Clean Code - Part-5: Control Structure & Errors
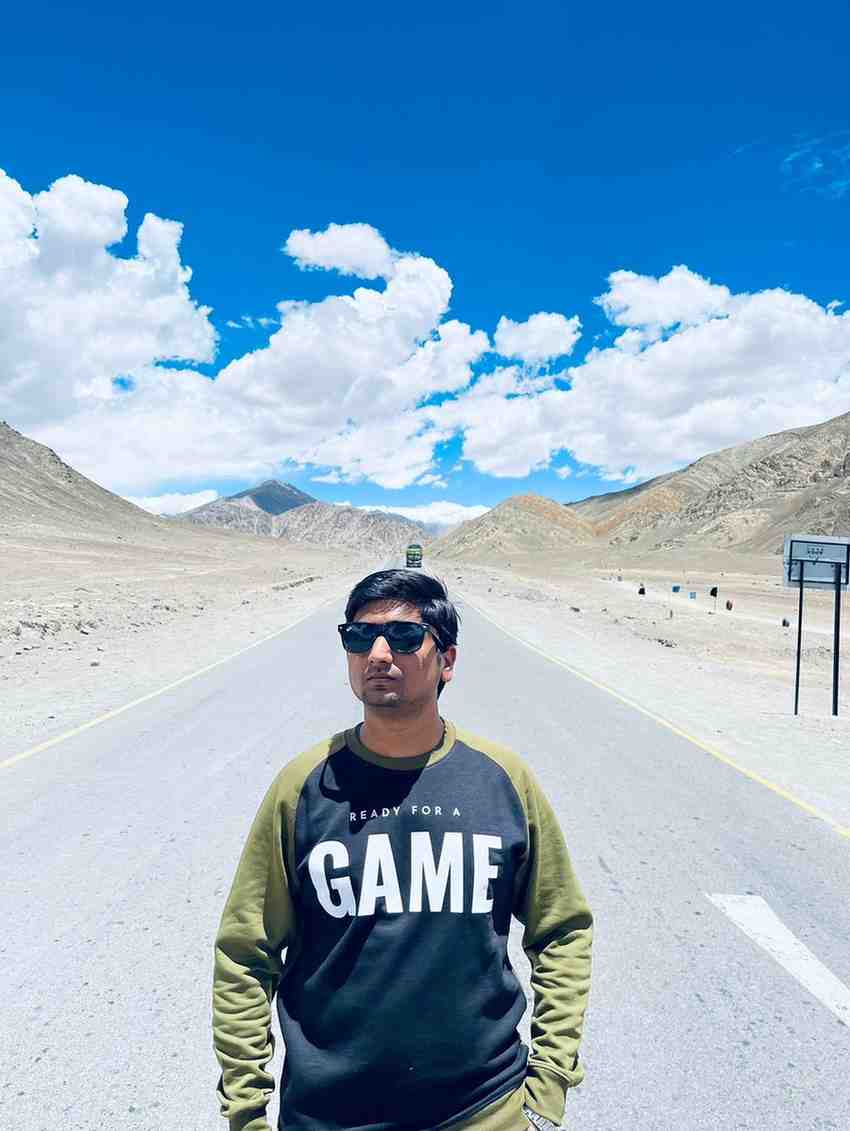

In this section, we'll delve into essential strategies for code refinement, including avoiding deep nesting, preferring positive checks, utilizing errors, leveraging factory functions and polymorphism, and modularizing code. By understanding and applying these principles, developers can enhance code readability, reduce complexity, and facilitate future maintenance and scalability.
Avoid Deep Nesting
Deeply nested code can be challenging to read, understand, and maintain. To mitigate this issue, it's advisable to use guards and fail-fast mechanisms. Guards are conditions placed at the beginning of a function or block of code to check for invalid inputs or conditions. If these conditions are met, the function exits early or raises an error. This approach helps reduce the level of nesting and improves code readability.
Example with Guards:
def calculate_total_price(items):
if not items:
return 0 # Guard clause to handle empty list
total_price = 0
for item in items:
if item['price'] <= 0:
continue # Guard clause to skip items with non-positive price
total_price += item['price']
return total_price
In this example, the guard clause if not items
checks if the list of items is empty, exiting the function early if it is. Inside the loop, another guard clause if item['price'] <= 0
skips items with non-positive prices, reducing the nesting level.
Prefer Positive Checks
Positive checks are generally easier to understand than negative ones because they express what is expected rather than what is not. It's recommended to extract control structures into functions with positive phrasing to improve code readability. Additionally, using clean function rules and inverting conditional logic can further enhance code clarity.
Example with Positive Check:
def is_valid_age(age):
return age >= 18 # Positive check for age validity
if is_valid_age(user['age']):
print("User is an adult.")
In this example, the function is_valid_age
performs a positive check to determine if the given age is valid (>= 18), making the code more straightforward and self-explanatory.
Utilize Errors
Throwing and handling errors can be an effective alternative to complex if statements, leading to more focused and readable functions. Utilizing try-catch blocks to handle errors gracefully and creating error guards to catch potential issues early in the code can greatly improve code robustness and maintainability.
Example with Error Handling:
def divide(x, y):
if y == 0:
raise ValueError("Cannot divide by zero")
return x / y
try:
result = divide(10, 0)
except ValueError as e:
print("Error:", e)
Here, the divide
function throws a ValueError
if the divisor is zero, and this error is caught and handled using a try-except block, providing a clear indication of what went wrong.
Use Factory Functions & Polymorphism
Factory functions can dynamically create objects or functions based on input parameters, contributing to cleaner control structures. By embracing polymorphism, methods with the same name can exhibit different behaviors based on input parameters, promoting modularity and extensibility.
