A Beginner's Guide to WebRTC: Demystifying NAT, STUN, and TURN (Part 1)
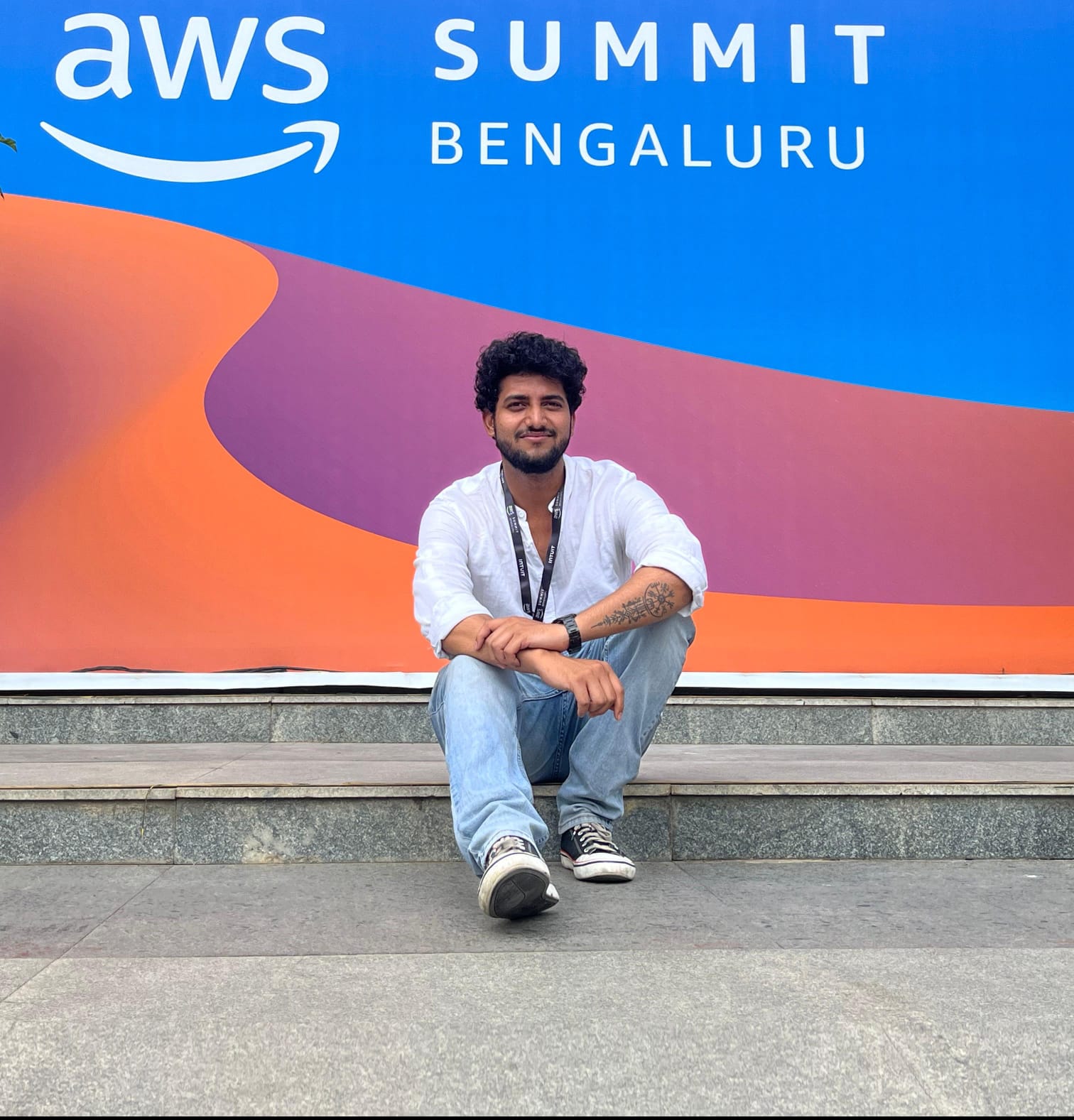
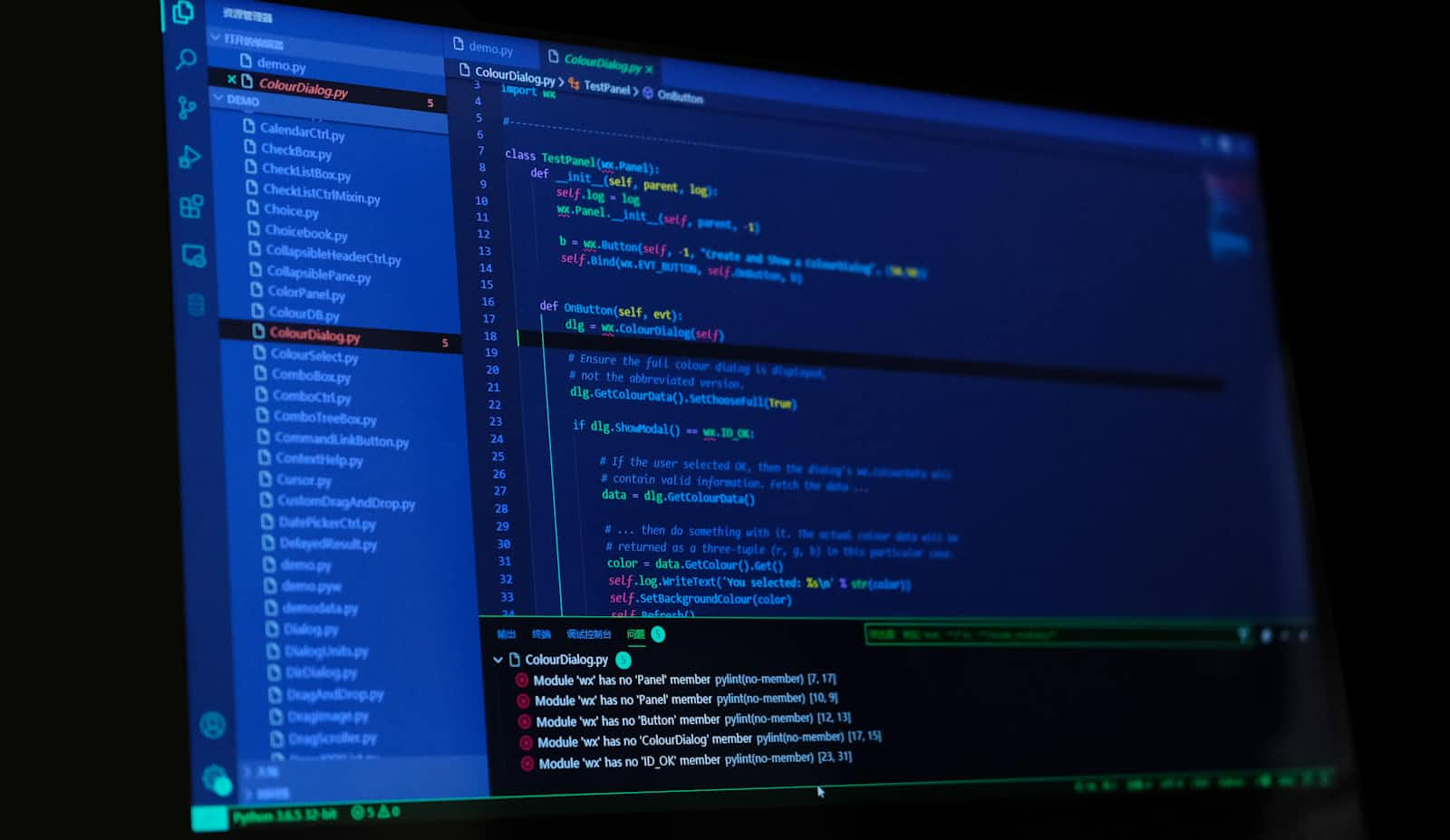
Welcome to our WebRTC learning series! In this first article, we'll explore the networking magic that makes peer-to-peer communication possible in web browsers. By understanding concepts like NAT traversal and the role of STUN/TURN servers, you'll build a solid foundation for creating real-time applications.
Series: Part 1 of 4
Required Knowledge: Basic web development, networking fundamentals
Introduction to WebRTC
WebRTC (Web Real-Time Communication) revolutionizes how we think about browser-based communication. When you make a video call or share your screen in apps like Google Meet or Discord, you're using WebRTC. But have you ever wondered how your browser finds and connects to other users across different networks?
