Floyd's Cycle-Finding Algorithm (Tortoise and Hare) – Beginner-Friendly Guide
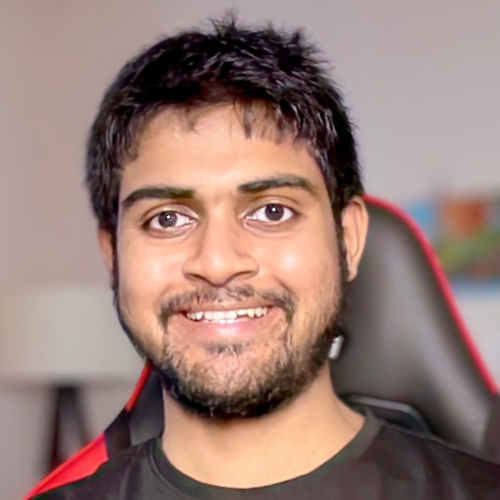
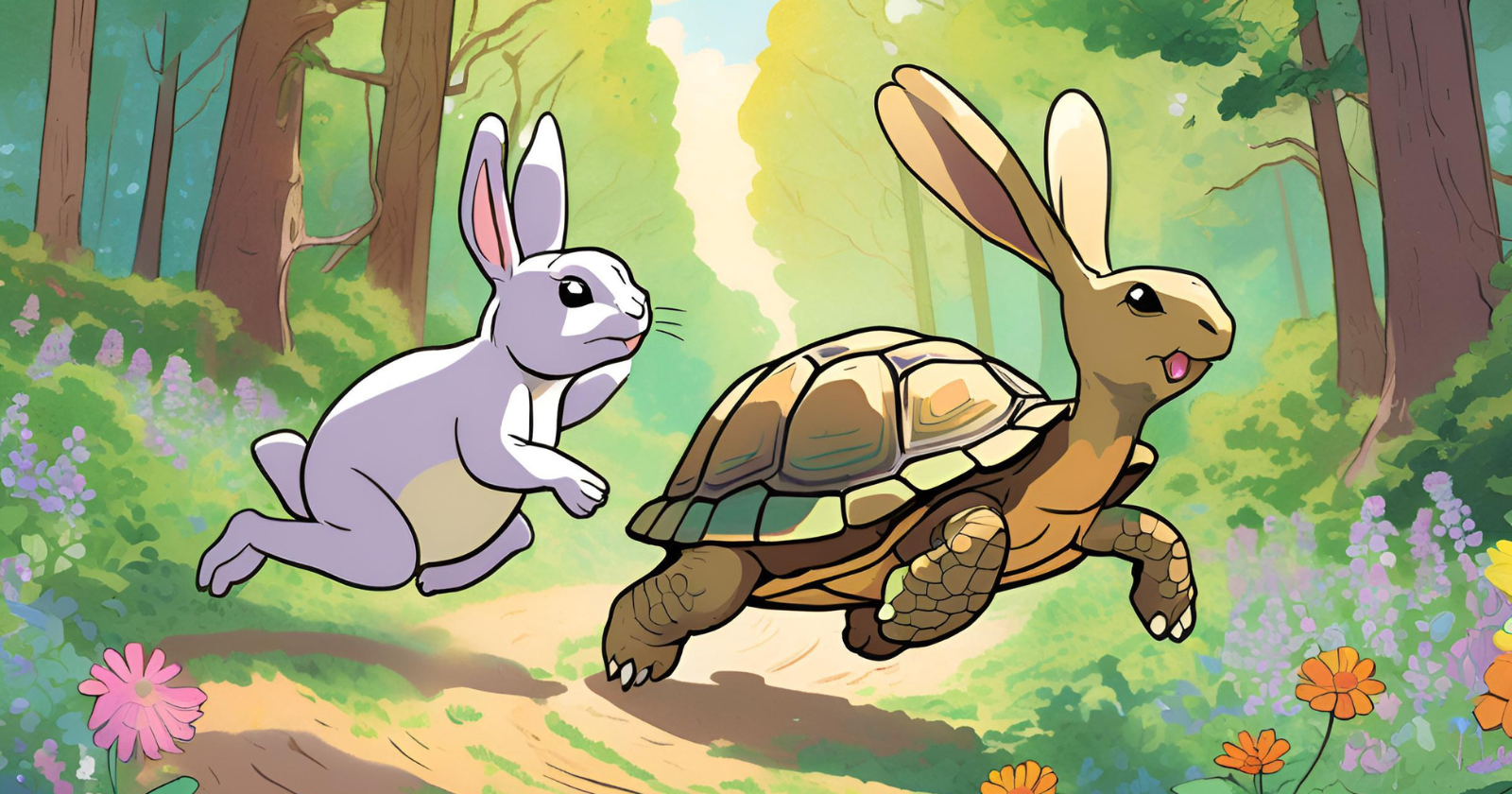
Floyd's Cycle-Finding Algorithm (Tortoise and Hare) – Beginner-Friendly Guide
Floyd’s Cycle-Finding Algorithm, commonly called the Tortoise and the Hare Algorithm, is a clever way to detect cycles in a linked list without using extra space. It uses two pointers:
A slow pointer (tortoise) that moves one step at a time
A fast pointer (hare) that moves two steps at a time
If there is a cycle in the linked list, the fast pointer will eventually meet the slow pointer. If there is no cycle, the fast pointer will reach the end of the list.
