Angular Basic Overview

Table of contents
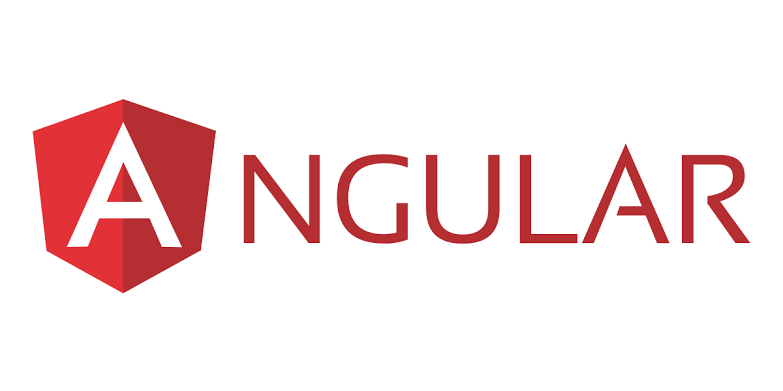
Angular is a popular open-source web application framework maintained by Google, used for building single-page client applications using HTML and TypeScript. Here's a quick overview of its key features and concepts:
Key Features:
Component-Based Architecture: Angular applications are structured as a tree of components, each with its own HTML template, CSS styles, and TypeScript logic.
Two-Way Data Binding
Two-way data binding is a powerful feature in Angular that allows for automatic synchronization between the data model and the user interface. This means that any changes made to the user interface elements, such as input fields, are immediately reflected in the underlying data model, and vice versa. This seamless connection ensures that the view and the model remain in sync at all times.
In practice, two-way data binding is achieved using Angular's
ngModel
directive. This directive binds the form controls in the template to properties in the component's class, allowing for real-time updates. For instance, when a user types into an input box, the corresponding property in the component class is updated instantly. Similarly, if the property value changes programmatically, the input box reflects this change without any additional code.Dependency Injection (DI): Angular's built-in DI system provides components with their dependencies, improving modularity and testability.
Directives
Directives in Angular are a core feature that allows developers to extend the HTML vocabulary and create custom behavior in their applications. They are special markers on a DOM element that tell Angular's HTML compiler to attach a specified behavior to that element or even transform the DOM element and its children. There are three main types of directives in Angular:
Component Directives: These are the most common directives and are used to create components. A component directive has a template, which is a piece of HTML that can be reused across the application. It also includes logic written in TypeScript, which defines the behavior of the component. Components are the building blocks of Angular applications and are used to encapsulate the functionality and presentation of a part of the user interface.
Structural Directives: These directives change the structure of the DOM by adding or removing elements. The most common structural directives are
*ngIf
,*ngFor
, and*ngSwitch
. For example,*ngIf
conditionally includes a template based on the value of an expression,*ngFor
repeats a template for each item in a collection, and*ngSwitch
switches between alternate views based on a value.Attribute Directives: These directives change the appearance or behavior of an existing element. Unlike structural directives, they do not change the DOM structure. Instead, they can modify the element's style, class, or other attributes. A common example is
ngClass
, which adds and removes a set of CSS classes.
By using directives, developers can create highly reusable and maintainable code. They allow for the separation of concerns, where the logic and presentation are kept distinct, making the application easier to understand and manage. Directives also enable the creation of complex user interfaces with minimal code, enhancing both the functionality and performance of Angular applications.: Special markers in templates that tell Angular to alter the DOM. Examples include structural directives (like *ngIf
and *ngFor
) and attribute directives (like [ngClass]
and [ngStyle]
).
Services and Dependency Injection: Encapsulate business logic and data retrieval, shared across multiple components.
Routing: Angular's
RouterModule
allows navigation between different views or pages, managing URL paths and route parameters.Forms Handling: Supports both template-driven and reactive forms for handling user inputs and validations.
RxJS and Observables
RxJS, or Reactive Extensions for JavaScript, is a library that enables developers to work with asynchronous data streams in a more manageable and efficient way. It provides a powerful set of operators that allow developers to compose and manipulate data streams with ease. Observables, a core feature of RxJS, represent a collection of future values or events. They are similar to promises but are more versatile, as they can handle multiple values over time, rather than just a single value or error.
In Angular, Observables are used extensively to handle asynchronous operations, such as HTTP requests, user input events, and other data streams. This approach allows developers to write cleaner and more concise code, as they can easily subscribe to these data streams and react to changes in real-time. The use of Observables also promotes a reactive programming style, where components can automatically update in response to changes in the data, leading to more dynamic and responsive applications.
Moreover, RxJS provides a wide range of operators, such as map, filter, and merge, which can be used to transform and combine data streams in various ways. This flexibility makes it easier to implement complex data handling logic without resorting to cumbersome and error-prone imperative code. Overall, RxJS and Observables are essential tools in the Angular ecosystem, enabling developers to build robust, scalable, and high-performance applications.: Provides a robust way to manage asynchronous data streams, enabling powerful reactive programming patterns.
Basic Angular Application Setup:
Installation:
Install Angular CLI:
npm install -g @angular/cli
Create a new Angular project:
ng new project-name
Navigate to the project directory:
cd project-name
Serve the application:
ng serve
Creating a Component:
ng generate component component-name
Creating a Service:
ng generate service service-name
Services are used to encapsulate business logic and can be injected into components using DI.
Routing: Add routes in
app-routing.module.ts
:const routes: Routes =[
{ path: 'path-name', component: ComponentName },
{ path: '', redirectTo: '/default-path', pathMatch: 'full' }, ];
Subscribe to my newsletter
Read articles from CWB directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