In this article, we'll focus on the networking foundations of WebRTC. While WebRTC offers many features like media streaming and data channels, understanding its networking layer is crucial for building reliable real-time applications.
The Challenge of Peer-to-Peer Communication
Imagine trying to send a letter to someone, but instead of using their home address, you only have their apartment number. This is similar to the challenge WebRTC faces: most computers are hidden behind private networks, making direct communication difficult.
Private Networks and NAT
When you connect to the internet from home or office, your device typically gets a private IP address (like 192.168.1.100). This address only works within your local network - you can't use it to communicate with the outside world directly. Instead, your router uses Network Address Translation (NAT) to help your device communicate with the internet.
Let's understand NAT with a real-world analogy: think of a large office building.
The building has one street address (public IP) that everyone can find
Inside, each office has its own room number (private IP)
The reception desk (NAT) maintains a record of which room number corresponds to which employee
When mail arrives, the reception routes it to the correct room
Types of NAT Behavior
Not all NATs work the same way. Understanding these differences is crucial for WebRTC:
Full Cone NAT
Most permissive type
Once an internal device sends data out, it can receive data from any external address
Like a receptionist who accepts any mail for you once you've registered
Restricted Cone NAT
More selective
Only accepts data from addresses you've previously contacted
Like a receptionist who only accepts mail from addresses where you've sent mail before
Port Restricted Cone NAT
Even more selective
Checks both the sender's address and specific port
Like a receptionist who checks both the sender's address and department
Symmetric NAT
Most restrictive
Creates a new mapping for each connection
Like having a different return address for each person you communicate with
Here's a simple code example to detect your NAT type:
class NATTypeDetector {
constructor() {
this.peerConnection = new RTCPeerConnection({
iceServers: [{ urls: 'stun:stun.l.google.com:19302' }]
});
}
async detectNATType() {
const candidates = [];
return new Promise((resolve) => {
this.peerConnection.onicecandidate = (event) => {
if (event.candidate) {
candidates.push(event.candidate);
} else {
// ICE gathering complete
resolve(this.analyzeNATFromCandidates(candidates));
}
};
// Trigger ICE gathering
this.peerConnection.createDataChannel('nat_detection');
this.peerConnection.createOffer()
.then(offer => this.peerConnection.setLocalDescription(offer));
});
}
analyzeNATFromCandidates(candidates) {
const srflxCandidates = candidates.filter(c => c.type === 'srflx');
if (srflxCandidates.length === 0) return 'No NAT detected';
// Further analysis to determine NAT type...
return srflxCandidates.length > 1 ? 'Symmetric NAT' : 'Cone NAT';
}
}
STUN Servers: Your Internet Address Finder
STUN (Session Traversal Utilities for NAT) servers help devices discover their public IP address and port. This is essential for establishing peer-to-peer connections.
How STUN Works
Your device sends a request to the STUN server
The server sees your public IP address and port
The server sends this information back to you
You can now share this public address with peers
Here's how to use a STUN server in WebRTC:
const configuration = {
iceServers: [
{
urls: [
'stun:stun1.l.google.com:19302',
'stun:stun2.l.google.com:19302'
]
}
]
};
const peerConnection = new RTCPeerConnection(configuration);
// Monitor ICE candidates
peerConnection.onicecandidate = (event) => {
if (event.candidate) {
console.log('Got ICE candidate:', event.candidate.type);
if (event.candidate.type === 'srflx') {
console.log('Public IP:', event.candidate.address);
console.log('Public Port:', event.candidate.port);
}
}
};
Setting Up Your Own STUN Server
While you can use public STUN servers, setting up your own gives you more control. Here's how to set up a STUN server using coturn
:
# Install coturn
sudo apt-get update
sudo apt-get install coturn
# Configure STUN
sudo nano /etc/turnserver.conf
# Add basic configuration
listening-port=3478
tls-listening-port=5349
listening-ip=YOUR_SERVER_IP
min-port=49152
max-port=65535
# Start the service
sudo systemctl start coturn
sudo systemctl enable coturn
TURN Servers: The Reliable Fallback
Sometimes, direct peer-to-peer communication isn't possible, usually due to restrictive firewalls or symmetric NATs. This is where TURN (Traversal Using Relays around NAT) servers come in.
