Creating Your First 3D Model Using Three.js: Spinning Sphere
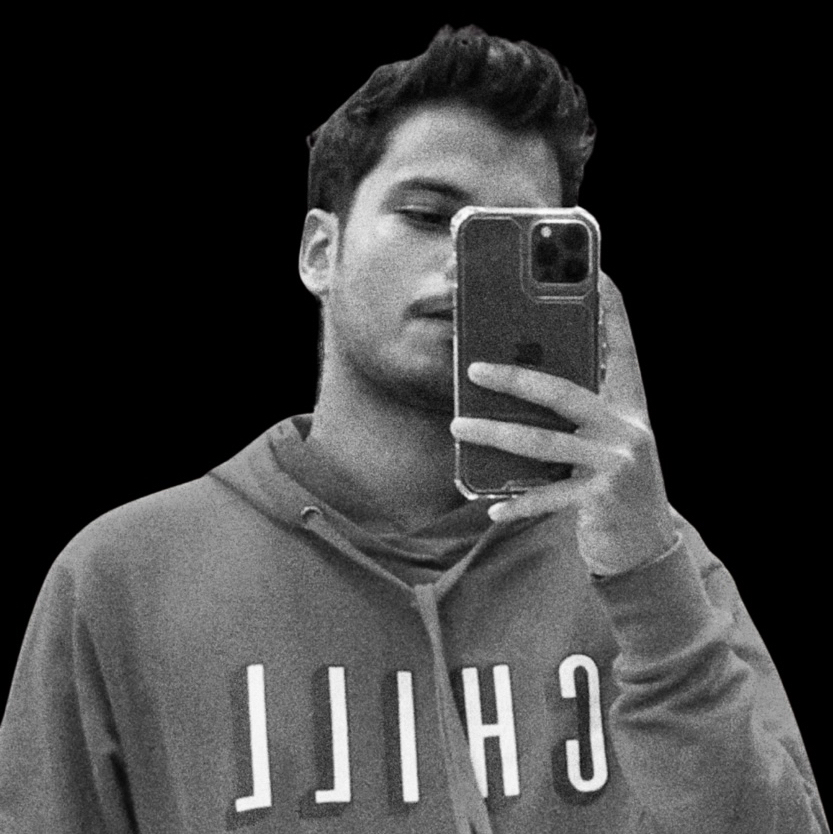
Three.js is a powerful JavaScript library that allows you to render 3D objects in a browser. It's perfect for creating interactive 3D scenes without requiring heavy desktop applications. In this blog, I'll walk you through the process of creating a simple spinning sphere using Three.js, and how you can customize colors, size, and textures.
Step 1: Setting Up Your Project
First, make sure you have Three.js installed in your project. If you're using a package manager like npm or yarn, you can install it like this:
npm install three
Alternatively, you can directly include it in your HTML using a CDN:
<script src="https://cdn.jsdelivr.net/npm/three@0.132.2/build/three.min.js"></script>
Step 2: Setting Up the Basic Scene
Now, let’s create a basic 3D scene where we’ll place our spinning sphere.
import * as THREE from 'three';
// Scene
const scene = new THREE.Scene();
// Camera
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
// Renderer
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
Here, we’ve set up a scene, camera, and renderer. The camera has a z
position of 5 to ensure it's not too close to the sphere.
Step 3: Creating the Sphere
Next, let’s create a sphere geometry and a basic material for it.
// Sphere Geometry and Material
const geometry = new THREE.SphereGeometry(1, 32, 32);
const material = new THREE.MeshBasicMaterial({ color: 0x0077ff }); // Customize color here
const sphere = new THREE.Mesh(geometry, material);
scene.add(sphere);
The SphereGeometry
constructor defines the size of the sphere. You can customize the radius and segments (32 for both width and height in this example). The MeshBasicMaterial
is a simple material that doesn't respond to lighting, and we’ve set its color to blue (0x0077ff
).
Step 4: Adding Animation to Make the Sphere Spin
To make the sphere spin, we will use the animate
function to rotate it continuously:
function animate() {
requestAnimationFrame(animate);
// Spin the sphere
sphere.rotation.x += 0.01;
sphere.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
We’re continuously updating the sphere’s rotation on both the X and Y axes to make it spin.