Example with Factory Function:
def create_operation(type):
if type == 'add':
return lambda x, y: x + y
elif type == 'subtract':
return lambda x, y: x - y
add_operation = create_operation('add')
subtract_operation = create_operation('subtract')
result1 = add_operation(5, 3)
result2 = subtract_operation(5, 3)
In this example, the create_operation
factory function dynamically creates different operations (addition or subtraction) based on the input type, enhancing code modularity and readability.
Modularize Code
Modularizing code involves organizing related functions into separate files or modules, promoting better code organization and maintainability. By grouping functions logically and importing them as needed, you can create a cleaner and more manageable codebase.
Example of Modularization:
# File: math_operations.py
def add(x, y):
return x + y
def subtract(x, y):
return x - y
# File: main.py
import math_operations
result1 = math_operations.add(5, 3)
result2 = math_operations.subtract(5, 3)
Here, the math operations are modularized into a separate module (math_
operations.py
), which is then imported into the main file (main.py
), enhancing code organization and readability.
Summary
In conclusion, mastering control structures and error handling techniques is essential for writing clean, maintainable, and scalable code. By applying the principles outlined in this guide—avoiding deep nesting, preferring positive checks, utilizing errors, leveraging factory functions and polymorphism, and modularizing code—developers can enhance code readability, reduce complexity, and future-proof their applications. With a commitment to smart coding practices and continuous improvement, developers can unlock the full potential of their software projects and deliver exceptional value to their users and stakeholders.
Subscribe to my newsletter
Read articles from Saurabh Mahajan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
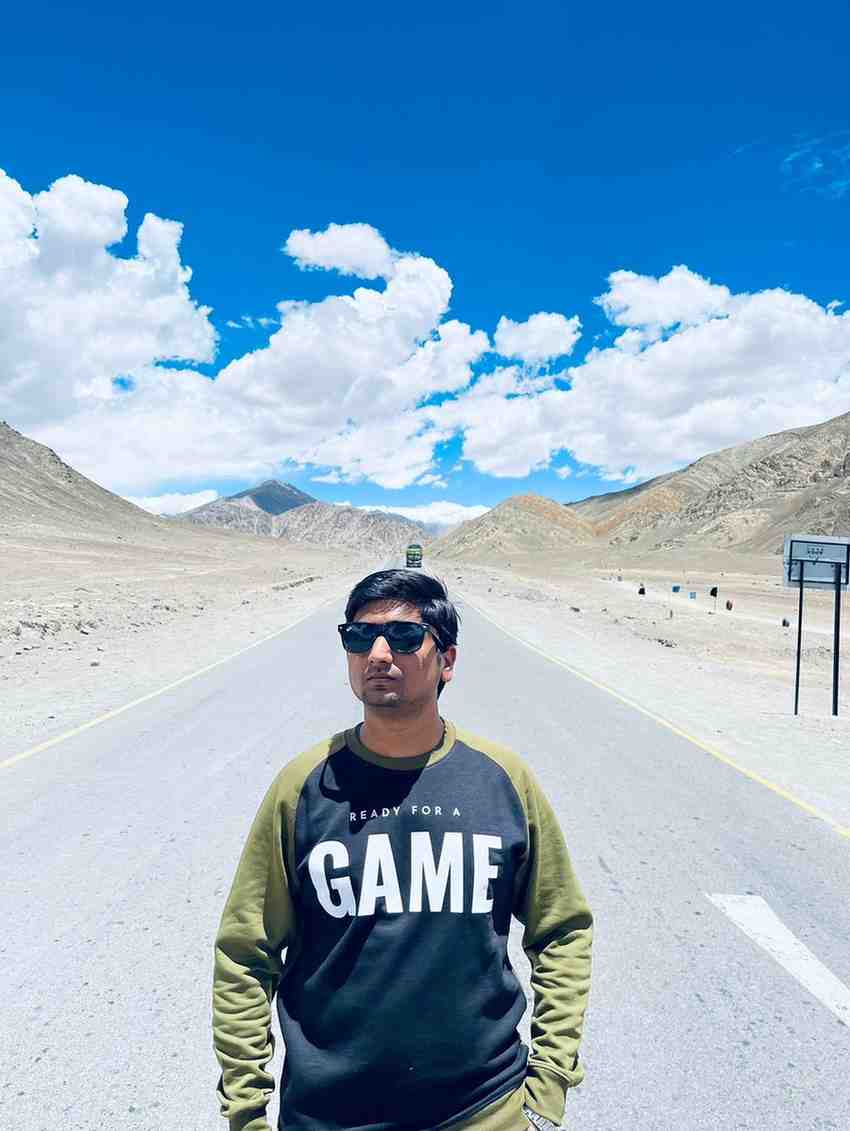
Saurabh Mahajan
Saurabh Mahajan
Greetings! 🚀 I'm a dynamic Fullstack Software Engineer, driving advancements in technology to empower organizations globally. Proficient in a diverse tech stack, I contribute to the architecture and development of high-performance applications. Beyond coding, I thrive on creating empowering and collaborative environments, championing technological innovation, and fostering connections to push the boundaries of excellence. As a Gamer🎮, and a Footballer⚽️, I bring passion to both my professional and personal pursuits. A Wanderlust🛣️ enthusiast and Orophile⛰️, I thrive on exploring diverse landscapes. A Melomane🎵 and avid TV😍 enthusiast, I find joy in the finer aspects of life. Also, a proud enthusiast of Tea☕️ who appreciates bike rides, beaches, and mountains alike. Always eager to connect with like-minded professionals and individuals who share a zest for technology, innovation, and life's adventures. Let's push boundaries together! 🌐