Deploying a .NET Application to DigitalOcean Using Docker Containers and Container Registry
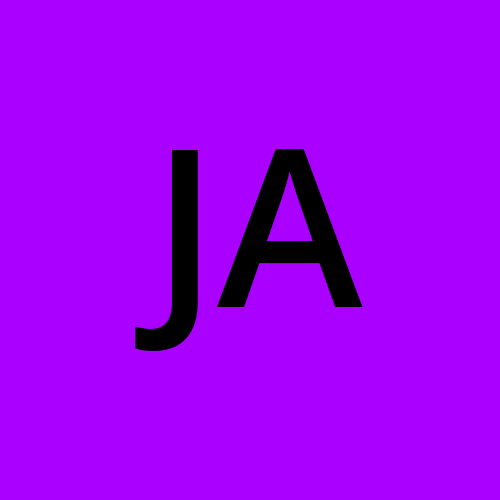

Deploying a .NET application to a cloud environment like DigitalOcean is a powerful way to ensure scalability, reliability, and ease of management. In this post, we’ll walk through deploying a real-world .NET application—a simple ASP.NET Core MVC app—to DigitalOcean using Docker containers and its container registry.
Prerequisites
Before we begin, ensure you have the following:
DigitalOcean Account: Sign up at DigitalOcean.
Docker Installed: Ensure Docker is installed and running on your local machine. Download it here.
dotnet CLI: Install the .NET SDK from dotnet.microsoft.com.
DigitalOcean CLI (doctl): Install
doctl
for managing DigitalOcean resources from your terminal. Follow the installation guide.A Sample .NET Project: Clone the sample app:
git clone https://github.com/dotnet-architecture/eShopOnWeb.git cd eShopOnWeb
Step 1: Build and Containerize the .NET Application
1.1 Create a Dockerfile
Navigate to the root directory of the sample app and create a Dockerfile
:
# Use the official ASP.NET runtime image
FROM mcr.microsoft.com/dotnet/aspnet:6.0 AS base
WORKDIR /app
EXPOSE 5000
# Use the .NET SDK image for building the app
FROM mcr.microsoft.com/dotnet/sdk:6.0 AS build
WORKDIR /src
COPY ["src/Web/Web.csproj", "src/Web/"]
RUN dotnet restore "src/Web/Web.csproj"
COPY . .
WORKDIR "/src/src/Web"
RUN dotnet build "Web.csproj" -c Release -o /app/build
# Publish the app
FROM build AS publish
RUN dotnet publish "Web.csproj" -c Release -o /app/publish /p:UseAppHost=false
# Create the final runtime image
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "Web.dll"]
1.2 Build the Docker Image
Build the Docker image for your app:
docker build -t eshoponweb:latest .
1.3 Test Locally
Run the container locally to ensure it works:
docker run -p 5000:5000 eshoponweb:latest
Visit http://localhost:5000
in your browser to verify the app is running.
Step 2: Push the Image to DigitalOcean Container Registry
2.1 Authenticate with DigitalOcean
Log in to your DigitalOcean account using the doctl
CLI:
doctl auth init
2.2 Create a Container Registry
Create a new container registry in DigitalOcean:
doctl registry create my-registry
Retrieve the registry URL:
doctl registry get
Example output:
Name: my-registry
Endpoint: registry.digitalocean.com/my-registry
2.3 Tag and Push the Docker Image
Tag your image for the DigitalOcean registry:
docker tag eshoponweb:latest registry.digitalocean.com/my-registry/eshoponweb:latest
Push the image:
docker push registry.digitalocean.com/my-registry/eshoponweb:latest
Verify the image was pushed successfully:
doctl registry repository list my-registry
Step 3: Deploy the App to a DigitalOcean Droplet
3.1 Create a Droplet
Spin up a new DigitalOcean droplet using the Docker image:
doctl compute droplet create \
my-droplet \
--region nyc3 \
--image docker-20-04 \
--size s-1vcpu-1gb \
--ssh-keys <your-ssh-key-id>
Retrieve the droplet’s IP address:
doctl compute droplet list
3.2 SSH Into the Droplet
SSH into the newly created droplet:
ssh root@<droplet-ip>
3.3 Pull and Run the Docker Container
Log in to the DigitalOcean registry from your droplet:
docker login registry.digitalocean.com
Enter your DigitalOcean personal access token when prompted.
Pull the container image:
docker pull registry.digitalocean.com/my-registry/eshoponweb:latest
Run the container:
docker run -d -p 80:5000 registry.digitalocean.com/my-registry/eshoponweb:latest
The app will now be accessible at http://<droplet-ip>
.
Step 4: Automate Deployment with Docker Compose (Optional)
To manage your app and any dependencies, use a docker-compose.yml
file:
version: '3.8'
services:
eshoponweb:
image: registry.digitalocean.com/my-registry/eshoponweb:latest
ports:
- "80:5000"
environment:
- DOTNET_ENVIRONMENT=Production
Deploy with Docker Compose:
docker-compose up -d
Conclusion
In this tutorial, we containerized a .NET app, pushed it to DigitalOcean’s container registry, and deployed it on a droplet. This setup ensures your app is production-ready and easily scalable. For advanced setups, consider adding CI/CD pipelines and monitoring tools.
Happy deploying!
Subscribe to my newsletter
Read articles from Joshua Akosa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
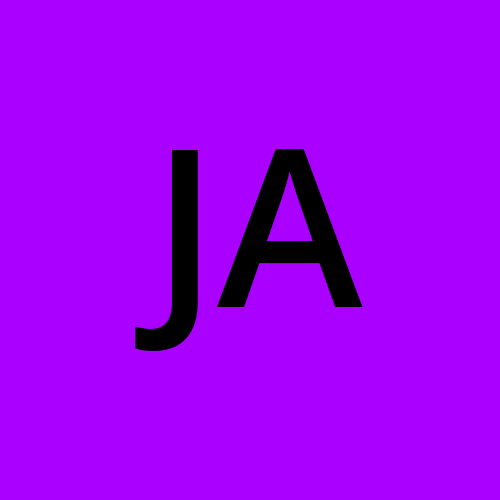