Lambda expressions and function types

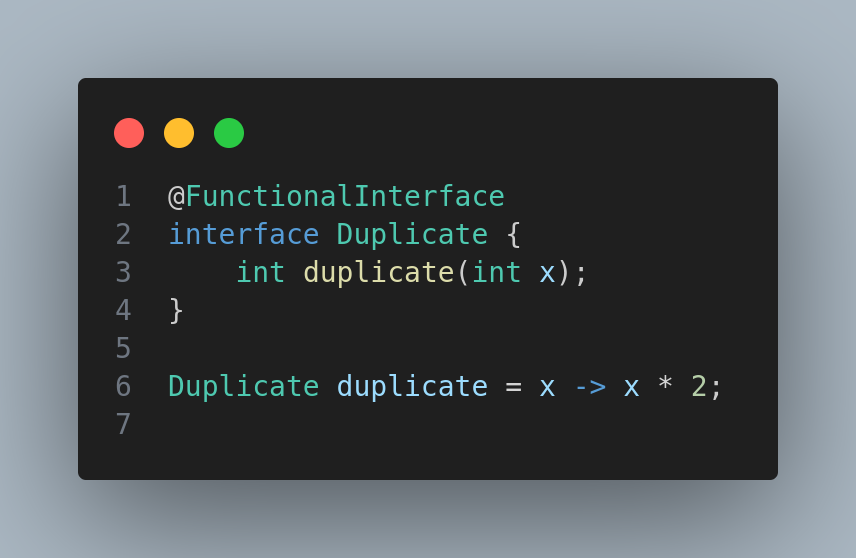
Introduction to Lambda Expressions
A lambda expression or anonymous method is a shortcut to define an implementation of a functional interface.
It has the following syntax:
(parameters) -> {statements/s}
(Integer number) -> {
return number * 2;
}
The parameter type can be inferred:
(number) -> {
return number * 2;
}
Since the method body is a single expression, the curly braces and the return keyword are not necessary.
(number) -> number * 2;
If there is only one parameter, the parentheses can be omitted:
number -> number * 2;
Now we have a very -maybe too much- compact lambda expression. But how do we use it ?
function duplicate(int number) -> number * 2; // Invalid code
and then we might want to invocate the function:
duplicate(3); // Invalid code
But this does not work, function
is not a Java keyword. There are only classes and interfaces in Java. In order to maintain its famous backward compatibility, the language designers decided to relate lambdas with ordinary interfaces:
@FunctionalInterface
public interface MyFunction {
int duplicate(int number);
}
We can finally write a valid lambda expression:
public class MyClass implements MyFunction {
MyFunction myFunction = (number) -> number * 2;
...........................................
}
and call it:
System.out.println(myFunction.duplicate(3)); // 6
We can now understand the definition given in the beginning of this article:
A lambda expression or anonymous method is an implementation of a functional interface. It was introduced in Java 8.
A functional interface is an interface that contains only one abstract method. Also called SAM ("single abstract method") interface.
The @FunctionalInterface
annotation is used to mark interfaces that only have one abstract method. It is not required, since there might be SAM interfaces declared in earlier Java versions.
@FunctionalInterface // This annotation is optional, but recommended
public interface MyFunction {
int duplicate(int number);
}
In other words, the implementation of the abstract method of a functional interface is the equivalent to a function in other languages. In a modern JVM language like Kotlin, this is the equivalent code:
fun duplicate(number: Int): Int {
return number * 2
}
Function Types
Do we have to write a functional interface every time we want to use a lambda expression ? No, because Java provides a series of pre-defined functional interfaces called function types
.
Function<T, R> and friends
These functional interfaces represent functions that take a parameter of type T
and return a result of type R
.
Function<Integer, Integer> duplicate = x -> x * 2;
System.out.println(duplicate.apply(3)); // 6
Notice the parameters are not primitive types, but objects.
Equivalent code in Kotlin:
fun duplicate(x: Int): Int {
return x * 2
}
It is probably more clear in Kotlin or Scala, but you can get used to it. In my opinion, Java language designers did a good work to maintain the backwards compatibility. However, using these pre-defined function types makes you to have to learn a number of other functional interfaces and their corresponding default methods.
Default methods are provided for the Function interface: andThen()
and compose()
Function<Integer, Integer> duplicate = x -> x * 2;
Function<Integer, Integer> addOne = x -> x + 1;
Function<Integer, Integer> duplicatePlusOne = duplicate.andThen(addOne);
System.out.println(duplicatePlusOne.apply(3)); // 7
That code is equivalent to this one:
Function<Integer, Integer> duplicate = x -> x * 2;
Function<Integer, Integer> addOne = x -> x + 1;
Function<Integer, Integer> duplicatePlusOne = addOne.compose(duplicate);
System.out.println(duplicatePlusOne.apply(3)); // 7
BiFunction<T, U, R> and friends
These functional interfaces represent functions that take two parameters of type T
and U
and return a result of type R
.
BiFunction<Integer, Integer, Integer> add = (x, y) -> x + y;
System.out.println(add.apply(3, 4)); // 7
Other pre-defined function types
UnaryOperator<Integer> square = (x) -> x * x;
System.out.println(square.apply(3)); // 9
BinaryOperator<Integer> add = (x, y) -> x + y;
System.out.println(add.apply(3, 4)); // 7
and when a primitive data type is returned:
ToIntFunction - takes a T and returns an int.
