Introducing Color Palette Plus: A Modern Color Generation Library for Flutter
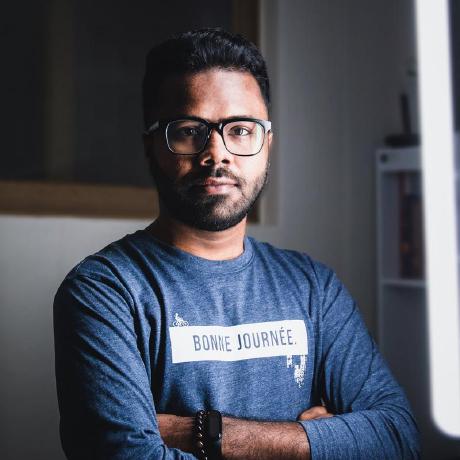
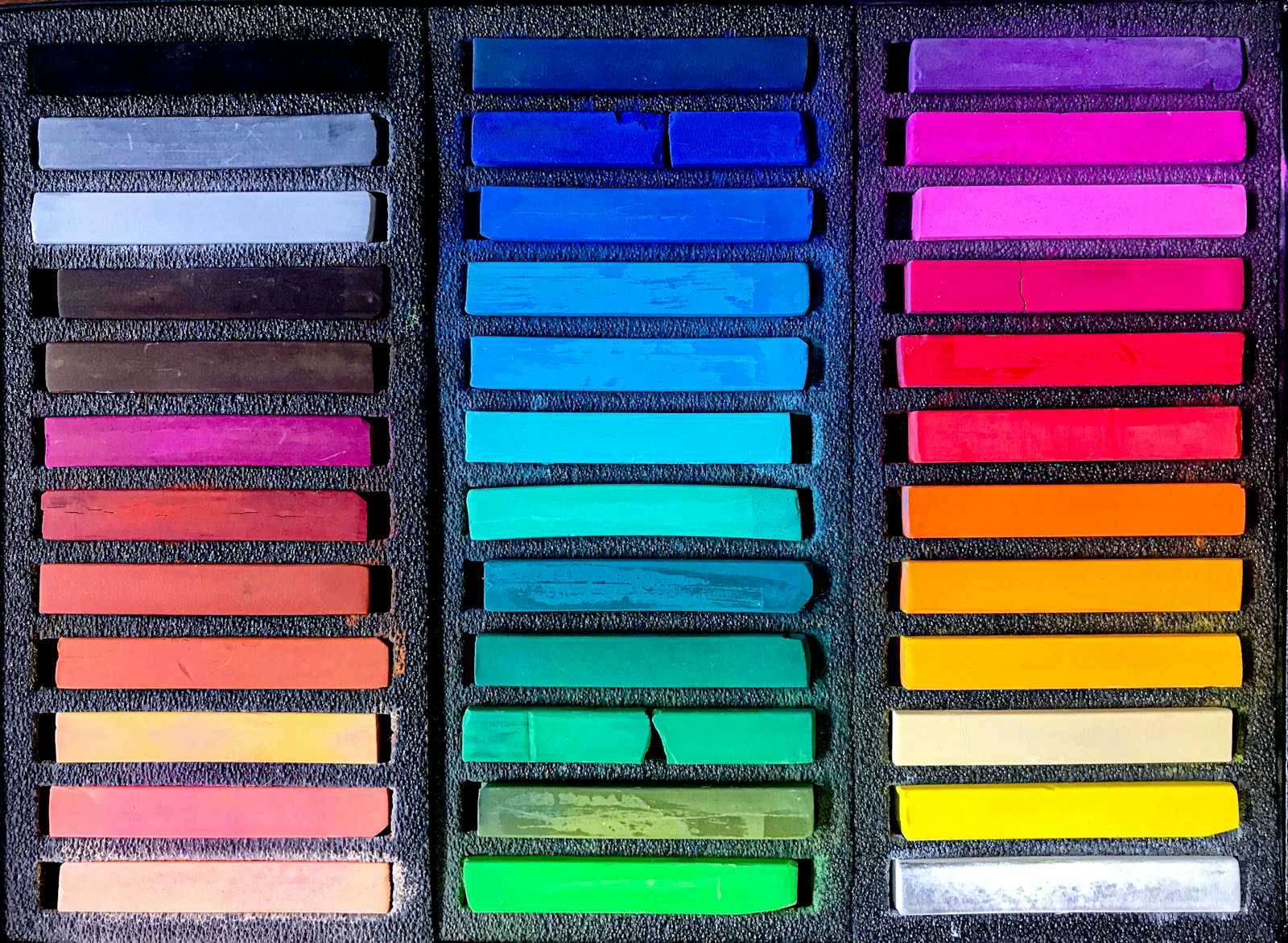
Color management in Flutter applications can be challenging, especially when dealing with color variations, shades, and harmonies. Today, I'm excited to introduce Color Palette Plus, a modern Flutter library that makes color palette generation both simple and efficient while adhering to Flutter's latest best practices.
Why Another Color Library?
