Mastering SQL Concepts: Performance Optimization, Deadlocks, and ACID Properties Explained
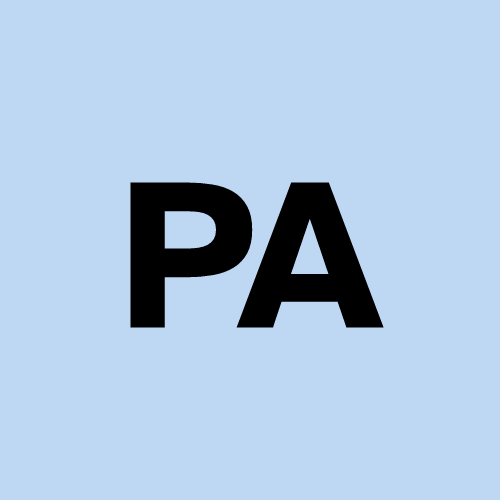
In today's data-driven world, the ability to efficiently manage and query databases is an indispensable skill for professionals. Whether you're preparing for a technical interview or honing your SQL skills, understanding performance optimization, deadlocks, and the foundational principles of ACID properties can set you apart. In this blog, we'll break down essential SQL concepts, provide practical examples, and share best practices to improve your database expertise.
1. What Are Indexes? How Do They Improve Query Performance?
Indexes:
An index is a database object that improves the speed of data retrieval operations on a table at the cost of additional storage and maintenance overhead.
It works like an index in a book: instead of scanning the entire book for a topic, you jump directly to the relevant page by referring to the index.
Types of Indexes in SQL Server:
Clustered Index:
Physically sorts and stores the data rows in the table based on the indexed column.
A table can have only one clustered index.
Non-Clustered Index:
Stores the index separately from the data.
Points to the data row via a pointer.
A table can have multiple non-clustered indexes.
How They Improve Query Performance:
Instead of scanning every row in the table (a full table scan), the database can use the index to directly locate the rows that satisfy the query conditions.
Example:
If you frequently query by
salary
, creating an index on thesalary
column speeds up the query:sqlCopyEditCREATE INDEX idx_salary ON employee (salary);
Queries like
SELECT * FROM employee WHERE salary > 5000
will now be faster.
Trade-offs of Indexes:
Storage Cost: Indexes take up additional storage.
Write Overhead: Insert, update, or delete operations become slower because indexes must be updated.
2. How Would You Optimize a Query That Takes Too Long to Execute?
Steps to Optimize:
Analyze the Query Execution Plan:
- Use SQL Server’s Execution Plan to see which part of the query is consuming the most resources.
Add Indexes:
- Create indexes on columns used in
WHERE
,GROUP BY
,ORDER BY
, and join conditions.
- Create indexes on columns used in
Avoid
SELECT *
:Fetch only the necessary columns instead of selecting all columns.
Example: Instead of:
sqlCopyEditSELECT * FROM employee;
Use:
sqlCopyEditSELECT name, department FROM employee;
Rewrite Subqueries to Joins:
Subqueries can often be rewritten as joins for better performance.
Example:
sqlCopyEditSELECT name FROM employee WHERE salary > (SELECT AVG(salary) FROM employee);
Can be rewritten as:
sqlCopyEditSELECT e.name FROM employee e CROSS JOIN (SELECT AVG(salary) AS avg_salary FROM employee) a WHERE e.salary > a.avg_salary;
Use Proper Data Types:
- Ensure columns have appropriate data types (e.g., avoid using
NVARCHAR(MAX)
unless necessary).
- Ensure columns have appropriate data types (e.g., avoid using
Partition Large Tables:
- Split large tables into smaller, manageable chunks using table partitioning.
Eliminate Unnecessary Joins:
- Remove joins to tables or columns that are not required for the query.
Use Query Hints:
- Provide execution hints (e.g.,
FORCESEEK
) to optimize performance.
- Provide execution hints (e.g.,
3. Explain Normalization and Denormalization with Examples
Normalization:
A process of organizing data in a database to reduce redundancy and improve data integrity.
Data is split into multiple related tables to achieve this.
Forms of Normalization:
1NF (First Normal Form):
No duplicate rows and each cell contains atomic values.
