Getting Started with Java - 01

Table of contents
- What is a Programming Language?
- How a Java Program Works: JVM, JRE, and JDK Explained
- Setting Up IntelliJ IDEA for Java Development
- Creating Your First Java Class
- Writing Your First Java Program
- Run Your Program
- Understanding the Key Concepts
- Adding Some Fun: A Simple Calculator
- Conclusion
- Bonus: Helpful Resources
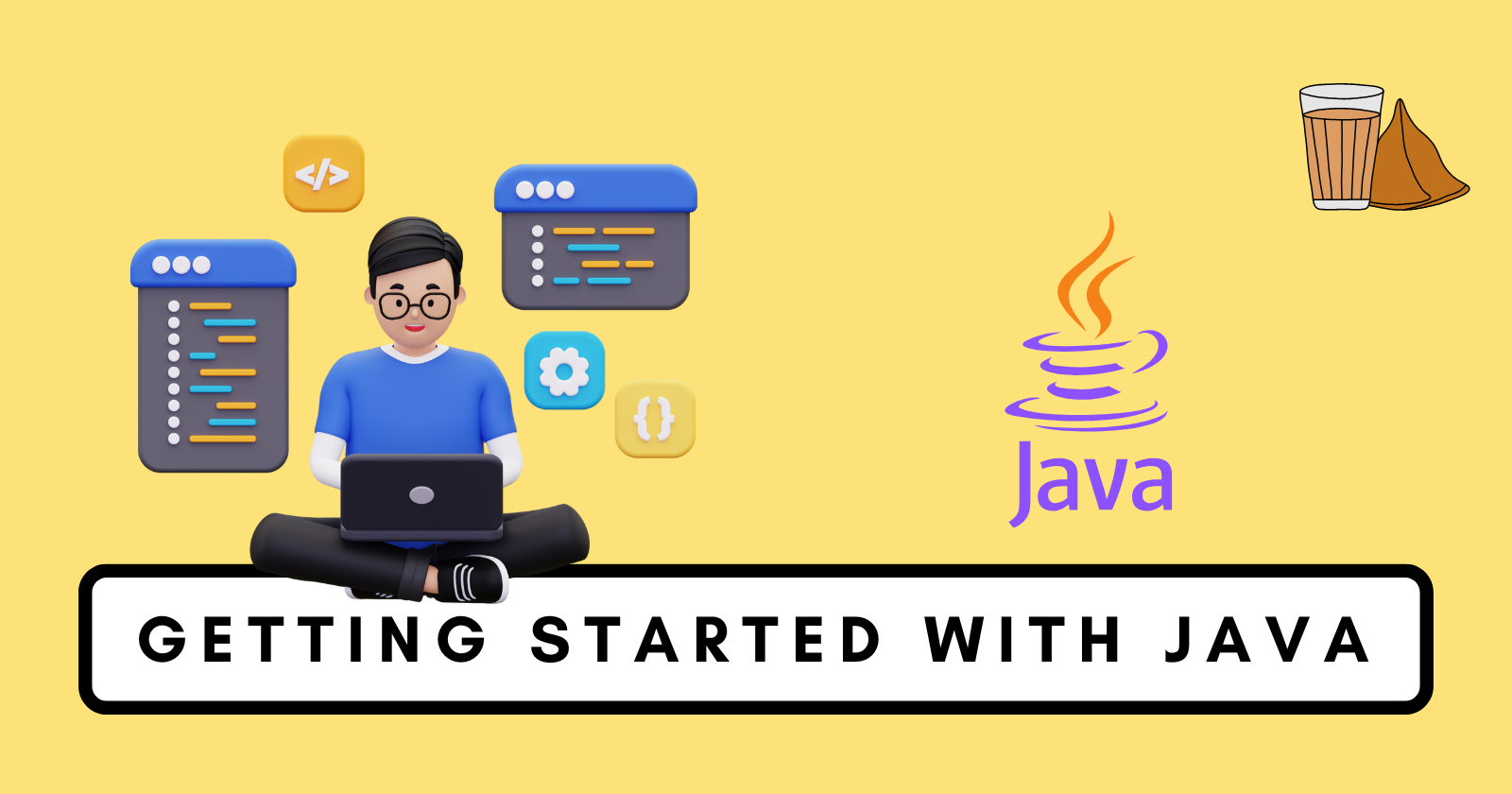
What is a Programming Language?
In simple terms, a programming language is a set of instructions that tells a computer what to do. Think of it like a recipe that guides a chef (the computer) to prepare a dish. Programming languages allow us to interact with computers to create software, websites, games, and more. They serve as the bridge between humans and machines.
Java is one such programming language, widely used for building everything from mobile apps (think Android!) to large-scale enterprise systems. What makes Java special? It’s simple, object-oriented, platform-independent, and secure. Plus, it’s been around since the 1990s, so it’s trusted and well-established.
