How Langchain Agents handling multiple workflows autonomously (Weather API case)
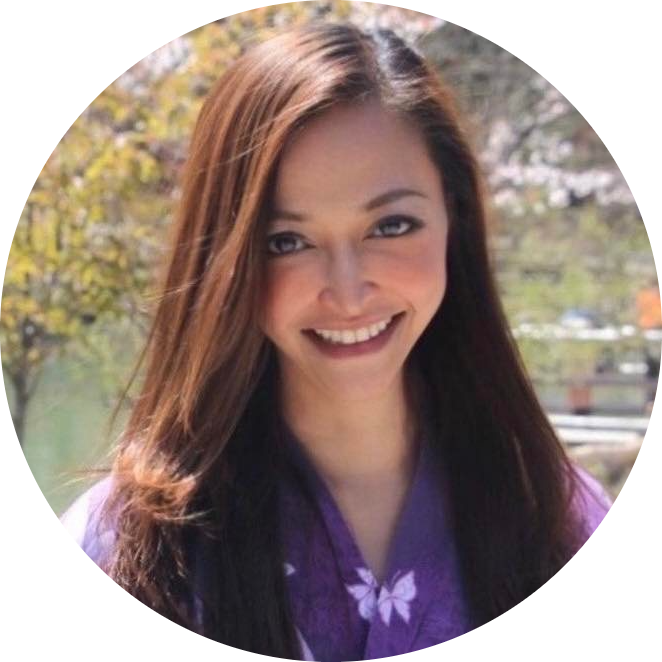
Let’s build an example where LangChain agents autonomously handle workflows by chaining tasks, such as extracting data from an API, summarizing it, and storing the output into a database.
Goal:
An agent fetches weather data from an API, summarizes the forecast, and logs the output into a database. No manual intervention required!
Code: LangChain Agent Workflow
1. Install Required Libraries
!pip install langchain openai sqlite3 requests
2. Import Libraries
from langchain.agents import initialize_agent, Tool
from langchain.tools import BaseTool
from langchain.llms import OpenAI
import sqlite3
import requests
3. Define Custom Tools
Tool 1: Fetch Weather Data
class WeatherTool(BaseTool):
name = "fetch_weather"
description = "Fetch weather data for a given city."
def _run(self, city: str):
api_key = "YOUR_WEATHER_API_KEY" # Replace with your API key
url = f"http://api.weatherapi.com/v1/current.json?key={api_key}&q={city}"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
return f"Weather in {city}: {data['current']['condition']['text']}, {data['current']['temp_c']}°C."
else:
return "Failed to fetch weather data."
def _arun(self, *args, **kwargs):
raise NotImplementedError("Async mode not supported.")
Tool 2: Store Data in SQLite
class DatabaseTool(BaseTool):
name = "store_data"
description = "Store a string of text in the database."
def _run(self, data: str):
conn = sqlite3.connect("weather.db")
cursor = conn.cursor()
cursor.execute("""
CREATE TABLE IF NOT EXISTS weather_log (
id INTEGER PRIMARY KEY AUTOINCREMENT,
summary TEXT
)
""")
cursor.execute("INSERT INTO weather_log (summary) VALUES (?)", (data,))
conn.commit()
conn.close()
return "Data saved to database."
def _arun(self, *args, **kwargs):
raise NotImplementedError("Async mode not supported.")
4. Set Up the LLM and Agent
# Initialize the language model
llm = OpenAI(model="gpt-3.5-turbo", temperature=0)
# Register tools
tools = [
WeatherTool(),
DatabaseTool()
]
# Initialize the agent
agent = initialize_agent(tools, llm, agent="zero-shot-react-description", verbose=True)
5. Run the Workflow
# Input to the agent
city = "San Francisco"
response = agent.run(f"Get the current weather in {city} and save it to the database.")
print(response)
Sample Output
Agent Logs (Verbose Mode)
> Entering new AgentExecutor chain...
Action: fetch_weather
Action Input: San Francisco
Observation: Weather in San Francisco: Partly Cloudy, 15°C.
Thought: I have the weather information. I will now save it to the database.
Action: store_data
Action Input: Weather in San Francisco: Partly Cloudy, 15°C.
Observation: Data saved to database.
Final Answer: The weather data has been fetched and stored in the database.
> Finished chain.
Database Content
Run the following to view the database content:
conn = sqlite3.connect("weather.db")
cursor = conn.cursor()
cursor.execute("SELECT * FROM weather_log")
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
Output:
(1, 'Weather in San Francisco: Partly Cloudy, 15°C.')
What’s Happening Here?
Agent Autonomy: The agent independently:
Fetches weather data from an API.
Processes the response and logs it into a database.
Chained Workflow: Tools are seamlessly integrated into the agent, automating end-to-end operations.
Flexibility: Modify tools to include new capabilities, such as sending the summary via email or adding more API integrations.
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
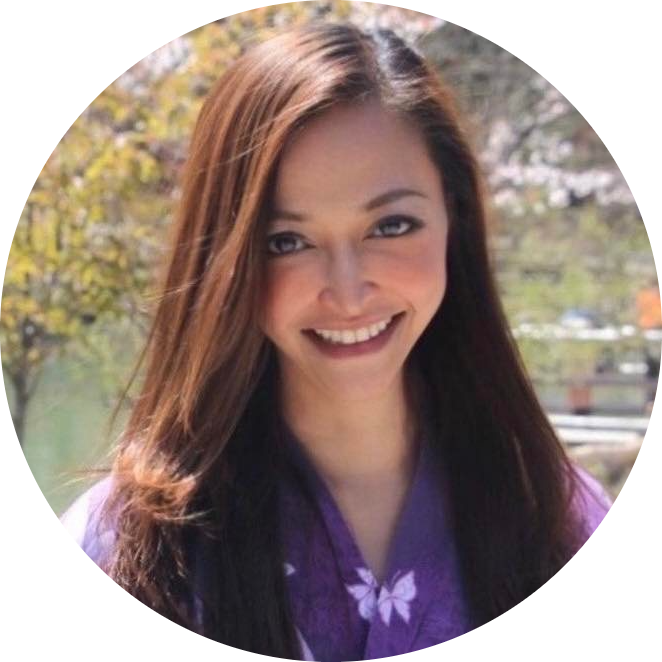