Clean Code - Part-3: Code Formatting
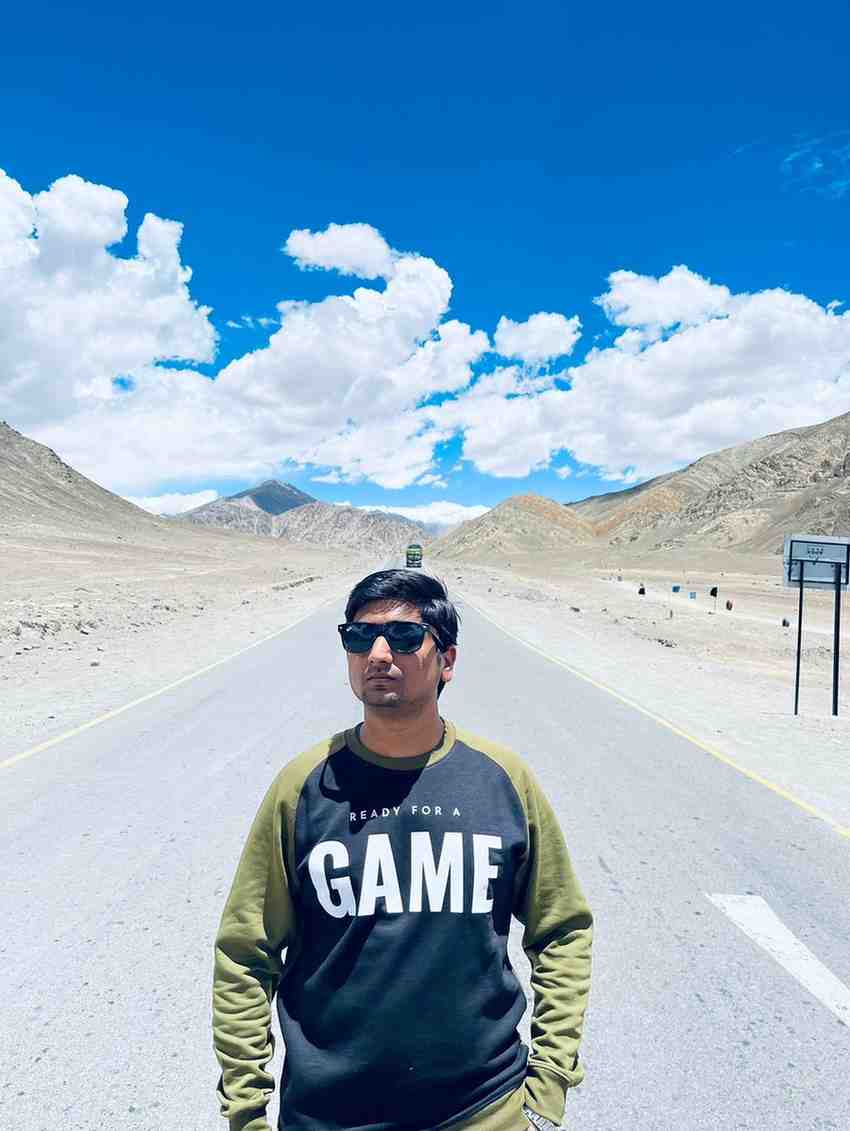
Table of contents
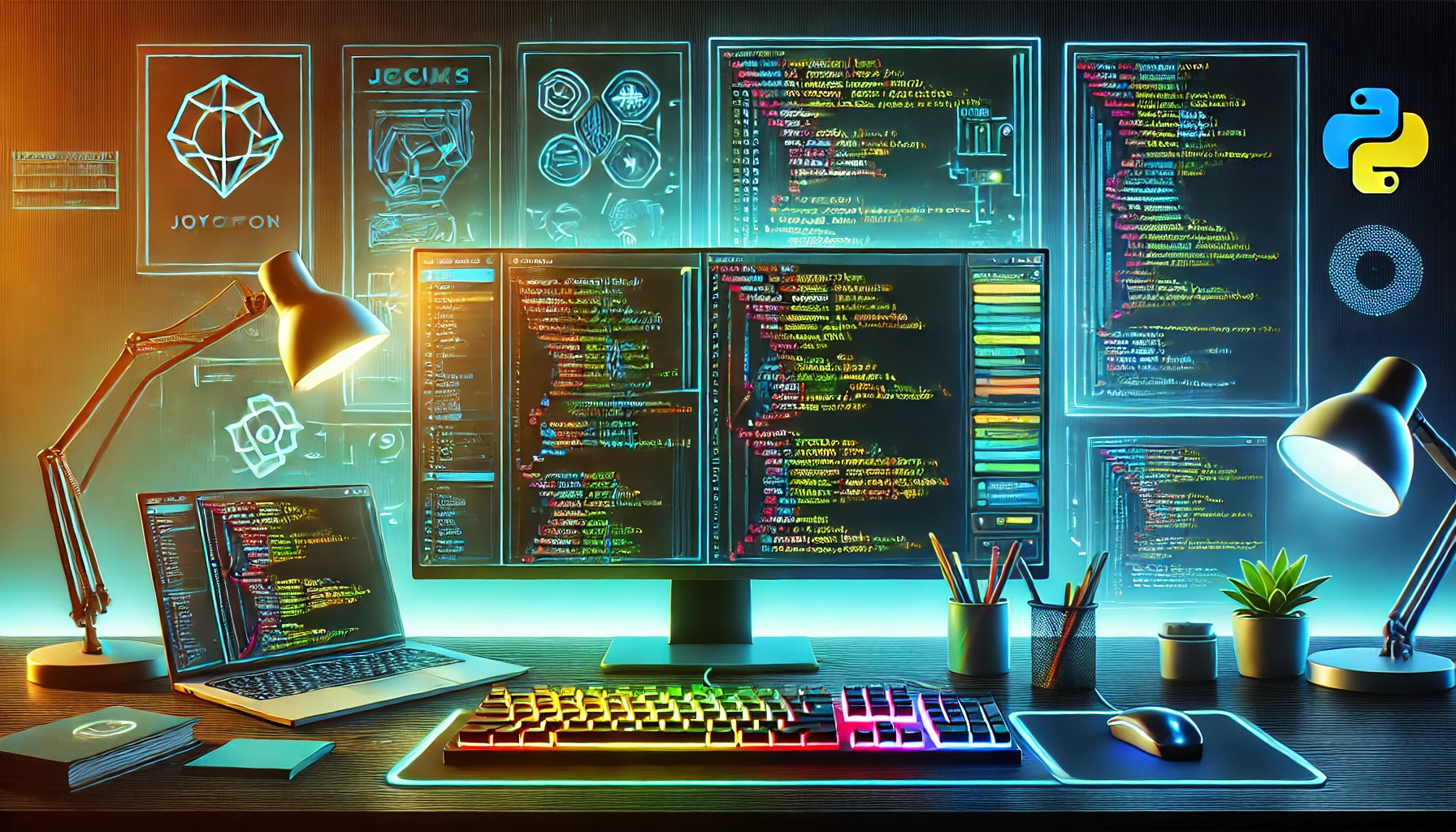
Code formatting is the practice of structuring code in a consistent and organized manner to enhance readability and convey meaning effectively.
Vertical Formatting
Vertical formatting concerns how code is organized from top to bottom, focusing on readability and logical grouping.
Readability like an essay:-
Code should be structured in a way that allows for smooth reading from top to bottom, without unnecessary jumps or disruptions. This ensures that the code flows logically and is easy to follow.
# Bad vertical formatting def main(): print("Hello,") print("world!") # Good vertical formatting def main(): print("Hello,") print("world!")
Separating Different Concepts:-
Different concepts or sections of code should be separated by spacing to visually distinguish them. This improves readability by clearly indicating transitions between different parts of the code.
# Bad: No spacing between different concepts def process_data(): # code for processing data def display_results(): # code for displaying results # Good: Separating different concepts with spacing def process_data(): # code for processing data # Spacer def display_results(): # code for displaying results
Related Concepts Close Together:-
Code that is related or serves a similar purpose should be kept close together. This makes it easier for developers to understand the context and relationship between different parts of the code.
# Bad: Related concepts separated by unrelated code def calculate_area(): # code for calculating area print("Results:") def display_results(): # code for displaying results # Good: Related concepts grouped together def calculate_area(): # code for calculating area def display_results(): # code for displaying results print("Results:")
Horizontal Formatting