How TURN Works
A TURN server acts as a relay between peers:
Instead of connecting directly, peers connect to the TURN server
The TURN server forwards data between peers
This ensures connectivity but increases latency
Here's how to use TURN in WebRTC:
class TURNConnection {
constructor(username, credential) {
this.configuration = {
iceServers: [{
urls: [
'turn:your.turn.server:3478',
'turn:your.turn.server:3478?transport=tcp'
],
username: username,
credential: credential
}],
iceTransportPolicy: 'relay' // Force TURN usage
};
}
async createConnection() {
const pc = new RTCPeerConnection(this.configuration);
pc.onicecandidate = (event) => {
if (event.candidate && event.candidate.type === 'relay') {
console.log('Using TURN server for:', event.candidate.address);
}
};
return pc;
}
}
Setting Up a TURN Server
Here's a production-ready TURN server configuration:
# Install coturn with SSL support
sudo apt-get install coturn openssl
# Generate SSL certificates
sudo openssl req -x509 -newkey rsa:2048 -keyout /etc/turn_server_pkey.pem \
-out /etc/turn_server_cert.pem -days 99999 -nodes
# Configure TURN
sudo nano /etc/turnserver.conf
# Add comprehensive configuration
listening-port=3478
tls-listening-port=5349
listening-ip=YOUR_SERVER_IP
external-ip=YOUR_PUBLIC_IP
realm=your.domain.com
# Authentication
lt-cred-mech
user=turnuser:turnpass
# SSL
cert=/etc/turn_server_cert.pem
pkey=/etc/turn_server_pkey.pem
# Performance
min-port=49152
max-port=65535
max-bps=0
no-multicast-peers
Putting It All Together
Let's create a complete WebRTC connection manager that handles NAT traversal:
class WebRTCConnectionManager {
constructor(config) {
this.config = {
iceServers: [
{ urls: 'stun:stun.l.google.com:19302' },
{
urls: config.turnServer,
username: config.turnUsername,
credential: config.turnPassword
}
],
iceTransportPolicy: config.forceTurn ? 'relay' : 'all'
};
}
async createConnection() {
const pc = new RTCPeerConnection(this.config);
// Monitor connection states
pc.oniceconnectionstatechange = () => {
console.log('ICE Connection State:', pc.iceConnectionState);
if (pc.iceConnectionState === 'failed') {
this.handleConnectionFailure(pc);
}
};
// Monitor ICE gathering
pc.onicegatheringstatechange = () => {
console.log('ICE Gathering State:', pc.iceGatheringState);
};
// Log ICE candidates
pc.onicecandidate = (event) => {
if (event.candidate) {
console.log('New candidate:', {
type: event.candidate.type,
protocol: event.candidate.protocol,
address: event.candidate.address
});
}
};
return pc;
}
async handleConnectionFailure(pc) {
console.log('Analyzing connection failure...');
const stats = await pc.getStats();
let failureReason;
stats.forEach(report => {
if (report.type === 'candidate-pair' && report.state === 'failed') {
failureReason = report.failureReason || 'Unknown';
console.log(`Connection failed: ${failureReason}`);
}
});
// Implement recovery strategy
if (failureReason) {
this.attemptRecovery(pc, failureReason);
}
}
async attemptRecovery(pc, reason) {
// Implement your recovery strategy
// For example: Force TURN, restart ICE, or create new connection
}
}
Best Practices and Common Pitfalls
When implementing WebRTC's networking layer, keep these points in mind:
Always use both STUN and TURN servers
STUN servers are cheap but not always sufficient
TURN servers are expensive but essential for reliability
Handle ICE failures gracefully
Monitor ICE connection states
Implement reconnection logic
Consider falling back to TURN when direct connection fails
Geographic Distribution
Deploy TURN servers in multiple regions
Use DNS-based load balancing
Consider latency when selecting servers
Security Considerations
Always use authentication for TURN servers
Keep credentials secure
Monitor for abuse
Implement rate limiting
What's Coming in Part 2
In the next article, we'll build on this networking foundation to create a complete video chat application. We'll cover:
Setting up a signaling server
Handling media streams
Managing peer connections
Implementing real-time data channels
Additional Resources
This article is part of our WebRTC series. Stay tuned for Part 2, where we'll start building a real video chat application using these concepts!