Example:
Non-normalized table:
javascriptCopyEditStudent_ID | Name | Subjects 1 | John | Math, Science
Normalized:
javascriptCopyEditStudent_ID | Name | Subject 1 | John | Math 1 | John | Science
2NF (Second Normal Form):
Remove partial dependencies (no attribute should depend on part of a composite key).
Example:
Table:
Order_ID
,Product_ID
,Product_Name
.Split into:
Table 1:
Order_ID
,Product_ID
.Table 2:
Product_ID
,Product_Name
.
3NF (Third Normal Form):
- Remove transitive dependencies (no non-prime attribute should depend on another non-prime attribute).
Denormalization:
Opposite of normalization, where redundant data is added to improve read performance.
Used in analytical systems where read speed is critical.
Example:
- Instead of splitting
Order
andCustomer
tables, denormalization would combine them into one table for faster reads.
- Instead of splitting
4. Differences Between DELETE, TRUNCATE, and DROP
Feature | DELETE | TRUNCATE | DROP |
Operation | Removes specific rows. | Removes all rows. | Deletes the table structure. |
WHERE Clause | Supported. | Not supported. | Not applicable. |
Rollback | Can be rolled back if in a transaction. | Cannot be rolled back. | Cannot be rolled back. |
Speed | Slower (logs individual rows). | Faster (minimal logging). | Fastest. |
Structure | Table structure remains intact. | Table structure remains intact. | Table structure is removed. |
5. What Is a Deadlock, and How Would You Resolve It in SQL Server?
Deadlock:
- A deadlock occurs when two or more transactions block each other by holding resources that the other transactions need.
Example:
Transaction A locks Table 1 and waits for Table 2.
Transaction B locks Table 2 and waits for Table 1.
Neither transaction can proceed.
Resolving Deadlocks:
Set Deadlock Priority:
- Use
SET DEADLOCK_PRIORITY
to control which transaction should be aborted.
- Use
Minimize Lock Duration:
- Use smaller transactions to reduce the chance of deadlocks.
Access Resources in a Consistent Order:
- Ensure all transactions request resources in the same order.
Use
WITH (NOLOCK)
:For non-critical reads, use
NOLOCK
to avoid locking.Example:
sqlCopyEditSELECT * FROM employee WITH (NOLOCK);
Monitor and Resolve:
- Use SQL Server Profiler or Deadlock Graph to identify and analyze deadlocks.
6. What Are ACID Properties in Databases?
ACID:
Atomicity:
Ensures that a transaction is all-or-nothing.
Example: In a money transfer, either both accounts are updated, or none are.
Consistency:
Guarantees that a transaction brings the database from one valid state to another.
Example: If a foreign key constraint exists, inserting invalid data is not allowed.
Isolation:
Ensures that concurrent transactions do not interfere with each other.
Example: Two users updating the same record do not overwrite each other’s changes.
Durability:
- Ensures that once a transaction is committed, it remains so, even in case of a system crash.
Example of ACID:
In a banking app:
Atomicity: Deduct from one account and add to another, or do nothing.
Consistency: Total balance across accounts must remain unchanged.
Isolation: One user transferring money doesn’t see intermediate states of another user.
Durability: A completed transfer persists even after a power failure.
SQL is more than just a tool for querying databases—it's a gateway to understanding the heart of modern data management. By mastering indexes, query optimization techniques, and concepts like normalization, deadlocks, and ACID properties, you'll be equipped to tackle real-world challenges with confidence. Keep experimenting, practicing, and exploring advanced concepts to unlock the full potential of SQL. Remember, every optimized query brings you one step closer to becoming a database expert. Happy querying!
Subscribe to my newsletter
Read articles from Pragyanshu Aryan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
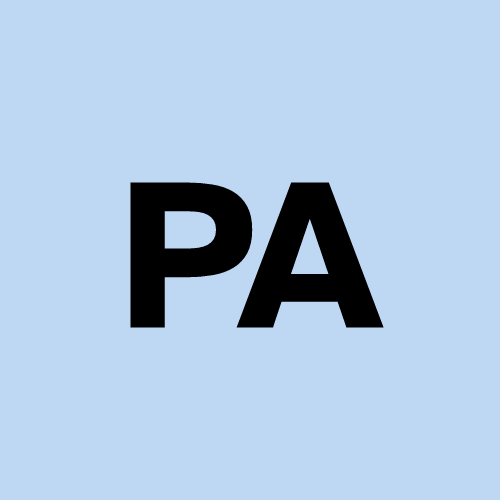