Horizontal formatting refers to how code is structured within a line, focusing on indentation, line breaks, and spacing.
Indentation:-
Indentation is crucial for readability and code organization. Even if not strictly required by the language syntax, consistent indentation enhances readability by visually representing the structure of the code.
# Bad: Inconsistent indentation def main(): print("Hello, world!") # Good: Consistent indentation def main(): print("Hello, world!")
Breaking Long Statements:-
Long statements should be broken into multiple shorter ones to improve readability and prevent excessively long lines of code. This enhances clarity and makes it easier for developers to understand each part of the statement.
# Bad: Long statement without line breaks result = calculate_some_long_expression() + another_long_expression() - \ some_other_long_expression() # Good: Breaking long statement into multiple lines result = (calculate_some_long_expression() + another_long_expression() - some_other_long_expression())
Clear but Not Unreadable Long Names:-
Variable and function names should be clear and descriptive, but not excessively long. This balance ensures that code remains readable while conveying the intent of each identifier.
# Bad: Unreadably long name def calculate_area_of_rectangle_given_length_and_width(length, width): pass # Good: Clear and concise name def calculate_rectangle_area(length, width): pass
Spacing Between Code:-
Proper spacing between elements of code improves readability and helps distinguish different parts of the code. Consistent use of spacing enhances clarity and makes the code easier to follow.