Real-Life Applications of Floyd’s Cycle-Finding Algorithm
While this algorithm is popular in competitive programming (LeetCode, Codeforces, etc.), it is also used in real-world applications by big tech companies like Google, Microsoft, and Meta for:
Memory Management & Garbage Collection:
Some programming languages (like Java and Python) use this algorithm to detect memory leaks.
If an object references itself (a cycle), it can lead to memory not being freed, causing inefficiency.
Networking (Detecting Loops in Routing Algorithms):
Network protocols like OSPF (Open Shortest Path First) need to check if a packet is stuck in an infinite loop.
This algorithm helps detect cycles in the network topology.
Blockchain and Cryptocurrency Transactions:
In blockchain networks, loops in transactions can cause double spending or deadlocks.
Detecting cycles can prevent fraudulent transactions.
Version Control Systems (Git):
- When merging branches, Git ensures no infinite loops are created in the commit history.
Database Deadlock Detection:
In databases, transactions might wait on each other, forming a cycle (deadlock).
Floyd’s Algorithm helps detect such cases.
Step-by-Step Explanation with an Example
1. Detecting a Cycle in a Linked List
💡 Example: Imagine a race track where a tortoise (slow pointer) and a hare (fast pointer) are running.
The hare runs twice as fast as the tortoise.
If the track is circular, at some point, the hare will lap the tortoise (meaning they meet).
If the track is straight (no cycle), the hare will reach the end without ever meeting the tortoise.
Let’s implement this in JavaScript:
class ListNode {
constructor(value) {
this.value = value;
this.next = null;
}
}
function hasCycle(head) {
let slow = head;
let fast = head;
while (fast !== null && fast.next !== null) {
slow = slow.next; // Moves one step
fast = fast.next.next; // Moves two steps
if (slow === fast) {
return true; // Cycle detected
}
}
return false; // No cycle
}
// Example Usage
let node1 = new ListNode(1);
let node2 = new ListNode(2);
let node3 = new ListNode(3);
let node4 = new ListNode(4);
node1.next = node2;
node2.next = node3;
node3.next = node4;
node4.next = node2; // Creating a cycle
console.log(hasCycle(node1)); // Output: true
2. Finding the Start of the Cycle
Once a cycle is detected, how do we find the first node of the cycle?
When slow and fast pointers meet, we stop.
Then, we take a new pointer (entry) at the head and move both slow and entry one step at a time.
The node where they meet is the start of the cycle.
🔍 Why does this work?
The distance from head to cycle start is the same as from meeting point to cycle start.
Code to Find the Cycle Start Node
function findCycleStart(head) {
let slow = head, fast = head, entry = head;
while (fast !== null && fast.next !== null) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) { // Cycle detected
while (entry !== slow) {
entry = entry.next;
slow = slow.next;
}
return entry; // Start of cycle
}
}
return null; // No cycle
}
// Example Usage
console.log(findCycleStart(node1).value); // Output: 2 (cycle starts at node2)
Time and Space Complexity
Time Complexity: O(n) (since each pointer moves at most n times).
Space Complexity: O(1) (no extra memory used).
Summary
Floyd’s Algorithm is used in real-world applications like networking, memory management, blockchain, and database deadlocks.
It detects cycles efficiently using slow and fast pointers.
Once a cycle is detected, a new pointer from the head can help find the cycle's starting node.
🚀 Mastering this will help you in interviews at companies like Google, Amazon, and Microsoft! Let me know if you have any questions. 😊
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
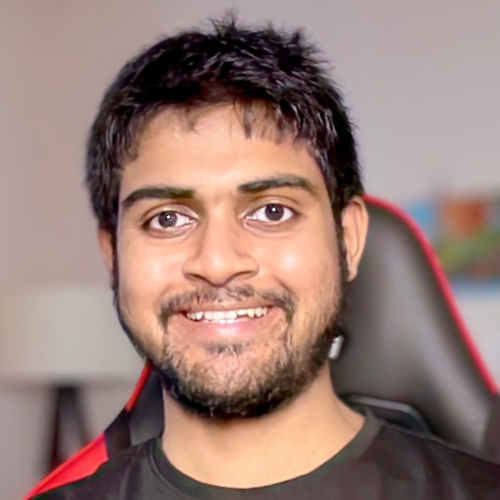
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.