Zero Bit In Javascript
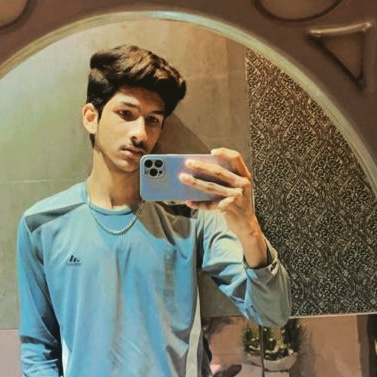
In JavaScript, a variable representing a "zero bit" means it holds no set bits, which is simply the number 0
. Here’s how you can work with such a variable and manipulate it effectively:
Initializing a Zero-Bit Variable
You can declare and initialize a variable with 0
:
let zeroBit = 0;
This variable has all bits unset.
Checking if a Variable Has Zero Bits
You can check if a number has no set bits using:
if (zeroBit === 0) {
console.log("This variable has zero bits set.");
}
Setting a Bit in the Variable
You can set a specific bit in the zero-bit variable:
let bit = 2; // Example: Setting the 2nd bit
zeroBit = zeroBit | (1 << bit);
console.log(zeroBit.toString(2)); // Output: "100"
Unsetting Bits
If the variable starts as 0
, all bits are already unset, so unsetting won't change it. But here’s how you can do it:
zeroBit = zeroBit & ~(1 << 2); // This would still result in 0
console.log(zeroBit.toString(2)); // Output: "0"
Using Zero-Bit Variable in Functions
For instance, if you’re working with a function that manipulates bits, you can use the zero-bit variable as the starting point:
function createNumberWithSetBits(bitPositions) {
let num = 0; // Start with a zero-bit variable
for (let bit of bitPositions) {
num = num | (1 << bit);
}
return num;
}
// Example usage:
let result = createNumberWithSetBits([0, 2, 4]); // Sets bits 0, 2, and 4
console.log(result.toString(2)); // Output: "10101"
Example: Working with Zero-Bit Variable
Here’s how you might use a zero-bit variable to construct a number with exactly n
set bits:
function createNumberWithNBits(n) {
let num = 0; // Start with zero bits
for (let i = 0; i < n; i++) {
num = num | (1 << i); // Set the i-th bit
}
return num;
}
// Example: Create a number with 3 set bits
console.log(createNumberWithNBits(3).toString(2)); // Output: "111"
Key Operations for Zero-Bit Variable
Set a bit:
num = num | (1 << bit)
Unset a bit:
num = num & ~(1 << bit)
Toggle a bit:
num = num ^ (1 << bit)
Check if a bit is set:
(num & (1 << bit)) !== 0
Subscribe to my newsletter
Read articles from Mikey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
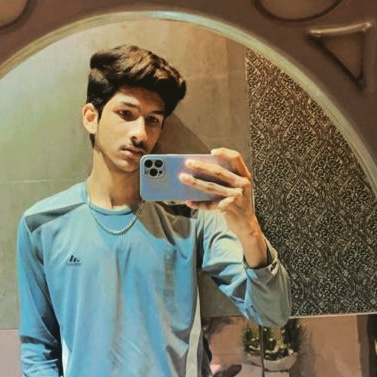
Mikey
Mikey
Undergrad Student in domain of Web developer at Chandigarh University.