, like Jaeger, Zipkin, or AWS X-Ray. These platforms offer visualizations to help identify where bottlenecks occur across distributed systems.
Visualizing Distributed Traces
Once traces are exported to a platform like Jaeger or Zipkin, you can analyze the full lifecycle of requests across services, pinpointing latency, failures, and unexpected behaviors that are hard to catch without tracing.
Conclusion
Mastering debugging in Go requires a mix of solid foundational skills, tools like Delve, and advanced techniques for diagnosing issues like memory leaks, performance bottlenecks, and issues in distributed systems. Delve offers fine-grained control for local debugging, while pprof and OpenTelemetry give insight into performance and distributed tracing. By incorporating these advanced debugging tools and techniques, you can ensure that your Go applications are not only functional but optimized and reliable. Whether you're debugging memory issues in a single service or tracing distributed requests across a microservices architecture, these tools are invaluable assets for any Go developer.
Subscribe to my newsletter
Read articles from JealousGx directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
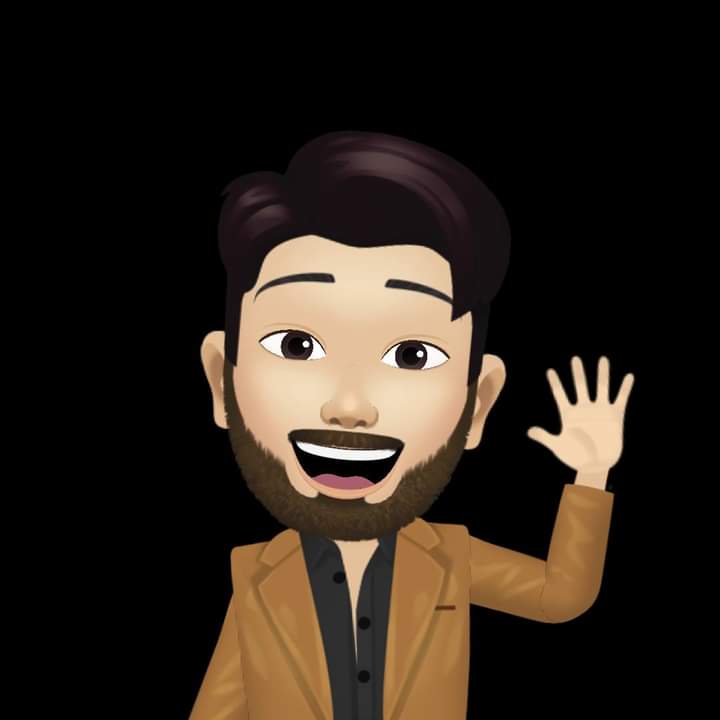
JealousGx
JealousGx
Hello, I'm a highly skilled full stack web developer with a rich background in creating and maintaining dynamic websites that drive online engagement and brand awareness. My expertise extends beyond WordPress to include a versatile skill set.