Clean Code - Part-2: Comments
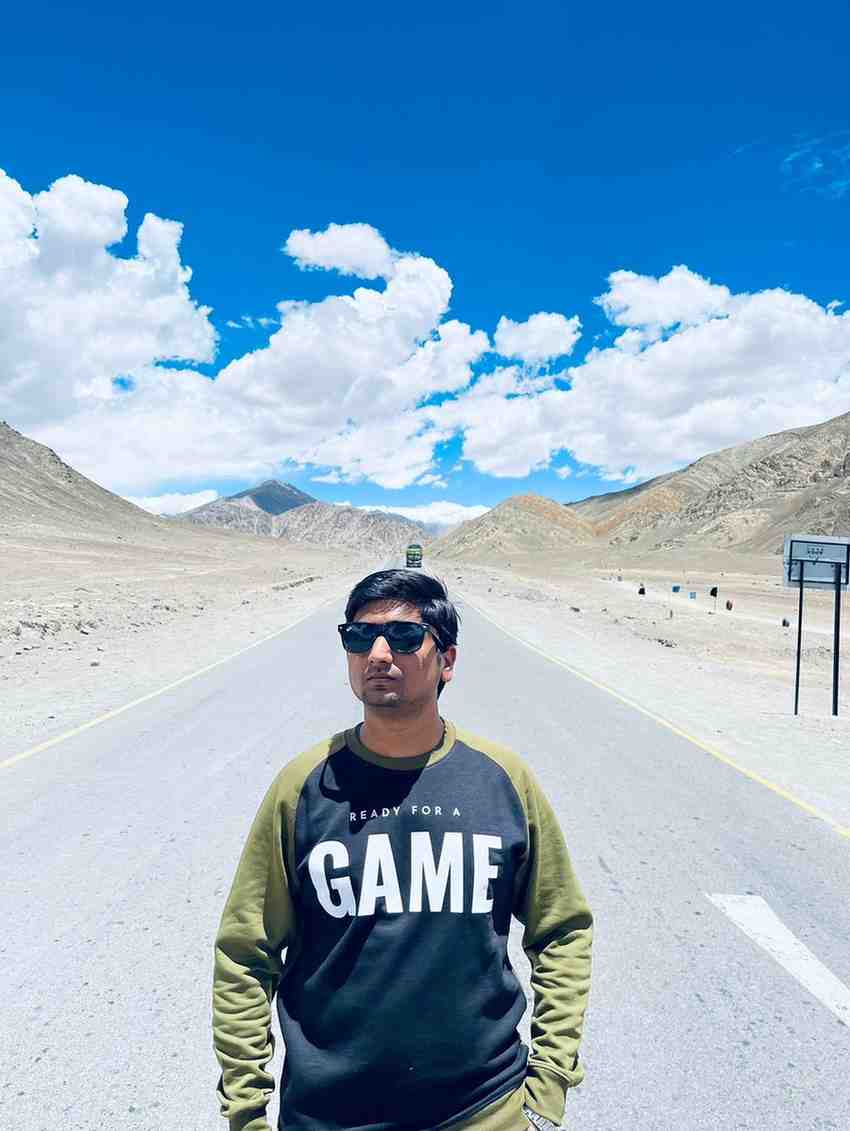
Table of contents
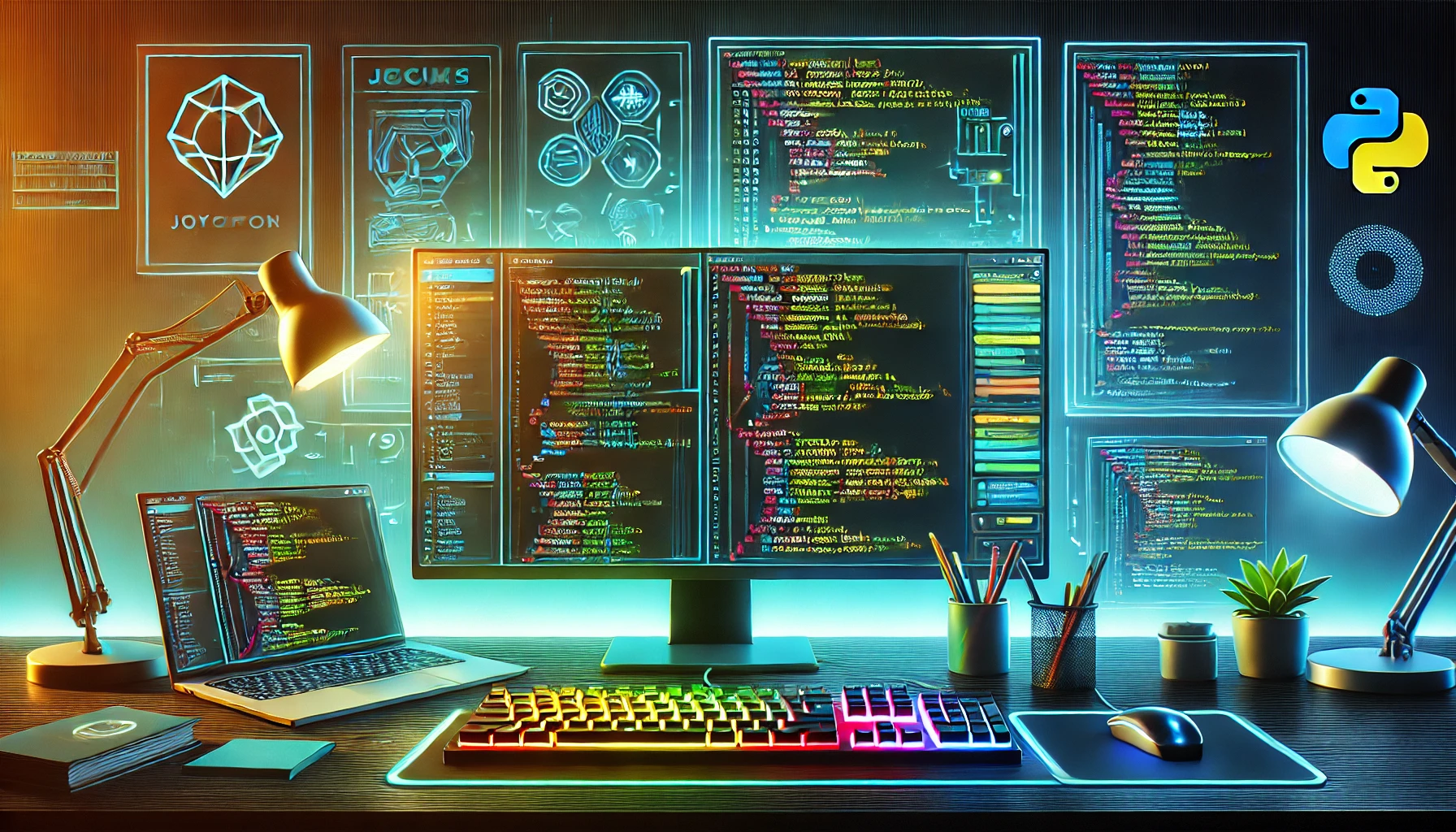
Our first priority should be that we shouldn't need to write comments in our code. The code should be clean enough (i.e. naming conventions, formatting, functions, etc) that it should self explain when looked at. But there are some cases where we might wanna consider writing comments.
Implementing the principles of good and bad comments in code requires careful consideration of clarity, necessity, and maintainability.
When writing code, it's best to make it easy to understand without needing lots of comments. This means using clear names for things and organizing the code in a logical way. Comments should only be used when necessary, like when something isn't obvious from the code alone.
Poor Comments
Redundant Comments:-
# Increment x by 1 x += 1
Comments like this are redundant because the code itself is self-explanatory and adding a comment doesn't provide any additional value.
Misleading Comments:-
# Calculate average of numbers total = sum(numbers)
Misleading comments can confuse other developers and lead to misunderstandings about the code's functionality. Like the above example has a comment that says 'Calculate average of numbers'. But actually, it's summing the numbers, not calculating the average.
Commented-out Code:-
# x = 10 # This line is commented out because we're not sure if we still need it.
Leaving commented-out code in the source can clutter the codebase and make it difficult to understand the active code.
Good Comments
Legal Information:-