Subscribe to my newsletter
Read articles from Brijesh Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
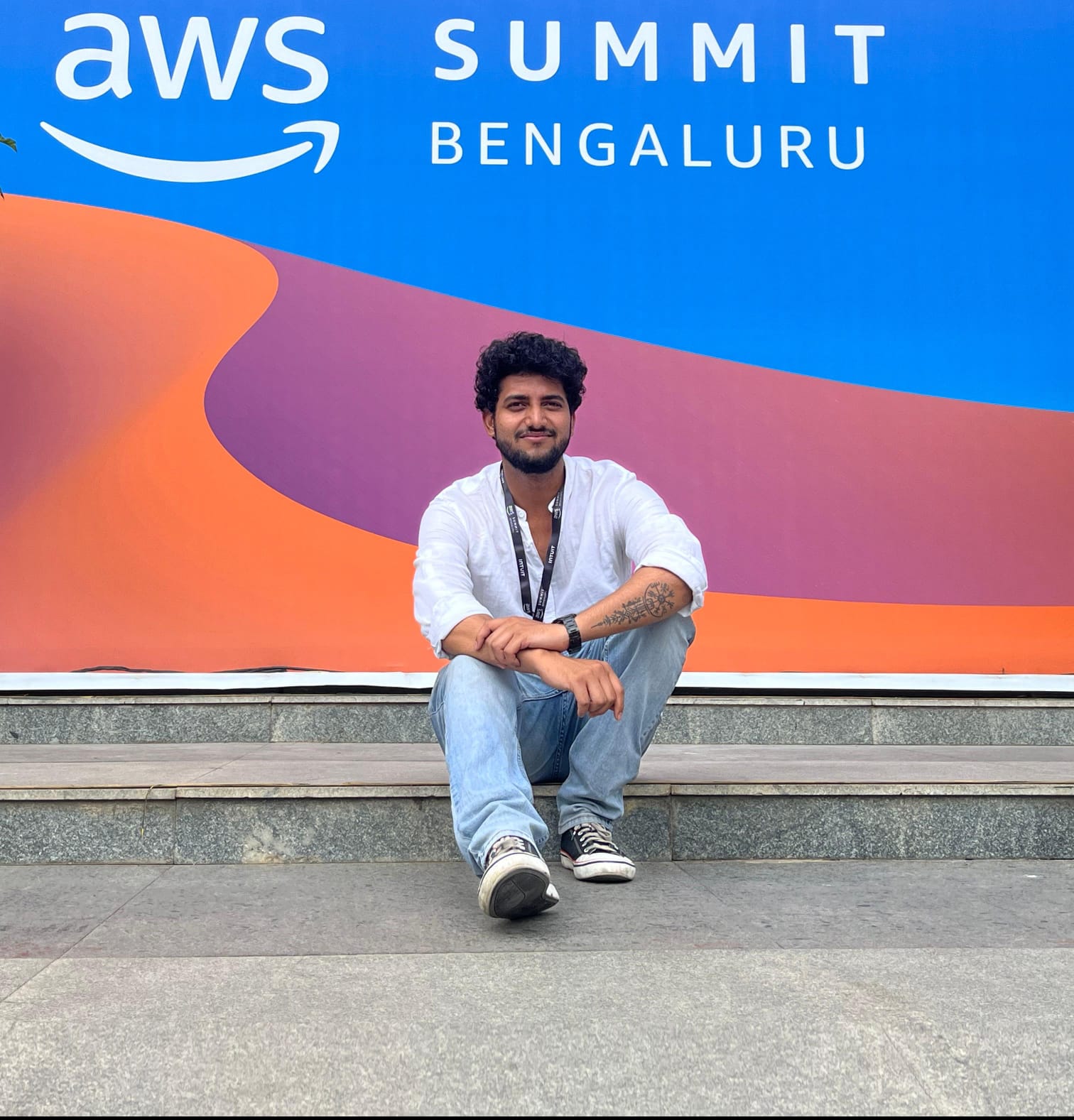
Brijesh Pandey
Brijesh Pandey
I'm a passionate software engineer with a strong background in web development, including both frontend and backend technologies. I'm particularly excited about the potential of Web 3.0 and enjoy working on cutting-edge projects in this space. Beyond coding, I'm an adventurous individual who loves to travel and explore new places, cultures, and cuisines. I'm also athletic and enjoy playing football, cricket, and badminton. I'm always up for a challenge and believe in trying new things!