ToLongFunction - takes a T and returns a long.
ToDoubleFunction - takes a T and returns a double.
The Function
interface is for operations that take a single parameter and return a single value, while the BiFunction
interface is designed for operations that take two parameters.
Predicate<T>
This functional interface represents functions that take a parameter of type T
and return a boolean value. It's mainly used for filtering and evaluating conditions.
Predicate<Integer> isEven = x -> x % 2 == 0;
boolean result = isEven.test(4); // true
The Predicate interface also provides several default methods: and()
, or()
, negate()
:
Predicate<Integer> isEven = x -> x % 2 == 0;
Predicate<Integer> isGreaterThan10 = x -> x > 10;
Predicate<Integer> isEvenAndGreaterThan10 = isEven.and(isGreaterThan10);
boolean result = isEvenAndGreaterThan10.test(12); // true
Predicate<String> containsA = str -> str.contains("A");
Predicate<String> containsB = str -> str.contains("B");
Predicate<String> containsEither = containsA.or(containsB);
Predicate<Integer> isEven = x -> x % 2 == 0;
Predicate<Integer> isOdd = isEven.negate();
Consumer<T>
It's a functional interface that takes a parameter of type T
and performs an action without returning a value.
Consumer<String> greeting = s -> System.out.println(s);
greeting.accept("Hello, World!");
In my opinion, the above default method could have been called apply()
in order to be consistent with the Function function type.
The Consumer interface also provides the default method andThen()
, which allows for chaining multiple Consumer operations. This is similar to the and()
method of the Function interface seen above.
Supplier<T>
It's a functional interface that returns a value of type T
without taking any input arguments. It's the oppossite of the Consumer. It has a single abstract method called T get()
.
Supplier<String> supplier = () -> "Hello, World!";
System.out.println(supplier.get());
Suppliers are useful to generate values in a lazy way. The exact meaning of this deserves its own article.
Method References
Besides lambdas, Java 8 introduced method references, which are a way to refer to a method without having to specify the full method signature.
Consumer<String> greeting = s -> System.out.println(s);
Consumer<String> greeting = System.out::println;
greeting.accept("Hello, World!");
The general syntax for method references is ClassName::methodName
, where ClassName
is the name of the class containing the method and methodName
is the name of the method to be called.
This is especially useful when using custom classes:
class Employee {
String getName() {
return name;
}
......................
}
With method references we can do this:
Consumer<Employee> printName = employee -> employee.getName();
Consumer<Employee> printName = Employee::getName;
Constructor References
Instead of
Supplier<Employee> employee = () -> new Employee();
use
Supplier<Employee> employee = Employee::new;
Lambda expressions and Streams
In conjunction with lambdas, Streams provide a way to process sequences of data elements in a functional style. Streams allow to perform operations like filtering, mapping, and reducing on data, making the code much more concise and readable.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sumOfEvenSquares = numbers.stream()
.filter(number -> number % 2 == 0)
.map(number -> number * number)
.reduce(0, Integer::sum);
System.out.println("Sum of squares of even numbers:" + sumOfEvenSquares);
There is much more to explain about the Streams API, but this is enough to show how they use lambda expressions.
Lambda Expressions in other Java packages
In addition to the function types, there are more pre-defined functional interfaces in other Java libraries.
@FunctionalInterface
public interface Comparator<T> {
int compare(T obj1, T obj2);
}
The abstract method above compares its two arguments for order. Returns a negative integer, zero, or a positive integer if the first argument is less than, equal to, or greater than the second. (Source: Comparator official docs)
When using custom classes, we probably have to create our own Comparator:
public class EmployeeComparator implements Comparator<Employee> {
@Override
public int compare(Employee empl1, Employee empl2) {
return Integer.compare(empl1.getAge(), empl2.getAge());
}
}
The Comparator interface has a number of default and static methods that can be used with lambda expressions.
Functional Interfaces before Java 8
Although the term functional interface
was introduced in Java 8, there were a number of interfaces with a single abstract method in earlier Java versions.
For example, the Comparator
interface was declared in the same way, but without using the @FunctionalInterface
annotation.
The main difference is that the Comparator
interface was implemented using anonymous inner classes:
Collections.sort(employeeList, new Comparator<Employee>() {
@Override
public int compare(Employee e1, Employee e2) {
return e1.getName().compareTo(e2.getName());
}
});
This was more verbose than the lambda expressions.
Conclusion
We have explained lambda expressions and functional interfaces, features introduced in Java 8. Then we explained the different function types, which allow us to create powerful transformations of data. Method references provide a shorthand for lambda expressions, making our code even more readable.
A small comparison with the equivalent code used with anonymous classes allows us to observe how powerful the lambda expressions are when used with pre-defined functional interfaces.
Subscribe to my newsletter
Read articles from José Ramón (JR) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

José Ramón (JR)
José Ramón (JR)
Software Engineer for quite a few years. From C programmer to Java web programmer. Very interested in automated testing and functional programming.