# Bad: Lack of spacing between code elements def main(): result=calculate_area_of_rectangle_given_length_and_width(5,10) # Good: Consistent spacing between code elements def main(): result = calculate_rectangle_area(5, 10)
Line Width:-
Lines of code should ideally be kept within a reasonable width to prevent horizontal scrolling and maintain readability. While there's no strict rule, a common guideline is to limit lines to around 80 characters.
# Bad: Exceeding recommended line width result = calculate_some_long_expression() + another_long_expression() - some_other_long_expression() # Good: Keeping line width within recommended limit result = (calculate_some_long_expression() + another_long_expression() - some_other_long_expression())
Different programming languages may have their own conventions and guidelines for code formatting. It's essential to follow these conventions to ensure consistency and readability within the specific language ecosystem.
In summary, code formatting plays a crucial role in improving readability and conveying meaning within the code base. By adhering to principles of vertical and horizontal formatting, and following language-specific conventions, developers can create code that is easier to understand, maintain, and collaborate on.
Subscribe to my newsletter
Read articles from Saurabh Mahajan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
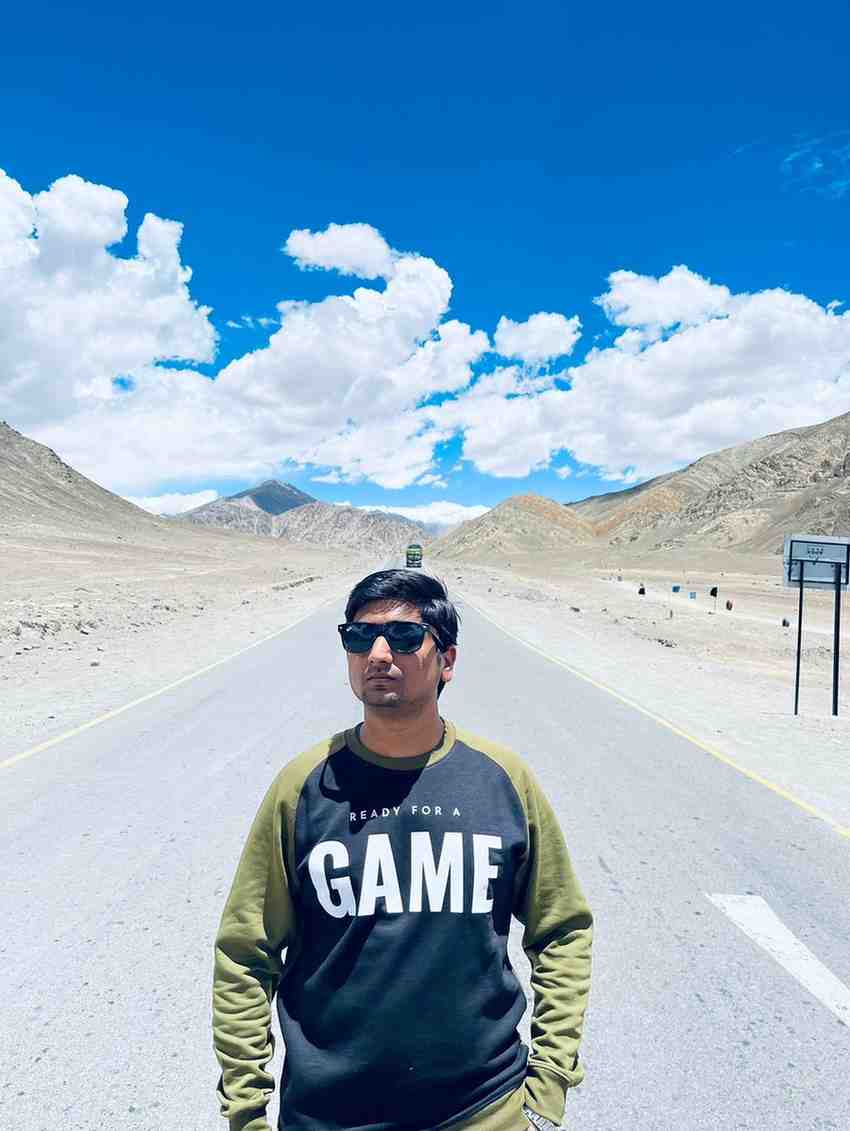
Saurabh Mahajan
Saurabh Mahajan
Greetings! 🚀 I'm a dynamic Fullstack Software Engineer, driving advancements in technology to empower organizations globally. Proficient in a diverse tech stack, I contribute to the architecture and development of high-performance applications. Beyond coding, I thrive on creating empowering and collaborative environments, championing technological innovation, and fostering connections to push the boundaries of excellence. As a Gamer🎮, and a Footballer⚽️, I bring passion to both my professional and personal pursuits. A Wanderlust🛣️ enthusiast and Orophile⛰️, I thrive on exploring diverse landscapes. A Melomane🎵 and avid TV😍 enthusiast, I find joy in the finer aspects of life. Also, a proud enthusiast of Tea☕️ who appreciates bike rides, beaches, and mountains alike. Always eager to connect with like-minded professionals and individuals who share a zest for technology, innovation, and life's adventures. Let's push boundaries together! 🌐