# Copyright (C) 2024 CompanyXYZ. All rights reserved.
This type of comment provides important legal information about the code's ownership and usage rights.
Explanations which can't be replaced by naming convention:-
# Explanation: Using a custom sorting algorithm due to specific requirements. def custom_sort(items): # Custom sorting algorithm implementation pass
In this case, the comment explains why a custom sorting algorithm is being used instead of a built-in sorting function. This explanation provides valuable context that cannot be conveyed solely through the function name or code itself.
Warnings:-
# WARNING: This function modifies the global variable 'counter'. def increment_counter(): global counter counter += 1 return counter
In this example, the comment warns other developers that the function
increment_counter()
modifies a global variable namedcounter
. This information is crucial for understanding the potential side effects of using this function.Todo:-
# TODO: Implement error handling for invalid input. def parse_input(input_str): # Code for parsing input goes here pass
Here, the comment indicates that the function
parse_input()
lacks error handling for invalid input. This serves as a reminder to implement this functionality in the future.Documentation Comments:-
""" This function calculates the area of a rectangle. :param length: The length of the rectangle. :param width: The width of the rectangle. :return: The area of the rectangle. """ def calculate_rectangle_area(length, width): return length * width
Documentation comments provide comprehensive documentation for functions, classes, or modules, enhancing code readability and maintainability.
