Extra memory for an Agentic AI w/Chroma
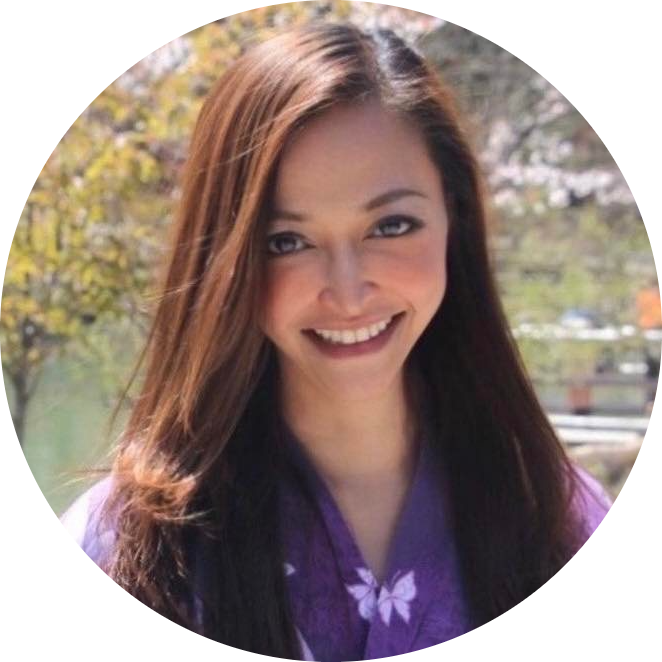
Scenario: Building an AI assistant with Chroma memory
Goal: Use Chroma to provide long-term, embedding-based memory for conversations.
The agent evolves its responses based on prior interactions, enabling personalized, context-aware conversations.
Step-by-Step Code Implementation
1. Install Required Libraries
!pip install chromadb langchain openai
Purpose:
chromadb
: Manages the memory system, storing embeddings and contexts.langchain
: Integrates Chroma memory with LLMs.openai
: Connects to OpenAI’s GPT-3.5 or GPT-4 for conversation.
What Happens: Libraries are installed for managing memory and building the agent.
2. Import Libraries
from langchain.chains import ConversationChain
from langchain.memory import ChromaMemory
from langchain.llms import OpenAI
from chromadb.config import Settings
Purpose:
ConversationChain
: Handles the flow of conversation using memory.ChromaMemory
: Embedding-based memory system that retains context.OpenAI
: Powers the LLM for natural language processing.
What Happens: These components will be used to integrate the memory system with the LLM.
3. Initialize Chroma Memory
# Configure Chroma settings
chroma_settings = Settings(
persist_directory="chroma_memory", # Directory to save memory
chroma_db_impl="duckdb+parquet", # Database type
anonymized_telemetry=False
)
# Create Chroma memory
memory = ChromaMemory(settings=chroma_settings)
Purpose:
Configures Chroma to store memory in a persistent directory (
chroma_memory
).Enables embedding-based storage for long-term memory retention.
What Happens:
A Chroma database is created to store conversation embeddings.
Memory will persist even after the session ends.
4. Initialize the LLM and Conversation Chain
# Set up the LLM (OpenAI GPT)
llm = OpenAI(model="gpt-3.5-turbo", temperature=0.7)
# Create a conversation chain with memory
conversation = ConversationChain(
llm=llm,
memory=memory,
verbose=True
)
Purpose:
llm
: Defines the language model (GPT-3.5 or GPT-4).conversation
: Combines the LLM and Chroma memory for context-aware conversations.
What Happens:
The AI agent is now capable of retaining memory between queries.
Verbose mode provides detailed logs of how memory is used during interactions.
5. Engage in a Conversation
# First interaction
response_1 = conversation.run("Hi, I love Python programming!")
print(response_1)
# Second interaction
response_2 = conversation.run("What did I just tell you about my interests?")
print(response_2)
# Third interaction
response_3 = conversation.run("Can you suggest a Python library for data visualization?")
print(response_3)
Purpose:
- Simulates a conversation where the agent retains memory and adapts responses based on past interactions.
What Happens:
First Interaction: The user’s preference for Python programming is stored in Chroma memory.
Second Interaction: The agent recalls and references the stored memory.
Third Interaction: The agent provides relevant suggestions based on the context.
Sample Output
Verbose Logs
> Entering new ConversationChain...
Memory retrieved: []
Thought: Respond to the user's greeting.
Output: Hi! Python programming is awesome. How can I assist you?
> Finished chain.
> Entering new ConversationChain...
Memory retrieved: [{'user_input': 'Hi, I love Python programming!', 'output': 'Hi! Python programming is awesome. How can I assist you?'}]
Thought: The user mentioned loving Python programming earlier. I should reference it.
Output: You mentioned that you love Python programming. How can I assist you further?
> Finished chain.
> Entering new ConversationChain...
Memory retrieved: [{'user_input': 'Hi, I love Python programming!', 'output': 'Hi! Python programming is awesome. How can I assist you?'},
{'user_input': 'What did I just tell you about my interests?', 'output': 'You mentioned that you love Python programming. How can I assist you further?'}]
Thought: The user is asking for a Python library. Recall their interest in programming.
Output: For data visualization, I recommend using Matplotlib or Seaborn. They are powerful Python libraries for creating charts and graphs.
> Finished chain.
Final Output
Agent Response 1: Hi! Python programming is awesome. How can I assist you?
Agent Response 2: You mentioned that you love Python programming. How can I assist you further?
Agent Response 3: For data visualization, I recommend using Matplotlib or Seaborn. They are powerful Python libraries for creating charts and graphs.
Step-by-Step Explanation of Impact
First Interaction:
The user mentions they love Python programming.
This input is embedded and stored in Chroma memory for future reference.
Second Interaction:
The agent retrieves the stored memory.
It references the user’s interest in Python programming to deliver a personalized response.
Third Interaction:
- The agent uses both memory and the context of the current query to suggest relevant Python libraries.
Why This Matters
Before Chroma: The agent would forget the user’s preferences after each query.
After Chroma: The agent retains and evolves its understanding, enabling more natural, personalized conversations.
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
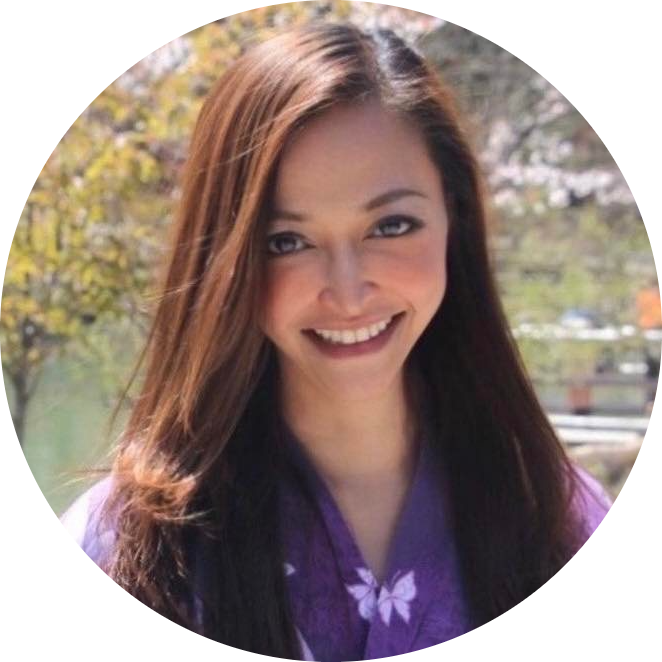