Step 5: Customizing the Model
Size: To change the size of the sphere, simply modify the radius value in the
SphereGeometry
constructor. For example, usenew THREE.SphereGeometry(2, 32, 32)
to create a sphere twice the size.Color: The color of the sphere can be customized by changing the
color
property in the material. For instance, usenew THREE.MeshBasicMaterial({ color: 0xff0000 })
for a red sphere.Texture: You can apply a texture to the sphere using a
TextureLoader
. Here's how to add a texture:
const textureLoader = new THREE.TextureLoader();
textureLoader.load('path-to-texture.jpg', function(texture) {
material.map = texture;
material.needsUpdate = true;
});
This code loads a texture image and applies it to the sphere. Make sure the path to your texture file is correct.
- Lighting: Since
MeshBasicMaterial
doesn’t react to lighting, you can switch to materials likeMeshLambertMaterial
orMeshPhongMaterial
if you want your object to be affected by lights. Here’s an example:
const material = new THREE.MeshLambertMaterial({ color: 0x0077ff });
And you’ll need to add a light source:
const light = new THREE.PointLight(0xffffff, 1, 100);
light.position.set(10, 10, 10);
scene.add(light);
Step 6: Rendering the Scene
Finally, call the render
method to display the scene with the camera:
renderer.render(scene, camera);
Final Code Example:
Here’s the full code for creating a spinning sphere with Three.js:
import * as THREE from 'three';
// Scene Setup
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
// Renderer
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// Sphere Geometry and Material
const geometry = new THREE.SphereGeometry(1, 32, 32);
const material = new THREE.MeshLambertMaterial({ color: 0x0077ff });
const sphere = new THREE.Mesh(geometry, material);
scene.add(sphere);
// Lighting
const light = new THREE.PointLight(0xffffff, 1, 100);
light.position.set(10, 10, 10);
scene.add(light);
// Animation Loop
function animate() {
requestAnimationFrame(animate);
sphere.rotation.x += 0.01;
sphere.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
Conclusion
With these steps, you’ve created your first 3D model—a spinning sphere—using Three.js! You can now customize the size, color, texture, and lighting to create more complex 3D scenes. Explore Three.js further to create intricate 3D visuals and animations for your projects.
Subscribe to my newsletter
Read articles from Athulkrishna TN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
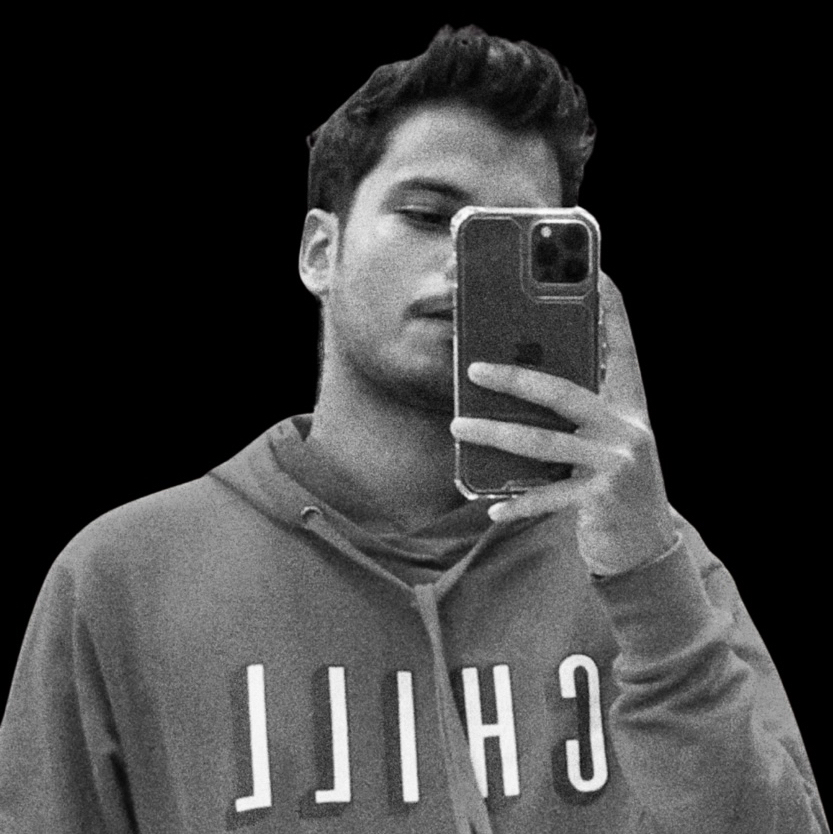