How a Java Program Works: JVM, JRE, and JDK Explained
Before you dive into writing code, it’s important to understand how Java works behind the scenes. At the core of Java are three key components: JVM (Java Virtual Machine), JRE (Java Runtime Environment), and JDK (Java Development Kit).
1. JVM (Java Virtual Machine)
The JVM is like a translator that helps your code run on any platform. When you write a Java program, it gets compiled into bytecode (a special intermediate format). The JVM then takes this bytecode and translates it into machine code that your operating system can understand.
- Key Point: Write once, run anywhere! Your Java code can run on any system with a JVM installed.
2. JRE (Java Runtime Environment)
The JRE is what you need to run Java programs. It includes:
The JVM.
Essential libraries and files needed for execution.
If you’re only running Java applications (not developing them), the JRE is all you need.
3. JDK (Java Development Kit)
The JDK is a complete package for developers. It includes:
The JRE.
Tools for compiling and debugging code (e.g.,
javac
, the Java compiler).
Fun Fact: If JRE is a bicycle for riding, JDK is a full workshop where you can build, fix, and customize bicycles.
How They Work Together
You write Java code (
.java
file).The JDK compiles it into bytecode (
.class
file).The JVM reads the bytecode and runs it.
Setting Up IntelliJ IDEA for Java Development
Step 1: Download and Install JDK
Head to the Oracle JDK download page.
Choose the version compatible with your system and install it.
Step 2: Download IntelliJ IDEA
Visit the IntelliJ IDEA download page.
Download the Community Edition (it’s free!).
Step 3: Set Up IntelliJ IDEA
Launch IntelliJ IDEA and select New Project.
Choose Java as the project type and select the JDK you installed earlier.
Name your project and click Finish.
✨ Pro Tip: Use IntelliJ’s auto-complete feature—it’s like having a coding assistant on steroids!
Creating Your First Java Class
A class is the blueprint for creating objects in Java. Think of it like a cookie-cutter: the class defines the shape, and objects are the cookies you make from it.
Here’s how to create a class in IntelliJ IDEA:
Right-click on the
src
folder in your project.Select New > Java Class.
Name your class (e.g.,
HelloWorld
) and click OK.
Your class will look like this:
public class HelloWorld {
// This is a class
}
Writing Your First Java Program
Now, let’s write a simple Java program that prints “Hello, World!” to the console. This is often the first program beginners learn—it’s like a rite of passage in coding!
- Inside your
HelloWorld
class, add amain
method. Themain
method is where the program starts execution.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Breaking It Down
public class HelloWorld
: This defines a class namedHelloWorld
.public static void main(String[] args)
: This is the entry point of the program.System.out.println("Hello, World!");
: This prints the text “Hello, World!” to the console.
Run Your Program
Click the green Run button in IntelliJ.
You’ll see the output in the console:
Hello, World!
🎉 Congratulations! You’ve written your first Java program.
Understanding the Key Concepts
1. Variables
Variables store data. For example:
int age = 25; // An integer variable
String name = "Alice"; // A string variable
- 🛠️ Analogy: Think of variables as labeled jars where you can store different types of items.
2. Data Types
Java has different data types:
int
: For integers (e.g., 10, 200).double
: For decimal numbers (e.g., 3.14, 9.99).String
: For text (e.g., "Hello").boolean
: For true/false values.
3. Conditional Statements
Conditions allow your program to make decisions.
Example:
int age = 18;
if (age >= 18) {
System.out.println("You are an adult.");
} else {
System.out.println("You are a minor.");
}
4. Loops
Loops help you repeat actions. For example, printing numbers 1 to 5:
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
Adding Some Fun: A Simple Calculator
Let’s create a program that adds two numbers:
import java.util.Scanner;
public class Calculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter first number: ");
int num1 = scanner.nextInt();
System.out.print("Enter second number: ");
int num2 = scanner.nextInt();
int sum = num1 + num2;
System.out.println("The sum is: " + sum);
}
}
Conclusion
Congratulations! You’ve taken your first steps into the world of Java programming. Here’s what you’ve learned:
What a programming language is.
How Java works with JVM, JRE, and JDK.
Setting up IntelliJ IDEA for development.
Writing and running your first Java program.
Basic concepts like variables, data types, conditionals, and loops.
What’s Next? From here, you can explore object-oriented programming (OOP), file handling, and building more advanced applications. Java’s versatility makes it an amazing language to learn, whether you’re a beginner or an experienced programmer.
Bonus: Helpful Resources
Keep coding, and enjoy the journey! 🚀
Subscribe to my newsletter
Read articles from Ajink Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ajink Gupta
Ajink Gupta
I am an engineering student pursuing a degree in Artificial Intelligence and Data Science at Datta Meghe College of Engineering. I have strong technical skills in Full Stack Web Development, as well as programming in Python and Java. I currently manage Doubtly's blog and am exploring job opportunities as an SDE. I am passionate about learning new technologies and contributing to the tech community.