Building Your Own Weather App with Node.js and Express
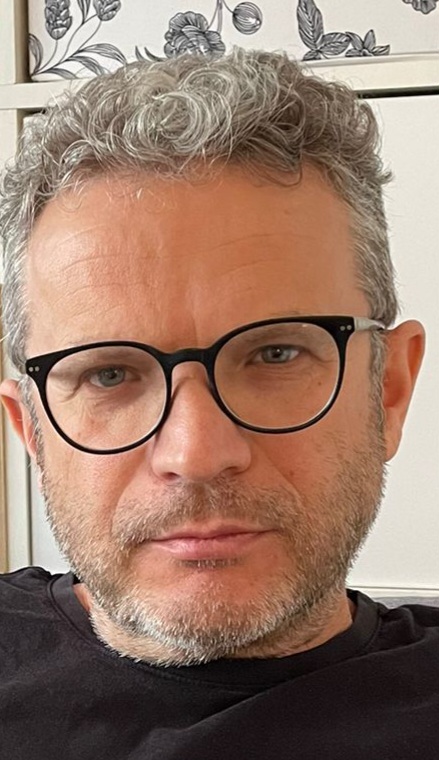
APIs (Application Programming Interfaces) are the backbone of modern applications. From social media to e-commerce to weather apps, APIs play a vital role in connecting services. In this article, we’ll build a simple weather app using Node.js and Express that fetches data from a weather API. This project is beginner-friendly and demonstrates how to integrate and utilize APIs effectively.
Project Overview
Goal:
Create a weather app that takes a city as input and returns the current weather data using the OpenWeatherMap API.
Prerequisites:
Basic knowledge of JavaScript
Understanding of Node.js and Express basics
Familiarity with APIs
Technologies:
Node.js
Express
OpenWeatherMap API
HTML/CSS for the user interface
1. Setting Up the Project
1.1 Install Node.js and npm
Ensure that Node.js and npm are installed on your system. Verify installation with:
node -v
npm -v
1.2 Initialize a New Project
Create a folder for your project and initialize it:
mkdir weather-app
cd weather-app
npm init -y
1.3 Install Dependencies
Install Express and Axios:
npm install express axios
2. Creating the Server Code
2.1 Main File Setup
Create a file named app.js
and add the following code:
const express = require('express');
const axios = require('axios');
const app = express();
// OpenWeatherMap API Key
const API_KEY = 'your_api_key_here';
// Middleware to serve static files
app.use(express.static('public'));
// Route for the homepage
app.get('/', (req, res) => {
res.sendFile(__dirname + '/public/index.html');
});
// Route for fetching weather data
app.get('/weather', async (req, res) => {
const city = req.query.city;
if (!city) {
return res.status(400).send('Please provide a city.');
}
try {
const response = await axios.get(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${API_KEY}&units=metric`);
res.json(response.data);
} catch (error) {
res.status(500).send('Error fetching weather data.');
}
});
// Start the server
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running at http://localhost:${PORT}`);
});
3. Creating the User Interface
3.1 HTML File
Create a file named index.html
in the public
folder with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather App</title>
</head>
<body>
<h1>Weather App</h1>
<form id="weather-form">
<input type="text" id="city" placeholder="Enter city" required>
<button type="submit">Get Weather</button>
</form>
<div id="weather-result"></div>
<script>
const form = document.getElementById('weather-form');
const resultDiv = document.getElementById('weather-result');
form.addEventListener('submit', async (event) => {
event.preventDefault();
const city = document.getElementById('city').value;
try {
const response = await fetch(`/weather?city=${city}`);
if (!response.ok) throw new Error('Error fetching data.');
const data = await response.json();
resultDiv.innerHTML = `
<h2>Weather in ${data.name}</h2>
<p>Temperature: ${data.main.temp}°C</p>
<p>Description: ${data.weather[0].description}</p>
`;
} catch (error) {
resultDiv.innerHTML = `<p>${error.message}</p>`;
}
});
</script>
</body>
</html>
4. Testing the App
Start the server:
node app.js
Open
http://localhost:3000
in your browser.Enter a city name and view the weather data.
Extensions
Styling: Add CSS to enhance the appearance of the app.
Error Handling: Improve error messages for the user.
Additional Features: Show a multi-day weather forecast or include more weather details.
Through this project, you’ve learned the basics of API integration and how to use Node.js and Express to build a simple web app. This is a great starting point for exploring more advanced web development concepts.
Subscribe to my newsletter
Read articles from Milaim Delija directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
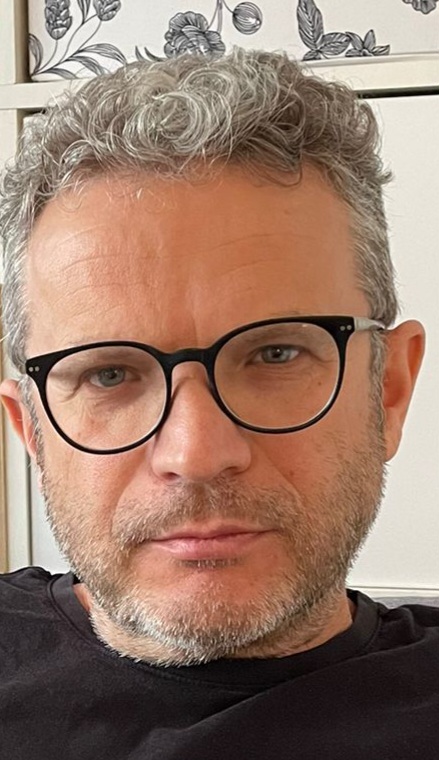