In short, good code speaks for itself. By keeping things clear and organized, we can avoid the need for too many comments. While comments have their place, we should aim for code that's easy to understand without them. If used, comments should add value by providing necessary context, warnings, or documentation, rather than stating the obvious or cluttering the code base with redundant or misleading information.
Subscribe to my newsletter
Read articles from Saurabh Mahajan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
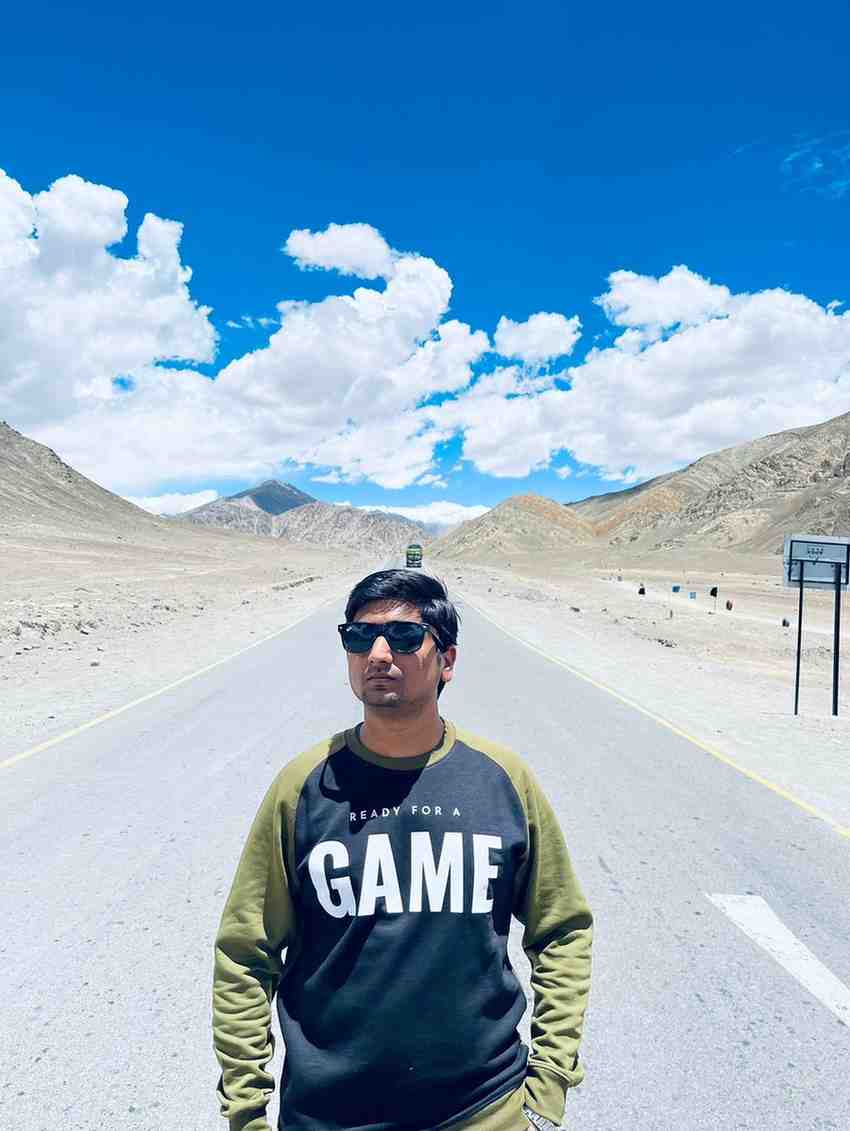
Saurabh Mahajan
Saurabh Mahajan
Greetings! 🚀 I'm a dynamic Fullstack Software Engineer, driving advancements in technology to empower organizations globally. Proficient in a diverse tech stack, I contribute to the architecture and development of high-performance applications. Beyond coding, I thrive on creating empowering and collaborative environments, championing technological innovation, and fostering connections to push the boundaries of excellence. As a Gamer🎮, and a Footballer⚽️, I bring passion to both my professional and personal pursuits. A Wanderlust🛣️ enthusiast and Orophile⛰️, I thrive on exploring diverse landscapes. A Melomane🎵 and avid TV😍 enthusiast, I find joy in the finer aspects of life. Also, a proud enthusiast of Tea☕️ who appreciates bike rides, beaches, and mountains alike. Always eager to connect with like-minded professionals and individuals who share a zest for technology, innovation, and life's adventures. Let's push boundaries together! 🌐