With Flutter's recent updates, many existing color manipulation libraries have become outdated, still using deprecated color APIs. Color Palette Plus was built from the ground up to:
Use Flutter's modern color component accessors (
.r
,.g
,.b
,.a
)Properly handle color components in the 0.0-1.0 range
Provide efficient color transformations
Maintain type safety and null safety
Key Features
1. Material Design Swatch Generation
Generate complete Material Design color swatches from any base color:
import 'package:color_palette_plus/color_palette_plus.dart';
final baseColor = Color(0xFF2196F3); // Blue
final materialSwatch = ColorPalette.generateSwatch(baseColor);
// Access shades
final primaryColor = materialSwatch[500];
final lightVariant = materialSwatch[200];
final darkVariant = materialSwatch[700];
2. Color Harmonies
Create harmonious color combinations with built-in generators:
// Monochromatic variations
final monochromaticColors = ColorPalettes.monochromatic(
baseColor,
steps: 5,
);
// Analogous colors
final analogousColors = ColorPalettes.analogous(
baseColor,
steps: 3,
angle: 30,
);
// Complementary colors
final complementaryColors = ColorPalettes.complementary(baseColor);
3. Flexible Shade Access
Access color shades in multiple ways:
// Get a specific shade
final shade = ColorPalette.getShade(baseColor, 500);
// Get all shades as a map
final allShades = ColorPalette.getAllShades(baseColor);
Real-World Usage Examples
1. Dynamic Theme Generation
Create dynamic themes based on a brand color:
MaterialApp(
theme: ThemeData(
primarySwatch: ColorPalette.generateSwatch(brandColor),
// Use specific shades for different elements
scaffoldBackgroundColor: ColorPalette.getShade(brandColor, 50),
appBarTheme: AppBarTheme(
backgroundColor: ColorPalette.getShade(brandColor, 500),
),
),
)
2. Custom Color Schemes
Generate custom color schemes for your UI:
class CustomColorScheme {
final Color primary;
final Color secondary;
final Color accent;
CustomColorScheme(Color baseColor) {
final swatch = ColorPalette.generateSwatch(baseColor);
primary = swatch[500]!;
// Generate analogous colors for variety
final analogous = ColorPalettes.analogous(baseColor);
secondary = analogous[1];
accent = analogous[2];
}
}
3. Accessible Color Combinations
Create accessible color pairs:
Widget buildAccessibleText(Color backgroundColor) {
// Get contrasting text color based on background
final textColor = backgroundColor.computeLuminance() > 0.5
? ColorPalette.getShade(backgroundColor, 900)
: ColorPalette.getShade(backgroundColor, 50);
return Container(
color: backgroundColor,
child: Text(
'Accessible Text',
style: TextStyle(color: textColor),
),
);
}
Performance Tips
1. Cache Generated Colors
Instead of generating colors in build methods:
class ColorProvider extends ChangeNotifier {
late final MaterialColor _primarySwatch;
late final List<Color> _analogousColors;
ColorProvider(Color baseColor) {
_primarySwatch = ColorPalette.generateSwatch(baseColor);
_analogousColors = ColorPalettes.analogous(baseColor);
}
MaterialColor get primarySwatch => _primarySwatch;
List<Color> get analogousColors => _analogousColors;
}
2. Avoid Repeated Generations
Cache commonly used shades:
class ThemeColors {
static final Map<Color, MaterialColor> _swatchCache = {};
static MaterialColor getSwatchFor(Color color) {
return _swatchCache.putIfAbsent(
color,
() => ColorPalette.generateSwatch(color),
);
}
}
Best Practices
Shade Selection
Use 500 for the primary color
Use 50-200 for backgrounds
Use 700-900 for text on light backgrounds
Use 50-100 for text on dark backgrounds
Color Harmony
Use monochromatic palettes for subtle variations
Use analogous colors for related UI elements
Use complementary colors for contrast and emphasis
Accessibility
Always test contrast ratios
Provide sufficient color contrast for text
Don't rely solely on color to convey information
Getting Started
- Add to your pubspec.yaml:
dependencies:
color_palette_plus: ^1.0.0
- Import the library:
import 'package:color_palette_plus/color_palette_plus.dart';
- Start using it in your code!
Requirements
Flutter ≥ 3.27.0
Dart ≥ 3.6.0
Pub Dev Link: color_palette_plus
Conclusion
Color Palette Plus simplifies color management in Flutter while ensuring you're using modern, efficient practices. Whether you're building a theme system, creating a design system, or just need some color variations, Color Palette Plus has you covered.
Check out the GitHub repository for more examples and documentation. We welcome contributions and feedback from the community!
What's Next?
We're planning to add more features in future releases:
Color palette export/import
Additional color harmony algorithms
Color accessibility scoring
Color naming suggestions
Stay tuned for updates, and don't forget to star the repository if you find it useful!
Happy coding! 🎨 ✨
Subscribe to my newsletter
Read articles from Ishanga Vidusha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
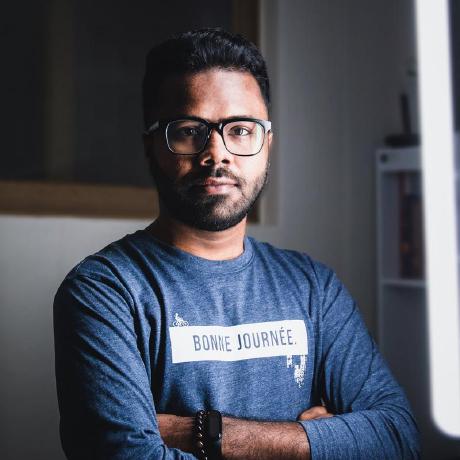